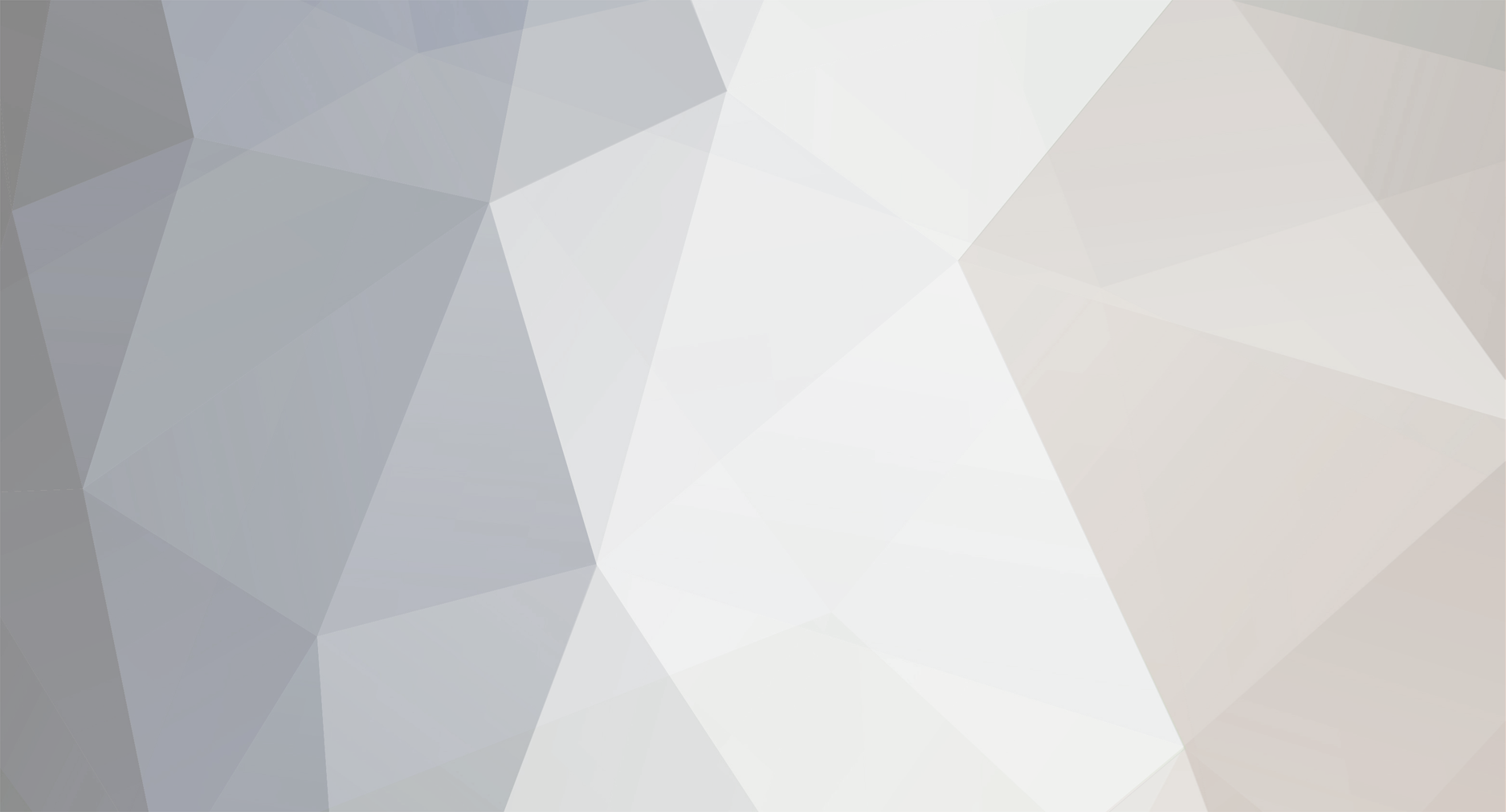
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
If you also want to return results that are only 3 characters, you need to edit the minimum word length in mysql. In /etc/my.cnf or /etc/mysql/my.cnf under the [mysqld] section, have this line: ft_min_word_len = 3 Then do a quick repair on the table REPAIR TABLE table_name QUICK;
-
I found a similar function online and changed it for the better. You can run this to test the function out. <?php $number = trim($_POST['number']); $denominator = trim($_POST['denominator']); if(!isset($_POST['denominator'])){ $denominator = 32; } ?> <html> <body> <form action="" method="post"> Number: <input type="text" name="number" value="<?php echo $number;?>"> Highest denominator: <select name="denominator"> <option VALUE="<?php echo $denominator; ?>"><?php echo $denominator; ?></option> <option VALUE="2"> 2</option> <option VALUE="3"> 3</option> <option VALUE="4"> 4</option> <option VALUE="8"> 8</option> <option VALUE="16"> 16</option> <option VALUE="24"> 24</option> <option VALUE="32"> 32</option> <option VALUE="48"> 48</option> <option VALUE="64"> 64</option> <option VALUE="100"> 100</option> <option VALUE="1000"> 1000</option> </select> <input type="submit"> </form> </body> </html> <?php function decimalToFraction($number, $max_denom=NULL) { //is there a number in it? if(!preg_match('#[0-9]#',$number)){ return $number; exit(); } else { //if is already a fraction, change back to decimal if(preg_match ('~/~', $number)){ $fraction = array('whole' => 0); preg_match('/^((?P<whole>\d+)(?=\s))?(\s*)?