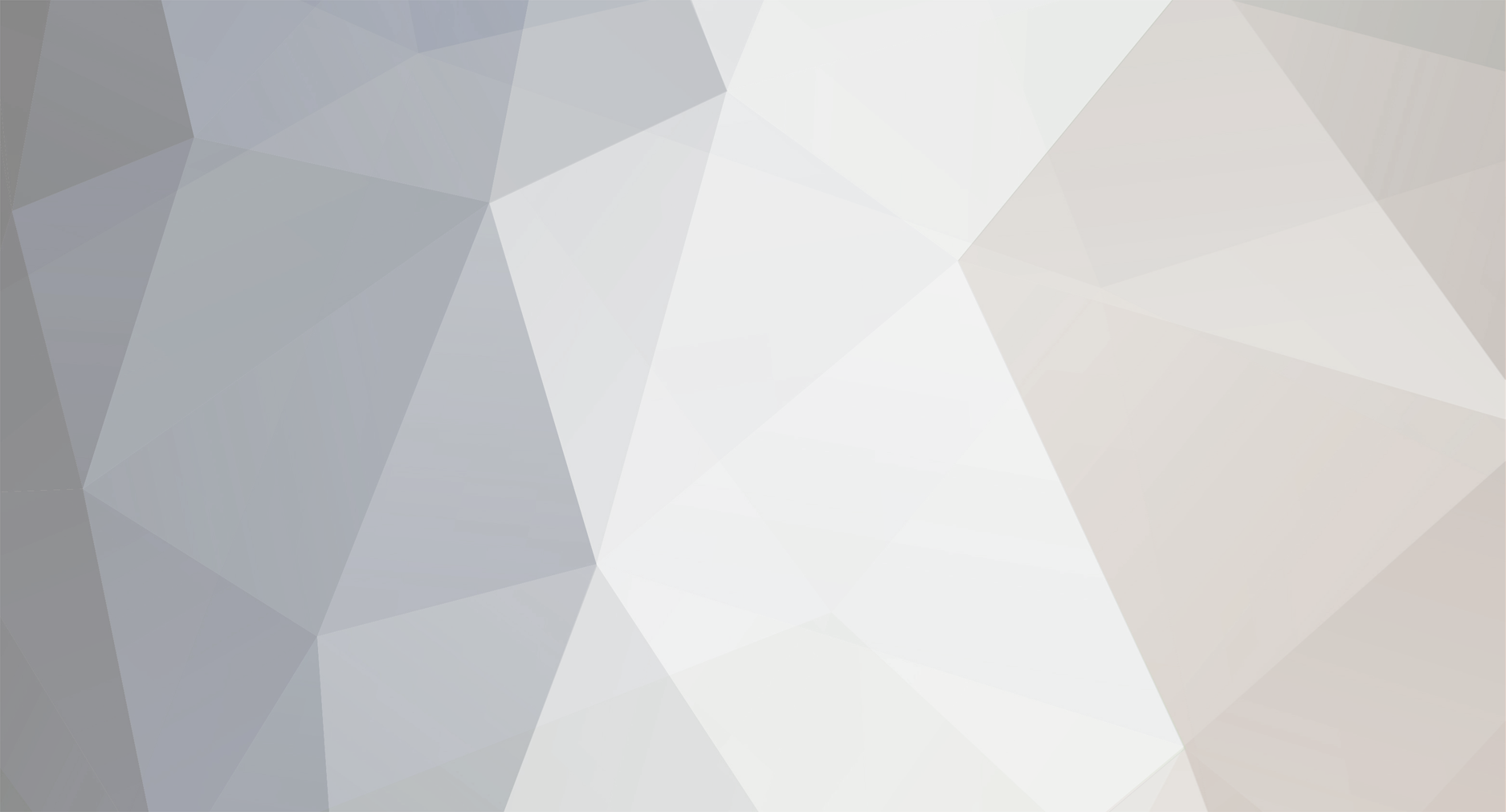
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
self::$my_static is the static class variable $my_static, $this->my_var is specific to the current copy (aka instance) of the object.
-
A simpler way to compare dates is as strings: $current_date_str = "{$current_date['year']}-{$current_date['month']}-{$current_date['day']}"; $event_date_str = "{$event_date['year']}-{$event_date['month']}-{$event_date['day']}"; if ($current_date_str < $event_date_str) { # Too early } elseif ($current_date_str == $event_date_str) { # Today } elseif ($current_date_str > $event_date_str) { # After today, need to see if it's before the end date } That'll make your code much simpler. As for events recurring at intervals, I would break it down into a few operations: 1. Check events and find when the next occurrence at or after the current day is 2. Check if that next occurrence is today A library like http://www.php.net/manual/en/datetime.add.php would help a lot for intervals of "1 calendar month" or "1 year". For weeks and fortnights you can just do arithmetic with days.
