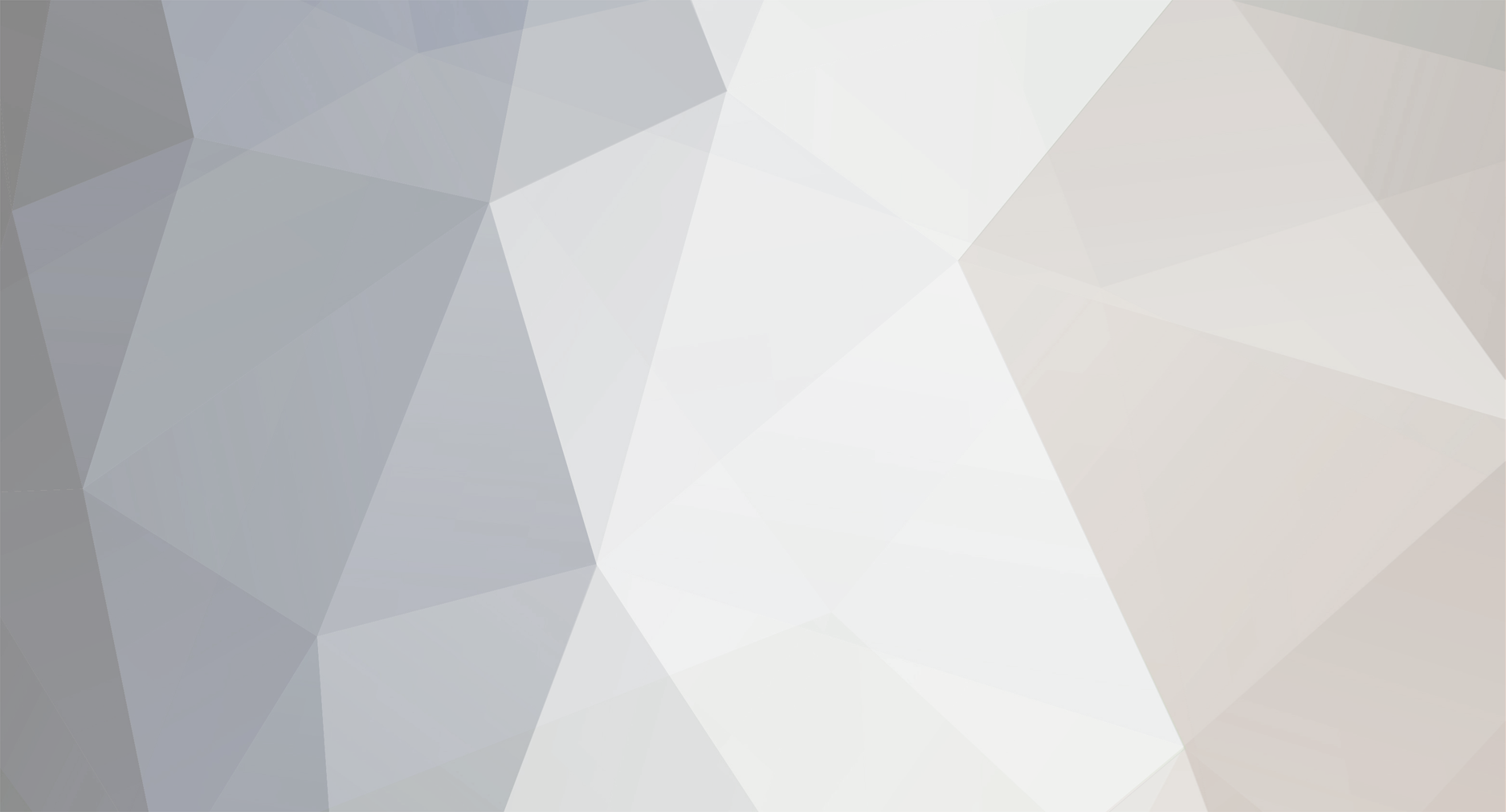
jazzman1
Staff Alumni-
Posts
2,713 -
Joined
-
Last visited
-
Days Won
12
Everything posted by jazzman1
-
You should avoid parsing a HTML content like in this example in php because of performance in the language!
-
Why in the loop David? Call the mail function only once and implode outgoing addresses as in my example above.
