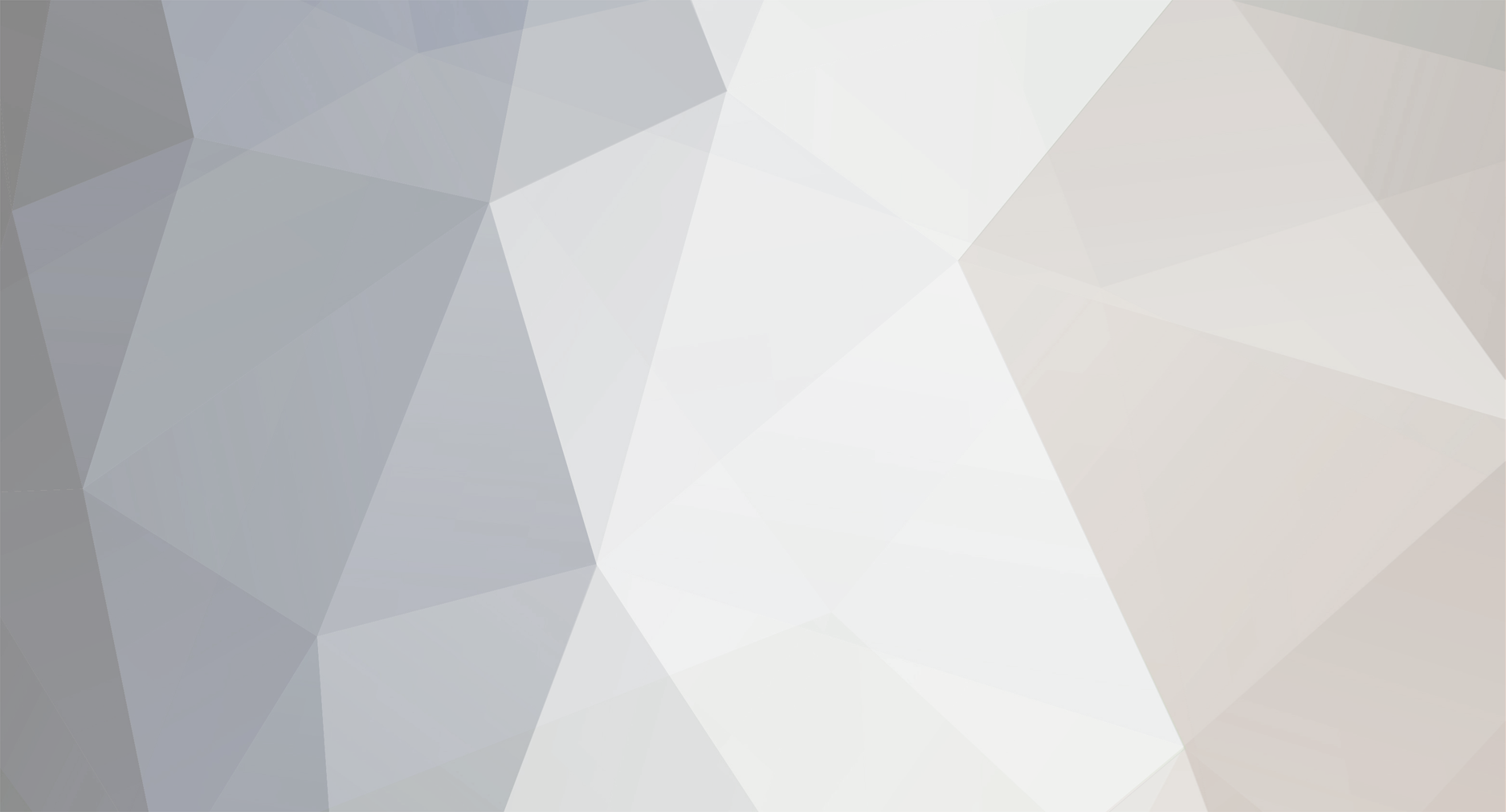
JAY6390
Members-
Posts
825 -
Joined
-
Last visited
Everything posted by JAY6390
-
Try this <?php $realmd = array( 'db_host'=> 'host', //ip of db realm 'db_username' => 'name',//realm user 'db_password' => 'pw',//realm password 'db_name'=> 'db',//character db name ); /*Connect and Select*/ $realmd_bc_new_connect = mysql_connect($realmd['db_host'],$realmd['db_username'],$realmd['db_password']) or die("Problem connecting to MySQL<br>" . mysql_error()); $selectdb = mysql_select_db($realmd['db_name'],$realmd_bc_new_connect) or die("Problem selecting the database<br>" . mysql_error()); if (!$realmd_bc_new_connect || !$selectdb){ echo "Could not connect to db."; die; } $action = array(); $action = mysql_query("SELECT * FROM `character_ticket`"); $action_rows = mysql_num_rows($action); for ($i = 1; $i != $action_rows; ++$i) { $result = mysql_query("SELECT `ticket_id`* FROM character_ticket WHERE ticket_id = '$i'"); $row = mysql_fetch_assoc($result); echo "<b>TICKET #</b>"; echo $row['ticket_id'].":</br>"; echo "<b>PLAYER: </b>"; echo $row['guid']."</br>"; echo "<b>MADE: </b>"; echo $row['ticket_lastchange']."</br>"; echo "<b>TICKET: </b></br>"; echo $row['ticket_text']."</br>"; } ?> Note that I removed the $name = ... line in the loop, since it wasn't being used. Also, you don't need to create a query for each individual item in a row. That will cause you all sorts of problems when you get multiple users doing the same queries, and will cause crashing no doubt
-
Include function from a third party website
JAY6390 replied to themistral's topic in PHP Coding Help
If you have access to the remove server you can create a simple script to output the data, or even ftp onto the server and retrieve the file via phps ftp functions. if not, you wont be able to do this -
Include function from a third party website
JAY6390 replied to themistral's topic in PHP Coding Help
If the file is parsed on the remote server before you receive it then you won't get it into your code. You need the file to be output. If you type the url directly into your browser can you see the correct code when you view the source? -
Matching specific amounts of space and tab characters
JAY6390 replied to Prismatic's topic in Regex Help
<?php function tab_replace($args) { $tmp = '[h1='.str_replace("\t",'/t', $args[0]).'][/h1]'; return $tmp; } $text = ' One tab Two tabs Three tabs '; $output = preg_replace_callback('/\t+/', 'tab_replace', $text); echo $output; -
change the line to $conn = mysql_connect('jjennings3db.bimserver2.com', 'jjennings3db', 'bullet1238'); Also if these are the correct credentials for the database you should change them as you've just posted them on a public forum
-
Are you sure that the remote server allows connections? Do you get any errors?