-
Have you verified that the phone actually is sending the range header?
-
You might want to try logging in with an ftp client like filezilla and looking around.
-
You can record the system time before and after the request, then subtract the start time from the end time. http://php.net/manual/en/function.microtime.php
-
If "true OOP" means you have to make everything into an object, then I am not a true believer OO is something to be used when it helps, and to be avoided when it will cause more trouble than it's worth. I think that making everything object-centric would be a mistake, just as making everything procedural would be a mistake, given that OO is available.
-
There's two basic approaches I would use 1. A regexp to extract the info 2. Parsing the HTML using a library, either event based or converting the HTML into a structure where the data can be extracted from.
-
If you find yourself writing functions with a common theme, like user_login(), user_logout(), user_get_last_login_time(), then that would make a good class. Or if you find yourself creating a seperate file to store a bunch of related functions, that's also a good class candidate. A class is a bunch of related stuff that more or less belongs together. It's analogous to a library in procedural programming, with some additional framework (keeping variables and functions together, automatic initialization and freeing with constructors/destructors, inheritance, privacy) to make life easier. You could call OO programming a "library-writing library" - a bunch of stuff useful for writing libraries. I'm also a procedural programmer who started on OO because my workplace used it.
-
Try this: var a = $(this).attr("id"); var b = $(this).attr("name"); $.post("rating.php?any="+a+"&value="+b,{}, Then you can use both $_REQUEST['value'] and $_REQUEST['any'] in rating.php
-
The query looks ok to me, assuming you're grouping by every column you are not aggregating (I haven't checked since there's so many). You say that "sometimes" it doesn't return the maximum id number - is it random, or does it depend on the input? If it's specific input, please give all details for one of the inputs it doesn't work for.
-
php can't solve equations - all it can do is follow your instructions exactly. You can program an algorithm for solving equations in php though. The code you wrote appears to converge on 2 for the real solutions to that equation. Yes, php can't handle complex numbers natively. You will need to either use or write a library that handles complex arithmetic. You can google for "php complex number class", that has some results, though I don't know how good they are.
-
Help with current folder & subfolders of domain
btherl replied to anthelo's topic in PHP Coding Help
You need to set $requested before you use explode() on it. Switch the order of the first two lines. Also $requested_parts[1] is what you need to look at, not $requested_parts. And the value to compare against is "videos", not "/videos/", because explode() will remove all the slashes. -
In that code you pasted, you are escaping the strings once. You probably have magic quotes on.
-
Almost: $sql= "UPDATE `ter` SET `BranchName` = " . ($_POST['BranchName'] ? "'{$_POST['BranchName']}'" : "`BranchName`") There was an extra double quote in the version you just posted. You'll need to do this for each column that may or may not need updating.
-
You can do it in SQL "`BranchName` = CASE WHEN '{$_POST['BranchName']}' != '' THEN '{$_POST['BranchName']}' ELSE `BranchName` END" Or in PHP "`BranchName` = " . ($_POST['BranchName'] ? "'{$_POST['BranchName']}'" : "`BranchName`") In both cases, what you're saying is "Set it to the new value if the new value is not empty, otherwise set it to the old value".
-
You've got two buttons called "submit" there. Rather than using isset(), you might want to check if $_POST['submit'] == 'Log In!' or if it == 'Submit!'. Also those forms there point to two different scripts, though it looks like this script handles both of them. What's going on there?
-
Help with current folder & subfolders of domain
btherl replied to anthelo's topic in PHP Coding Help
Try this: $requested_parts = explode('/', $requested); print "Element 1 is {$requested_parts[1]}<br."; That should show "videos" when you are at /videos/, and also when you are at /videos/something_else/ -
Check that you don't have magic_quotes enabled, and also check that you are not calling mysql_escape_string() twice on the same data. If escaped correctly, you will not have the backslashes in the database.
-
You can do it in one query with either of these methods: SELECT (SELECT count(*) FROM reviews WHERE review_rating BETWEEN 0 AND 20) AS zero_to_twenty, (SELECT count(*) FROM reviews WHERE review_rating BETWEEN 21 AND 40) AS twentyone_to_forty But that's really 5 queries all stuck together into one. SELECT SUM(CASE WHEN review_rating BETWEEN 0 AND 20 THEN 1 ELSE 0 END) AS zero_to_twenty, SUM(CASE WHEN review_rating BETWEEN 21 AND 40 THEN 1 ELSE 0 END) AS twentyone_to_forty FROM reviews This method is truly one query, and pretty much guarantees you'll only look at the table once.
