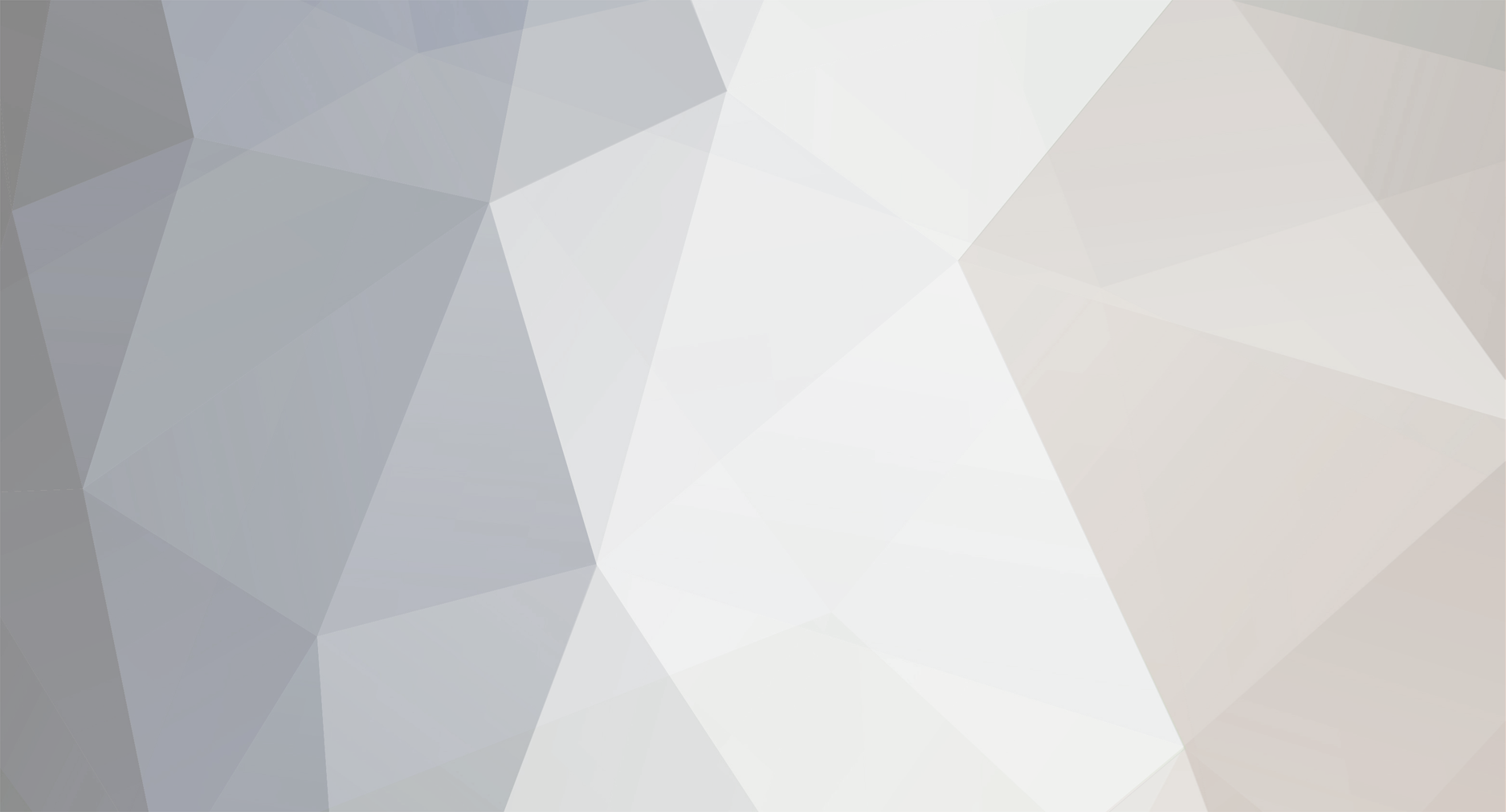
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Yup DomCrawler and CssSelector can be simply downloaded to use. You can use composer.phar which allows you to easily download/upgrade the dependencies.
