-
Posts
3,584 -
Joined
-
Last visited
-
Days Won
3
JonnoTheDev last won the day on April 16 2015
JonnoTheDev had the most liked content!
About JonnoTheDev
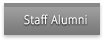
- Birthday 04/29/1981
Profile Information
-
Gender
Male
-
Location
Liverpool, UK
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
JonnoTheDev's Achievements

Regular Member (3/5)
19
Reputation
-
Implications of database schema on PHP implementation
JonnoTheDev replied to NotionCommotion's topic in PHP Coding Help
I can only see the need for 2 tables. A documents table and a document_types table. The documents table references the documents_type table by type_id I.e. documents ---------- doc_id type_id date_added doc_name document_types ---------- type_id type_title document_types would contain people, companies, and projects, and any other types. Each has an id and is used in a join from documents when you want to say fetch all company documents. You do not want to be creating separate tables for each type as there is no flexibility in the design and as you have already found out it is hard to create the business logic. -
Here's a challenge for anyone using OOP so you can see how it benefits. I've started it off and you can see there are issues that need to be corrected. An online shop needs an order dispatch system for sending out parcels using different couriers. At the start of the day a batch will be started and at the end of the day it will be closed when no more parcels are going to be shipped. This is called the dispatch period. Each parcel sent out with a courier is called a consignment. Each consignment will be given a unique number and each courier will provide an algorithm for generating the number. At the end of the dispatch period a list of all consignment numbers need to be sent to each courier. Each courier has their own method for accepting the list i.e. UPS uses email, Fedex uses FTP. Build a structure using OOP to facilitate the implementation of the above scenario. It does not need to be a final product. Assume that your library is given to another developer to use who will build a front end for it that will have the following features: 1. Start a new batch 2. Add a consignment 3. End the batch /** * Interface CourierInterface */ interface CourierInterface { /** * Fetch a consignment number * @return int */ public function fetchConsignmentNumber(); /** * Dispatch a consignment number to a courier */ public function dispatch($number); } class FedexCourier implements CourierInterface { /** * @var int */ private static $count = 10000; /** * We will use a simple static counter here to return a number * @return int */ public function fetchConsignmentNumber() { self::$count++; return self::$count; } /** * Send to courier * @param $number * @return string */ public function dispatch($number) { } } class Consignment { /** * @var CourierInterface */ protected $courier; /** * @var int $number Should contain a consignment number */ protected $number; /** * Consignment constructor. * @param CourierInterface $courier */ public function __construct(CourierInterface $courier) { $this->courier = $courier; // fetch a consignment number from the courier $this->number = $this->courier->fetchConsignmentNumber(); } /** * Simple getter * @return int */ public function getNumber() { return $this->number; } /** * @return mixed */ public function process() { return $this->courier->dispatch($this->number); } } class Batch { /** * @var array */ protected $consignments = []; /** * Batch constructor. * Start the dispatch period * This could take in a storage dependency for persistence */ public function __construct() { } /** * Add a consignment to the current batch * * @param Consignment $consignment */ public function addConsignment(Consignment $consignment) { $this->consignments[] = $consignment; } /** * Process each consignment */ public function endDispatchPeriod() { // loop through each of the consignments // the storage dependency could be use here to track the consignment numbers // i.e. $c->getNumber() foreach ($this->consignments as $c) { $c->process(); } } } Client <?php // client code // start a new batch $batch = new Batch(); // add some consignments $parcel1 = new Consignment(new FedexCourier()); $batch->addConsignment($parcel1); $parcel2 = new Consignment(new FedexCourier()); $batch->addConsignment($parcel2); // end the batch $batch->endDispatchPeriod(); ?>
-
You are using PHP 4 syntax that is obsolete. You should not use the var keyword, nor should you give a method the same name as the class. In PHP 4 this was how you defined the constructor. I suggest you read up on how to use classes in PHP 5. Refactored <?php class Customer extends VerySimpleModel implements TemplateVariable { public static $meta = array( 'table' => CUST_TABLE, 'pk' => array( 'cust_id' ), 'joins' => array( 'location' => array( 'constraint' => array( 'cust_id' => 'loc.cust_id' ), ), 'service' => array( 'constraint' => array( 'service_level' => 'service.id' ), ), ), ); public $id; public $name; public $contact; public $fname; public $lname; public $contact_phone; public $phone_number; public $fax_number; public $location; public $domain; public $service_level; public $row; public $address; private $_location = null; public function getCustomer( $id = 0 ) { echo 'here'; $this->id=0; if($id && ($info=$this->getInfoById($id)) ){ $this->row=$info; $this->id=$info["id"]; $this->name=$info["cust_name"]; $this->domain=$info["domain"]; $this->location=$this->getLocationInfo($id); $this->service_level=$info["service_level"]; } } } //page that is loading the Grid data $custs = Customer::objects();
-
Have a look here https://github.com/ziadoz/awesome-php Most of these will be available through packagist
- 3 replies
-
- open-source
- packagist
-
(and 1 more)
Tagged with:
-
Legend! Had to make a slight mod as, 'quantity' was ambiguous. I owe you a pint, cheers! SELECT product_model_id,model_code,GROUP_CONCAT(qty, ':', total_ordered ORDER BY qty ASC) AS qty_orders FROM product_model LEFT JOIN ( SELECT product_model_id, quantity AS qty, COUNT(op_id) AS total_ordered FROM order_product GROUP BY product_model_id, qty ) AS sq USING (product_model_id) GROUP BY product_model_id;
