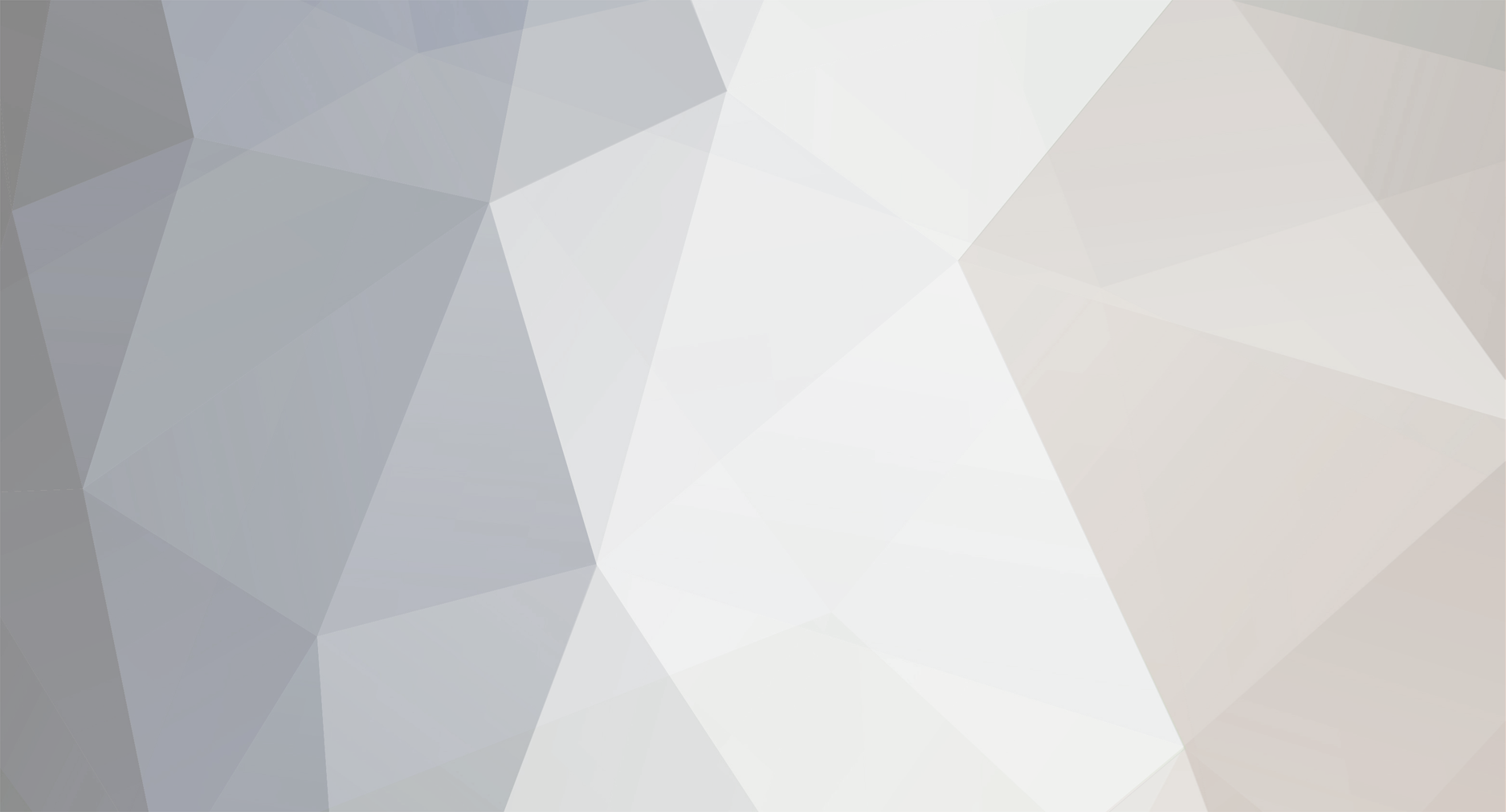
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
It would be 40 if the division was integer division. But php doesn't use integer division. From the manual:
-
Ok so the last line is solved .. I'm a bit lost in that latest code you posted. Can I recommend using some functions? For example: function format_latlong($lat1, $long1, $lat2, $long2, $timect, $colour) { $str = ''; $str .= "Color: $colour\nLine: 6,0,\"Stationary Front at ".$timect."\"\n"; $str .= ($lat1/10) . ', -' . ($lon1/10) . "\n"; $str .= ($lat2/10) . ', -' . ($lon2/10) . "\n"; $str .= "End:\n"; return $str; } print format_latlong($latstnry[$i+1], $lonstnry[$i+1], $latstnry[$i+2], $lonstnry[$i+2], $timect, $colour); Alternatively you could do the division by 10 outside the function. Either way, I think it will make the code more readable, which means it's easier to understand, and therefore easier to debug I'm also a bit lost in the logic flow because of the indenting. If you increase indent every time you enter an "if", "else" or "for", then it makes the logic much clearer.
-
It would return false. If you want a custom character set you can do it a few ways.. one is like this: if (preg_match('|^[a-zA-Z0-9 ]*$|', $string)) Meaning "If the string consists of any number of characters a-z A-Z 0-9 or space, then it's a match". Again, that will match empty strings. If you don't want empty strings you can change the * to a +, which means "one or more"
-
You can probably get away with this: if (ctype_alnum($string)) { # Yes, all alpha or numeric } Do be aware that an empty string will match because it doesn't contain any characters that are not alphanumeric, so if you don't want empty strings you'll need to check for them seperately.