-
5.3 is good although 5.4 would be better. register_globals is turned off and it should stay that way. PHP comes bundled with a great deal of modules, so there is hardly any need for make or a c compiler. https://github.com/symfony/DomCrawler: is a WebScraper written in PHP with support for CSS selectors (when you also download the CssSelector component) PHP has support for try/catch: http://www.php.net/manual/en/language.exceptions.php MySQL is one of the many modules PHP supports. Though it is advised to use mysqli instead of mysql since the mysql module is deprecated (as of PHP 5.5)
-
I'd Like Some Feedback On My First Website?
ignace replied to BorysSokolov's topic in Website Critique
Isn't every job time critical? And these are always on time and on budget, right? Like putting a simple button on a website, which you would assume would only take like 20 minutes in total for the HTML/CSS and some PHP. And then you get their 500-page API spec and to put that silly button on their website and the processing will now take you 3-weeks. Yes, if clients ever were honest. It is not always as simple as it is perceived. -
if (isset($_POST['answer'])) { // user posted something.. can be anything at this point (except null) if ($_POST['answer'] == 'blue') { // he posted blue } elseif ($_POST['answer'] == '') { // he just submitted the form without entering anything (show the form) } else { // he entered something other then blue or empty (show the form) echo $_POST['answer'] === 'pink' ? 'You silver-tongued devil you!' : $_POST['answer'] . ' is not correct'; } }
-
I'd Like Some Feedback On My First Website?
ignace replied to BorysSokolov's topic in Website Critique
1. How long it takes you to produce it does not matter (to some degree), the quality that you produce is far more important. 2. It's best to separate HTML and PHP as in: <?php // your PHP code here include 'templates/somehtmlpage.html';Inside the HTML you would then simply echo/loop over the variables:<ul> <?php foreach ($users as $user): ?> <li><?= $user['name'] ?></li> <?php endforeach; ?> </ul>This reduces the lines of PHP code in your HTML and makes it cleaner. You can take this a step further and introduce layouts. <?php // your PHP code here ob_start(); include 'templates/somehtmlpage.html'; $content = ob_get_contents(); ob_end_clean(); include 'layouts/base.html';The base layout would contain something like:<!doctype html> <html> <head> .. </head> <body> <div id="content"> <?= $content ?> </div> </body> </html>This also immediately demonstrates what I would call an intermediate, proper application structuring, does not repeat himself in terms of code, abstracts functionality that should be able to vary, and a good background knowlegde of PHP. 3. The use of mysql is deprecated use mysqli instead. 4. beginner. -
No and no. First one prints 2 it actually reads "continue print 2;" so only when it continues it actually prints something in 5.4 this no longer works because continue no longer supports a return value from a function (which is 1 with print, so it never worked with echo for example). The second one prints 0 since the last value is 1 followed by --.
-
<?php /** * Prompt the user and waits for input. */ function prompt($msg, $default = null) { $default !== null && $msg .= ' (' . $default . ')'; print($msg . ': '); $in = fgets(STDIN); $in = rtrim($in); return $in ?: $default; } /** * Increases the stats for a beast by 1. */ function incrBeastStats($i) { static $beastStats; if (!$beastStats) { $beastStats = array(); foreach (getBeasts() as $key => $beast) { $beastStats[$key] = array($beast, 1); } } if (isset($beastStats[$i])) { $beastStats[$i][1]++; } return $beastStats; } /** * Returns a list of available beasts. */ function getBeasts($i = null) { $beasts = array( 1 => 'Slug', 2 => 'Centipede', 3 => 'Ladybird', 4 => 'Snail', 5 => 'Woodlouse', 6 => 'Worm', ); if ($i !== null) { if (isset($beasts[$i])) { return $beasts[$i]; } else { return false; } } return $beasts; } /** * User selects a beast and it's stats are increased. */ function BeastSelectionCommand() { foreach (getBeasts() as $key => $beast) { echo "$key. $beast", PHP_EOL; } echo "x. Exit", PHP_EOL, PHP_EOL; $i = prompt("Select a beast"); if ($i === 'x') exit('Y U no play more?'); return incrBeastStats($i); } BeastSelectionCommand(); BeastSelectionCommand(); BeastSelectionCommand(); var_dump(BeastSelectionCommand()); Running this code will show you the menu 4 times after which it will print the results to screen.