(?P<numerator>\d+)\/(?P<denominator>\d+)$/', $number, $fraction); $number = $fraction['whole'] + $fraction['numerator']/$fraction['denominator']; } $whole = floor($number); $decimal = $number - $whole; $denominators = array(2, 3, 4, 8, 16, 24, 32, 48, 64, 100, 1000);//add or remove highest denominations from array to your liking if(!is_null($max_denom) && is_numeric($max_denom)){ $leastCommonDenom = $max_denom; } else { $leastCommonDenom = max($denominators); } $roundedDecimal = round($decimal * $leastCommonDenom) / $leastCommonDenom; if($roundedDecimal == 0) return $whole; if($roundedDecimal == 1) return $whole + 1; foreach($denominators as $d) { if($roundedDecimal * $d == floor($roundedDecimal * $d)) { $denom = $d; break; } } return ($whole == 0 ? '' : $whole) . " " . ($roundedDecimal * $denom) . "/" . $denom; } } if(isset($_POST['number']) && $number != ''){ $result = decimalToFraction($number, $denominator); if (preg_match('#[0-9]#',$result)){ echo $result; } else { echo "$result is not a number."; } }else { echo "Insert a number to convert to a fraction."; } ?>
-
or this $theusername = trim($_POST['username']); if(isset($_POST['username']) && $theusername == '' ){ die(header("Location: login.php?error=allfields")); } same thing, just one less line
-
try this $theusername = trim($_POST['username']); if(isset($_POST['username']) && $theusername == '' ){ header("Location: login.php?error=allfields"); die(); }
-
add the line I posted like so $date = $xml->posts->post ['date']; $date = date("D, d M Y", strtotime($date)); echo '<p>'.$date.'</p>';
-
There are still other searches and website indexes that still use the meta keywords. Also for Google News-accredited sites, this is a fairly new one. <meta name="news_keywords" content="Insert your keywords here">
-
This should do it $date = date("D, d M Y", strtotime($date));
-
best way of geting url form user and validate them...