- 2 replies
-
- mysql
- group_concat
-
(and 1 more)
Tagged with:
-
I am trying to generate a report that will assist a client in determining price changes for their products. The query I need to use is bugging the hell out of me. I have extracted a portion of it as the other parts are irrelevant. What I am trying to do is display the number of times a product has been ordered of a certain quantity. I am attempting to concatenate the quantity with the number ordered. i.e. product_model_id model_code qty_orders ---------------------- --------------- ------------- 12 12345 1:32,2:12,3:2 So for the above product (12) the following info is returned A quantity of 1 has been ordered 32 times A quantity of 2 has been ordered 12 times A quantity of 3 has been ordered 2 times I can pull the qty_orders data out without issue by specifying the id of the product, however I need to display for all products including the additional information (product_model_id, model_code). Here is the database structure: product_model ------------- product_model_id model_code order_product ------------- op_id order_id product_model_id quantity I know that I need to do the sub query within a join but I can't get it quite right. Here is the part I have down. I need to get rid of the WHERE product_model_id=12 bit and create a join to product_model so I can display all products. SELECT GROUP_CONCAT(quantity, ':', total_ordered) AS qty_orders FROM ( SELECT quantity, COUNT(op_id) AS total_ordered FROM order_product WHERE product_model_id=12 GROUP BY quantity ) AS sq Complex SQL isn't really my forte so any help would be appreciated.
- 2 replies
-
- mysql
- group_concat
-
(and 1 more)
Tagged with:
-
1. Remove any PHP / Server Side code 2. Strip out any <img> tags 3. Strip out any <script> tags 4. Strip out any <link> tags to CSS files Job done. Should run like lightning!
-
Also consider Yii http://www.yiiframework.com/
-
how to upload website created with php and mysql
JonnoTheDev replied to computernerd21's topic in MySQL Help
Yes Don't see any reason why not as long as you haven't hard coded any paths to files / folders using Windows format You can export the database and re-import on the live server. Does vertrigo come with the phpmyadmin package to manage your databases. If so use this to export the database. When you have a shared server hosting package it will come with a control panel that you can log into to setup various things including mysql databases. There will be a page within the control panel for you to create a database and create a database user. Once this is done you will get an option to manage the database. This will simply take you to phpmyadmin where you can import the structure and data from your local copy. You will need to edit your connection details in your code so it matches the username / password / database name that you setup in your control panel.- 4 replies
-
- website upload
- hosting
-
(and 3 more)
Tagged with:
-
Have you tried a simple test script to check the connection to the servers by simply just using: $address = "tcp://www.example.com:80"; $timeout = 30; if(!$fp = stream_socket_client($address, $errno, $errstr, $timeout)) { echo $errstr . "(" . $errno . ")"; exit(); } echo "ok";
-
how to upload website created with php and mysql
JonnoTheDev replied to computernerd21's topic in MySQL Help
As straight forward and to the point as I can be. vertrigoserv is a web environment for your desktop computer so you can execute php code and have mysql databases, etc. Forget that you are using it for development as many people build websites and run them from their desktop computers and then upload them to a live web server later on. Here's the basics on what you need to do. 1. Buy a domain name (i.e. from godaddy.com or another reseller) 2. Find a web host. This is a company that hosts servers where your website will sit and may offer other services such as email for your domain name. You can buy hosting from all over the place for your budget, just do a Google search. If you are on a small budget then you probably want to look for a simple shared hosting package using LAMP (Linux, Apache, MySQL, PHP). i.e. you share the server with other people and it usually comes with a simple control panel that you can setup websites, email, mysql databases, etc. from. 3. Find out what the nameservers are for the webhost you choose i.e. NS1.MYWEBHOST.COM & NS2.MYWEBHOST.COM and point your domain name at them. You can do this from the website that you bought your domain name from. You may have a my account area where you can manage your domain names. You can then follow the instructions you get from your hosting company to add your domain into your account. Once you do this the folders should be setup to upload your website files to. 4. Setup any mysql databases you need from your server control panel 5. Setup an FTP account so you can upload your website files if this is not done for you automatically on your server control panel 6. Download FTP software such as Filezilla. You can even use Dreamweavers built in FTP functionality if you are familiar with it and enter the FTP details for your server. 7. Upload the PHP, etc. files to your server in the correct folder. This is called the website document root. On shared servers it is usually a folder labelled www or public_html 8. Type your domain into your web browser and away you go. Note that changing the nameservers for a domain i.e. pointing the domain to your webhost can take upto 24 hours to complete. Also websites that sell domain names may also offer web hosting as part of a package when you buy your domain name. To keep everything in one place this maybe the simplest option but do some research on the reliability and the quality of the support that the company offers because there are a lot of poor webhosts out there.- 4 replies
-
- website upload
- hosting
-
(and 3 more)
Tagged with:
-
Making documents only accessible to specific users?
