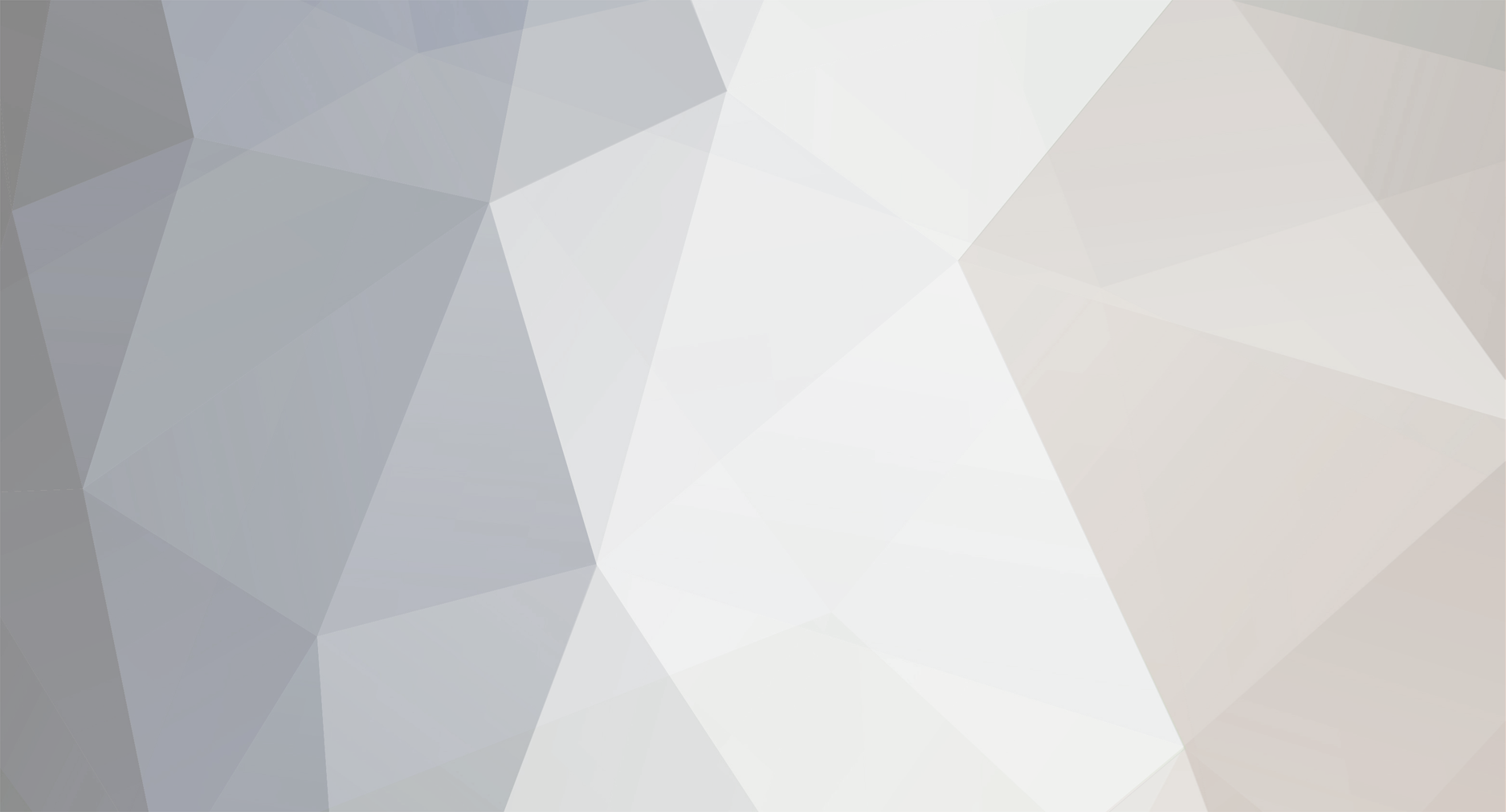
davidannis
Members-
Posts
627 -
Joined
-
Last visited
-
Days Won
2
Everything posted by davidannis
-
I see three big issues with the code at a glance: First, you use $_POST whether the value is set with the POST or GET method. For example: if(isset($_POST['sex'])) { $sex = $_POST['sex']; }elseif(isset($_GET['sex'])) { $sex = $_POST['sex']; } Then you don't sanitize the input, leaving yourself open to an SQL injection attack. You should do something like: if(isset($_POST['sex'])) { $sex = mysqli_real_escape_string($link,$_POST['sex']); Finally, you are using || in your queries where you should use &&. If I am looking for a girl in District 5 both being a girl and being in District 5 must be true (using an or gives me all girls and all people in district 5). You also need to omit from the query any fields on which the user is not searching so if district was left blank you don't only get records with a blank district in the result set.
