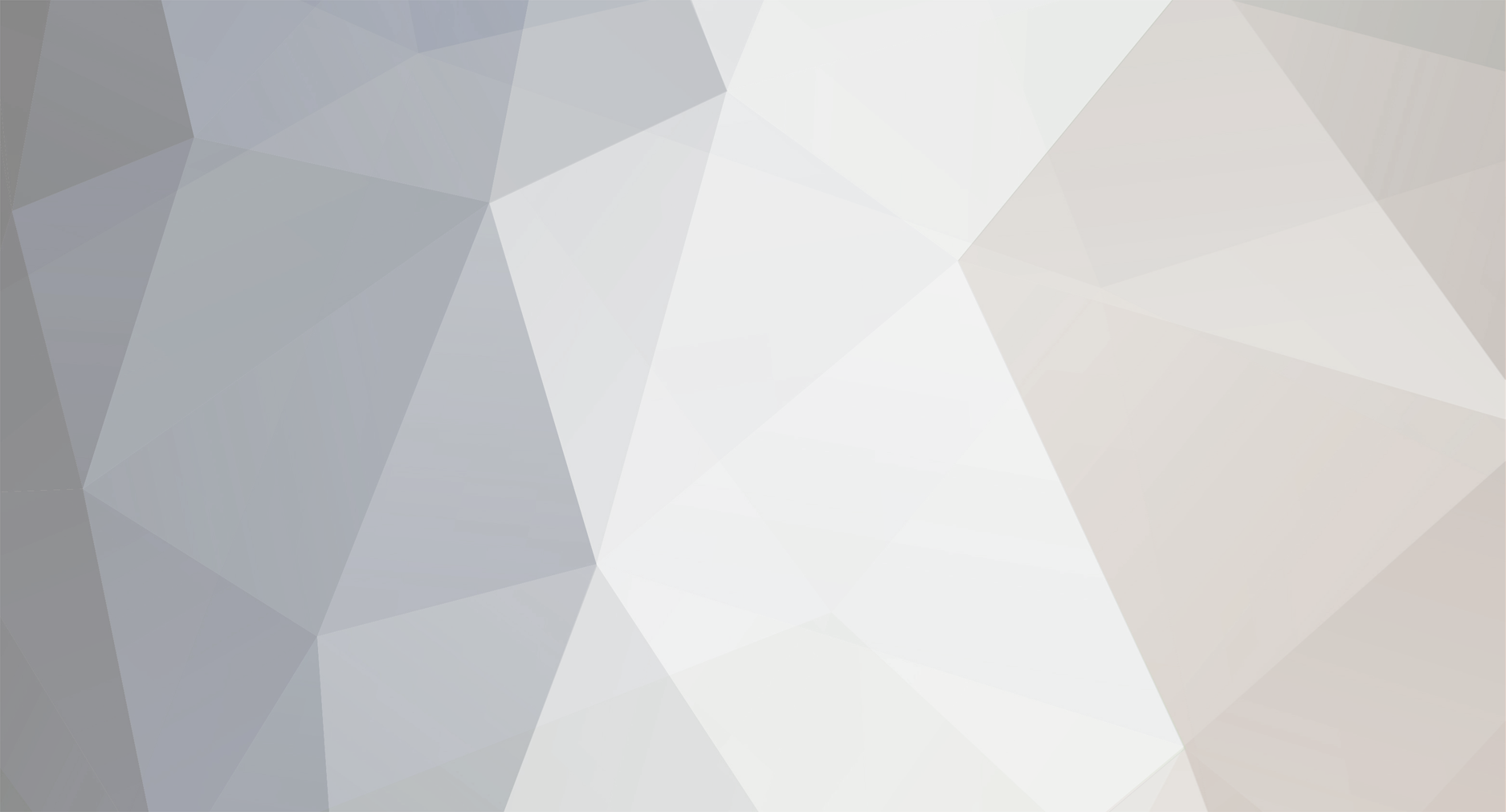
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Change Field Name to Current Date in MySQL DB
DavidAM replied to anonymoose's topic in PHP Coding Help
While, Gizmola may have been harsher than I would have been, his statements are 100% accurate. Any time you find yourself trying to alter a table definition in the middle of an application, you have a poorly designed database. It is vital to stop and reconsider the design before too much effort is wasted on coding. Make no mistake, any effort spent on coding to a poor database design is wasted. At some point, in the not too distant future -- and usually before the application is even finished -- it will all have to be re-done. This is always another way to do it. But not all of the ways are valid, or even maintainable. Never in a million years!! The database design you started with would likely be less efficient than using index cards in a card file. I too believe in sharing solutions. The problem with your original code: $mydatevar = $yearvalue . "_" . $monthvalue . "_" . $dayvalue; $result=mysql_query("ALTER TABLE mytable CHANGE price `$mydatevar' VARCHAR(30)") or die(mysql_error()); and the reason the new code "works": $today = date("mdY"); mysql_query("ALTER TABLE cookies CHANGE CurPrice `$today` VARCHAR(10)") or die(mysql_error()); is that $yearvalue, $monthvalue, and $dayvalue were never defined. Therefore, $mydatevar was being set to "__" (two underscores) which is exactly what you said you were getting. So, the code did work - in that it was doing exactly what you told it to do. As to your original database design, did anybody ever give any consideration to how you would write the queries to get the data or summarize the data? Did anyone ever consider the unnecessary load you would be putting on the database server? If my memory serves me correctly, an ALTER TABLE statement completely copies and rebuilds the table. Since you said you were renaming the "Price" column every day, presumably, you were ADDing the Price column in some code somewhere. So, ALTER TABLE ... ADD COLUMN ... ... ALTER TABLE ... (rename column) -- that's TWO copy and rebuilds of the entire table. Every Day! Edit:Actually, three rebuilds, since