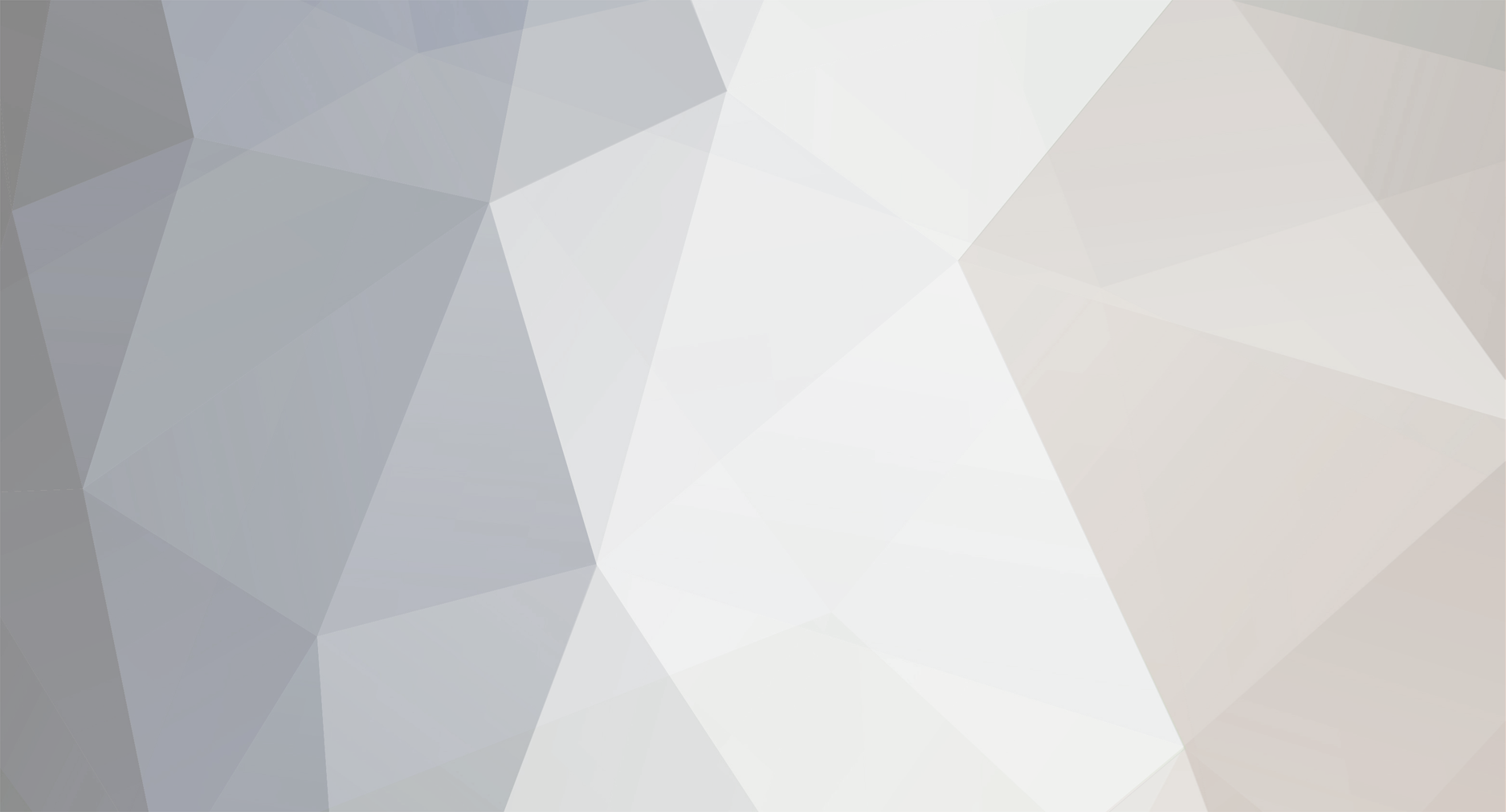
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Having more efficient/maintanable/readable code to return errors
ignace replied to zend_play's topic in PHP Coding Help
It's a standard try/catch: try { $this->activateFunction(); $this->activatePlan(); .. } catch (ComponentActivationFailed $e) { return $e->getMessage(); } class ComponentActivationFailed extends Exception {}If you need to specifically know which component failed: try { $this->activateFunction(); $this->activatePlan(); .. } catch (FunctionActivationFailed $e) { .. } catch (PlanActivationFailed $e) { .. } catch (ComponentActivationFailed $e) { // catch-all } class FunctionActivationFailed extends ComponentActivationFailed {} class PlanActivationFailed extends ComponentActivationFailed {} -
Most browsers give you the option to replay Ajax requests. There are even browser extensions that allow you to create ajax calls with for example POST and JSON as body. @faces3 if you have Chrome installed (if not, install it) go to your page where you would call the ajax. Options (the three horizontal lines) > Extra > Developer Tools. This opens a new pane at the bottom. Click Network and click on XHR at the bottom. Now fire your ajax and you'll see that a new line appears. Click it and select Response, fix your code, come back and right-click on the line and select Replay XHR, another line appears, click it and select Response. Keep repeating this process until you have the output you expect.
