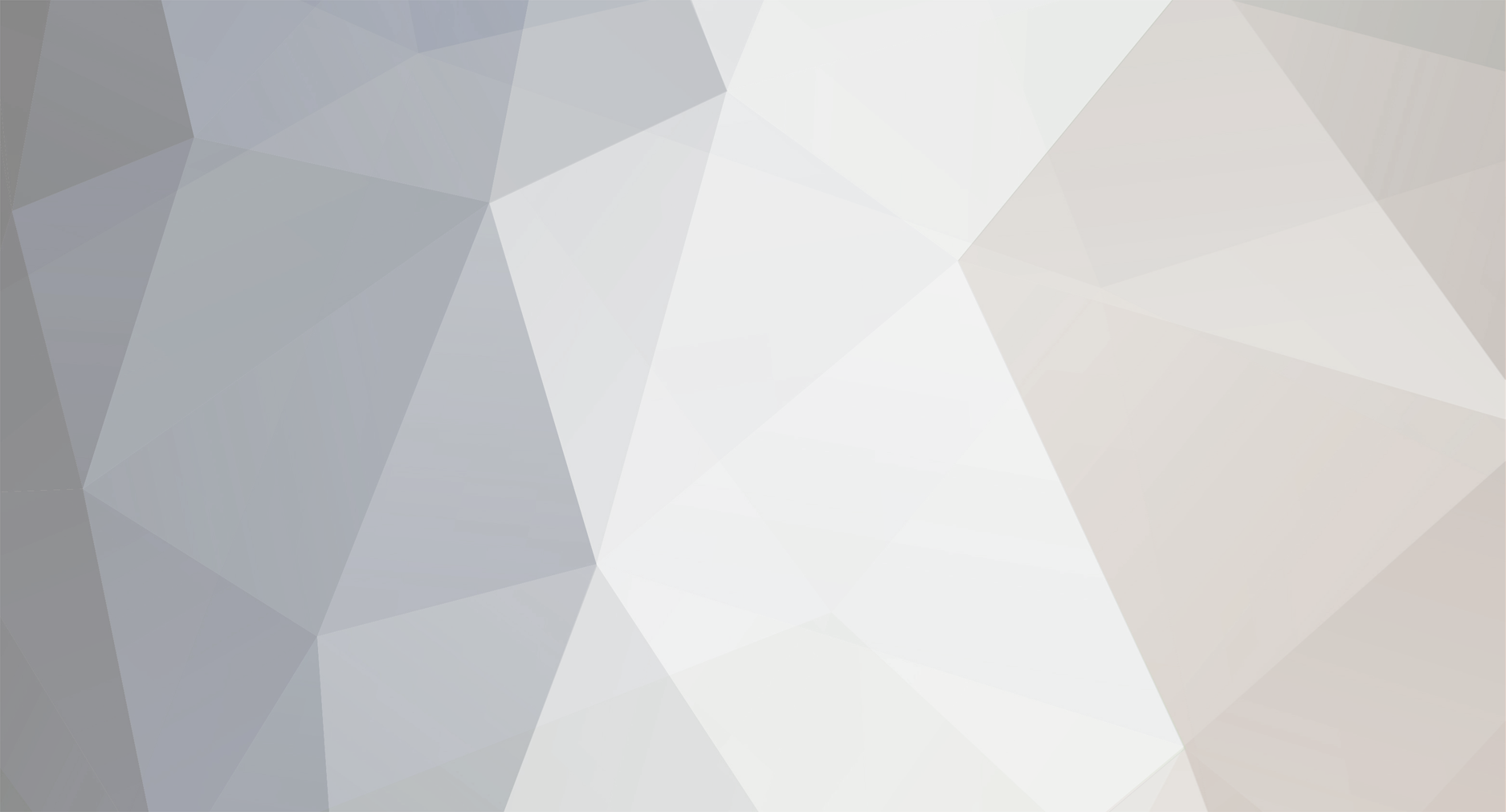
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
You've got a couple of problems here. $success = mail($webMaster, $emailSubject, $body, $headers, $attachment); 1) This is the line that sends the email. You have this line before you load the attachment, so you have sent the email without any attachments; 2) Attachments are sent as part of the body of the message. The fifth parameter to the mail call is for additional parameters to the sendmail call.