QuickOldCar replied to thara's topic in PHP Coding Help
When dealing with urls, I found the best way to validate them is to run them through curl, you can follow them to actual urls after redirects, check for response codes that they are able to connect. Here's an example, if you want to turn this into a true/false function to just do checking...that can work fine. There is some extra values you may not need, but are there in case you want them. <?php $url = "https://godaddy.com/"; /* connect to the url using curl to see if exists and get the information */ //$cookie = tempnam('tmp','cookie'); //$cookie_file_path = "tmp/"; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); //curl_setopt($ch, CURLOPT_COOKIEJAR, $cookie); //curl_setopt($ch, CURLOPT_COOKIEFILE, $cookie_file_path); curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/6.0 (Windows NT 6.2; WOW64; rv:16.0.1) Gecko/20121011 Firefox/16.0.1'); curl_setopt($ch, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1); curl_setopt($ch, CURLOPT_TIMEOUT, 10); curl_setopt($ch, CURLOPT_MAXREDIRS, 15); curl_setopt($ch, CURLOPT_HEADER, 1); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_AUTOREFERER, true); curl_setopt($ch, CURLOPT_FILETIME, 1); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE); curl_setopt($ch, CURLOPT_ENCODING, ""); $curl_session = curl_init(); //curl_setopt($curl_session, CURLOPT_COOKIEJAR, $cookie); //curl_setopt($curl_session, CURLOPT_COOKIEFILE, $cookie_file_path); curl_setopt($curl_session, CURLOPT_USERAGENT, 'Mozilla/6.0 (Windows NT 6.2; WOW64; rv:16.0.1) Gecko/20121011 Firefox/16.0.1'); curl_setopt($curl_session, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1); curl_setopt($curl_session, CURLOPT_ENCODING, ""); curl_setopt($curl_session, CURLOPT_TIMEOUT, 10); curl_setopt($curl_session, CURLOPT_HEADER, 1); curl_setopt($curl_session, CURLOPT_SSL_VERIFYPEER, FALSE); curl_setopt($curl_session, CURLOPT_HEADER, true); curl_setopt($curl_session, CURLOPT_MAXREDIRS, 15); curl_setopt($curl_session, CURLOPT_RETURNTRANSFER, true); curl_setopt($curl_session, CURLOPT_AUTOREFERER, true); curl_setopt($curl_session, CURLOPT_HTTPGET, true); curl_setopt($curl_session, CURLOPT_URL, $url); $string = mysql_real_escape_string(curl_exec($curl_session)); $html = mysql_real_escape_string(curl_exec($ch)); $response = curl_getinfo($ch); $valid_url = "Invalid"; $valid_array = array(200, 201, 202, 203, 204, 205, 206, 207, 300, 301, 302, 303, 304, 305, 306, 307); if (in_array($response['http_code'], $valid_array)) { $valid_url = "Valid"; ini_set("user_agent", "Mozilla/5.0 (Windows NT 6.1; WOW64; rv:12.0) Gecko/20100101 Firefox/12.0"); $headers = @get_headers($response['url']); //print_r($headers); if (!$headers) { die("Unable to fetch website."); } $location = ""; foreach ($headers as $value) { if (substr(strtolower(trim($value)), 0, 9) == "location:") { if ($value == "Location: /") { $value = $url; return $value; } else { return trim(substr($value, 9, strlen($value))); } } } } if (preg_match("/window\.location\.replace\('(.*)'\)/i", $html, $value) || preg_match("/window\.location\=[\"'](.*)[\"']/i", $html, $value) || preg_match("/location\.href\=[\"'](.*)[\"']/i", $html, $value) ) { $finalurl = $value[1]; } else { $finalurl = $response['url']; } //show the final url and response code echo $finalurl."<br />"; echo $response['http_code']." - ".$valid_url."<br />"; ?> -
this might help you to track it down http://tools.pingdom.com/fpt/
-
Happens all the time, it could be anything from a slow query, not able to load an image, or accessing slow 3rd party sites are linked to. I guess if you post the link, people can look at it and might see what is wrong.
-
Need Advice & Understanding - xml sitemaps & google SEO
QuickOldCar replied to yandoo's topic in Miscellaneous
If you want them included you should hyperlink them so they can be found. -
It doesn't matter so much what theme you decide on. What does matter is the payment methods you are looking to integrate into your site. You can follow a tutorial like this to integrate paypal into your site. http://wp.tutsplus.com/tutorials/creative-coding/wordpress-and-paypal-an-introduction/ Or even add a shopping cart plugin to handle it. http://wordpress.org/extend/plugins/wp-e-commerce/
-
You can try using Rolling Curl to do this. https://github.com/takinbo/rolling-curl
-
In case want rgb function html2rgb($color) { if ($color[0] == '#') $color = substr($color, 1); if (strlen($color) == 6) list($r, $g, $B) = array($color[0] . $color[1], $color[2] . $color[3], $color[4] . $color[5]); elseif (strlen($color) == 3) list($r, $g, $B) = array($color[0] . $color[0], $color[1] . $color[1], $color[2] . $color[2]); else return false; $r = hexdec($r); $g = hexdec($g); $b = hexdec($B); return array($r, $g, $B); }
-
it couldn't do fullscreen, did you add the parameter? allowfullscreen='true'
-
I made the exact thing you are looking for and did lots of experimenting along the way. http://dynaindex.com/snapshot.php Take a look at these 2 posts for what I suggested in the past and links to information about it. http://forums.phpfreaks.com/topic/261536-screenshot-software-for-windows-your-recs/?do=findComment&comment=1340333 http://www.phpfreaks.com/forums/index.php?topic=352033.msg1662293#msg1662293
-
I didn't make that script. But i may have an answer for moves. if is a noun, it's move, if is a verb it's moves. Either way there are many missing from the script, here are over 100 for instances http://www.scribd.co...ouns-in-English di,dice octopus,octopi woman,women child,children These remain the same plural and singular bison buffalo deer fish moose pike sheep salmon trout swine plankton I haven't seen an all working solution that's 100% correct when it comes to making a word plural or singular. There will even be variations or accepted usages of plural forms depending where it originates.
