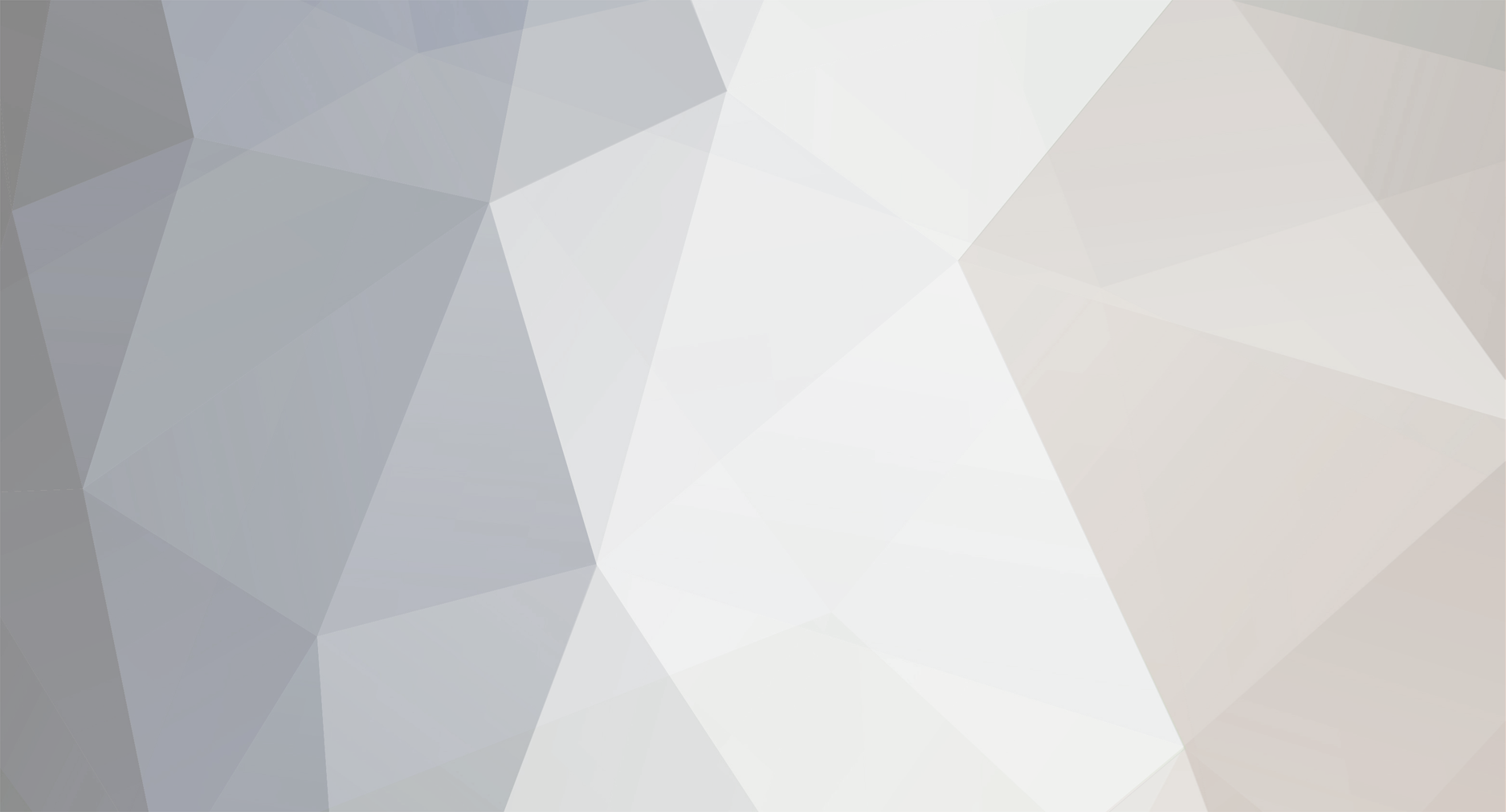
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
The data cannot be correctly inserting, being that the data does NOT exist. That is what the notices are telling you. There will be gaps in the data, unless you add some de-bugging, you will not ever know what loop the notices are created on. You also are only asking for offsets 1 - 35, so I don't know where that "undefined offset 36" came from. *rambling on* You should also change this to a simple while loop, since the do section will never do anything on the first pass, until while is evaluated. In other words, you are creating the $data variable AFTER the first run of the loop, so there is no need to have a do/ while loop. Make it a simple while loop. You should also switch to the mysqli extension or PDO, and bind the parameters. That way you can type cast it correctly. I would also break up the inserts into chunks (multi row insert query), so I didn't have so many database calls. *rambling off*
-
To read it without any styling. <?php $file = 'mytext.txt'; $contents = file_get_contents($file); $contents_formatted = nl2br($contents); echo $contents_formatted;
-
You should be able to export the data via .csv files, or the ODBC connector available i MS Access. MySQL has documentation on that... http://http://dev.mysql.com/doc/connector-odbc/en/connector-odbc-examples-tools-with-access-export.html
-
Don't know if it will work the way you want, but you could try to do the sleep function after the redirect. //All of the form processing. //.... ///..... header('Location: http://mysite.com/page2.htm'); sleep(10); //send email //... //... exit();
-
You need to do some data normalization, as well as storing dates in the correct column types. You were right about the data being confusing, but I think I got you on the right track. I wasn't sure how or what the last column (balance) was suppose to be calculated by, so I didn't include that. It would be easy to add, I would like to see what you come up with. Edit: on second look, the last balance is in there, but you image had me adding another one. Ahh 16hr days... <?php /////////////////////////// //Set your database details define('DATABASE','test'); define('DATABASE_USER','root'); define('DATABASE_PASSWORD',''); define('DATABASE_HOST','localhost'); /////////////////////////// //database connection; $db = new mysqli(DATABASE_HOST,DATABASE_USER,DATABASE_PASSWORD,DATABASE); //query string: $sql = "SELECT `item_code`,`item_name`,`address`,`quantity_receive`,`ret`,`sold` FROM `pro_user_employee` JOIN `pro_request` USING(`employee_id`) ORDER BY `address`"; //run query and check for results; if(!