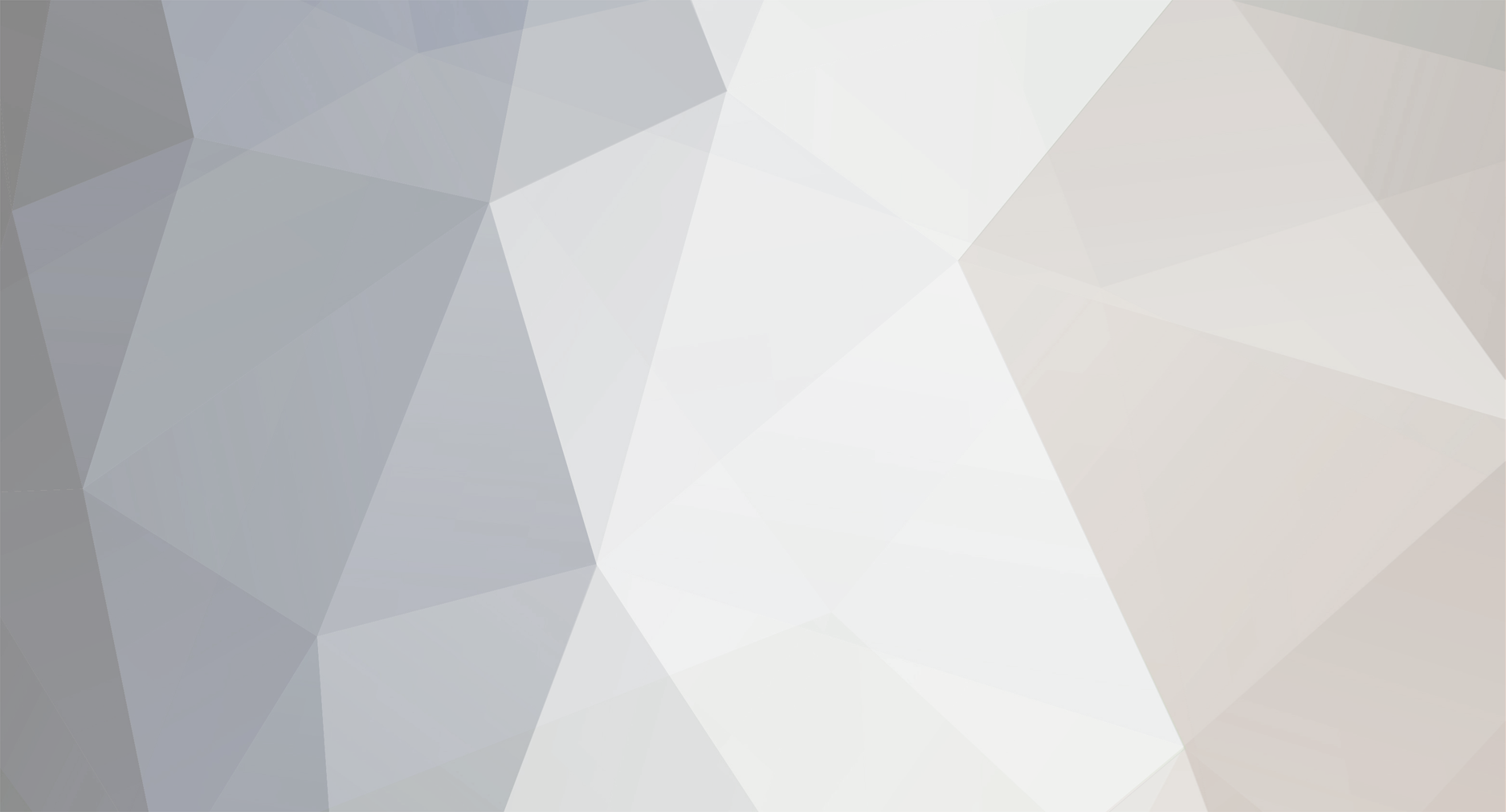
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
So the sql is correct, but the values are not in the database? There are still many possibilities. 1. The query fails 2. The query succeeds, but another query overwrites the values later 3. The query never runs at all 4. The query succeeds, but the code which reads the values is faulty, or is run at the wrong time. If the query never ran, then the print statement would never run. So it's very likely the query was run. If the query failed, you would get an error message, as you are checking for errors. That leaves 2 possibilities.. Next question: How do you check the values in the database? Can you post the code? Or are you using a direct interface like phpmyadmin?
-
The statement [code=php:0]UPDATE users SET users_pagesviewed = users_pagesviewed + 1 WHERE users_id = $users_id[/code] will work, assuming $users_id is set to the id of your user.
-
Instead of adding 86400, add 1 to the day value before passing to mktime(). mktime() will compensate for ends of months, ends of years and daylight savings, and generally do what you want it to do. Take a look at the comments in the online manual for more tips. http://sg.php.net/manual/en/function.date.php http://sg.php.net/manual/en/function.mktime.php
-
I suggest you remove the open_basedir restriction, and do your own checks to ensure that the files you include are "ok". Programming always involves a tradeoff between security and flexibility, and in this case you need a little more flexibility. "./" goes to the current directory, not the root directory. "/" is the root directory. You can find your current directory with getcwd(), if that helps.