-
someone made a class that has pluralize and singular in it. http://www.kavoir.com/2011/04/php-class-converting-plural-to-singular-or-vice-versa-in-english.html Here is a fixed version <?php /* vim: set expandtab tabstop=4 shiftwidth=4 softtabstop=4: */ // +----------------------------------------------------------------------+ // | Akelos PHP Application Framework | // +----------------------------------------------------------------------+ // | Copyright (c) 2002-2006, Akelos Media, S.L. http://www.akelos.com/ | // | Released under the GNU Lesser General Public License | // +----------------------------------------------------------------------+ // | You should have received the following files along with this library | // | - COPYRIGHT (Additional copyright notice) | // | - DISCLAIMER (Disclaimer of warranty) | // | - README (Important information regarding this library) | // +----------------------------------------------------------------------+ /** * Inflector for pluralize and singularize English nouns. * * This Inflector is a port of Ruby on Rails Inflector. * * It can be really helpful for developers that want to * create frameworks based on naming conventions rather than * configurations. * * It was ported to PHP for the Akelos Framework, a * multilingual Ruby on Rails like framework for PHP that will * be launched soon. * * @author Bermi Ferrer Martinez * @copyright Copyright (c) 2002-2006, Akelos Media, S.L. http://www.akelos.org * @license GNU Lesser General Public License * @since 0.1 * @version $Revision 0.1 $ */ class Inflector { // ------ CLASS METHODS ------ // // ---- Public methods ---- // // {{{ pluralize() /** * Pluralizes English nouns. * * @access public * @static * @param string $word English noun to pluralize * @return string Plural noun */ function pluralize($word) { $plural = array( '/(quiz)$/i' => '\1zes', '/^(ox)$/i' => '\1en', '/([m|l])ouse$/i' => '\1ice', '/(matr|vert|ind)ix|ex$/i' => '\1ices', '/(x|ch|ss|sh)$/i' => '\1es', '/([^aeiouy]|qu)ies$/i' => '\1y', '/([^aeiouy]|qu)y$/i' => '\1ies', '/(hive)$/i' => '1s', '/(?[^f])fe|([lr])f)$/i' => '\1\2ves', '/sis$/i' => 'ses', '/([ti])um$/i' => '\1a', '/(buffal|tomat)o$/i' => '\1oes', '/(bu)s$/i' => '\1ses', '/(alias|status)/i'=> '\1es', '/(octop|vir)us$/i'=> '\1i', '/(ax|test)is$/i'=> '\1es', '/s$/i'=> 's', '/$/'=> 's'); $uncountable = array('equipment', 'information', 'rice', 'money', 'species', 'series', 'fish', 'sheep'); $irregular = array( 'person' => 'people', 'man' => 'men', 'child' => 'children', 'sex' => 'sexes', 'move' => 'moves'); $lowercased_word = strtolower($word); foreach ($uncountable as $_uncountable){ if(substr($lowercased_word,(-1*strlen($_uncountable))) == $_uncountable){ return $word; } } foreach ($irregular as $_plural=> $_singular){ if (preg_match('/('.$_plural.')$/i', $word, $arr)) { return preg_replace('/('.$_plural.')$/i', substr($arr[0],0,1).substr($_singular,1), $word); } } foreach ($plural as $rule => $replacement) { if (preg_match($rule, $word)) { return preg_replace($rule, $replacement, $word); } } return false; } // }}} // {{{ singularize() /** * Singularizes English nouns. * * @access public * @static * @param string $word English noun to singularize * @return string Singular noun. */ function singularize($word) { $singular = array ( '/(quiz)zes$/i' => '\1', '/(matr)ices$/i' => '\1ix', '/(vert|ind)ices$/i' => '\1ex', '/^(ox)en/i' => '\1', '/(alias|status)es$/i' => '\1', '/([octop|vir])i$/i' => '\1us', '/(cris|ax|test)es$/i' => '\1is', '/(shoe)s$/i' => '\1', '/(o)es$/i' => '\1', '/(bus)es$/i' => '\1', '/([m|l])ice$/i' => '\1ouse', '/(x|ch|ss|sh)es$/i' => '\1', '/(m)ovies$/i' => '\1ovie', '/(s)eries$/i' => '\1eries', '/([^aeiouy]|qu)ies$/i' => '\1y', '/([lr])ves$/i' => '\1f', '/(tive)s$/i' => '\1', '/(hive)s$/i' => '\1', '/([^f])ves$/i' => '\1fe', '/(^analy)ses$/i' => '\1sis', '/((a)naly|(b)a|(d)iagno|(p)arenthe|(p)rogno|(s)ynop|(t)he)ses$/i' => '\1\2sis', '/([ti])a$/i' => '\1um', '/(n)ews$/i' => '\1ews', '/s$/i' => '', ); $uncountable = array('equipment', 'information', 'rice', 'money', 'species', 'series', 'fish', 'sheep'); $irregular = array( 'person' => 'people', 'man' => 'men', 'child' => 'children', 'sex' => 'sexes', 'move' => 'moves'); $lowercased_word = strtolower($word); foreach ($uncountable as $_uncountable){ if(substr($lowercased_word,(-1*strlen($_uncountable))) == $_uncountable){ return $word; } } foreach ($irregular as $_plural=> $_singular){ if (preg_match('/('.