$result = $db->query($sql)) { //if query failed, find out why; echo $db->error; } //make sure results are returned: if($result->num_rows > 0) { //if there are results, run them while($row = $result->fetch_assoc()) { //dump all data into an array for sorting. $address_array[] = $row['address']; $storage[$row['item_code']][$row['address']] = array('name' =>$row['item_name'], 'received' => $row['quantity_receive'], 'retorn' => $row['ret'], 'sold' => $row['sold'] ); } //process the storage array, sorting the output. //clean up the address array: $address_array = array_unique($address_array); //clean up addresses array $address_array = array_values($address_array); //keys to adresses $keys = array_keys($address_array); //how many address do we have: $count = count($address_array); //build the table headers: $table = <<<'EOF' <table border=1> <tr> <th rowspan = "2">Item Code</th> <th rowspan = "2">Description</th> EOF; foreach($address_array as $value) { $table .= '<th colspan="4">' . $value . '</th>'; } //close first row, open second. $table .= '</tr> <tr>'; //build secondary headers, based on how many addresses. for($i = 0; $i < $count; $i++) { $table .= <<<'EOF' <th> Received </th> <th> Retorn </th> <th> Sold </th> <th> Balance </th> EOF; } //close second row. $table .= '</tr>'; //loop through the stored data, and sort. foreach($storage as $item_code => $addresses) { //each item has it's own row, so start it, first column is the item code, so drop it here. $table .= '<tr> <td>' . $item_code . '</td>'; $row_starts = 0; //lets us know if the row has been started, This controls the data flow AFTER the item code. $cols = NULL; //clear our column variable. foreach($keys as $value) { //for each key from the addresses array. if(array_key_exists($address_array[$value],$addresses)) { //see if that address exists in the storage array, under the current item code. $value = $address_array[$value]; //if we got here, it did, so lets get the key that existed. if($row_starts == 0) { //if our row hasn't started, we need to get the item description. $name = '<td>' . $storage[$item_code][$value]['name'] . '</td>'; //storing it in an array named ($name). $row_starts = 1; //now our row as started, so lets change the row starts variable. } $cols .= '<td>' . $storage[$item_code][$value]['received'] . '</td>' .'<td>' . $storage[$item_code][$value]['retorn'] . '</td>' .'<td>' . $storage[$item_code][$value]['sold'] . '</td>' .'<td>' . ($storage[$item_code][$value]['received'] - $storage[$item_code][$value]['sold']) . '</td>'; //list out our columns, getting our balance by simply math. } else { //if the address was not in the stored data, then assign the number 0 to the column. $cols .= '<td>0</td>' .'<td>0</td>' .'<td>0</td>' .'<td>0</td>'; } } $table .= $name . $cols; //before we open a new item, lets close this one by appending the name and cols variables back to the table variables. $table .= '</tr>'; //then close the current row. } $table .= '</table>'; //all items have completed the run, now close the table. echo $table; //print the table out to the page. //echo '<pre>' . print_r($storage,true) . '</pre>'; //de-bugging purposes only. }
-
Users shouldn't have separate tables, because databases are not spreadsheets. 1 table for users, then use primary/foreign keys to tie the users to the correct data in the other tables.
-
