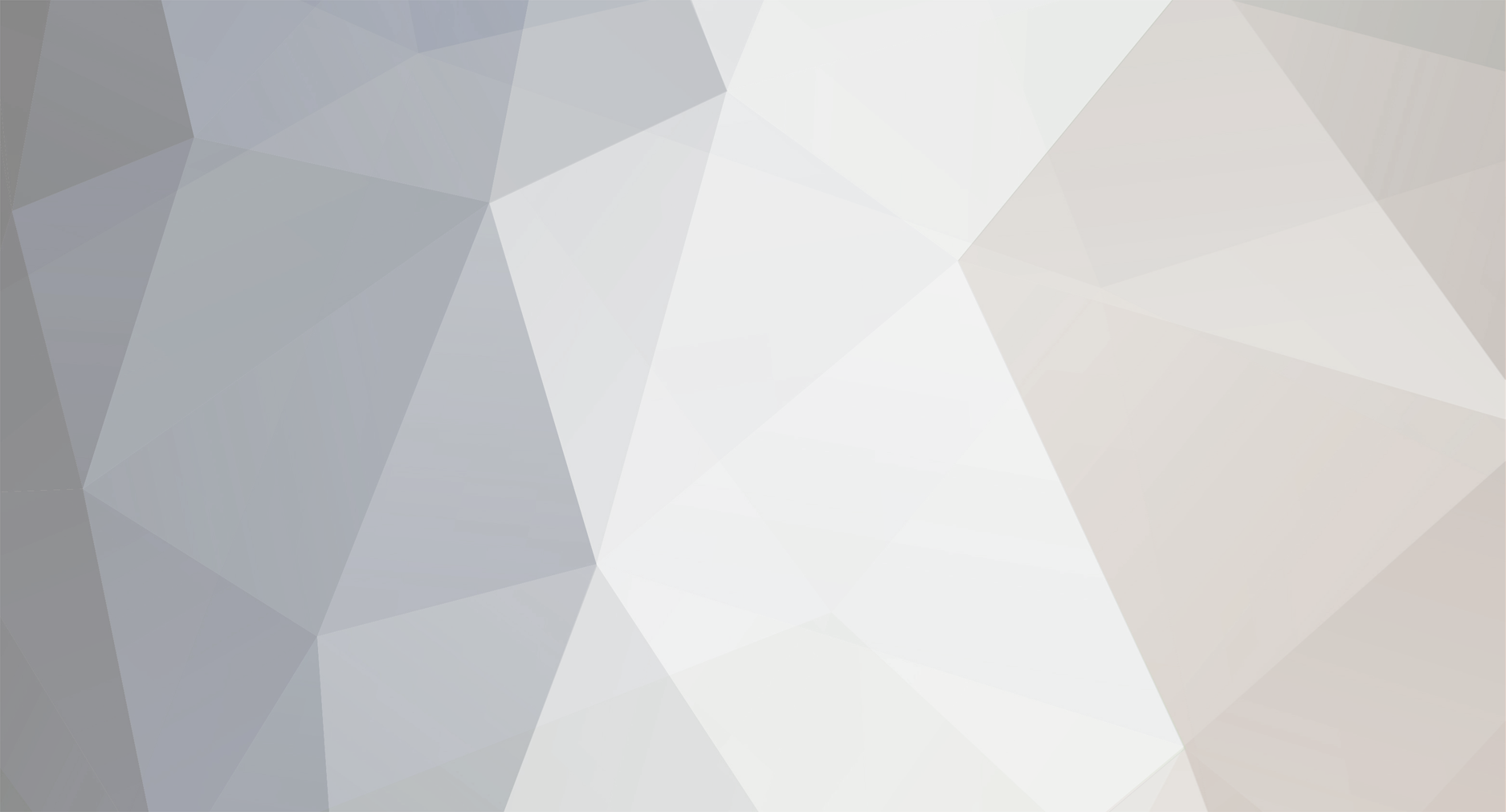
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Passing parameters and returning values by reference?
btherl replied to jipskin's topic in PHP Coding Help
Another tidbit of information to help efficiency.. arguments passed by value are passed copy-on-write. As long as you don't modfy them, the data is shared. But once you modify them, you will trigger copying of any large data structures. That means you don't need to pass large data structures by reference if you don't plan to modify them. -
What happens when you try those scripts? You might try increasing the timeout on the second one a little.. try 5 instead of 1. That means your script will take 5 seconds to determine that the server is down however.