-
Sorry, I forgot to mention one thing - at the end of the loop you will have one unprocessed line in $cur_line, so you need to add that final line into the array. I often forget to do that myself when using this programming pattern. So at the end: if ($cur_line !== null) $lines_arr[] = $cur_line;
-
Have you tried #1? If it works, it sounds like the best solution. If mysql and php agree on timezone then everything is easy, and you just need to convert times when you're displaying them to the user. We actually run our db servers in AEST timezone which has daylight savings, and it makes things painful I definitely wouldn't recommend that.
-
WHERE column like '%' will fetch everything. The wildcards only work for "like", not for "=".
-
What works on your home server but not on the webserver?
-
base 36 isn't a multiple of 2 which complicates things. It might be easier to use base 32, eg a-z and 0-5. Then you can encode exactly 5 bits of data in each character. Another alternative would be to start with base 64 encoding, and then do a conversion like this: a => a b => b ... z => z 0 => 0 1 => 1 ... 8 => 8 So everything from a-z and 0-8 is left unchanged, but the number 9 is reserved as a special character to indicate something from the other half of the base 64 character set. That other half can be encoded as 9 followed by one of a-z and 0-8. eg 9 => 99 A => 9a B => 9b C => 9c ... What you'll end up with is an encoding using only 36 characters.
-
Which error does it give you?
-
Try adding this to the top of your script: ini_set('display_errors', 1); It looks to me like you have a space after $schRec in that last line.
-
You forgot the "." between $_POST['sid'] and "AND CourseID ="
-
There's a few approaches to this. 1. Do a "SELECT" just before the insert, and hope no-one else inserts the same data inbetween. If you need high reliability you can lock the table first. 2. Add a unique index (or unique constraint) on StudentID and CourseID. Then if the same entry gets added twice, the second addition will get an error and will not get added. 3. Use a left join to check if the row is already in the table. This is a somewhat mind-bending option, so I don't recommend it unless you're comfortable with left joins.
-
Usually, $this->set() is a wrapper for setting a class member to a value. $this->set('foo', 'bar'); is a wrapper for $this->foo = 'bar'; The reason it's a wrapper is in case you want to change the internals of the class without affecting the interface.
-
Are you looking for a technical explanation, like "It sets users in the current object to the return value of $this->model->findAll()", or an explanation of what it actually achieves? I can only help with the technical part unfortunately.
-
Oh dear.. those line breaks complicate things. There's 2 approaches I would use in that situation: 1. Pre-process the file, sticking together broken lines into full lines, so all the HIGHS are on a single line. Then process as before. 2. Use a state based approach - Once you see the "HIGHS" line, remember that you are currently processing HIGHS. Then when you get a new line you can continue reading more HIGHS data in. Given that the line break occurs between a MD and a LATLON this approach is going to be difficult, so I would go with approach 1. For approach 1, using the rule "If we have seen the VALID line and the current line starts with a digit, append it to the previous line", the code is: $lines_arr = array(); $cur_line = null; $seen_valid = false; while ($line = fgets($file)) { if (!$seen_valid) { if (strpos($line, 'VALID') === 0) { $seen_valid = true; } continue; # Jumps up to the top of the "while" } if (!$seen_valid) continue; # Skip lines before we see the "VALID" line if (ctype_alpha($line{0})) { # Line starts with letter if ($cur_line !== null) { $lines_arr[] = $cur_line; } $cur_line = chop($line); # Making sure to remove newline with chop() } elseif (ctype_digit($line{0})) { # Line start with digit. Append to previous line $cur_line .= ' ' . chop($line); # Making sure to remove newline with chop(). And adding a space to separate the values. } } foreach ($lines_arr as $line) { # In here you can do processing on full lines, no need to worry about line breaks. }
-
Add this line back in, from my first post: if (strpos($line, "HIGHS") === 0) { .... explode and other stuff } That will make it so it processes only lines starting with "HIGHS" As for reading the file, line by line is best: $file = fopen('fff.txt','r'); while($line = fgets($file)) { if (strpos($line, "HIGHS") === 0) { $line = chop($line); # Remove newline from end of line $exploded = explode(" ", $line); $exploded_length = count($exploded); for ($i = 1; $i < $exploded_length; $i += 2) { print "MD: " . $exploded[$i] . " LATLON: " . $exploded[$i+1] . "\n"; } } } fclose($file);
-
The kind of error checking I'm talking about is like this: // create array to hold returned values $fbi = $facebook->api($apiGet); if ($fbi === false) { trigger_error("Facebook api fetch failed!", E_USER_ERROR); } I don't know if that code is correct though as I don't know what $facebook->api() returns when it fails. Usually "false" means failure.
-
Aha.. then you're looking for either obfuscation or encryption. If it's just for fun you could use mcrypt() with a basic algorithm like 3DES in ECB mode. That keeps things simple. Except that the decrypted data is padded with null bytes up to the block size of the algorithm used - you'll need to remove those bytes after decrypting.
-
That code looks fine to me, and the results are interesting, especially about how slow function_exists() is. I did some similar tests comparing arrays to switches, the results are here: http://btherl.livejournal.com/31513.html
-
If the number of items is variable, here's one way you could do it: $exploded = explode(" ", $line); $exploded_length = count($exploded); for ($i = 1; $i < $exploded_length; $i += 2) { print "MD: " . $exploded[$i] . " LATLON: " . $exploded[$i+1] . "\n"; } That gives you access to each item in turn, which you can either print right away or store into an array. Starting at index 1 skips the "HIGHS" at the start of the line, and the $i += 2 makes it jump 2 entries each time.
-
You could try it like this: if (strpos($line, "HIGHS") === 0) { list($highs, $mb1, $latlon1, $mb2, $latlon2) = explode(" ", $line); } That's assuming there are always the same number of MB and LATLON in each line like that. If not, then it'll be a bit more complex.