you are also dropping the oldest column every day. Did anybody ever consider using NUMERIC or DECIMAL (or even INTEGER) for the datatype? Using VARCHAR for a Price column is another terrible idea. This will completely prevent you from manipulating the data in SQL in any way -- SELECT MAX(), MIN(), AVERAGE() will NOT provide accurate results on numbers stored as VARCHAR. In the design suggested by TeNDoLLA, it is a simple matter to get the lowest, highest or average value over a range of dates (one-week, two-weeks, three-days, whatever). To get that same information from your suggested design, would mean building a new query using dates to build column names. By the way, what are you going to do when you get to work one day and find out that the process failed TWO days ago? Your table will be missing a column. If you run it now and it uses the current date, it will put the wrong date as a column name. Then when the process runs tonight, it will fail again because the column name already exists. There's a reason we call it "database design". It needs to be designed, and all of its currently known uses need to be considered, and potential future questions need to be considered. What happens in the original design when the boss walks in your office and says, "I need to see the trend of pricing over the past month"? You don't even have the data, and your table structure will not support it until you rewrite code to allow one month of data as column names instead of two weeks. In the suggested design, you can keep the data longer than the boss suggests so you have it when he later asks for it. Then you just change the date range on the query. In my experience, one of the first questions the boss asks when an application is "finished" is directly contrary to the requirements he gave when you started building the system. -
session_destroy destroys the entire session, not an individual variable stored in the session. To remove a variable from a session you can just unset it: if(isset($_POST['businsert_x'])){ unset($_SESSION['busID'], $_SESSION['pap']); Most likely this code has always been throwing an error. Your error_reporting value was probably set in such a way as to prevent the errors from being displayed.