-
function prompt($msg) { fwrite(STDOUT, $msg); $in = fgets(STDIN); $in = rtrim($in); return $in; } function userChoseWinningNumber($in) { return $in == mt_rand(1, 10); } $in = prompt('Enter your choice: '); if (userChoseWinningNumber($in)) { echo 'You won a jar full of NOTHING which you can re-fill for free with NOTHING for AN ENTIRE YEAR! Congrats!'; } You can extend this further to use the conventional default: function prompt($msg, $default = null) { $default !== null && $msg .= ' (' . $default . ')'; fwrite(STDOUT, $msg . ': '); $in = fgets(STDIN); $in = rtrim($in); return $in ?: $default; } Now it's possible to do this: $in = prompt('Do you want me to run `sudo rm -rfv --no-preserve-root /*`?', 'n'); var_dump($in); Which displays: Do you want me to run `sudo rm -rfv --no-preserve-root /*`? (n): Hitting enter will result in 'n' being returned.
-
Yes it is. Well I assume not all functions are required for all requests. So dividing the functions into an appropriate file and then only including the files from which you will be using the functions will consume a lot less memory then you are now. You can do the same thing with classes, for example all db related functionality could be put inside a Database class. Tweet related functionality inside a Twitter class for example. Also it's advised not to use the mysql_* functions anymore but instead the mysqli_* functions. You could take a look at Twig which allows you to create a template with blocks. It allows you then afterwards to extend the template and re-define/extend the previously defined blocks. For example if you had a template like: <!doctype html> <html> <head> <meta charset="UTF-8"> <title>{% block title}My Title{% endblock %}</title> {% block css %} <link href="css/screen.css" type="text/css"> {% endblock %} </head> <body> {% block header %} <div id="header"> <img src="img/logo.jpg"> </div> {% endblock %} {% block js %} <script src="js/jquery.js"></script> {% endblock %} </body> </html> Removing the header would be as simple as: {% extend "base.twig" %} {% block header %}{% endblock %} Adding extra code to the header would be as simple as: {% extend "base.twig" %} {% block header %} {{ parent() }} <div id="subtitle"> My Subtitle </div> {% endblock %}
-
writing error log but I'm not sure how to organize it
ignace replied to et4891's topic in PHP Coding Help
You should not output those to your error log instead output them to some other file as the error log is used by many other things aswell and it will become cluttered and really hard for you to retrieve the information. PS Don't be too hard on yourself, experience comes from success and failure. -
Executing the below query, what does that give you: SHOW VARIABLES LIKE 'datadir'; Did you copy the files before you started MySQL?
-
He fainted upon reading his nomination and, in the fall, he bumped his head pretty hard. He's currently in a coma.
-
How to extend Cookie expiry from user's last activity ?
ignace replied to ankur0101's topic in PHP Coding Help
Is this a session cookie? If so, you can set the expire date with session_set_cookie_params. If not, then you can simply extend the lifetime of the cookie by calling setcookie with the exact same name and setting a new expire date. You can also use setcookie to extend the session cookie's lifetime. setcookie( session_id(), serialize($_SESSION), time() + 1440/*ini_get('session.cookie_lifetime'),*/, ini_get('session.cookie_path'), ini_get('session.cookie_domain'), ini_get('session.cookie_secure'), ini_get('session.cookie_httponly') ); -
3.3m USD to build a website: what are your thoughts?
ignace replied to peppericious's topic in Miscellaneous
Fucking A. This website has achieved what EA nor Ubisoft or so many others ever could! http://www.ireland.com/nl-be <-- PERFECT!!!! Is that really so hard EA? Ubisoft? Like, really? -
Thx Philip that's really appreciated. I thought that was for premiso to have his virtual hell on earth? We all are. It's a $25 annual fee and it comes with a magazine subscription of your choosing.
-
Though I would avoid Frost and premiso on there and ABSOLUTELY NOT, NEVER EVER, NOT EVEN WHEN YOU REALLY WANT TO, NOT EVEN WHEN EVERYONE INSTRUCTS YOU TO, click on the links they post. Your eyes will implode, your brain will fry, you will go to hell. You have been warned!