The way I would do it is have a couple of different tables. An item table An order table A item_order table Items hold all of your items available (quantity, description, sku, price, etc). The order table holds all the order details (transaction id, processed, total, etc). The item_order ties the orders to the items (via foreign keys). This would require you to learn about data normalization, and relational database design. This will save you headaches in the future with data collision. Note** This is as simple of a design as it gets, you can go much more complex. I would sit down and determine the features that I wanted, the data I expected to collect, then design the application from there. Hacking it in later, is always a headache, and never turns out clean and bug free. My 2 cents.
-
I am willing to help you, but I would need a database dump with sample data, 5 rows would be enough.
-
You need to order by the indexed column. This will ensure that all the column names are returned together. $x = "SELECT REQUEST.request_id, USER.address FROM pro_user_employee AS USER JOIN pro_request AS REQUEST ON USER.employee_id = REQUEST.employee_id ORDER BY USER.address";
-
OR, $sql = "SELECT TIMESTAMPDIFF(HOUR,`date`,NOW()) as hours FROM `table`";
-
How are you trying popen?
-
Make sure to change your database credentials, and table names. You will have to do the table styling also. <?php //create the sql query string, take note that the order by clause sorts the countries in alphabetical order $sql = "SELECT a.country, b.city FROM country AS a JOIN city AS b ON a.country_id = b.country_id ORDER BY a.country"; //create a mysqli object $db = new mysqli("localhost", "my_username", "my_password", "my_database"); //query the database $result = $db->query($sql); //create a variable to hold the last known country name. $last_country = NULL; //fetch the data, until there are no rows left. while($row = $result->fetch_assoc()) { //if the last_country is not equal to the current country, then we start a division (<div>) and a table (<table>) if($last_country != $row['country']) { //but if last country does not explicitly equal null, then we first must close the division and the table. if($last_country !== NULL) { echo '</table></div>'; } echo '<div style="float:left;"> <table style="border:1px solid black"> <tr> <th>' . $row['country'] . '</th> </tr>'; } //every run of the while loop should output the city echo '<tr><td>' . $row['city'] . '</td></tr>'; //after everything is done, we now set the current country, to the last_country (for the new loop). $last_country = $row['country']; }
-
What is the column type for the news_image? Or, just give us a structure dump of the table.
-
Passing an argument as opening and closing tag
jcbones replied to eldan88's topic in PHP Coding Help
Yes, but it doesn't have to be named $response, just pass what you want $response to be. <-might not be real clear, but that is how my mind is working today! -
I read that twice, and I still have no idea what you want. Slow down, and type out your question, concisely. So that someone, that doesn't know how to read minds, can understand your question.