-
Cannot understand what is wrong with mod_rewrite
DavidAM replied to Dantonybrown's topic in Apache HTTP Server
Is this a cut-and-paste, or did you type it? There is a missing period: Options +FollowSymLinks Options +Indexes RewriteEngine On RewriteRule ^English-Lighthouses/(.*)$ English-Lighthouses-Page.php?Lighthouse=$1 #---------------------------------^ Right There Otherwise, it could be an issue with the directory name. Is this at your DOCUMENT ROOT or is it in a sub-directory? I'm not a genius at this, so if the missing period is not the problem, you might post your directory structure so someone with more mod_rewrite experience can take a swing at it. -
Something like this should be close: SELECT wordlist.id, wordlist.term FROM wordlist JOIN is_reviewed ON wordlist.id = is_reviewed.wordlist_id WHERE is_reviewed.is_reviewed = 1 GROUP BY wordlist.id, wordlist.term HAVING COUNT(is_reviewed.id_user) = (SELECT COUNT(id) FROM users WHERE is_reviewer = 1) I have not tested this code -- I don't have a test environment here. This should return the wordlist entries that have the same number of is_reviewed as there are reviewers.
-
Then we have to wrap them in quotes: $sql = "SELECT ProfileID, GROUP_CONCAT(Interest SEPARATOR ',') AS Interests FROM table WHERE ProfileID IN (" . implode(",", $profile_id_array) . ") AND Interest IN ('" . implode("', '", $interest_array) . "') GROUP BY ProfileID HAVING COUNT(*) = " . count($interest_array);
-
If you mean how do you get them into the query from PHP (I'm assuming they are integers) $sql = "SELECT ProfileID FROM table WHERE ProfileID IN (" . implode(",", $profile_id_array) . ") AND Interest IN (" . implode(",", $interest_array) . ") GROUP BY ProfileID HAVING COUNT(*) = " . count($interest_array); If you mean, how do you get them as a column returned by the query ... $sql = "SELECT ProfileID, GROUP_CONCAT(Interest SEPARATOR ',') AS Interests FROM table WHERE ProfileID IN (" . implode(",", $profile_id_array) . ") AND Interest IN (" . implode(",", $interest_array) . ") GROUP BY ProfileID HAVING COUNT(*) = " . count($interest_array); I guess that second statement is redundant since they will be the same as provided in the query.
-
split() returns an array of values based on the pattern. list() assigns array values to scalar variables. // This line of code ... list($name,$shout) = split("\|\|\|\|\|",$row); // ... is equivalent to ... $ar = split("\|\|\|\|\|",$row); $name = $ar[0]; $shout = $ar[1]; "Undefined offset 1 ..." indicates that the returned array contains a single value (which is element zero) and $ar[1] does not exist. The manual for split says this will happen if the pattern is not found or the string is empty. split() will return false if it encounters an error (in that case offset 0 (zero) will be undefined). So, in this case, the variable $row is empty or does not contain the pattern. Note: The manual says this function is deprecated. You should use preg_split or explode instead.
-
mySql is a Relational database, it is not sequential. There is no concept of the "latest row". In theory, when you do not specify an ORDER BY, the server is free to return the rows in any order it wants, and that order could be different at different times. So, in order to answer your question, we need to know how you define "the latest row" in this particular table. By the way, SELECT * DISTINCT ... is invalid syntax, that syntax should be SELECT DISTINCT * .... Note: this will NOT solve your problem. I suspect the solution is going to require a subquery. Perhaps something along the lines of SELECT * FROM myTable WHERE ID = (SELECT MAX(ID) FROM myTable). But until we know how to determine the "latest row" (and possibly the table structure), we can't be sure of the solution.
-
mySql's implementation of triggers does NOT allow the trigger to touch the table that fired the trigger. Since triggers were invented to aid with data integrity, this is (in my opinion) a serious limitation. The best solution, (again, my opinion) is to create a stored procedure or function that will update the "current" row and insert the new one. Having said that, let me say that your triggers are messed up. Your AFTER insert is updating all rows where End = 0, this would update the row you just inserted as well. You would need to do that update BEFORE the insert. Also, there is the possibility that the NOW() value in BEFORE would be different than the NOW() value in AFTER. Without knowing the application, I don't know if this is significant or not. Actually, I'm not sure if NOW() changes during trigger/procedure execution, so that last statement might not be correct. Also, watch out, END is (I believe) a reserved word. I would choose something different to prevent problems there (perhaps StartTIme and EndTIme). Having said all that, I question whether that database design is optimal. Is it necessary to have an EndTime in a record? You could always get the current record by selecting for the MAX StartTime. You could determine the previous record by looking at the previous ID (not the most accepted way to do it) or the MAX StartTime that is LESS THAN the "current" record's StartTime.