-
***SOLVED Counting duplicates in a MySql query
btherl replied to switchdoc's topic in PHP Coding Help
Switchdoc, do you want seperate lines for each LinkID/clickdate combination (day-by-day stats), or just a seperate line for each LinkID (summary)? From your first post I thought you wanted to ignore clickdate, but now I am unsure.. Nicklas, what output does it produce? The query doesn't specify which value clickdate should take after grouping.. -
Select a list of words in a DB and replace them if present in a text
btherl replied to anarchoi's topic in PHP Coding Help
Can you give an example of what you want to do? -
You need to print out $_POST instead of returning it. Return values are only picked up through direct exec() calls.. in this case, you are execing curl which does an HTTP request. An HTTP request returns values by printing them out. Start with print_r($_POST) at the end of test2.php, and then adjust the printing format to suit your needs.
-
I can give you an overview of how I would do it.. First I would put all the relevant data into an 2-level array, like this: $files[] = array( 'name' => $filename, 'size' => $filesize($filename), ); Then I would sort it using a user sort function, like this: usort(&$files, 'filesize_cmp'); function filesize_cmp($a, $b) { if ($a['size'] < $b['size']) return -1; if ($a['size'] > $b['size']) return 1; return 0; } Take a look at the documentation page for usort() to get an idea of what it's about. It's a very flexible function.
-
***SOLVED Counting duplicates in a MySql query
btherl replied to switchdoc's topic in PHP Coding Help
Oops.. you want them grouped by LinkID only right? Ignoring the date? [code]SELECT LinkID, sum(1) as Hits FROM table GROUP BY LinkID[/code] "Select *" will not work because it selects clickdate without aggregating it (in theory anyway.. I have not tried this in mysql) -
To elaborate on that rather brief answer, you can do [code]$var = <<<EOF blah blah blah EOF; print nl2br($var);[/code] All nl2br() does is converts each newline into a br tag
-
I don't see how array_unique() can be used here.. You can structure it like this: $products[$id] = $count; if (isset($products[$id])) { $products[$id] += $another_count; } else { $products[$id] = $another_count; } Then your final array will look like: array( 111222333 => 4, 444555666 => 1, 777888999 => 3 );
-
***SOLVED Counting duplicates in a MySql query
btherl replied to switchdoc's topic in PHP Coding Help
[code]SELECT LinkID, clickdate, sum(1) as Hits FROM table GROUP BY LinkID, clickdate[/code] There you go :) -
As a general rule, flat files are faster for small sets of data. Databases are better for large sets, and for complex sets.
-
Here's how: [code]foo(array( 'result' => &$result, )); function foo($args) { $result = &$args['result']; }[/code] Because the reference is in the array it remains a reference, even though the array itself was passed copy-on-write. The referencing must be done in both places, as the variable would normally be copied both during the function call and during the assignment within the function.
-
Thanks! Then what is missing from your code is this: [code]if (!isset($_GET['ID'])) { print "No player was selected to attack.<br>"; } else { $playerID = $_GET['ID']; }[/code] That code needs to go before you use $playerID to select data from the database.