-
Clearly someone needs to explain to you the principles of OO programming. Ever heard of a concept called reusability? The whole point of not changing your class from MySQL to some other vendor but instead use polymorphism for that is that you could break existing functionality. What would you do for example if some part of your app. uses MySQL (front-end for example) and the other uses MSSQL due to some legacy system that is build upon MSSQL? I highly suggest you take a look at Zend\Db which allows you to have a Slave/Master setup like what you are trying to do with the ADMIN, READWRITE, READONLY thingy.
-
Download/Install Zend\Form Create an abstract form type to represent a quiz qa (ie setQuestion, setAnswers/setOptions, getUserSelectedAnswers) extending Zend\Form Create a form for each of the different quiz question categories, add additional methods where required (but favor configuration) Make sure each of the forms render correctly Create a factory to build the different form types (used to build the form objects from text in your database)
-
Writing your own database class is nice as an experiment. When you are building software for a client however it's better to use battle-tested code as to avoid bugs and security issues. In general I try to find open-source tested code for any boilerplate tasks in a project so that I can focus on the hard, more interesting stuff of the application and not bother myself with how I would want to write my database class or my authentication handler.
-
It's better to use the constant instead of 4 since if they ever change it you need to update all your code: if ($_FILES['upload']['error'] === UPLOAD_ERR_NO_FILE)
-
Since you speak german (well a dialect of sorts) and also understand english (since your pc is set to it) I don't think it will be too hard to translate between those two languages. Otherwise if you happen to have Win7 Ultimate edition you can just select a different system language (or using the Anytime Upgrade you can upgrade to ultimate).
-
I mentioned MySQL to infer this was more work to setup which is why I suppose everyone opted for XML, YAML, or INI. And I simply wanted to remind everyone there is also SQLite (IMO a far better choice then XML, YAML, or INI).
-
YAML, XML, INI. Did everybody forget there are other DB vendors then MySQL? Like SQLite?! A lot easier to switch later on to for example MySQL too. Also if for some reason the application would become more popular supports indexing and other things we have come to expect of a database.
-
No, my files love git so much they add themselves
-
I see now you used SVN which is similar to Git which would have been fine too. If you want to stick with Git, you can import or track your svn repo using http://git-scm.com/docs/git-svn so you don't lose the history of your svn repo. It is anyhow advisable to remove these directories/files from your Git repo: $ git rm --cached .svn/* .DS_* $ git add -u $ git commit -m "Removed svn and macosx files" $ git pushThe --cached option leaves the actual files on your hard-drive but removes them from Git. Then create a .gitignore file and add these lines: .svn/* .DS_Store .DS_Store?
-
Simpler then git? Is that possible?! 1. git init 2. make some code changes 3. git commit -m "My awesome commit message" 4. make more code changes 5. git commit -m "More Changes!!" 6. git branch myawesomebranchedfeature 7. make even more code changes 8. git commit -m "OH MY GOD !! THIS IS AWESOME !!" 9. git checkout master 10. git merge myawesomebranchedfeature 11. git branch -d myawesomebranchedfeature
-
Sharing source files over dropbox accounts will get REALLY messy. And everyone would have to constantly make backups of their source code and then download the new source, do a diff between directories and transfer their code changes back in, and then somehow make sure everybody else get their changes... By using Git you avoid all of these problems and you can collaborate easily with others, even allowing some to branch off your main development line (for example because you have 2 ideas for the battling system, using branches allows this parallel development to take place).
-
krisnarocks if you want people to help you on your project it would be best to put it in a versioning system like git and create a repo on github for it. 1. Install Git 2. Create an account on GitHub 3. Create a public repository (free) 4. Open the git gui which is installed alongside git and clone your new github repository 5. Learn git, make some changes, commit those changes and don't forget to push them to github. This way others can join you in your effort, make changes and build your rpg.