-
In the context you are using, a subquery can return only ONE column. You are returning multiple columns in each of the subqueries. Also the last subquery needs to use IN instead of equals (=). The query should be something like this: $sql = "SELECT ump_id FROM ump_names WHERE `$what_sport` = '1' AND ump_id NOT IN (SELECT ump_id FROM bad_school WHERE `sport` = '$what_sport' AND `school` = '$home_team') AND ump_id NOT IN (SELECT ump_id FROM days_off WHERE `day` = '$day_of_game') AND ump_id NOT IN (SELECT ump_id FROM scheduled_umps WHERE `game_id` IN ('$values') ) "; jcbones beat me to it. But he did not mention the other problem in the last subquery.
-
Two things I would do here: 1) Make sure that country is indexed in the table. If it is not, then that query will have to do a table scan (it will read EVERY row in the table). 2) Let the database engine do all of the work: SELECT COUNT(*) AS CountryCount, SUM(agestamp) AS AgeTotal, SUM(deathstamp) AS DeathTotal FROM stats WHERE country='UK' Then PHP only has to process ONE row of data. This assumes that agestamp and deathstamp are defined as numeric fields (i.e. integer, decimal, etc).
-
You don't want a LEADING comma either
-
Just for the record, player_id can NOT be the PK and can NOT be a Unique Index in the Scores table. There will be multiple scores for any given player. This table could use a composite primary key (player_id and score_date perhaps -- or add the course_id as well) but I usually stick with the auto_increment id.
-
Oops! my mistake. We need to ADD each condition to the $where value. I changed each $where = to $where .=. Sorry about that. $where = ''; $qry = "SELECT * FROM tickets "; if (!empty($var1)) $where .= (empty($where) ? "WHERE " : "OR ") . "ticket_id = '$var1' "; if (!empty($var2)) $where .= (empty($where) ? "WHERE " : "OR ") . "dept_id = '$var2' "; if (!empty($var3)) $where .= (empty($where) ? "WHERE " : "OR ") . "proj_id = '$var3' "; if (!empty($var4)) $where .= (empty($where) ? "WHERE " : "OR ") . "status = '$var4' "; if (!empty($var5)) $where .= (empty($where) ? "WHERE " : "OR ") . "severity = '$var5' "; $qry .= $where; $result=mysql_query($qry) or die(mysql_error());
-
If var1 through var5 are coming from an HTML form, they will most likely NOT be NULL. They are probably empty strings. NULL is a special value and is NOT the same as an empty string. Using the code provided: $qry = "SELECT * FROM tickets WHERE ((ticket_id='$var1') OR ('$var1' IS NULL)) and ((dept_id='$var2') OR ('$var2' IS NULL)) and ((proj_id='$var3') OR ('$var3' IS NULL)) and ((status='$var4') oR ('$var4' IS NULL)) and ((severity='$var5') oR ('$var5' IS NULL))"; and the first example: the resulting SQL will (most likely) be: SELECT * FROM tickets WHERE ((ticket_id='15') OR ('15' IS NULL)) and ((dept_id='') OR ('' IS NULL)) and ((proj_id='') OR ('' IS NULL)) and ((status='') oR ('' IS NULL)) and ((severity='') oR ('' IS NULL)) Which will generally return an empty resultset (because '' is NOT NULL). By looking at the resulting SQL (as suggested by fugix), it is pretty clear what needs to be done: change all of the "IS NULL" conditions to "= '' ". If var2 through var5 are, in fact, NULL, you will get the same SQL. If they are, instead, the string "NULL", then you have to remove the single quotes from around the $var# variables in the php code. However, this will result in errors when any of the values are not NULL. The usual way to resolve this dilemma, is to build the query dynamically, including only the non-empty query parameters. Something along the lines of: $where = ''; $qry = "SELECT * FROM tickets "; if (!empty($var1)) $where = (empty($where) ? "WHERE " : "OR ") . "ticket_id = '$var1' "; if (!empty($var2)) $where = (empty($where) ? "WHERE " : "OR ") . "dept_id = '$var2' "; // and so forth ... $qry .= $where;