-
Ok.. in the link used to access this script you pasted, how is the player being attacked specified? Can you post an example of one of the links? Then we can show you how to use that id to fetch the player's data from the database. I would expect something like http://domain.com/script.php?playerID=5
-
[quote author=doni49 link=topic=114338.msg465246#msg465246 date=1163041187] ... see http://us2.php.net/manual/en/function.func-get-args.php [/quote] Unfortunately that has the same limitations as optional arguments to functions.. how do you tell the function that you want to set 'foo' to true, but don't want to set 'baz' to any value? func_get_args() implements variable length argument lists, but doesn't implement named arguments. Roopurt18, well spotted, I've edited that post :) Of course you must also use '&' when you call the function, unlike standard function calls which create a reference as long as it's mentioned in the function declaration.
-
Like [code]$result = foo(array( 'arg' => $arg, ));[/code] For functions which only ever take one argument I usually don't use arrays. But once the number of arguments start growing, and particularly when there are optional arguments, I switch the function over to using array arguments. It makes life so much easier for functions like this: [code]$result = foo(array( 'dbh' => $dbh, 'term' => $term, 'distance' => 3, 'wibble_tolerance' => 0.3, 'globify' => true, 'puppydogs' => 'cute', ));[/code]
-
No problems.. I will try again :) In your database query, you are fetching the player whose id is $playerID. But, you have not told php what $playerID is. So it says "undefined value". It's like you are saying to php "Fetch the player from the database", and it says "Which player? You haven't told me which one you want!" The questions to ask to solve the problem are: 1. How does my script know who player 2 is? Does it get the value from $_GET, $_POST or $_REQUEST? 2. What variable name is player 2's id stored in? Is there a line like "$playerID = $_GET['playerID']" ? 3. Why is $playerID not set to player 2's id? (You know it's not set correctly because php said "undefined value" when you tried to use it) Or you can post the whole script here and we will take a look :)
-
I often write my functions like this: [code]function foo($args) { if (array_key_exists('dbh', $args)) $dbh = $args['dbh']; if (array_key_exists('result', $args)) $result = &$args['result']; # pass by reference }[/code] Does anyone else do this? It's great for optional arguments. But it's a little annoying having all that code at the top of every function just to process the arguments. Then again, any decent function will be validating its arguments anyway. But the benefits when you call such a function are clear, especially when it has many optional arguments. No more filling in dummy arguments and trying to remember what order they should be in.
-
There's another more flexible method of passing arguments, which avoids a lot of the hassle caused by ordering of optional arguments. [code]function foo($args) { if (array_key_exists('dbh', $args)) $dbh = $args['dbh']; if (array_key_exists('result', $args)) $result = &$args['result']; # pass by reference }[/code] This allows you to set as many arguments as you want. It's quite popular in perl programming. Note that array_key_exists() allows null valued arguments, whereas isset() will not allow passing of null values. Edit: No it's me who's tired :) Now THAT'S pass by reference..
-
That error is your problem.. $playerID was never set to anything. If it's not set to any value, you can't use it to fetch data from the database. Take a look at where you set $playerID. There will be a mistake there, probably a typo, causing it not to be set. The reason the error doesn't show when you upload it is just because the server is configured not to show notices. It's not really an "error", it's just a "notice", meaning you might want to take a look at it. The problem is still there even when it doesn't show the message.
-
Next, print out the values that $atk2 was set from.. and if they are not what you expect, print out the values those values were set from :) Once you get back far enough, you'll find the problem. It may just be a typo.
-
What are the symptoms? How do you know it's being written twice? And what happens if you print something out straight after the function is called?
-
Count function won't return 0 when counting arrays from the DB
btherl replied to mschrank99's topic in PHP Coding Help
If there's nothing to be found, then $row will be set to false. The value false consists of one item, so counting it will always give you one. Instead, use [code]if ($row === false) print "Finished fetching data from database\n";[/code] -
Does the redirection get triggered even if your script is called with cmd=login as an argument? Try calling the script directly with cmd=login and see what happens. I'm not sure if Location: allows partial urls either.. giving a full url may help. But try the other suggestion first.
-
Try displaying the values of $atk1, $atk2 and everything they depend on ($userstats3['atkskill'] and so on). You'll probably notice something odd. It's the ideal method for debugging this kind of problem.