-
$password = "blahblahblah" (this value will pull from a form though) $db_password = md5($password);Correct.
-
The reason that SQL returns everything as a string except NULL which since a certain PHP version, I think 5.3, actually returns NULL, is because that it simply can't translate the types correctly. It can't translate a TINYINT, a SMALLINT, a MEDIUMINT, a BIGINT, a DECIMAL, a DATETIME, a YEAR, a TIME, a VARBINARY, a DOUBLE, an ENUM, a SET, ... And these are just the ones that I can think of out of the top of my hat. Not to mention any custom data types in MySQL. So thus since it can only translate about 2 or 3 types correctly it simply doesn't bother and it's expected of the user to do this conversion themselves if desired. Now you may wonder why I included TINYINT, SMALLINT, MEDIUMINT, ENUM, YEAR, etc. This is because you probably don't understand type translation between languages. When the column is a tinyint then in PHP it should be the equivalent not a 32-bit signed integer or you will get problems when you want to update the row (where this translation would also exist, and you can't fit a 32-bit signed integer into a tinyint). The same goes for example a SET, which would be an array where you can only add certain values. SQL: SET('foo', 'bar', 'bat', 'baz') PHP: $mySet[] = 'world'; // error: world is not in set('foo', 'bar', 'bat', 'baz')
-
Username change/login issues.
ignace replied to Dragosvr92's topic in PHPFreaks.com Website Feedback
Racists, free the cockies now!! -
Storing Calendar Events / One-time vs recurring
ignace replied to Jessica's topic in Application Design
Here is a crazy idea, how about using the same working as a cronjob? The problem is that a DATETIME type has no option to blank out certain value (like month, day, or year) which is what you actually need. And using words like 'recurs' or something like it only makes it harder to query. The database schema would be something like: events (id, date_start, date_end, ..) events_dates (event_id, date_id) dates (id, minute, hour, day, weekday, month, year)Assuming NULL means * you can construct your queries to find the events that occur today, this week, this month, this year. Things like */3 would have to be resolved to multiple dates. Though I guess https://github.com/mtdowling/cron-expression could help achieve you get those dates. The event would have another field that defines when the event ends. The date start will help you query for any relevant events, date_start is always set (creation date otherwise). When date end is NULL then it is ongoing. The dates table holds how many times it should occure. What do you think? -
exit; break;@barand You want to be really sure, he?
- 17 replies
-
- php forms
- radio button
-
(and 3 more)
Tagged with:
-
Something like this in jQuery (assumes all your internal URL's are relative) $('[href^="http"]').click(function(e) { e.preventDefault(); window.location = 'http://yoururl.com/exit.php?url=' . encodeURIComponent(this.href); });
-
you mean like this? <?php $file = "rbc/" . basepath($_GET['parse']); if (!file_exists($file)) { printf("file %s does not exist", $file); exit; } foreach (json_decode(file_get_contents($file, true))->RubricCriterion as $rubric) { echo $rubric->name; }
-
Need help separating big project into smaller PHP projects
ignace replied to bcassol's topic in PHP Coding Help
git submodules. You can still use composer at the same time (if need be or afterwards). -
Hey, I need help with the following Classes syntax
ignace replied to elme's topic in PHP Coding Help
class GenderEnum { const MALE = 'M'; const FEMALE = 'F'; const ALIEN = 'A'; public static isValid($gender) { return in_array($gender, array(self::MALE, self::FEMALE, self::ALIEN)); } } class User { private $gender; public function setGender($gender) { if (!GenderEnum::isValid($gender)) { throw new UnexpectedValueException(); } $this->gender = $gender; } }Unless you have different behavior for your Male and Female classes these should be just one class. And by different behavior I mean: class MaleUser extends User { public function doThingA() { // do stuff } } class FemaleUser extends User { public function doThingA() { // do it completely different } } -
So at worst their software exists only out of 80% of crap code.
-
$soapClient = new SoapClient("http://www.fastcash4homes.com/WS/LeadSubmission.asmx?WSDL", array('trace' => true, 'exceptions' => true));When you pass trace=>true then extra features become available: SoapClient::__getLastRequest SoapClient::__getLastRequestHeaders SoapClient::__getLastResponse SoapClient::__getLastResponseHeaders