-
Save the html output of this searchable link into a file. Then grab the content (line by line) of this file and use DOM or some regEx to handle your desirable data. The script bellow I've written in BASH for my friend which searches open ports on the web ( for satellite TV's), you can get ideas. However, for sure you have to save somewhere this data before to handle it, not just to display it in the browser. #!/bin/bash echo -n 'Enter your ip address and its range: ' read b1 b2 r1 r2 if [ $r1 -lt 256 ] && [ $r2 -lt 256 ] && [ $b1 -lt 256 ] && [ $b2 -lt 256 ]; then if [ -f hosts.txt ];then rm -f hosts.txt fi START=$(date +%s) for n in $(seq $r1 $r2);do HOST="$b1.$b2.$n.0/24" nmap --max-retries 0 -p T:80,8080 $HOST | grep --basic-regexp 'Nmap scan report for' &>> hosts.txt done sort hosts.txt | grep --only-matching '[[:digit:]]*\.[[:digit:]]*\.[[:digit:]]*\.[[:digit:]]*' | sort --output=hosts.txt while read line; do curl --basic --max-time 1 $line | grep --perl-regexp '.*(enIgma weB Interface)' "--ignore-case" &> /dev/null if [ $? -eq 0 ]; then chmod 600 outputs echo $line &>> outputs fi done < hosts.txt clear sort --numeric-sort --unique --output=outputs outputs chmod 400 outputs END=$(date +%s) DIFF=$(expr $END - $START ) cat outputs echo "This script has been executed in $DIFF seconds" else echo 'There is something wrong! Please try again!' exit fi
- 5 replies
-
- preg_match
-
(and 2 more)
Tagged with:
-
Most of the chat programs on the web using javascript and AJAX to handle the data. I'm not entirely sure how you can manipulate JS by cURL.
-
If the emails are coming from your database in the array(), just implode them. For example: $to = array('example_1@example.com,example_2@example.com,example_3@example.com,example_4@example.com,example_5@example.com'); $emails= implode(',', $to)."\n"; if(mail($emails, $title,$body,$headers)) { echo 'OK.......mails was sent'; // redirect the page if you want it } else { echo 'ERROR: .........mails failed'; }
-
Add this: $result = mysql_query($getemail); // here while ($row = mysql_fetch_assoc($result)) { $to=$row['email']; } echo $to; exit; OR, $to = array(); while ($row = mysql_fetch_assoc($result)) { $to[] = $row['email']; } echo '<pre>'.print_r($to, true).'</pre>'; See the output and post the result here.
-
It sounds like, you didn't even bother trying to read what David wrote We are not here to re-write your entire code, just to guide you through it. Try to debug the script step by step as David is already suggested and come back again with some specific question.
-
What is difference in this code and the one from your first post?
-
Is it a dedicated or shared server? Something like that is off the top of my head ( using I/O redirect) <?php error_reporting(0); $str = "Incorrect username or password!<br /> Please verify your account at <a href=https://login.yahoo.com>https://login.yahoo.com</a> "; $mbox = imap_open('{imap.mail.yahoo.com:993/ssl/novalidate-cert}', 'userName@yahoo.com', "userPass"); if($mbox === FALSE){ echo shell_exec("php -f error_log.php ".escapeshellarg($str)); } error_log.php <?php echo $argv[1]; Result: Did you try using a php header location function?
-
I've never thought about this special feature, master
-
Can I see your code?
-
Or, UPDATE t1 SET MySqlDate = DATE_FORMAT(STR_TO_DATE(InputDate, '%d/%m/%Y'), '%Y-%m-%d'); It will be update the date column in format : YYYY-MM-DD (0000-00-00)
-
What debugging steps have you taken so far? I see many places in your code that could go wrong.
-
Don't forget to call the exit function after calling a header() function! EDIT: I meant location header
-
Try what Barand said to pass mysqli_assoc option: $found_user = $result->fetch_array(MYSQLI_ASSOC) OR $found_user = $result->fetch_assoc()
-
PHP provides(for better or for worse) a bunch of numbers of predefined variables. So, in this example you might be consider using $argc and $argv. Take a look at examples, I'm using a shell_exec() function instead exec(). launcher.php: <?php $argv[1] = "first argument"; $argv[2] = "second argument"; $simple = "simple"; echo shell_exec("php -f launched.php $argv[1] $argv[2] $simple"); launched.php var_dump($argc); // The number of arguments passed to script var_dump($argv); // Array of arguments passed to script As you can see the output above, in $argv[1] and $argv[2] the value is being treated by the shell command with two different arguments, b/s of the blank spaces between the words. You could create some custom function to escape this empty space with "\ " symbol. That's off the top of my head: $argv[1] = "first argument"; echo shell_exec("php -f launched.php ".EscapeEmptySequence($argv[1])); function EscapeEmptySequence($str){ $search = ' '; $replace = "\ "; return str_replace($search, $replace, $str); } Personally, I rarely use php to execute commands in the shell and prefer BASH instead!
-
Can you post the link to this tutorial, please?
