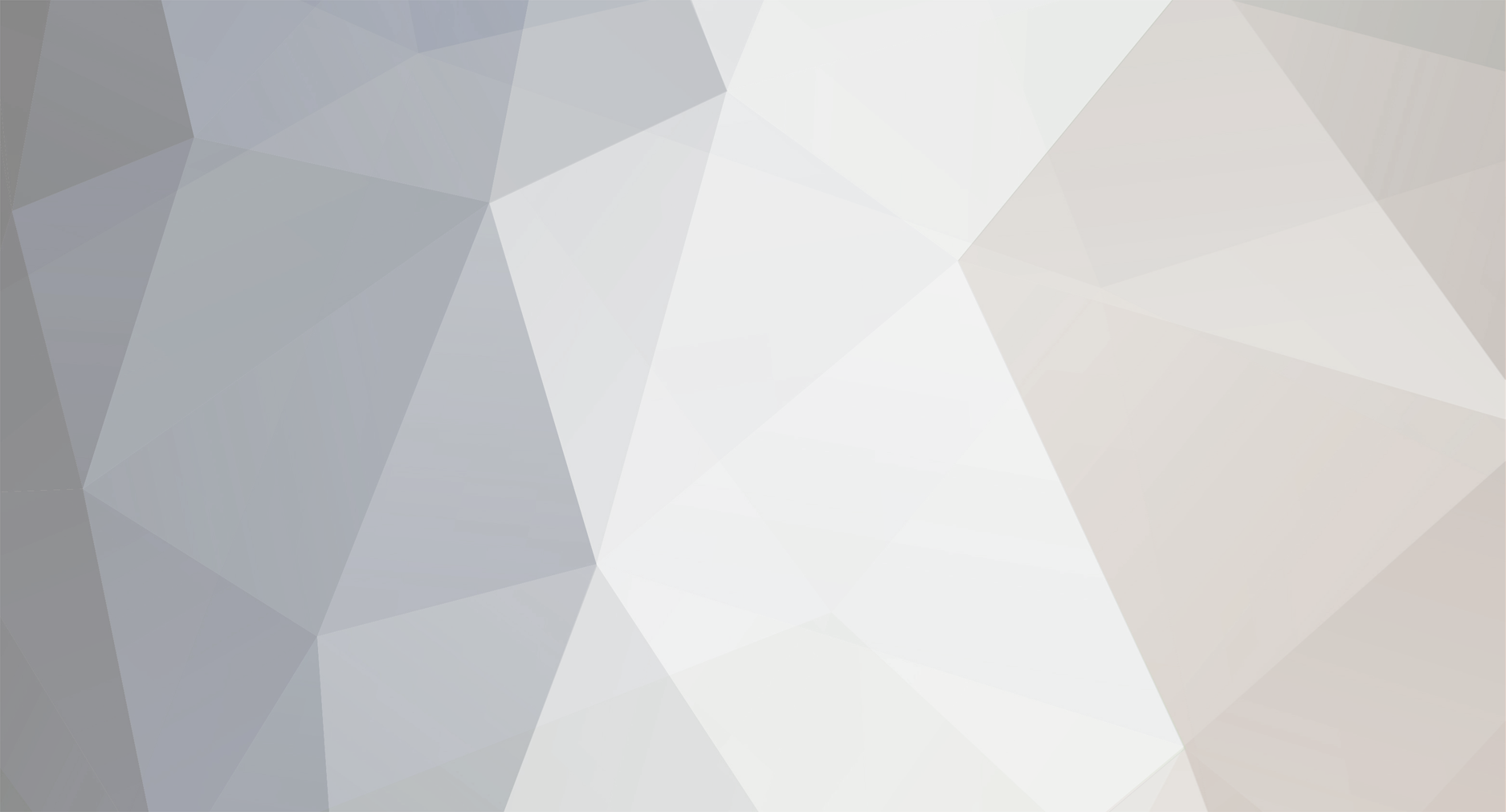
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
PHP is server side. It has no idea about buttons, javascript, forms, etc. All it knows is that you are telling it to insert the data in the foreach loop, which would insert each line as it processes them. What you would have to do, is create a second script, and pass the info to it via a POST. This can be done by a form with hidden values and a submit button, or a submit button that sends it via javascript (AJAX). You would really only need to send the row ID to the second page, as you can do everything inside of the database with an INSERT...SELECT statement.
-
I don't see anywhere where you are trying to limit the courses based on attendance. So I am not sure that I understand the question correctly. However, I did notice that you have severely limited yourself with your database design. You should look into database normalization, I think this would help eliminate some if not all of your problems. You should also look into separating you logic from your output.
-
I noticed that your "real_human" input didn't have a valid type. AFAIK, the only valid types are: text | password | checkbox | radio | submit | reset | file | hidden | image | button
-
Only if you use the facebook API.
-
Stored procedures are "stored" on the database server, therefore you cannot use them in the PHP server. However, you can store a procedure, and call it from a different program such as PHP. In standard SQL you would use the MySQL CALL or the SysBase CALL or the Oracle CALL syntax. Of course, that list goes on and on.
