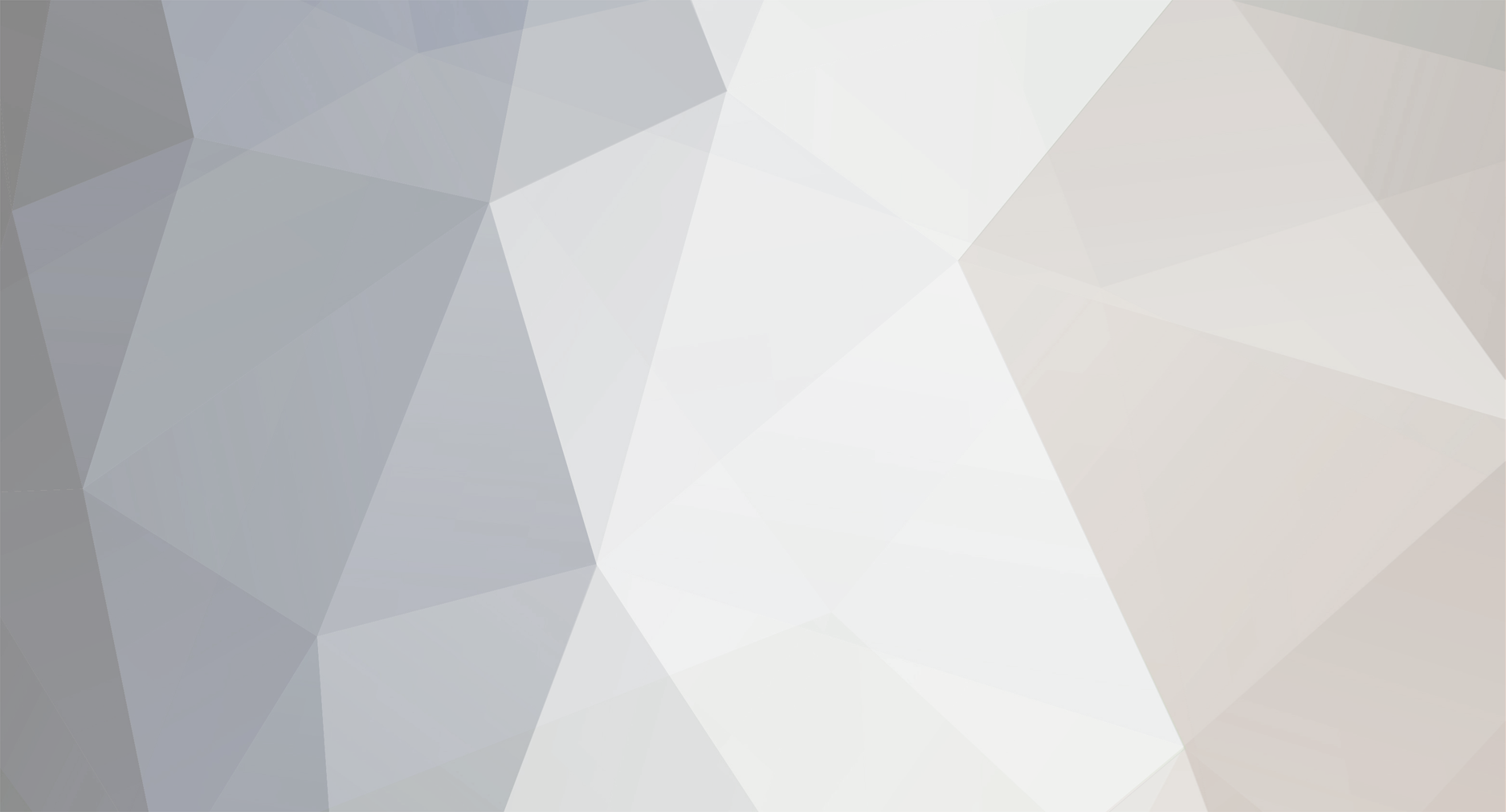
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Yes, it does. The global namespace is implied.
-
We don't know what each class does since the OP did not give any concrete code. So it's best to advice composition over inheritance IMO.
-
Some basic questions about PHP to talk about...Please.
ignace replied to makuc's topic in Miscellaneous
JS is a fun language to play around in. For example if you are running Chrome or IE8/9 press F12. A new dialog should appear on the bottom of your screen, press Console. At the bottom you'll find an input field. Type console.dir(new Date()); You'll find a __proto__ property in Chrome, expand it to find the available methods. Now type: console.dir(console); And admire Output may differ between vendors. Chrome for example shows memory and profiles properties along with the known __proto__ property. Whenever I wonder how something works or will it work? I use this method to find it out quickly while I'm coding. You can run PHP from the command line (on Windows [Windows Key]+R), so that looks like: php -r "var_dump([whatever neat stuff you want to check comes here]);" -
Unit-Testing is an automated process. Sure you could write code and just run it in a browser, see if any of it failed. And as your project grows and new functionality is implemented other parts might break without you knowing so to avoid it you will have to start making a checklist of your entire website, going over each one of them to make sure it still works. If one of them fails on your checklist, you alter your code to fix it, and you will have to start back from the top (since something else may have broke) going over each one of them until something else fails, again and again and .. Unit-Testing automates this process. It allows you to add functionality or refactor some code without having to worry something will break, because if it does you'll know it by the push of a button. That said, it means that your tests have to be thorough otherwise they are just false-positives. To ensure they are thorough you write positive and negative tests. Where positive tests check whether it works and negative tests check whether if it gets something unexpected it will fail gracefully.