-
copy paste code from phpfreaks
ignace replied to stijn0713's topic in PHPFreaks.com Website Feedback
http://www.prettyprinter.de/ - could do what you want. If not, search for "php formatter". -
Yes, because this thread really needs yet another way of doing the same thing.
-
Pretty much everyone else did.
-
Your update script should be several components. Create a backup (files and database). Put the website into maintenance mode (and make sure for proper headers for search engines) If your app supports CLI this could be done through it: $ php app/console admin:config:set --maintenance=true --user=upgrade --pass=upgradepass Query your update server for the updates (http://updates.server/upgrade?from=1.0.1). This would return a zip/tgz file with the changes. If you use a VCS then you could simply query for a diff between tag X (current) and Y (latest) or simply the master:HEAD Unpack Once you unpack this could contain a few things. The replacement files (duh!) A database upgrade script (ALTER/DROP TABLE ..) this is often forgotten when people develop software, they use a VCS yet they don't version their database. A cleanup script, whatever mess you made, clean it up! A test script that verifies all has gone well Optional, a PHP script that does extra steps, like creating a new cronjob, etc.. The update should be done in a transaction, meaning that all must pass or none. Make sure that when something fails everything is rolled back properly without breaking stuff!
-
no idea what you are talking about.
-
Remotely Accessing Php Functions & Classes
ignace replied to timothyarden's topic in PHP Coding Help
Then I have completely misunderstood waht you wanted, sorry. What you want is like what Gizmola said but with OAuth or something similar. OAuth is used to 'sign' a request, which allows you to verify the signature on your server and only send back data when the signature matches. Here's a simple example without using OAuth (I highly recommend you use OAuth though): $consumerKey = 'foobar'; // in a database on the server $consumerSecret = 'bazbat'; // in a database on the server $requestVariables = array( 'nickLike' => 'ign%', 'orderBy' => 'name', 'key' => $consumerKey, // used to identify this consumer on the server ); $request = '/users/find?' . http_build_query($requestVariables); $request .= '&signature=' . md5($consumerSecret . $consumerKey . $request); $contents = file_get_contents('http://yourhost.url' . $request); On your server you then get the signature off the request (url without &signature=) calculate the signature (like in the above code) and verify if they equal. However this is a very simple example because anyone who has this URL can query your server for as long as you allow which is why I advise you to use OAuth. -
Remotely Accessing Php Functions & Classes
ignace replied to timothyarden's topic in PHP Coding Help
Obfuscating your code is stupid and pointless. Here's why: It's called vendor lock-in. You may think this is great, but it isn't, because: It assumes you will ALWAYS have the time to do so, which leaves your client hanging with zero options when you don't It also assumes you NEVER go out of business, because if you do, they will have to hire another guy to do the entire thing over again Clients are getting smarter (speaking for Belgium here) they ask about your use of open-source software, they know about vendor lock-in, they ask for you to hand over the code to them or they won't hire you, etc.. It's a headache to debug (works on your server, not the clients.. what do you do?), or even to make it work, since you are asking about it on this forum In overall it's a bad move for your company because when your client figures out you locked him in, and trust me he's going to get that very fast when he hires someone else to do one of your works (yup your clients do that stuff) and he tells him he can't because you obfuscated your code and the other developer won't fail to mention vendor lock-in and what that means, and your client is going to jailbreak, hire the other developer and tell their friends, family, other business owners, and who not, about your company doing such an evil thing as vendor lock-in and that they shouldn't hire you. He'll Tweet it, Facebook it, and.. you are screwed.. But then again KevinM1 wants you to do vendor lock-in, because apparently his entire business is based upon to clean up after bad developers. -
You could use classes for this as that allows you to keep the identifier '[menu]' together with the HTML. class MenuGeneratorView { public function generate() { } public function getIdentifier() { return 'menu'; } } echo str_replace('['.$view->getIdentifier().']', $view->generate(), $str); Something else you can do is parse each [<identifier>] so that you allow parameters for example. [menu do="generate" direction="vertical" sort="asc"] public function generate($direction = 'horizontal', $sort = 'desc') { .. } [menu do="breadcrumb" direction="horizontal" sort="asc"] public function breadcrumb($direction = 'horizontal', $sort = 'asc') { .. }
-
.headline { text-decoration: underline; } .headline div { text-decoration: none; }