-
Usually, session data is stored in files. It may be stored in /tmp , or in some other directory. There's more information at http://sg.php.net/manual/en/ref.session.php But I would recommend keeping your own records in a database if possible. A text file would also be good enough. Keep in mind that if someone just closes the browser without logging out, their session will stay forever unless you have a system in place for clearing out old sessions.
-
If that was my script, I would add [code=php:0]print "SQL: $sql<br>";[/code] right after you set $sql for the insert. If zeroes are going into the database, then it's probably because the sql statement is putting zeroes into it :) Then go back through your script printing out the values of $music and so on, until you find where they are going wrong.
-
You should use this syntax instead: [code=php:0]$dbconn = pg_connect("host=sheep port=5432 dbname=mary user=lamb password=foo");[/code] Note that it allows you to specify the user and password. There is no need to use "apache" or "SYSTEM" as the username. See http://sg.php.net/manual/en/function.pg-connect.php
-
That means it does not have permission to open jobs.xml (or possibly the directory containing it). How to fix it depends on if you are using unix or windows..
-
Is that your whole code right there? That won't do anything :) [code=php:0]$sql = "DROP TABLE users"; $result = mysql_query($sql) or die("Error on $sql: " . mysql_error());[/code] You will also need to connect to the database first using mysql_connect(), using the information given by your hosting provider.
-
DROP means to remove the table completely. Are you checking for errors?
-
You will certainly need a ";" at the end of the drop table, eg "DROP TABLE users;" It might be better to do two seperate queries, one to drop the table and one to create the table. That will make error handling much simpler.
-
MIME Email Attachments Received Corrupted/Incomplete
btherl replied to atchua's topic in PHP Coding Help
If you can install this package, it will make life much easier: http://pear.php.net/package/Mail_Mime Or it may already be installed. One thing you might want to check is that chunk_split() is producing the correct linefeeds. It may be producing \n only. If it is, you'll need to run $data through [code=php:0]str_replace("\n","\r\n", $data)[/code]. That could easily cause truncated messages due to line length limits. -
Yes, there is a VERY close connection between forms and $_REQUEST. If you use [code=php:0]<form method=get>[/code], then all form variables will be available in $_GET and $_REQUEST when the form is submitted. If you use [code=php:0]<form method=post>[/code], then all form variables will be available in $_POST and $_REQUEST when the form is submitted. This is all done for you by PHP. You don't need to set $_GET, $_POST or $_REQUEST. Cookies are quite different, and are closely linked to sessions. For example: [code=php:0]<form method=post> <input type=text name='foo' value='bar'> <input type=submit name=Submit value=Submit> </form>[/code] If that form is submitted, then you will find [code=php:0]$_POST['foo'] = 'bar'[/code], and [code=php:0]$_POST['Submit'] = 'Submit'[/code]. You will also find those variables in $_REQUEST.
-
To learn a bit more, create the following script phpinfo.php [code=php:0]<?php phpinfo(); ?>[/code] Then call it like this: http://www.domain.com/phpinfo.php?var=value&othervar=othervalue Then scroll down and see what values are in $_GET and $_REQUEST.
-
That means "If customer_name is set as a cookie, or was sent through a GET request, or was sent through a POST request, then use whichever one is set in [code=php:0]$xtpl->assign("CUSTOMER_NAME", whichever one was set)[/code] $xtpl->assign() looks like it sets a variable for a template. Does the script use Smarty templates?
-
You don't need to zero fill once it's an integer, but it won't do any harm :) Apart from slowing things down.
-
mjdamato is right.. the field should be integer or numeric. But you might be able to get away with [code=php:0]ORDER BY CAST(score as unsigned integer)[/code]
-
Yes you can. Try it and see for yourself :)
-
You can find it using [code=php:0]$families = array_keys($eventsArray, $i);[/code] That will return an array of all the families who have a reservation on that day.
-
lucerias, try working with an example which creates two instances of a class, AND which stores data within the class. Then it should be clear why Foo::Variable() cannot use $this. While you are working with class methods only, it won't be clear why there is a difference.
-
Can you give an example of a site requesting these ids? Is it this? http://www.asp-shareware.org/pad/
-
Moving that brace to immediately after the while() should fix it. [code]while($art = mysql_fetch_array($getart)) { $title = $art[title]; ...[/code] And remove the brace after the "$content = ..." line
-
You don't need global when including files. You need global when you want to access a variable from inside a function. Eg [code=php:0]$var = 5; function foo() { global $var; print "var = $var\n"; }[/code]
-
More specifically it refers to an instance of a class. If you create two class objects, then $this will tell you which instance is being used. [code=php:0]$first = new A; $second = new A; $first->getVar(); $second->getVar()[/code] How does the class know which $var to get? When you call $first->getVar(), $this->var is the $var from $first. When you call $second->getVar(), $this->var is the $var from $second.
-
There's a few oddities in there.. Firstly, you are using the count for the total number of rows in the database to iterate through $checkbox. Probably you should count $checkbox instead. Secondly, you are displaying the table before doing the deletion. This means the table will look the same in the request in which you delete some rows, even though you are deleting some of the rows. As far as working out what's going wrong goes, try doing a var_dump() or print_r() of $checkbox, and also try printing out each $sql that you use for deleting rows. That should give you an idea of what's going wrong.