-
You need curly-braces to group the code in the IF part and the ELSE part: if($state == 'FL') { $price = $prices_array[$size]; // Set Price $tax = number_format($price * .07,2); // Set tax $amount = number_format($price + $tax,2); // Set total amount } else { $price = $prices_array[$size]; // Set Price $tax = number_format($price * .0,2); // Set tax $amount = number_format($price + $tax,2); // Set total amount; } As you have it, you should get an error about the "unexpected ELSE". You should be developing code with error reporting on so you can see the errors. Put this at the top of your php page: error_reporting(E_ALL); ini_set('display_errors', 1);
-
mysql_query($sql1, $sql2, $sql3, $sql4, $con); mysql_query accepts 2 parameters. The first is the SQl to be executed and the second is the connection. You cannot pass it 4 SQL statements like that. You will need to execute each one independently. mysql_query($sql1); mysql_query($sql2); mysql_query($sql3); mysql_query($sql4); Note: The connect parameter is optional, I personally never supply it; but that is up to you. Note: You should probably add some error checking in between there and print appropriate messages.
-
This is no place for a UNION. This requires a 3-table JOIN, which AbraCadaver posted for you in your original topic: http://www.phpfreaks.com/forums/index.php?topic=339140.msg1598717#msg1598717
-
Do you want to verify that the string only contains valid characters and reject it if it is wrong? OR Do you want to change any invalid characters to something else? You say you want to verify the string only contains letters and numbers, and then make sure that there are only single spaces ... - If you validate that there are only letters and numbers, then there are not any spaces. It sounds like you have user input, and you want to "clean it up". If this is the case, I would suggest the following: [*]Replace all occurrences of CR-NL, CR, and NL with a single space (use str_replace); [*]Replace all occurrences of two-or-more spaces with a single space (use preg_replace with a pattern like: ~ {2,}~); [*]Check for instances of not-valid characters (use preg_match with a pattern like: ~[^A-Z0-9 ]~i) You could actually accomplish #1 and #2 with a single call to preg_replace(). In either case, the array of patterns (or strings) to match need to be listed in the order given above. If the preg_match() (#3) returns 1 (one), then there are invalid characters. If it returns 0 (zero) then there are NO invalid characters. If your desire is to change the invalid characters to something specific, you can use preg_replace() in #3, instead. The following code is not tested: $input = 'User entered 1,203 characters of bald a$$ lies'; $find = array("~\r\n~", "~\r~", "~\n~", "~ {2,}~"); $input = preg_replace($find, ' ', $input); if (preg_match("~[^A-Z0-9 ]~i", $input) != 0) trigger_error("Invalid Input", E_USER_ERROR); //OK
-
The Action attribute in your FORM tag is blank. That means it will post to the same script. There are no fields in that form with the account_number or tran_id, so these fields will NOT be POSTed to the script. This is why the query is not updating the database.
-
// create email headers $headers = 'From: '.$email_from."\r\n". 'Reply-To: '.$email_from."\r\n" . 'X-Mailer: PHP/' . phpversion(); @mail($email_to, $email_subject, $email_message, $headers); header("Location: thankyou.html"); The value you supply in the "From:" header needs to be a valid email address. It also needs to be an email address that belongs to the server that is sending the mail. Some servers will NOT send mail if the from is not an address it owns (since that indicates you are trying to fake a message), and some servers will NOT accept mail if the from address does not belong to the server sending it (again, it appears to be an attempt to impersonate someone). You have set $email_from to the NAME entered by the user, which is likely not a valid email address. You are also setting the "Reply-To:" header to the user's name. This should be a valid email address as well. You should set this to the email address that the user entered. Your $email_to variable should be set (as it originally was) to the person you want to receive the message.
