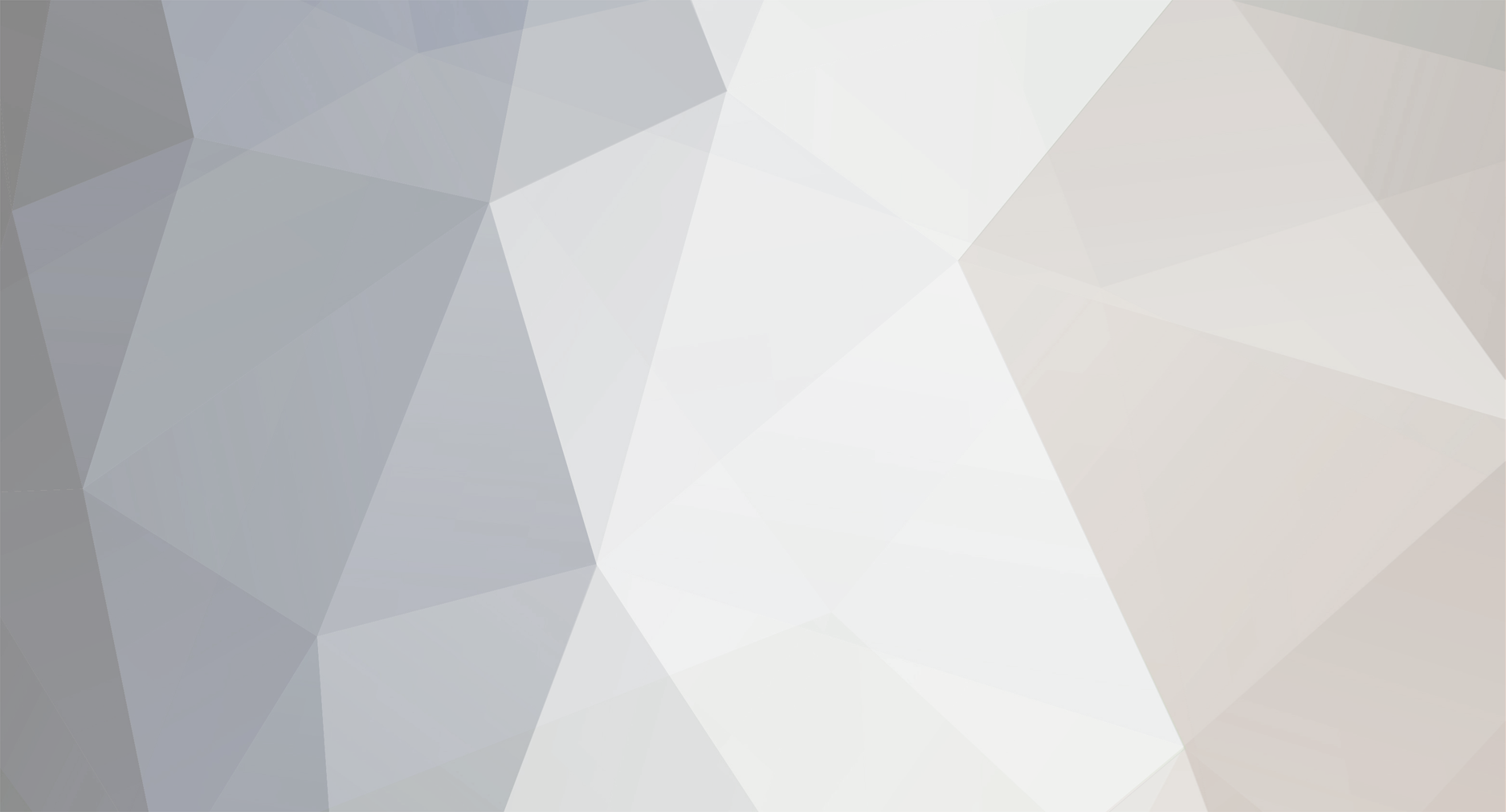
davidannis
Members-
Posts
627 -
Joined
-
Last visited
-
Days Won
2
Everything posted by davidannis
-
KevinM1 is right. Generally, the GET method should be used when the data is not changed (just a query and display) and POST used when the data is changed (records added, etc.) The reason it matters is that browsers will ask if a user is sure they want to resubmit if a POST is used and the user goes back in history but often just send the request again for a GET. It is explained in more detail here. You should still check for double submissions if they would cause a problem because the browser does not prevent them even with a POST. This article gives a good explanation of the differences with more detail including situations in which you may want to break the general rule.