- 17 replies
-
- html
- javascript
-
(and 1 more)
Tagged with:
-
There are all scripts that I used for the test. main.html <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" lang="en" xml:lang="en"> <head> <meta http-equiv="Content-Language" content="he" /> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <script type="text/javascript" src="scripts.js"></script> <title>textbox changing with ajax - php - version 1</title> </head> <body id='body'> <div id='div_textarea'></div> </body> </html> scripts.js var xhr = null; if (window.XMLHttpRequest) { // Mozilla, Safari, ... xhr = new XMLHttpRequest(); } else if (window.ActiveXObject) { // IE 8 and older xhr = new ActiveXObject("Microsoft.XMLHTTP"); } else { alert('No instance of a class that provides this functionality'); } xhr.onload = function() { document.getElementById('div_textarea').innerHTML = this.responseText; button_onload(); } function button_onload() { /* first version */ /* ------------- */ document.getElementById('button').innerHTML = 'button 1'; document.getElementById('button2').value = 'button 2'; document.getElementById('button3').value = 'clear/clean'; } /* fffff */ function button() { /* show what the textarea inside text */ //alert(document.getElementById('div_textarea_text').value); document.getElementById('div_textarea_text2').value = document.getElementById('div_textarea_text').value; } /* fffff */ function button2() { /* show what the textarea inside text */ //alert(document.getElementById('div_textarea_text').value); document.getElementById('div_textarea_text2').value = document.getElementById('div_textarea_text').value; } /* textarea functions */ /* ------------------ */ /* fffff */ function clear_textarea1_2() { clear_div_textarea_text(); clear_div_textarea_text2(); } /* fffff */ function clear_div_textarea_text() { document.getElementById('div_textarea_text').value = ''; } /* fffff */ function clear_div_textarea_text2() { document.getElementById('div_textarea_text2').value = ''; } /* fffff */ function disabled_div_textarea_text2() { document.getElementById('div_textarea_text2').disabled = true; document.getElementById('div_textarea_text3').readOnly = true; } xhr.open("post", "index.php",true); xhr.send(); index.php <?php $str1 = '<br />'; $str2 = '<textarea id="div_textarea_text"></textarea>'; $str3 = '<div id="button" onclick="button();"></div>'; $str4 = '<input type="button" onclick="button2();" id="button2" value="" />'; $str5 = '<textarea id="div_textarea_text2"></textarea>'; $str6 = '<input type="button" onclick="clear_textarea1_2();" id="button3" />'; $str7 = '<textarea id="div_textarea_text3"></textarea>'; echo($str1 . $str2 . $str1 . $str3 . $str1 . $str4 . $str1 . $str5 . $str1 . $str6 . $str1 . $str7);
- 17 replies
-
- html
- javascript
-
(and 1 more)
Tagged with:
-
No, AJAX doesn't work in that way. AJAX uses by default asynchronous method. That means sending the request and receiving the data from the server, it's not going to be immediately. That's why, it's always a good idea to use callBack functions and the part of the rest of the js code to be parsed from javascript after the result of this request is finished (success).
- 17 replies
-
- html
- javascript
-
(and 1 more)
Tagged with:
-
That happens, b/s you call this "button_onload()" function before to get the requested data from the server! Use my js code instead yours.
- 17 replies
-
- html
- javascript
-
(and 1 more)
Tagged with:
-
Yours all scripts look bad. Spend time and learn more about the good practice in javascript and php! Anyways...... you should call button_onload() function inside xhr.onload(). Try, var xhr = null; if (window.XMLHttpRequest) { // Mozilla, Safari, ... xhr = new XMLHttpRequest(); } else if (window.ActiveXObject) { // IE 8 and older xhr = new ActiveXObject("Microsoft.XMLHTTP"); } else { alert('No instance of a class that provides this functionality'); } xhr.onload = function() { document.getElementById('div_textarea').innerHTML = this.responseText; button_onload(); } function button_onload() { /* first version */ /* ------------- */ document.getElementById('button').innerHTML = 'button 1'; document.getElementById('button2').value = 'button 2'; document.getElementById('button3').value = 'clear/clean'; } /* fffff */ function button() { /* show what the textarea inside text */ //alert(document.getElementById('div_textarea_text').value); document.getElementById('div_textarea_text2').value = document.getElementById('div_textarea_text').value; } /* fffff */ function button2() { /* show what the textarea inside text */ //alert(document.getElementById('div_textarea_text').value); document.getElementById('div_textarea_text2').value = document.getElementById('div_textarea_text').value; } /* textarea functions */ /* ------------------ */ /* fffff */ function clear_textarea1_2() { clear_div_textarea_text(); clear_div_textarea_text2(); } /* fffff */ function clear_div_textarea_text() { document.getElementById('div_textarea_text').value = ''; } /* fffff */ function clear_div_textarea_text2() { document.getElementById('div_textarea_text2').value = ''; } /* fffff */ function disabled_div_textarea_text2() { document.getElementById('div_textarea_text2').disabled = true; document.getElementById('div_textarea_text3').readOnly = true; } xhr.open("post", "index.php",true); xhr.send(); PS: Learn more about Ajax from here. This is the one of mine favourite resource on the web.
- 17 replies
-
- html
- javascript
-
(and 1 more)
Tagged with:
-
This is not just a good machine it's a beast These confs look good for me. However, be careful b/s sometimes the problems aren't on the db and apache conf files but comes from bad scripts wrote by someone! Check out the log files (every day or more) and look carefully if you see something wrong or bad.
-
Before doing anything, backup this table
-
Does you hosting allows you to use opening php short tags "<?" instead of "<?php"? Why are you using exit() on the bottom? It terminates execution of the scrip ???
-
Can you show us the script of the spend.php file? Is there a php header() function inside this file?