-
which will cause this file to work alone, however will cause an error is you include/require this file into another php file Just for the record (and any future readers), you do NOT have to close out of PHP at the end of an included (or required) file. If you DO close PHP ("?>"), and there is anything more than an immediately trailing newline, it will be considered data to be sent to the browser. This will cause the dreaded "Headers already sent" message when you try to send a header or start a session. Personally, I NEVER close out of PHP at the end of included files or page scripts. [ see the note at http://us.php.net/manual/en/language.basic-syntax.instruction-separation.php ]
-
The query you posted did not have any values for account number or transaction id. That would indicate that either the fields were not POSTed to the script or they were empty. This would imply that there is a problem with the form that POSTed to this script. Take a look at the form in approve_transactions.php, the one with the "Approve" button. You can post the code here (from <FORM ...> to </FORM>). The form needs to have its method set to POST and the action set to the script you are talking about here (approve_page.php). There should be two INPUT fields in that form that are either hidden or text (TYPE=). These fields should have the names: account_number and tran_id. These fields should have their value attribute set to the correct value (account number and transaction id). Note that if you set the DISABLED attribute, some (most) browsers will NOT POST the field when the form is submitted. You can use READONLY to prevent the user from changing the value and still have it POSTed. Note: There really is NO way to be 100% sure that the user did not change the value when they POST the form. It is possible (and relatively easy) to modify these values even if they are hidden or readonly or disabled. From a security standpoint, it is much safer to pass these values in a session.
-
The empty() function will return true when the value is zero. So you need to use an isset() call there: $_SESSION['views'] = (isset($_SESSION['views'])) ? $_SESSION['views']++ : 0;
-
The "blank space" as option and value is most likely the result of having a comma at the beginning or end of your original string. The explode() function splits the string on ALL occurrences of the delimiter. To prevent this from happening, remove the leading and/or trailing comma. You can do this with trim(). Based on the code from requinix: "<optgroup label=\"Others added\">"; $langwording = array_unique(array_map("trim", explode(",", trim($profile_languagegeneral, ',')))); sort($langwording); foreach ($langwording as $langexi) {...
-
Not quite (see include): Without using the output buffering (ob_start(), etc), any output of the script is sent to the browser (or stdout). However, if you use: $foo = include('myfile.php'); $foo will receive true or false (depending on the success of the include); unless the included file executes a return('some value here');; in which case the returned value is assigned to $foo. Sounds confusing, so let's show an example: incSource.php <?php print('<H1>Hello World</H1>'); return('Fooled You'); main.php ob_start(); include "incSource.php"; $content = ob_get_clean(); # $content now contains "<H1>Hello World</H1>" $message = include 'incSource.php'; # $message now contains "Fooled You"
-
I think the query is simpler than you thing: SELECT u.id, u.userid, MAX(l.logindate), DATEDIFF(CURDATE(), MAX(logindate)) as days_since_login FROM users AS u LEFT JOIN login as l ON u.userid = l.tuserid GROUP BY u.id should do it (I think). I don't have a test environment here. Using a LEFT JOIN (with the ON phrase instead of a WHERE) should result in any reference to fields in login being NULL if there is no matching record. You could do it with a WHERE, but it is uglier ... SELECT u.id, u.userid, MAX(l.logindate), DATEDIFF(CURDATE(), MAX(logindate)) as days_since_login FROM users AS u, login as l WHERE (u.userid = l.tuserid OR l.tuserid IS NULL) GROUP BY u.id @ebmigue NULL means the value IS NOT KNOWN. Which is a very real possibility in the REAL WORLD as well. Since I do not know your favorite color and I do not know fenway's favorite color; both of those values are NULL to me. It is NOT possible to say that ebmigue.favorite_color = fenway.favorite_color -- it may turn out that the values are equal once we learn what they are, but until then, we cannot say they are equal -- we cannot say that one UNKNOWN is the same as another UNKNOWN. Representing a NULL in some other way, does not yield accurate results. If we choose to use an empty string instead of NULL, then ebmigue.favorite_color = fenway.favorite_color would evaluate to true. And a query of the database to group people by favorite_color, would yield incorrect results. Perhaps it is not that critical with colors, but what if we were grouping by gender or political affiliation? I really don't see how you can say the Relational Model is based on "2-valued logic". The whole idea is that we can relate multiple values to any one or more elements.