-
jcombs you would help us to help you if you would post some more information than just those little pieces of information. Give us an ERD to look at and we can help you normalize it and/or provide advice/suggestions in how to improve the design.
-
Fetching Data from Database using PHP classes (OOP)
ignace replied to salmander's topic in Application Design
Take a look at the available PEAR packages. They already have classes that will create an HTML table from a database result. If you want to start from scratch, you can take a look at how they did it or try to combine some of the packages (like HTML_Table) with already existing built-in functionality like PDO. -
Why then not do something like: fields field_id field_name actions action_id action_name fields_actions field_id action_id
-
foreign key (field1) references actions(id) foreign key (field2) references actions(id) foreign key (field3) references actions(id) That is indeed correct. foreign key(field1, field2, field3) references actions(id, id2, id3) This would be used for compound keys.
-
@1 Add an alert to both statements, I'm 99.99% sure the a[rel=external] is run before the plugin that adds the rel="external" or add a .delay() to a[rel=external] @2 .attr('title', node.attr('title') + '') @3 There are plugins that already do this check the Plugin section on jQuery.com
-
UPDATE the_table SET A = if(A = 1, 1, B), B = if(A = 0, 1, A) WHERE ID = 1
-
iterating through array while passing an index
ignace replied to johnmerlino's topic in PHP Coding Help
$elements = new Enumerator(array('email','fax','phone','postal mail')); $elements->each(function($l, $i){ echo form_checkbox("checked[]",$l); if (!($i % 2)) echo "<br>"; }); have you tried this? Also take a look at the php manual page for array_walk as you'll see that it passes 2 parameters. -
The problem is that file() loads all the lines in memory probably exceeding the assigned limit. To avoid this you should read line by line: $fopen = fopen('list.txt', 'rb'); while ($fopen && ($line = fgets($fopen)) { // do something with $line } // all lines processed if ($fopen) fclose($fopen);
-
Although I would like to think/see it otherwise, I must agree.
-
I really like how the OP actually posted a fun exercise, we all posted our solution in either OO or procedural, and now we are all discussing each others solution. I think we should do this more, allow the entire community to participate and defend their design/decisions. With the greater goal of everyone learning new things from each others code/comments.
-
Can you already see the dollar bills in your childrens' eyes? :evil:
-
I typed it as I read the task description: class BattleRecorder { private static $instance; private function __construct() {} private function __clone() {} public static function get() { if (is_null(self::$instance)) { self::$instance = new self(); } return self::$instance; } public function record($msg) { echo $msg, PHP_EOL; } } abstract class Warrior { public $name; public $health; public $attack; public $defence; public $speed; public $evade; public $turns; public $recorder; public static function get($warrior, $name) { $className = ucfirst(strtolower($warrior)); return new $className($name); } public function __construct($name) { $this->name = $name; $this->_init(); } public function isDefeated() { return !$this->isAlive() || !$this->hasTurnsLeft(); } public function isAlive() { return $this->health > 0; } public function hasTurnsLeft() { return $this->turns > 0; } public function evadedAttack() { return $this->evade == mt_rand(1, 100) / 100; } public abstract function attack(Warrior $w); public abstract function defend($attack); protected abstract function _init(); } class Ninja extends Warrior { public function attack(Warrior $w) { --$this->turns; $damage = $this->attack * (0.05 == mt_rand(1,100) / 100 ? 2 : 1); $this->recorder->record($this->name . ' dealt ' . $damage . ' damage.'); $w->defend($damage); } public function defend($attack) { if ($this->evadedAttack()) { $this->recorder->record($this->name . ' evaded the attack.'); return; } $damage = $attack - $this->defence; if ($damage > 0) $this->health -= $damage; $this->recorder->record($this->name . ' defended, but lost ' . $damage . ' health.'); } protected function _init() { $this->health = mt_rand(40, 60); $this->attack = mt_rand(60, 70); $this->defence = mt_rand(20, 30); $this->speed = mt_rand(90, 100); $this->evade = mt_rand(30, 50) / 100; } } class Arena { public static function fight(Warrior $w1, Warrior $w2) { $w1->turns = 30; $w1->recorder = BattleRecorder::get(); $w2->turns = 30; $w2->recorder = BattleRecorder::get(); list($w1, $w2) = self::drawFirstBlood($w1, $w2); while (!$w1->isDefeated() && !$w2->isDefeated()) { $w1->attack($w2); $w2->attack($w1); } if ($w1->isAlive() && $w2->isAlive()) BattleRecorder::get()->record('draw!'); elseif ($w1->isAlive()) BattleRecorder::get()->record($w1->name . ' won!'); else BattleRecorder::get()->record($w2->name . ' won!'); } protected static function drawFirstBlood(Warrior $w1, Warrior $w2) { $r = array($w1, $w2); if ($w1->speed > $w2->speed) { $w1->attack($w2); $r = array($w2, $w1); } else if ($w1->speed == $w2->speed) { if ($w1->defence < $w2->defence) { $w1->attack($w2); $r = array($w2, $w1); } else { $w2->attack($w1); } } else { $w2->attack($w1); } return $r; } } Might not be a good example as for best practices Took me around 40 minutes I think. Call like: Arena::fight( Warrior::get('Ninja', 'Foo'), Warrior::get('Ninja', 'Bar') );
-
If the original is no longer maintained, you can still check some of the forks on github.