-
Alternatively, if you want to stick with date_sub in my original code, this appears to work: $date = date('Y-m-d'); $date1 = date_create($date); date_sub($date1, date_interval_create_from_date_string('14 days')); $dateTwoWeeksAgo = date_format($date1, 'Y-m-d'); echo ' method 2: '.$dateTwoWeeksAgo.'<br>'; There is also a way to do the date calculation directly in the MySQL Query. I'm guessing somebody else could supply that SQL statement easier/better than I can (Barand is a master).
-
Sorry, mangled the code for subtracting 14 days. I tried this and it seems to work: $dateTwoWeeksAgo = date('Y-m-d',strtotime('-2 weeks')); echo ' Give us a date of: '.$dateTwoWeeksAgo.'<br>';
-
I'll give it a try, fix, and repost.
-
Try something like this: //open db here $date = date('Y-m-d'); date_sub($date, date_interval_create_from_date_string('14 days')); $query="SELECT * FROM mytable WHERE sent=0 and date<='$date'"; $result = mysqli_query($link, $query); while ($row=mysqli_fetch_assoc($result)){ //send email }
-
A whitelist of IP addresses would be reasonable, but I'd time them out so an IP that got on the list was not there forever. You could require a cookie on the browser that was tied to an IP address storeed on the server. If the user logs in with the same browser and is at the same IP he gets in with just a password, otherwise he gets challenge number 2. Folks with a DHCP server may end up being challenged every time even if they are sitting in the same place though usually your server will see their firewall/router's IP. A personal rant about secure passwords. I understand the desire to have a long, hard to crack, changing password from the perspective of a system administrator but I am a user too. The tougher the password requirements, the more chance that I'll forget it. After all how many 12 character passwords that require 2 cases, 2 numbers and a special character can I remember, especially if it changes every few months. So, the more secure the password, the larger the chance that I leave it on a post it or use the "I forgot my password, please reset it mechanism" The more frequently the reset password mechanism gets used, the more pressure to make it easy and quick for the user (less secure). So, there is a point at which requiring more secure passwords may create less secure results.
-
If security is a big concern, another idea is authentication above and beyond the password. Send an SMS or e-mail with a one time code valid for an hour as part of the login process or challenge the user with security questions (mother's maiden name, home phone, account number, etc.). You can choose to use these additional challenges only on new browsers (allow them to set a cookie if they expect to use the computer again).
-
It looks good to me at a glance. They salt and hash the passwords, guard against brute force attacks, etc.
-
Turned out the issue was not a configuration issue. I noticed that phpMyAdmin worked if I used a different browser, so I cleared cookies from localhost (which was not enough) then cleared my history (which worked).
-
I am running Zend Server's MAMP on a Mac. Life was good until I noticed an error on logging in to phpMyAdmin: Being compulsive (or crazy), I decided to fix the error that actually had caused no problems for months. I edited /usr/local/zend/share/phpmyadmin/config.inc.php and added something between the quotes to the line: $cfg['blowfish_secret'] = ''; After I did so, I could no longer log in to phpMyAdmin, any attempts just brought me back to the login page. So, I removed the secret phrase I had added. Now, when I try to log in I get: I see nothing unusual in /usr/local/zend/var/log/error.log or php.log (just a few errors from the last application I tested). I have tried restarting the browser, restarting mysql, and restarting the system. Any help greatly appreciated. Next time I'll leave well enough alone.
-
Not get session in my chat apps
davidannis replied to IlaminiAyebatonyeDagogo's topic in PHP Coding Help
Not in the code you posted. <?php $ses = null; You need to to have the session_start() at the top of every page that uses the $_SESSION data. -
(isset($_POST["firttName"]) has a typo tt instead of st
-
We need more information (field names) to really help but one strategy is to select from journeys and just show those SELECT * From Journeys ORDER BY Pickup and have a single dropdown with available journeys. Another strategy would be to have them select pickup first and then display the SELECT list of destinations that they can go to from that pickup SELECT Destination FROM Jouneys WHERE pickupfieldname='$pickupselectedbyuser' You can create the destination select list on the fly using AJAX.