-
For the record, the error message Was complaining about the subquery after the first FROM clause: -- SELECT columns FROM (subquery) AS ALIAS ... SELECT sum(f.forums), sum(t.topics), sum(p.posts) FROM ( SELECT COUNT(*) as forums FROM ".TBL_PREFIX."forums f UNION SELECT COUNT(*) as topics FROM ".TBL_PREFIX."topics t UNION SELECT COUNT(*) as posts FROM ".TBL_PREFIX."posts p) AS SubQuery However, that query will not work anyway. The inner query (the UNIONs) will return three rows, with ONE COLUMN each. And that column name WILL BE "forums". I usually do this as: SELECT 'Forums' AS RowType, COUNT(*) as RowCount FROM ".TBL_PREFIX."forums f UNION SELECT 'Topics', COUNT(*) FROM ".TBL_PREFIX."topics t UNION SELECT 'Posts', COUNT(*) FROM ".TBL_PREFIX."posts p You get three rows (in this case) and I look at the first column to determine which is which
-
Fatal error: Call to a member function fetchAll() on a non-object
DavidAM replied to Mufleeh's topic in PHP Coding Help
I have provided all the help I can based on the code posted. Did you check the $query? Is it valid? Did you run it directly? Did it work? -
You need to look into the UNION clause. This combines two queries into a single resultset. However, the two queries must return the same number of columns with the same data-types in the same order; and the column names in the result set will be the column names used in the FIRST query. This should not be a problem, though. You should not use SELECT * in a query unless you actually intend to use ALL of the columns returned. (SELECT "Room" AS ResType, ID, Name, Description FROM Rooms) UNION (SELECT "Equipment", ID, Name, Manufacturer FROM Equipment) ORDER BY Description This query will return four columns. They will be named "ResType", "ID", "Name", and "Description". The first column is a literal indicating (to me) what type of resource the row describes - I showed this as an example of adding data to the query AND using an Alias - the name of this column is "ResType" (in PHP $type = $row['ResType']). The fourth column is named Description eventhough it will contain Manufacturer for "Equipment" rows, it will still be named "Description". The result will be sorted by the fourth column, regardless of which table it came from.
-
This is a dangerous habit to get into. In an RDBMS (Relational Database Management System) there is no concept of "current row" or "most recent" or even an order (other than an using the ORDER BY clause). If no ORDER BY is provided, the server can return rows in any order it chooses. Since different query plans (based on JOINs or index size) can result in different internal sorts; as your database grows, a query that returns rows in one sequence today might return them in a completely different sequence next week (or year). The proper way to return the "most recent row" is to determine what "most recent" means. If you want the most recent by date, add a CreateTime column, set it to NOW() (on insert and/or update) and ORDER BY that column DESCENDING. If you want the "last row inserted", you need to create an ID column as AUTO INCREMENT, capture the "Last Insert ID" (using the LAST_INSERT_ID() function on the database server or the mysql_insert_id PHP function) and use that value to retrieve the row. Note: The last insert id is based on the connection in use. So, different users WILL get their last insert ID NOT one from a different user. And yes, when selecting a single row, you do not need to use a while loop. You can just fetch the first row returned (after verifying that a row was actually returned). But do NOT select a bunch of rows if you only need one. The database server has to process the rows and WILL SEND THEM TO PHP, and PHP (internally) will have to capture and buffer the ROWS. This is a lot of resources (time, memory, cpu, bandwidth) WASTED on data you never intend to look at. Use "LIMIT 1", or a where clause providing a unique ID, when you only want one row.
-
@kisleki - You need to start your own thread for a new question. @doddsey_65 When you include a file within a function, any variables defined in that file are defined within the scope of the function. So, the $l variable only exists within the scope of the LoadFile method. Outside of that method, it is undefined. If you return this variable, then you need to capture the return value: $langMap = $lang->loadFile('common'); Although, in keeping with the encapsulation concept of OOP, I think I would assign that variable to a private property within the class, and define another method to translate. Something like this: class languageClass { public static $lang; private $_map; // PROPERTY FOR LANGUAGE MAP public static function setLang($lang) { self::$lang = $lang; } public static function getLang() { include(dirname(dirname(__FILE__)).'/languages/'.self::$lang.'.php'); return $lang; } public function loadFile($file) { $include = dirname(dirname(__FILE__)).'/languages/'.self::$lang.'/'.$file.'_lang.php'; if(file_exists($include)) { include $include; $this->_map = $l; // STORE THE MAP IN A PRIVATE PROPERTY return $l; // OR RETURN THE MAP TO THE CALLER (OR BOTH) } else { echo 'File does not exist'; } } // METHOD TO TRANSLATE A WORD public function translate($word) { return $this->_map[$word]; } } Of course, I just copied your code and added a couple of things. I don't know why you are using STATIC for parts of it and not for others. You probably need to reconsider the design. Also, you'll need to add error checking (what if $word does not exist in the map?).