-
DOMDocument::GetElementsByTagName() returns a DOMNodeList, and each item is a DOMNode. A DOMNode has a hasAttributes() method, and an attributes property of type DOMNamedNodeMap. I would expect the attributes to be in there, and accessible using DOMNamedNodeMap::getNamedItem()
-
Is this what you want? <?php float(2.93653); function float($r) { while (true) { $kissi = sqrt(1+4*(pow($kissi,2))*pow($r,2)) / sqrt( pow( (1-pow($r,2)),2)+4*pow($kissi,2)*pow($r,2)) ; print "kissi: $kissi\n"; if (abs($kissi - 0.2) < 0.00001) { break; } } echo $r ; } There were a few problems - the "for" loop was resetting $r to 0, and you need to allow a small error when testing floating point numbers for equality. Output is this: kissi: 0.131178362464 kissi: 0.164754658597 kissi: 0.181082009573 kissi: 0.189658683627 kissi: 0.194298231975 kissi: 0.196842376102 kissi: 0.198247114247 kissi: 0.199025558919 kissi: 0.199457788156 kissi: 0.199698040948 kissi: 0.199831663857 kissi: 0.199906006228 kissi: 0.199947374871 kissi: 0.199970397253 kissi: 0.19998321034 kissi: 0.199990341676 2.93653
-
What exactly are you trying to do? Are you trying to solve the equation for r?
-
Is there a reason to lean on foreach vs for? I've tried to use foreach many times and for some reason I just cannot wrap my head around it. Is it a performance/security issue? It's more of a flexibility issue. "for" will only work for arrays indexed by ordered integers, beginning at the expected number and with no gaps. For example if your indexes are "0, 1, 2, 4, 5, 6, 7" because you deleted the item with index #3, then "for" will no longer work as expected. The end result is you have to keep renumbering your arrays in order for them to work with "for". But foreach works with ANY array. It can be indexed by words, numbers with gaps, mixed numbers and words, anything. There's no need to renumber arrays after removing elements because foreach will skip them automatically. The only situation I use "for" is if I have a special reason to use ordered integer indexes in my arrays, and it's important that those indexes match up. For example if my array is storing objects at coordinates on a grid, and the indexes are the X and Y coordinates, then "for" will work better than "foreach". The other good thing about foreach is that it automatically handles the situation where your array has variable size. With "for" you need to know how long your array is, eg "for ($i = 0; $i < $array_length; $i++)", vs "foreach ($array as $item)".
-
Making the & and | bitwise operators work higher than 2^31
btherl replied to Goldeneye's topic in PHP Coding Help
Yep, by concatenation. I wouldn't call it "using a bitmask", although the effect is what you would get if you used a bitmask and then shifted the result, if php was able to do bitwise operations on more than 32 bits. I would probably go for the "l" option in pack(), "signed 32 bit integer, machine byte order". As long as you don't transport packed strings between different machines, the byte order doesn't matter. $str = pack("l*", 1,2,3); print urlencode($str) . "\n"; $ints = unpack("l*", $str); var_dump($ints); Unfortunately php doesn't accept arrays as arguments to pack(). So you'll either need to have a fixed number of integers, or you can use a loop to generate the packed string, packing 1 integer at a time and concatenating the results. You can still unpack them all in one call to unpack(), and you'll get an array as the result. -
Making the & and | bitwise operators work higher than 2^31
btherl replied to Goldeneye's topic in PHP Coding Help
The only possible reason I can imagine for using that data structure is to save memory. Is that your goal? If it is, then I can describe a complicated but very efficient way to store bits. But if you're not trying to save memory, just store the data like this: $fields = array( '5' => true, '15' => true, ); If you do want to save memory, the most efficient way to store data in php is to pack it into a string. You can pack as many integers as you want into a string, just by putting them one after another. Each integer can store 32 bits. You can read and write these integers using http://php.net/manual/en/function.pack.php and http://php.net/manual/en/function.unpack.php The integer where a bit should be stored is floor($bit_no / 32). This will give you 0 for bits 0-31, 1 for bits 32-63, and so on. If the bit numbers start with 1, then you can subtract 1 before doing the division. Does that make sense? Once you've fetched the integer you can use the normal bit manipulation operators. Then you can store it back again.