-
1. You should add stripslashes on these. 2. The menu on top doesn't highlight the current page you are on.
-
It's passed your due date but I found it interesting to try it out myself: class BattleRecorder { private static $instance; private function __construct() {} private function __clone() {} public static function get() { if (is_null(self::$instance)) { self::$instance = new self(); } return self::$instance; } public function record($msg) { echo $msg, PHP_EOL; } } abstract class Warrior { public $name; public $health; public $attack; public $defence; public $speed; public $evade; public $turns; public $recorder; public static function get($warrior, $name) { $className = ucfirst(strtolower($warrior)); return new $className($name); } public function __construct($name) { $this->name = $name; $this->_init(); } public function isDefeated() { return !$this->isAlive() || !$this->hasTurnsLeft(); } public function isAlive() { return $this->health > 0; } public function hasTurnsLeft() { return $this->turns > 0; } public function evadedAttack() { return $this->evade == mt_rand(1, 100) / 100; } public abstract function attack(Warrior $w); public abstract function defend($attack); protected abstract function _init(); } class Ninja extends Warrior { public function attack(Warrior $w) { --$this->turns; $damage = $this->attack * (0.05 == mt_rand(1,100) / 100 ? 2 : 1); $this->recorder->record($this->name . ' dealt ' . $damage . ' damage.'); $w->defend($damage); } public function defend($attack) { if ($this->evadedAttack()) { $this->recorder->record($this->name . ' evaded the attack.'); return; } $damage = $attack - $this->defence; if ($damage > 0) $this->health -= $damage; $this->recorder->record($this->name . ' defended, but lost ' . $damage . ' health.'); } protected function _init() { $this->health = mt_rand(40, 60); $this->attack = mt_rand(60, 70); $this->defence = mt_rand(20, 30); $this->speed = mt_rand(90, 100); $this->evade = mt_rand(30, 50) / 100; } } class Arena { public static function fight(Warrior $w1, Warrior $w2) { $w1->turns = 30; $w1->recorder = BattleRecorder::get(); $w2->turns = 30; $w2->recorder = BattleRecorder::get(); list($w1, $w2) = self::drawFirstBlood($w1, $w2); while (!$w1->isDefeated() && !$w2->isDefeated()) { $w1->attack($w2); $w2->attack($w1); } if ($w1->isAlive() && $w2->isAlive()) BattleRecorder::get()->record('draw!'); elseif ($w1->isAlive()) BattleRecorder::get()->record($w1->name . ' won!'); else BattleRecorder::get()->record($w2->name . ' won!'); } protected static function drawFirstBlood(Warrior $w1, Warrior $w2) { $r = array($w1, $w2); if ($w1->speed > $w2->speed) { $w1->attack($w2); $r = array($w2, $w1); } else if ($w1->speed == $w2->speed) { if ($w1->defence < $w2->defence) { $w1->attack($w2); $r = array($w2, $w1); } else { $w2->attack($w1); } } else { $w2->attack($w1); } return $r; } } Call/use like: Arena::fight( Warrior::get('Ninja', 'Foo'), Warrior::get('Ninja', 'Bar') ); Brawler and Samurai are not implemented so that's up to you. To get a specific type use Warrior::get() as demonstrated, you can pass this from the form to the function. Note that it has no error checking.
-
how many people try to hack a web app game?
ignace replied to JeremyCanada26's topic in Miscellaneous
I would. I already did a few times in the past, even cash-games, but I make it obvious it has been hacked. I would set my score to something like 99999999999999 which usually results in the highest possible number for a 32-bit signed integer. Usually the leak is patched 'quickly' thereafter otherwise I keep hacking it until they do The exploits I'm talking about are of real basic nature and should be easy for anyone with some background in programming to find and exploit/fix them. -
can anyone tell what font-family this text is in ?
ignace replied to jasonc's topic in Miscellaneous
http://www.tipsotricks.com/2009/08/find-fonts-name-from-image.html -
We will need CActiveRecord too.
-
PHP 6 years and I'm currently getting my feet wet with Python, Lisp, and Perl or PLP as I call it. I never deviate much from my roots
-
Like Adam already said ::model() returns a different object. This object in turn either has a property TypeOptions public $TypeOptions or a __set method.