-
Fatal error: Call to a member function fetchAll() on a non-object
DavidAM replied to Mufleeh's topic in PHP Coding Help
That error message indicates that (in your case) $result does NOT contain an object. Since $result is set (presumably to a result object) in the previous statement, this would indicate that $dbh->query($query); failed. This would indicate that either the query failed -- you need to check the value of $query; or there is no database connection -- you need to check the code that assigns $dbh. You may also need to check the base class used to create $dbh (if it is a home-grown class). -
preg_match returns two different results for same string
DavidAM replied to mrgrammar's topic in PHP Coding Help
As AyKay47 stated, you do not want to "treat" the data before testing it. The purpose of these "escaping" functions is to change the string in some way for a specific purpose. So, you need to understand what the functions you call are doing. And you need to call the appropriate function at the appropriate time. Your code: $data = strip_tags(htmlspecialchars(mysql_real_escape_string($data))); The reason the preg_match call returns a different result, is that the string is different. mysql_real_escape_string "escapes" specific characters in the string to prepare it for sending to the database. One of the characters it escapes is the newline ("\n"). So, after calling mysql_real_escape_string() there will be NO instances of CR-LF ("\r\n") in the string because a backslash has been inserted BEFORE the newline. So, it will be: "\r\\n" (the newline is escaped). (It also escapes the carriage-return, but that does not enter into the reason for the preg_match() "failure".) This function should NOT be used as a general protection function. It is intended to be used when you get ready to send data to the database server. htmlspecialchars converts certain characters into HTML tags to prepare it for sending to the browser. This function should be called just before sending a string to the browser when you want the HTML to be displayed and not interpreted. strip_tags removes certain HTML tags from a string. This is handy when you want to display some user-entered text but do not want any HTML to be interpreted and you don't want it displayed. Calling any of these functions (as well as several others) will change the value in a variable. Since the value is different before and after the function call, tests performed against the variable may return different results. -
<input type="text" ID="islandid_js"> <input type="text" ID="formsubmit" value="1"> It is the name attributes that are used when posting forms. The id attribute is NOT used. You need to add name attributes to the fields that you want to access in PHP.
-
<form method="post" action="index.php" name="loginform" id="loginform"> The action attribute specifies the page that the form is posted to. That needs to be changed. If you are posting to the same script (page) that displays the form, you can provide an empty string for the action attribute: <form method="post" action="" name="loginform" id="loginform">
-
From the standpoint of the compiler (or, in this case, the interpreter) it does not matter what name you use for the variable. You could call them $apple and $orange for all it cares. From a programmer standpoint, it is helpful to use variable names that help you remember what it is. I generally use $sql for the query string and $res for the query resource (mysql_query()). Then I use $row for the returned data (mysql_fetch()). When I see "if(empty($res))" somewhere in my code, I know it is checking the query resource, not the query string and not the returned data. The important thing is to choose a naming convention that works for you, and be consistent. When you are working on a team, these naming conventions are (or should be) defined and documented, so everyone on the team can quickly identify a variable no matter who wrote (or last worked on) the code.
-
Change Field Name to Current Date in MySQL DB
DavidAM replied to anonymoose's topic in PHP Coding Help
"Kill 'em with kindness", my dad always said. Sometimes people don't learn until they hit the brick wall. I just hate having to cleanup all the blood on the wall. In reality, all these hinky database designs I keep seeing here drive me batty. Sometimes, I just have to dump on them. Maybe the OP, or someone later reading this thread, will at least learn some questions to ask. @Gizmola: I hope you did not take offense with my reply. I meant none!