-
The data from the query is stored in $row. You have to reference it from there or copy it to another variable: print "<input type=radio name=Poll value=" . $row['poll_answer'] . " id=" . $row['answer_id'] . " />" . $row['poll_answer'] . "";
-
This SQL is not valid. You cannot have a subquery in the SELECT phrase that returns multiple rows. SELECT polls.ID as pollID, polls.question, polls.totalVotes, (SELECT pollAnswers.ID, pollAnswers.answer FROM pollAnswers WHERE pollAnswers.pollID = polls.ID) FROM polls INNER JOIN pollAnswers ON polls.ID = pollAnswers.pollID WHERE polls.statusID = '1' ORDER BY polls.ID DESC LIMIT 1 Since you have LIMIT 1, the WHILE loop is useless, you are only going to have one row to process. I think, in this case, you will have to do two queries. The first query will get the Poll info and the second will get the answer list. Something along these lines: Note: I also rearranged the FORM contents a bit, some of the elements were mixed in with the FIELDSET, and should not have been. function getpoll($dbc) { $query = "SELECT polls.ID as pollID, polls.question, polls.totalVotes, FROM polls WHERE polls.statusID = '1' ORDER BY polls.ID DESC LIMIT 1"; $result = mysqli_query($dbc, $query); if ( ($result) and (mysqli_num_rows($result) > 0) ) { $row = mysqli_fetch_array($result); $pollID = $row['pollID']; $question = $row['question']; $totalVotes = $row['totalVotes']; $query = "SELECT pollAnswers.ID, pollAnswers.answer FROM pollAnswers WHERE pollAnswers.pollID = $pollID"; print "<form action=efedmanager/processes/poll.php method=post name=poll id=poll>"; print "<input type=hidden name=pollID value=".$pollID." />"; print "<fieldset>"; print "<legend>".$question."</legend>"; $result = mysqli_query($dbc, $query); if ( ($result) and (mysqli_num_rows($result) > 0) ) { while ($row = mysqli_fetch_array($result)) { print "<label>"; print "<input type=radio name=Poll value=" . $row['answer'] . " id=" . $row['ID'] . " />" . $row['answer']; print "</label>"; } } print "</fieldset>"; print "<input type=submit name=submit id=submit value=Vote />"; print "</form>"; } else { print "<p class=none>There are currently no open polls."; } } This code is not tested and may contain syntax or logic errors . It is provided as a example of an approach that should work for the stated problem.
-
Your problem is here: if(preg_match("/".$banWord."/i", $checkComment)){ essentially, you are using "/.at/i" as a regular expression. But the period in a regexp means match anything. To match a period, you have to escape it with a backslash. You should have a look at preg_quote. So your statement would look like this: if(preg_match("/" . preg_quote($banWord, '/') . "/i", $checkComment)){
-
Count how many months it is back to November.
DavidAM replied to jakebur01's topic in PHP Coding Help
Oh, you're looking for Previous Year-To-Date (i.e. Nov 2010 thru May 2011, and Nov 2009 thru May 2010). To make it scale, set $prevEnd to $curEnd - 12. Also, it appears that I tested the only two months of the year that that formula works for (May and December). Try this approach to make the calculations independent of the date: $thisMonth = intval(date('m')); $curEnd = 27; // Always the current period $curStart = ($thisMonth <= 11 ? $curEnd - ($thisMonth + 1) : $curEnd - ($thisMonth - 11)); // November $prevEnd = $curEnd - 12;// This month a year ago $prevStart = $curStart - 12; // Previous November You'll want to test that, but I think it is correct. -
See this thread concerning a similar problem. Basically, you select using JUST the ID to find the record. You should use the same WHERE clause on the UPDATE. By trying to match every field in the row, against the NEW data, the update is not finding any rows to update.