$_singular.')$/i', $word, $arr)) { return preg_replace('/('.$_singular.')$/i', substr($arr[0],0,1).substr($_plural,1), $word); } } foreach ($singular as $rule => $replacement) { if (preg_match($rule, $word)) { return preg_replace($rule, $replacement, $word); } } return $word; } // }}} // {{{ titleize() /** * Converts an underscored or CamelCase word into a English * sentence. * * The titleize function converts text like "WelcomePage", * "welcome_page" or "welcome page" to this "Welcome * Page". * If second parameter is set to 'first' it will only * capitalize the first character of the title. * * @access public * @static * @param string $word Word to format as tile * @param string $uppercase If set to 'first' it will only uppercase the * first character. Otherwise it will uppercase all * the words in the title. * @return string Text formatted as title */ function titleize($word, $uppercase = '') { $uppercase = $uppercase == 'first' ? 'ucfirst' : 'ucwords'; return $uppercase(Inflector::humanize(Inflector::underscore($word))); } // }}} // {{{ camelize() /** * Returns given word as CamelCased * * Converts a word like "send_email" to "SendEmail". It * will remove non alphanumeric character from the word, so * "who's online" will be converted to "WhoSOnline" * * @access public * @static * @see variablize * @param string $word Word to convert to camel case * @return string UpperCamelCasedWord */ function camelize($word) { return str_replace(' ','',ucwords(preg_replace('/[^A-Z^a-z^0-9]+/',' ',$word))); } // }}} // {{{ underscore() /** * Converts a word "into_it_s_underscored_version" * * Convert any "CamelCased" or "ordinary Word" into an * "underscored_word". * * This can be really useful for creating friendly URLs. * * @access public * @static * @param string $word Word to underscore * @return string Underscored word */ function underscore($word) { return strtolower(preg_replace('/[^A-Z^a-z^0-9]+/','_', preg_replace('/([a-zd])([A-Z])/','\1_\2', preg_replace('/([A-Z]+)([A-Z][a-z])/','\1_\2',$word)))); } // }}} // {{{ humanize() /** * Returns a human-readable string from $word * * Returns a human-readable string from $word, by replacing * underscores with a space, and by upper-casing the initial * character by default. * * If you need to uppercase all the words you just have to * pass 'all' as a second parameter. * * @access public * @static * @param string $word String to "humanize" * @param string $uppercase If set to 'all' it will uppercase all the words * instead of just the first one. * @return string Human-readable word */ function humanize($word, $uppercase = '') { $uppercase = $uppercase == 'all' ? 'ucwords' : 'ucfirst'; return $uppercase(str_replace('_',' ',preg_replace('/_id$/', '',$word))); } // }}} // {{{ variablize() /** * Same as camelize but first char is underscored * * Converts a word like "send_email" to "sendEmail". It * will remove non alphanumeric character from the word, so * "who's online" will be converted to "whoSOnline" * * @access public * @static * @see camelize * @param string $word Word to lowerCamelCase * @return string Returns a lowerCamelCasedWord */ function variablize($word) { $word = Inflector::camelize($word); return strtolower($word[0]).substr($word,1); } // }}} // {{{ tableize() /** * Converts a class name to its table name according to rails * naming conventions. * * Converts "Person" to "people" * * @access public * @static * @see classify * @param string $class_name Class name for getting related table_name. * @return string plural_table_name */ function tableize($class_name) { return