-
I changed the things that stood out to me. This code is un-tested though, so I'm sure I could have missed something. <?php // Connect to the MySQL database include "storescripts/connect_to_mysql.php"; // This block grabs the whole list for viewing $i=0; $firstRun = true; //holding. $sql = mysql_query("SELECT * FROM products ORDER BY date_added DESC"); $productCount = mysql_num_rows($sql); // count the output amount if ($productCount > 0) { $dyn_table = '<table border="0" cellpadding="4"><tr>'; //added <tr> to start the first row. while($row = mysql_fetch_array($sql)){ $id=$row["id"]; $product_name=$row["product_name"]; $price=$row["price"]; $category=$row["category"]; $first_subcategory=$row["first_subcategory"]; $second_subcategory=$row["second_subcategory"]; $date_added=$row["date_added"]; //remove the appending . from $dynamicList, because I'm sure you don't want 15 tables on the last table cell. $dynamicList = ' <table width="20%" border="1" cellspacing="0" cellpadding="4"> <tr colspan="3"> <td><a href="product.php?id='.$id.'"><img src="inventory_images/'.$id.'.jpg" width="243" border="1" height="240" alt="$dynamicTitle" /></a></td> </tr> <tr> <td> ' .$product_name . '</br> <hr> $ ' .$price . '</br> <hr> <a href="product.php?id='.$id.'">View Product Details</a></td> </tr> </table>'; if ($i++ % 3==0 && $firstRun == false){ //increment $i before EDIT: AFTER using, so on the first run 1 / 3 != 0; $dyn_table .='</tr><tr>'; //added a closing </tr> so that the row will end on the 3rd run. } //took out else statement, as there is no need to type things twice. $dyn_table .='<td>' . $dynamicList .'</td>'; $firstRun = false; //changed firstrun to false, so that the increment will work correctly. } $dyn_table .= '</tr></table>'; } else { $dynamicList = "We have no products listed in our store yet"; } mysql_close(); ?>
-
Creating site which displays data from another sites
jcbones replied to merkont's topic in PHP Coding Help
First you would need to read the Terms of Service of the websites, and make sure they allow the use of their data. Then you need to find out if they have an available API that you could use. If they don't, then you proceed to crawl the page and scrape the data. You will find that most all websites that allow data use, will have an API though. -
For development you should have error_reporting set to full, and display_errors set to on. If you don't have access to your php.ini (where it should be set), then you can set it at runtime by adding the following to the top of your scripts. error_reporting(-1); ini_set('display_errors',1);
-
If it were me, I would not re-direct after a cURL call. Instead process the cURL, and wait for the server response. Depending on that response, I would then either: A throw an error back to the user, or B Throw a success back to the user, AND record it to the database. Most payment gateway's have API's to help with this, along with full documentation to guide you in the process.
-
cURL is the most common way to do what you are asking.
-
I am with Adam Bray on this one. Here is an exercise: Run the following code in a stand alone script: <?php $color_to_hex = array( 'black' => '#000000', 'blue' => '#0000ff', 'green' => '#00ff00', 'red' => '#ff0000', 'white' => '#ffffff', ); if($_SERVER['REQUEST_METHOD'] == 'POST') { echo 'The color you selected is ' . $_POST['color'] . ' and has a hex value of ' . $color_to_hex[$_POST['color']] . '<br />'; } echo '<form method="post"> Select font color: <br /> <select name="color" id="color">'; foreach($color_to_hex as $key => $value) { echo '<option value="' . $key . '">' . ucfirst($key) . ' Frame</option>'; } echo '</select> <input type="submit" value="Submit" /> </form>';
-
need help with my site page using browers ,txt not showing
jcbones replied to hanna21's topic in PHP Coding Help
I see the same thing in Firefox, Chromium, and Opera. -
$category is an object. You cannot then change it to an array. Object: $category->category_id Cannot change to array: $category['links'] = $links->result(); //can't do it, oh NOES you can't.