JonnoTheDev replied to Kristoff1875's topic in MySQL Help
If you have users then you need to authenticate a user before they are allowed to upload or download a file. i.e. They need to login to the website. If your users are stored in a database table then each user will have an id (primary key). Store the uploaded filenames in a database table along with the id of the user who uploaded them. A user should only be allowed to download their own files. i.e. If my user id is 123 I can only download files where user_id = 123 Simple database schema user ====== user_id name username password user_file ======= file_id user_id filename You only need to split the folders up if you are going to have thousands of files uploaded otherwise just upload the files to a folder that it outside of the website document root. -
On another note, to answer your question OOP and Procedural Programming are terms used to describe code structure. There is no, "one is better than the other". Each is suited to particular scenarios. Procedural code basically refers to line by line structure meaning you write code from the top to the bottom in the order it is to be executed i.e 1. check if a form has been submitted 2. validate the data 3. display any errors 4. if no errors save the data to the database and redirect the user 5. display the form OOP refers to a system where all the component parts are separated into what are known as objects i.e. users, database, forms, apples, oranges, pears, etc. Objects can interact with each other i.e. A user object can use a database object that will allow it to generate a fruit object that can be an apple, an, orange or a pear. If you were to try and write this sort of behavior in a procedural style you may think ah, easy. However, what if I want to change the type of database I am using, what if I want to add a new type of fruit, or add extra types of forms. You are going to have to add to or edit many lines of code to achieve this and may end up forgetting to alter one line in a certain page of your site breaking the whole application. Procedural code can quickly become spaghetti code that is difficult to read an debug. This is where OOP would be better suited. I use both types of programming every day. If I am churning out a small website with a contact form, a gallery, maybe a blog. I would write the majority of it procedurally. I'm going to use a few objects here and there i.e using the mysqli object for connecting to and running database queries. There is no need for OOP in this scenario, procedural is the better choice. If I have the task of writing an e-commerce application that may have a multi user CMS that requires role based access controls, many types of forms and lots of different types of data to validate, and include an API for third party apps to connect into and extract data. I am certainly not going to write this in a line by line Procedural style, especially if there will be more than one developer working on it. Something like this will grow and I will want to make it as extendable as possible without having to break any of the core code. This is where OOP is perfect, the better choice. In fact you have stated that you have used the CodeIgniter framework. This my friend is OOP. Again, I would not use a framework for a small task just because I know how to.
- 3 replies
-
- oop
- frameworks
-
(and 2 more)
Tagged with:
-
Welcome to the community. Don't feel like your on your own when it comes to the way you have progressed. Usually a developer will only progress as far as the company they work for requires them to (unless you are using your free time to extend your knowledge). i.e. If your company is churning out websites that don't need masses of programming code then why would the company need a developer to invest their time into learning a framework. It's good that you have decided to broaden your skills by learning about frameworks and OOP on your own. You will certainly get the help you need here. I would certainly not shy away from learning frameworks. I think it it good to have worked with 2 frameworks to get an idea of the pros and cons of each. You will find that they will overlap in terms of how code is laid out in a lot of parts. You already have CodeIgniter under your belt, and that is great. It has one of the best sets of documentation out of all of the frameworks and that is important. Forget about what the critics say, at the end of the day you are learning and you can make your own mind up. If you're looking for the next framework to learn that is the next step up from CI then I would strongly recommend Yii (not version 2 yet) http://www.yiiframework.com/ It has good documentation, a strong community, and lots of tutorials on youtube, etc. I moved onto Yii from using CodeIgniter for quite a few projects and haven't looked back. I would also get a copy of the following eBook to read through http://yii.larryullman.com/
- 3 replies
-
- oop
- frameworks
-
(and 2 more)
Tagged with:
-
Larry Ullman's material is good. I am going through his Yii book at the minute.