Inflector::pluralize(Inflector::underscore($class_name)); } // }}} // {{{ classify() /** * Converts a table name to its class name according to rails * naming conventions. * * Converts "people" to "Person" * * @access public * @static * @see tableize * @param string $table_name Table name for getting related ClassName. * @return string SingularClassName */ function classify($table_name) { return Inflector::camelize(Inflector::singularize($table_name)); } // }}} // {{{ ordinalize() /** * Converts number to its ordinal English form. * * This method converts 13 to 13th, 2 to 2nd ... * * @access public * @static * @param integer $number Number to get its ordinal value * @return string Ordinal representation of given string. */ function ordinalize($number) { if (in_array(($number % 100),range(11,13))){ return $number.'th'; }else{ switch (($number % 10)) { case 1: return $number.'st'; break; case 2: return $number.'nd'; break; case 3: return $number.'rd'; default: return $number.'th'; break; } } } // }}} } ?>
-
You can use what's called stemming to include the plural words as well within the search results. http://sphinxsearch.com/docs/current.html#conf-morphology And for mysql the Porter stemmer is popular to use http://dev.mysql.com/worklog/task/?id=2423 You can use a thesaurus, produce an array of similar definition words and provide all the results. As for ending in ing, es, ed, etc.. , would need to make word associations, could be time consuming to create, or can try using a wildcard with * Wordnik has a free thesaurus api you can use, has some limitations, is a pro version too. http://developer.wordnik.com/ Is also wordnet that can do them, is an online version, also can install your own local version. http://wordnet.princeton.edu/ It would take a real long time to explain how exactly I do my various searches, but I use sphinx to search for links, mysql fulltext in booleon mode for my simple site search, and then if are a member I let them use various advanced searches to narrow down exactly what they need. Here's a simple search using a combination of wordnick thesaurus with also my own local version of wordnet as suggestions. Instead of suggestions like I did, you could add the same into a query. I chose not to because some of the meanings are most likely not what they are looking for. http://dynaindex.com/search.php?s=jobs One last item that may be of interest to you is ajax based suggestions, which could also be used as the query. http://robertnyman.com/ajax-suggestions/ http://www.w3schools.com/php/php_ajax_php.asp Personally your best bet may be using sphinx, it's well documented and also articles written to do additional or special searches with it.
-
Fultext is most likely the most advanced can get with it, with indexing can fetch fairly fast results depending on the amount of data. Not sure if you looked into all the operators or weighting methods. http://dev.mysql.com/doc/refman/5.6/en/fulltext-boolean.html Take a look into sphinx, it can provide some advanced and also fast search results. http://sphinxsearch.com/ I wrote a few posts for fulltext search and gave some examples on some advanced search and multiple dynamic queries. http://forums.phpfreaks.com/topic/262145-best-search-algorithm-to-accomplish-this/?do=findComment&comment=1343591
-
It seems the code had a hard time displaying this geoplugin.net/php.gp?ip='.$ip
-
As Christian said, not reliably. http://www.maxmind.com/en/geolocation_landing http://www.geoplugin.net can try this $ip = $_SERVER['REMOTE_ADDR']; //if running this code locally, to get a result if($ip == $_SERVER['SERVER_ADDR']){ $ip = $_SERVER['SERVER_NAME']; } echo $ip."<br />"; $geolocation = unserialize(file_get_contents('http://www.geoplugin.net/php.gp?ip='.$ip)); $geo_city = trim($geolocation['geoplugin_city']); $geo_country = $geolocation['geoplugin_countryName']; $location = $geo_city.",".$geo_country; echo "Location: ".$location."<br />"; What you do with their city,country info would be up to you, save it to a user session or database