-
Why did you start a new topic for the same problem? That's against the rules. To address your problem, I would try moving the onclick to the IMG tag. It may be that the IMG tag is "eatting" the click event so the A tag does not actually see it. Of course, this is just a "shot in the dark".
-
Count how many months it is back to November.
DavidAM replied to jakebur01's topic in PHP Coding Help
How about something like this? $curEnd = 27; // 27 $curStart = 27 - abs(11-date('m')); // 27 - 6 = 21 $prevEnd = $curStart - 1; // 21 - 1 = 20 $prevStart = $prevEnd - 11; // 20 - 11 = 9 $curTotal = 0; $prevTotal = 0; while (odbc_fetch_row($rs)) { for ($col = $curStart; $col <= $curEnd; $col++) { if ($col == 1) $colName = 'SALES_OLDEST_PD1'; elseif($col == 26) $colName = 'SALES_LAST_PD_26'; elseif($col == 27) $colName = 'SALES_CURR_PD_27'; else $colName = 'SALES_PD_' . $col; $curTotal += odbc_result($rs,$colName); } for ($col = $prevStart; $col <= $prevEnd; $col++) { if ($col == 1) $colName = 'SALES_OLDEST_PD1'; elseif($col == 26) $colName = 'SALES_LAST_PD_26'; elseif($col == 27) $colName = 'SALES_CURR_PD_27'; else $colName = 'SALES_PD_' . $col; $prevTotal += odbc_result($rs,$colName); } } -
1) localhost is pretty much a reserved name meaning "myself". If you have the Web server running on Machine1 and you try to access http://localhost on Machine2, Machine2 will be trying to find the webserver on itself. You should (probably) change the virtual server name. 2) If you created an Apache virtual server name on Machine1, you need to tell Machine2 how to find that name. Basically, you need a DNS entry somewhere telling Machine2 that VirtualWeb1 (or whatever) is located at IP address 192.129.92.29 (or whatever). I have always added these entries into the "hosts" file on Machine2 (in this case). -- Of course, if your router is handling DHCP for your LAN, it is giving out dynamic IP addresses, and the IP address of Machine1 could change. I'm no expert at networking, and I suspect I have always done it the hard way. But it works, and I don't know how else to do it. You might be able to place the DNS entry in your router telling it that requests for VirtualWeb1 (or whatever) should go to Machine1 or to the IP address of Machine1. 3) To access it from OUTSIDE your router (i.e. over the internet), you will have to tell the router to forward port 80 to the proper machine. I am not sure how to make this approach work with virtual server names. I've never really tried.
-
I don't know why they called it session_destroy(). It does not really destroy the session. The session data file is left on the server and the session cookie is left on the client. If you look at the manual for that function, the first example given does a lot more than call session_destroy(). Here is the code from the example: <?php // Initialize the session. // If you are using session_name("something"), don't forget it now! session_start(); // Unset all of the session variables. $_SESSION = array(); // If it's desired to kill the session, also delete the session cookie. // Note: This will destroy the session, and not just the session data! if (ini_get("session.use_cookies")) { $params = session_get_cookie_params(); setcookie(session_name(), '', time() - 42000, $params["path"], $params["domain"], $params["secure"], $params["httponly"] ); } // Finally, destroy the session. session_destroy(); ?> It may be sufficient to clear the $_SESSION array, since that will remove all data from the session. Even if they go back to a previous page, a call to session_start() would result in an empty $_SESSION array; which should mean that there is NOT a logged in user.
-
The ones that already exist, will come back from the query. The ones that don't, won't. $domains_list = implode("','", array_map('trim', $lines)); $query = "SELECT domain FROM domains WHERE domain IN('$domains_list')"; $result = mysql_query($query); $exists = array(); $count = mysql_num_rows($result); if ($count > 0){ while ($row = mysql_fetch_assoc($result)) { $exists[] = $row['domain']; $error[] = $row['domain'] . " is already in use"; } } $not_exists = array_diff(array_map('trim', $lines), $exists);