-
If you want the results on the same page, not a new page then you need to use javascript and hide/show a div.
-
So you write a script called linktodisplay.php that does this: mysql_connect("localhost", "database_monitor", 'password') or die(mysql_error()); mysql_select_db("monitorr_acrisoft") or die(mysql_error()); $id=mysql_real_escape_stirng($_GET['id']); $result = mysql_query("SELECT * FROM prova WHERE ID= '$id'") or die (mysql_error()); ($mem_row = mysql_fetch_array( $result )){ echo "All of the info you want to echo"; }
-
to elaborate on Josh's answer and show how to link to a program to display the full record.
-
Put the codes into a database, once a code is used mark the record as used. I've done something similar where a user whose id was stored in $auth_id could vote only once, though in my example they could vote for up or down and change their vote. Here's the relevant bit of code from the up vote section. $id=$_POST['id']; $id = mysqli_real_escape_string($link, $id); $query="SELECT * FROM master_program_votes WHERE auth_id='".$_SESSION['auth_id']."' AND program_id='$id'"; if ($result= mysqli_query($link, $query)){ $master_program_votes= mysqli_fetch_assoc($result); //print_r($master_program_votes); if ($master_program_votes['auth_id']!=''){//user already voted once if ($master_program_votes['down_vote']==1) { //$vote_change=2; $update_query="UPDATE master_program SET up_votes=up_votes+1, down_votes=down_votes-1 WHERE program_id='$id'"; }else{ $no_vote_change=true;//they have already voted //$update_query="UPDATE master_program SET up_votes=up_votes+1 WHERE auth_id='".$_SESSION['auth_id']."' AND program_id='$id'"; } $master_program_votes['date']=date('Y-m-d'); $master_program_votes['up_vote']=1; $master_program_votes['down_vote']=0; $where = "WHERE auth_id='".$_SESSION['auth_id']."' AND program_id='$id'"; //print_r($master_program_votes); $update_result= dbRowUpdate('master_program_votes', $master_program_votes, $link, $where); }else{ $master_program_votes['date']=date('Y-m-d'); $master_program_votes['up_vote']=1; $master_program_votes['down_vote']=0; $master_program_votes['auth_id']=$_SESSION['auth_id']; $master_program_votes['program_id']=$id; dbRowInsertTEST('master_program_votes', $master_program_votes, $link); $update_query="UPDATE master_program SET up_votes=up_votes+1 WHERE program_id='$id'"; } }
-
If you are worried about using return because it stops the execution of the function and you want to output multiple things to the browser, either concatenate the strings or put them in an array and use return at the end of the function then print them at the appropriate point in the program.
-
I am not advocating that you use superglobals as a way to pass data in and out of functions, just pointing out that they break the rule of encapsulation. When I have a lot of values to pass out of a function my approach is often to build an array and use the array as a return value. What do you think of that approach?
-
Note that you can always access superglobals like $_SESSION inside a function even if the variable is not passed in.
-
a game masterserver in php that is having issues
davidannis replied to Lethedethius's topic in PHP Coding Help
The line $result = sqlCall($con, "SELECT * FROM Servers"); is running the query that the fetch_array tries to get the data from. If the query succeeds, the variable $results contains an object. If not, it contains a boolean false. So the query is failing. You need to see what error mysql is returning and fix that. -
Why does the second query contain Events.Faith_ID but the first does not (the Join is different too)?