-
ah yes I forgot the vital part
-
You should run this in stages. If you're trying to create one big query then you need to remember that your memory usage will be the memory taken from the loaded file in addition to the memory usage of your query you are creating Personally I would use something like fgetcsv() for this, loading in line by line and processing the data. If you're creating a query to run perhaps running them in 20 sets of data batches at a time rather than all of them at once. Also be aware of the time limit set for scripts, you will need to set the max time limit to a higher level if you've got a lot of data like this to process
-
Is there any reason why you need the whole of this file imported at once? what sort of file is it (ie what are you wanting to do with the data). If you change the size to a bigger memory limit it will probably work, but you shouldn't have to do that
-
The first method seems like the right choice to be honest. You could end up with spammers uploading files with no intention to make a payment - The other way round there's no issue with it
-
A regex or parse_url I'd imagine
-
I also recommend that you actually remove the get url in the form submission completely, and go with a hidden input instead <form name="form1" method="post" action="update_skill.php'"> <input name="id" id="id" value="<?php echo $id; ?>" /> Then in your php use $_POST['id'] rather than $_GET['id']
-
change it to <form name="form1" method="post" action="update_skill.php?id=<?php echo $id; ?>">
-
Getting an array populated by a database to shuffle?
JAY6390 replied to morocco-iceberg's topic in PHP Coding Help
What exactly does the print_r show? the code you have should work as it is -
Getting an array populated by a database to shuffle?
JAY6390 replied to morocco-iceberg's topic in PHP Coding Help
Why not let MySQL do it for you SELECT * FROM table ORDER BY RAND() -
Me thinks someone has code written for php where register globals is allowed
-
if(file_exists("$file_for_game")) { $stop = 'true'; } is how you can fix that line I have no idea wtf you were trying to do with the = $stop == but that is not going to work
-
clearly the system you have now has full error reporting on now. You can disable it by putting error_reporting(E_ALL ^ E_NOTICE); at the top of your php scripts (after the <?php on a new line)
-
Here's a good video on regex for beginners that you might find useful http://www.phpvideotutorials.com/regex/
-
Use /^[A-Za-z0-9]+$/
-
Missing a semicolon at the end of this line $query = "select * from swr_player" should be $query = "select * from swr_player";
-
The problem is that you have the assigning of array_search() to $key in an if() statement. The first pass of this won't work simply because 0 is equivalent to false, meaning that the unset will never happen for the first character (at key 0). This is visible if you do some debugging. Just add echo "LETTER: $letter - KEY: $key - i: $i<br />"; at the very end of the while loop just before the } and you get the following output LETTER: l - KEY: 0 - i: 0 LETTER: i - KEY: 1 - i: 1 LETTER: n - KEY: 2 - i: 2 LETTER: u - KEY: 3 - i: 3 LETTER: s - KEY: - i: 3 It becomes more apparent with this, as you see the $key is matched as 0 with the l, but $i does not increase when it should. Changing your code to function jots($word1, $word2) { // The guessed word $g_word = str_split($word1); // The challenger's word $c_word = str_split($word2); // Initialize counter $i = 0; if ($c_word == $g_word) { echo 6; // This is if the person gets the word correct...6 is my indicator for a winning game } else { while ($letter = array_shift($g_word)) { $key = array_search($letter, $c_word); if ($key !== FALSE) { // Remove the found letter from the challenge word array unset($c_word[$key]); $i++; } } echo "The word has {$i} letters in common"; } } Hope that clears up the reasoning
-
How about this instead - I finally guessed what it was the game did function jotts($answer, $guess) { $count = 0; $a = str_split($answer); $g = str_split($guess); foreach($g as $v) { $key = array_search($v, $a); if($key !== FALSE) { unset($a[$key]); $count++; } } echo 'Total jotts for <strong>'.$guess.'</strong> in word <strong>'.$answer.'</strong> is <strong>'.$count.'</strong><br />'; } jotts('class', 'slimy'); jotts('class', 'saucy'); jotts('class', 'sassy'); jotts('class', 'class'); jotts('linus', 'linux'); jotts('light', 'right'); This returns Total jotts for slimy in word class is 2 Total jotts for saucy in word class is 3 Total jotts for sassy in word class is 3 Total jotts for class in word class is 5 Total jotts for linux in word linus is 4 Total jotts for right in word light is 4
-
Wait, I think I've misunderstood, you don't want just the number of letters that aren't present do you? You want the number of letters right and in the right place is that correct?
-
How abouts we make it a really simple function instead function jotts($answer, $guess) { $out = array_diff(str_split($answer), str_split($guess)); return strlen($answer) - count($out); } echo jotts('linus', 'linux');