-
Why not just use glob. There shouldn't be a reason to use a database for this, unless you are storing when it was executed (but you could write that back to a file, also).
-
There are a lot of different tutorials to upload files to the server via a form and php. http://http://www.google.com/search?q=php+image+upload+form If you get stuck on a particular area, we could help you further. If you are looking for someone to write the code for you, then you may need to head over to the freelance section of this forums. Hope this helps.
-
Need Help Controlling Output from Query and Sub-Query
jcbones replied to spock9458's topic in PHP Coding Help
I bet if you post your database structure, and your two queries (in the MySQL section), those guru's in the MySQL section would be able to give you a very efficient way of handling this all in 1 query. -
(PHP Button) So simple yet so complicated?!? Please help
jcbones replied to Saves's topic in PHP Coding Help
I see no reason why that code would not work. Un-comment the debugging section, and then run the code, and/or look at the page source to see if it holds what it is suppose to. -
The heredoc syntax requires <<<END_TEXT to be the end of the opening line: Edit: since there are no variables that need interpolating, you could change this line to a nowdoc (by putting single quotes around the opening 'END_TEXT'). $myText = <<<END_TEXT But think not that this famous town has only harpooners, cannibals, and bumpkins to show her visitors. Not at all. Still New Bedford is a queer place. Had it not been for us whalemen, that tract of land would this day perhaps have been in as howling condition as the coast of Labrador. END_TEXT;
-
<?php if($_SERVER['REQUEST_METHOD'] == 'POST') { //if page is posted, then form was submitted. $update = sprintf("UPDATE sport SET sport_ime='%s', sezona='%s' WHERE id=%d", mysql_real_escape_string($_POST['sport_ime']), mysql_real_escape_string($_POST['sezona']), $_GET['id']); //put values into proper formatted, and escaped string. if(!mysql_query($update)) { //either query successfully. echo mysql_error(); //or error out. } } $sql = "SELECT * FROM sport"; //build the main query. if(!emtpy($_GET['id']) { //if the $_GET index is present, then we need to get just that record. $edit = 1; //specify this page is an edit. $id = (int)$_GET['id']; //cast value to integer. $sql .= "WHERE id = $id"; //add the where clause to the query. } $query = mysql_query($sql); //run the query. while($row = mysql_fetch_array($query)) //fetch results. { if(isset($edit)) { //if this page is an edit page. echo '<form action="" method="post">' . "\n" .'<input type="hidden" name="id" value="' . $row['id'] . '" \>' . "\n" .'<label for="sport_ime">Sport IME</label>' . "\n" .'<input type="text" name="sport_ime" value="' . $row['sport_ime'] .'"/>' . "\n" .'<label for="sezona">Sezona</label>' . "\n" .'<input type="text" name="sezona" value="' . $row['sezona'] . '">' . "\n" .'<input type="submit" name="submit" value=" SUBMIT " />' . "\n" .'</form>'; //build the form. } else { //if it isn't. echo $row['sport_ime'].' '.$row['sezona'].'<a href="?id=' . $row['id'] . '">Edit</a><br>'; //show the rows with a clickable edit link (which reloads the page and starts over). } }
-
2 problems. 1. You are not closing the file after fwrite, because fclose is inside of a comment. 2. header expects an actual URI, with relative URI's it works sometimes, but isn't reliable. So, spell out that protocol. header('Location: http://mysite.com/index.php');
-
Or, you could never update, only insert. Returning the last row inserted, until you need to see what was revised.
-
OR, change the if, if, if, if, else statement to an if,elseif,elseif,elseif,else statement.
-
I see the $message in the OP, changing a double quoted string to a heredoc is not really a help in this situation IMHO (unless I am totally missing something). I would go back to Jazzman's post and say var_dump the incoming $_POST to make sure you have the index names right.