-
The display block in the error condition does not have a <tr> <td> tags and is displayed i the middle of the table. Perhaps the browser doesn't know how to display it. View source and see if it is there.
-
Lazro. I'm not a MySQL expert so I don't understand how you know his database structure. All you have is the field names from his query. What am I missing that allows you to duplicate the database?
-
I would start by echoing $verify_Event_sql and running it against the database perhaps in phpMyAdmin to see what results you get. Then do the same with $get_Event_sql. Perhaps the second query is not producing results because it looks like the first query counts all events in the current month (Day is not considered) but WHERE DAY(Events.Event_Date)= Day(Now()) And MONTH(Events.Event_Date)=Month(Now()) And YEAR(Events.Event_Date)=YEAR(Now()) looks at only events happening today.
-
If you don't want a single person viewing a profile to be counted twice you can either store each view in a database (works with logged in users across browsers and machines) or store who's been looked at in a cookie (works without requiring a login but only within individual browsers so if the user looks at the office and home he is counted twice) or you can use both mechanisms if you have both logged in and non-logged in users.
-
Going to sit on a beach for a few weeks with a keyboard and tablet and really learn jQuery. Be back in a month or so.
-
Capitalization
-
Does the file name end in .php? If not perhaps godaddy is displaying it as html.
-
It should be possible. Use a loop. Pseudocode: $perm_conn=mysqli_connect(...combined_data); truncate combined data // make sure you start with an empty table $databases=Array('db0', 'db1); foreach ($databases as dbname){ connection=mysqli_connect....$dbname); $select=//your select goes here $result=mysqli_query($select); while $row=mysqli_fetch_assoc($result){ //clean up here and/or at end //write $row to perm_conn here; } close connection to $dbname and free $results here (don't want 100 open connections) } //clean up, dedupe and send here Actually I like PravinS approach better, which you can also do in a loop.
-
capture image of server side output (not displayed to user)
davidannis replied to Q695's topic in PHP Coding Help
I think that you want to capture the content of the output buffer http://php.net/manual/en/function.ob-get-contents.php -
PHP Drop Down menu will not show array value?
davidannis replied to Dicko_md's topic in PHP Coding Help
try putting the variable in curly braces {$array[i]} from the php echo page examples: -
PHP CODE NOT UPDATING MYSQL DATABASE TABLE
davidannis replied to rleahong's topic in PHP Coding Help
Oops, my bad. I almost exclusively use mysqli. Should have advised the OP to switch to mysqli since mysql is deprecated. -
PHP CODE NOT UPDATING MYSQL DATABASE TABLE
davidannis replied to rleahong's topic in PHP Coding Help
On the last line the variable should be $results and the connection variable should be before the query variable. You should also sanitize the data before the query. -
Email upload confirmation code help
davidannis replied to danielwilliamsongle's topic in PHP Coding Help
the place you put it in post 6 seems seems fine to me. Also, when you post code please use the code tags (the "<>" symbol in the editor). It will make your code easier to read. -
Looks like your host does not let you include files that are in certain directories (probably those above /home/a1632545/public_html) as a security precaution. That way if I can get a webpage on your server I can't do things like include '/etc/passwd' ; If you move the files you want to include to a directory like /home/a1632545/public_html/includes it should fix the problem.
-
You can make a program that serves the mp3 file only if the link has not already been used. Here's how I'd do it: When a link is created for a user create a random number and store it in a database along with the link id (autoincrement) and the name of the file to serve. Then output a link that looks like this http://mydomain.com/servefile.php?id=12345&key=734871637846 when servefile.php runs it will read the database $id=mysqli_realescape_string($dbconn, $_GET['id']) ; $query="SELECT * FROM tablename WHERE id=$id"; $result=mysqli_query($query); $row=mysqli_fetch_assoc($result); if ($row['key']==$_GET['key'] && $row['used']!=true ){ //serve file here and mark link as used in database }else{ // print an error message here; }