-
Taking hansford's comment and expanding on it. $query = "SELECT * FROM table_name ORDER BY name"; //query to send to database. try { $con = new PDO('mysql:host=localhost;dbname=your_db_name', $username, $password); //pdo connection to database. $con->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); //set attributes of connection. $result = $con->prepare($query); //prepare our query. $result->execute(); //execute the query. } catch(PDOException $e) { //if an error occurred. echo $e->getMessage(); //get the error. } $table = "<table cellpadding=10 cellspacing=0 border=1>"; //start a table variable. $table .= "<tr>"; //append a table row to the variable. $table .= "<th>Name</th><th>Contact</th><th>Code</th><th>Type</th><th>Quanitiy</th>"; //append a table header row. $table .= "</tr>"; //append closing row tag. $name = NULL; //declare a name variable. $price = 0; //declare a price variable. while($row = $result->fetch(PDO::FETCH_ASSOC)) { //get the results from the database resource. if($name != $row['name'] && $price > 0) { //if the name variable doesn't match the row from the database, //AND the price variable is greater than 0. $table .= "<tr><td colspan=\"5\">Total: \${$price}</td></tr>"; //*Then we append the total row to the table variable. $price = 0; //and reset the price variable back to 0. //*NOTE: You may want to run number_format() on the price before sending it to the page. } $table .= "<tr>"; //but on every new database row, we append a new row to the table. $table .= ($name == $row['name']) ? "<td> </td>" : "<td>" . $row['name'] . "</td>"; //and a new name, UNLESS the name is the same as the last one. $table .= ($name == $row['name']) ? "<td> </td>" : "<td>" . $row['contact'] . "</td>"; //and a contact, UNLESS the name is the same on the last one. $table .= "<td>" . $row['code'] . "</td>"; //new codes are always appended. $table .= "<td>" . $row['type'] . "</td>"; //as are types. $table .= "<td>" . $row['quanity'] . "</td>";//and quantities. $table .= "</tr>"; //and of course we close each row. $name = $row['name']; //now that we are done, we need to make sure that we know what the current name is on the next loop. $price += $row['price'] * $row['quantity']; //appending the price of the current type, multiplied by the quantity. } $table .= "</table>"; //loops are done, close the table. //of course, now you need to echo the data. echo $table;
-
I'll be blunt also. So, I commented your file. <?php $conn = mysqli_connect($host, $user, $password, $database); //Make connection, un-declared variables used $host,$user,$password,$database. $host; is; 'localhost'; //un-defined constant, assumed string, un-expected string, $user and $password; ""; //un-expected string. $database; 'USERS' //un-expected string. //This whole block is NOT PHP, but is consistant with the andriod API (Which is based on JAVA) Table USERS` . `USERS` // CREATE TABLE IF NOT EXISTS public void createTable(){ try { mydb = openOrCreateDatabase(DBNAME, Context . MODE_PRIVATE, null); mydb . execSQL("CREATE TABLE IF NOT EXISTS " + TABLE + " (ID INTEGER PRIMARY KEY, NAME TEXT, PLACE TEXT);"); mydb . close(); } catch (Exception e) { Toast . makeText(getApplicationContext(), "Errore durante la creazione della tabella", Toast . LENGTH_LONG); } //End of ANDRIOD API ?> <?php $con=mysqli_connect(USERS.mwb); //calling a connect function AGAIN, but this time without the required arguments. //Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } $sql="INSERT INTO USERS (FirstName, Address, EmailAddress,TelephoneNumber, Password) VALUES ('$_POST[firstname]','$_POST[Address]','$_POST[EmailAddress]' , '$_POST[TelephoneNumber , '$_POST[Password]']')"; if (!mysqli_query($con,$sql)) { die('Error: ' . mysqli_error($con)); } echo "1 record added"; mysqli_close($con); if (isset($_POST['submit'])); { //did not close the bracket, will result in an "un-expected end$" error. ?> PS. you cannot "save" a MySQL database in PHP, either it exists, or it doesn't.
- 3 replies
-
- php
- registration
-
(and 2 more)
Tagged with:
-
Divs nesting in foreach loop when they shouldn't
jcbones replied to AdRock's topic in PHP Coding Help
So, you open three (3) <div>'s in the first foreach, and close two (2) </div>'s after the inner foreach's finish. I'm willing to bet, if you throw another </div> before the ending endforeach; that it will work like you want it to. -
This is why Captcha's are getting harder for users to see. No help from me.
-
Sorry, here is some ways to correct it. 1. Set a default value to the data column, of 0 of course. 2. In your columns array, instead of setting it to the word NULL, just set it to 0.
-
Here is where your problem is: empty()
-
How to change all content between set of brackets.
jcbones replied to madjack87's topic in PHP Coding Help
preg_match() is not the droid you are looking for. preg_match_all() is what you are looking for. -
I would like to advise not even using the isset($_POST['submit']), and instead suggest using the $_SERVER superglobals. if($_SERVER['REQUEST_METHOD'] == 'POST') This is due to what Barand is talking about.
-
Instead of !in_array(), pass the $data_to_add to this function instead. function uniqueName($data,$lines) { $name = explode('|',$data); //separate the name out of the pipe delimited list; foreach($lines as $line) { //go through each line of data; if(substr($line,0,strlen($name[0])) === $name[0]) { //test for the first word to match $name; return false; //if it does, it isn't unique, so return false; } } return true; //if it doesn't return true, because it truly is unique. } Of course, test it for yourself. *note* depending on array size, testing for uniqueness in a file could take a while. That is why databases are always suggested, as they are optimized for this task.
-
I suggest mcrypt. There are plenty of examples at the link.
-
session_start(); $_SESSION['variable'] = (isset($_SESSION['variable'])) ? $_SESSION['variable'] : false;
-
An "API" is an Application Programming Interface, and is used to provide all data that you need to do any task you wish (inside of the sites TOS) with data from that specific site. Most sites provide one so that you don't eat up bandwidth by scraping the site, as API's generally only return the specified data that you need. How an API returns data is pretty site specific. Most return in a JSON, XML, or CSV format, but many can return in any format you wish. Yahoo finance API code is listed at: http://code.google.com/p/yahoo-finance-managed/wiki/YahooFinanceAPIs