-
The 303 response code is a very good idea, but don't know how to do that in php. Looks like response code is just added to the header() but I'm not sure of the syntax. True, but the OP wasn't trying to build a user click tracking system. 1. User data can be validated 2. (a) in the example I provided it is just either true or something else so that is not an issue (b) the user can go anyplace by typing in a URL. Unless I am missing something there is nothing magical in going to a URL via a redirect that creates a vulnerability that wouldn't be there if you went there directly. That was my original thought too (#2) but SocialCloud pointed out that not all browsers send the http_referrer (#3). The hidden field was a way to make sure it worked in those cases.
-
Good idea but I'd tweak it a bit. The issue I would have with that is you would need to clear $_SESSION['form_registration'] on every other page on the site or you would be redirected back to the registration page, even if you logged in 10 pages after visiting the registration page. What if instead you added a hidden field on the registration page and checked for that in login.php. <input type="hidden" name="fromreg" value="true"> if (isset($_POST['fromreg']) && $_POST['fromreg']=='true'){ header ('Location: regpageuri'); }
-
something like this in the login script (after you make sure they are logged in): if ($_SERVER['HTTP_REFERER']=='url of your registration page here'){ header ('Location: url of your registration page here'); } Note: header must be used before any output to the browser (or you can buffer the output).
-
Registration and Upload not working
davidannis replied to IlaminiAyebatonyeDagogo's topic in PHP Coding Help
sorry, sleep deprived and cranky. I actually use partial URIs in my own code, it is only a missing space after the colon that I have ever seen cause a problem. -
Registration and Upload not working
davidannis replied to IlaminiAyebatonyeDagogo's topic in PHP Coding Help
I have seen a malformed header cause issues. Most browser will have no problem but who wants to track down a bug that affects one user in a thousand? quote stolen from comment on http://php.net/manual/en/function.header.php -
Registration and Upload not working
davidannis replied to IlaminiAyebatonyeDagogo's topic in PHP Coding Help
Please put these two lines at the top of your program ini_set("display_errors", "1"); error_reporting(-1); and tell us what error messages you get. Another issue I noticed that would keep it from working is that when you use header() you MUST have a capital L in location and a space after the : -
Registration and Upload not working
davidannis replied to IlaminiAyebatonyeDagogo's topic in PHP Coding Help
YOu are missing a period '"$new_ should be: '".$new_ -
Thank you Ch0cu3r. I will replace the MDB2 code with standard mysqli. I am much more comfortable with procedural style rather than oo. I have a concern that I will cause problems if I do the database operations in procedural style while the rest of the code is oo but can't think of any particular issue. Is there something I'm missing? requinix: I started to try t figure out how to fix the static methods (other than to change the error reporting level) but it looks like I can't based ob the answer here: http://stackoverflow.com/questions/19248503/non-static-method-peariserror-should-not-be-called-statically FWIW, I think that the problem extends beyond the warnings. It is the last line MDB2 Error: not found that makes me believe MDB2 failed to connect to the database. When I installed MDB2 I failed to install the mysqli driver, which reading the documentation I see I also needed to do. http://pear.php.net/manual/en/package.database.mdb2.intro.php However, trying to install the driver fails % sudo ./bin/PEAR install MDB2#mysqli WARNING: "pear/Console_Getopt" is deprecated in favor of "pear/Console_GetoptPlus" Skipping package "pear/MDB2", already installed as version 2.4.1 downloading PEAR-1.9.4.tgz ... Starting to download PEAR-1.9.4.tgz (296,332 bytes) .............................................................done: 296,332 bytes Warning: mkdir(): File exists in System.php on line 285 Warning: mkdir(): Not a directory in System.php on line 285 ERROR: failed to mkdir /usr/local/zend/bin/PEAR/ChannelFile and so I'm throwing up my hands and going to put up the time in to completely rip out MDB2 since it is not being maintained.
-
Sorry I mangled the example. It works but proves nothing. Try this: <?php $a1='this is in myvar'; $myvar='$'.'a'.'1'; eval("\$myvar = \"$myvar\";"); echo $myvar; ?> though ignace has an easier way.
-
<?php $a1='this is a1'; $myvar='$'.'a'.'1'; eval("\$a1 = \"$a1\";"); echo $a1; ?> This works as an example.