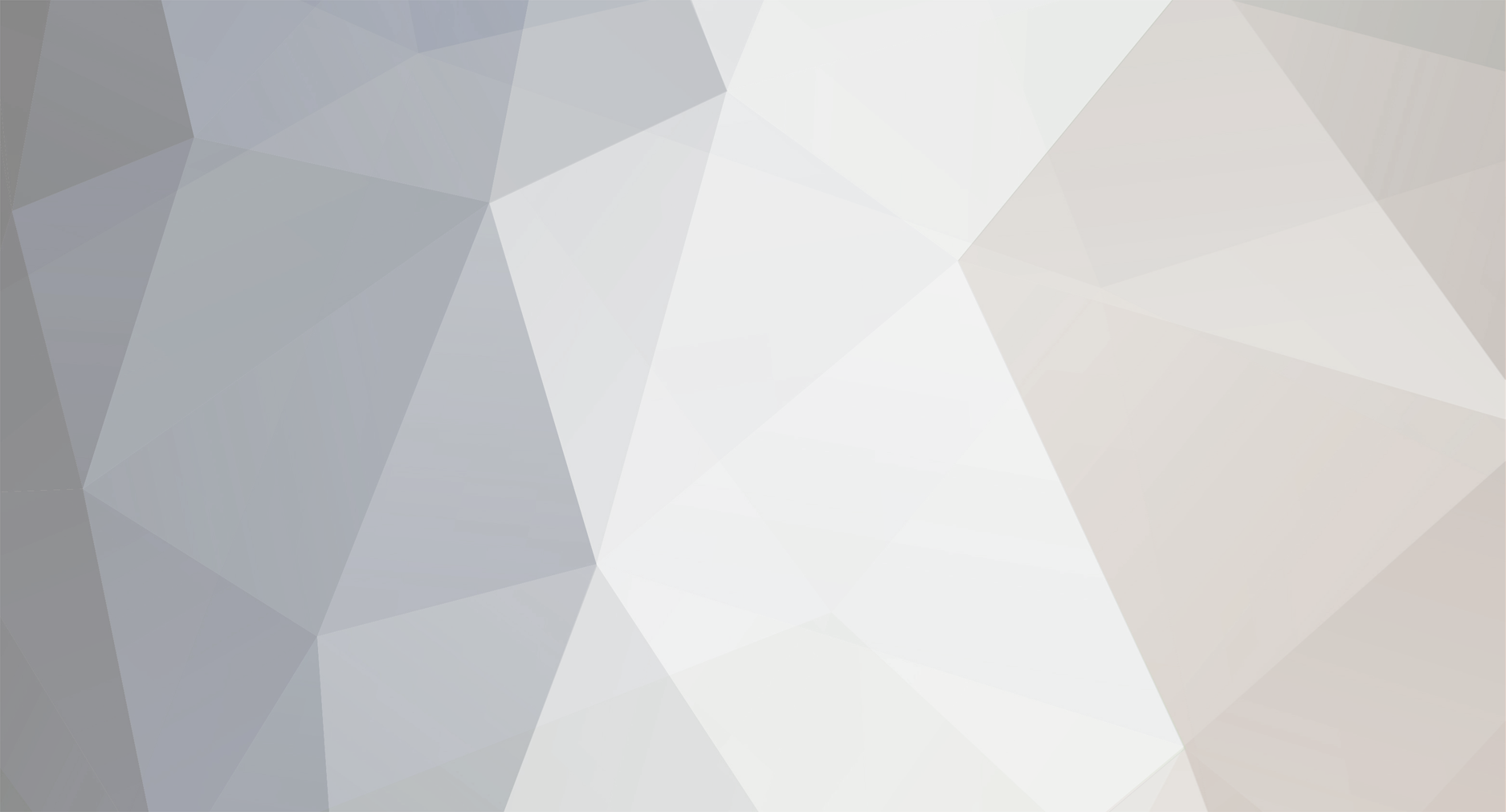
Ninjakreborn
Members-
Posts
3,922 -
Joined
-
Last visited
Everything posted by Ninjakreborn
-
<?php //---- //Personal Programmers Assistant //Created by Joyel Puryear (http://www.freelancebusinessman.com //---- //Copyright (c) 2006, "Freelance Businessman Network" //All rights reserved. //License Location: /ppassistant/userdocumentation/license.txt //---- // file functions // Function // Purpose: Get File Extensions // Parameter: Takes the full name of a file (file.ext). it strips // out the extension and leaves the .ext part. function getext($file) { $pos = strrpos($file, '.'); if(!$pos) { return FALSE; }else { $str = substr($file, $pos, strlen($file)); return $str; } } // Function // Purpose: Checks an extension for type // Parameter: You create an array of the extensions you want // to be approved, then you put them in array with the . // example .gif or .jpg. The array can be as many as you want // then you call the function and put the returned value from // getext() and the array. It returns true if it was on the // allowed list, and false if it was not (allowing you to // populate your error handler, or however you want to act on it.) function testext($ext, $allowedextensions) { if (!is_array($allowedextensions)) { return "2nd parameter has to be an array"; }else { foreach($allowedextensions as $k => $v) { if ($ext == $v) { $success = TRUE; return TRUE; } } if ($success !== TRUE) { return FALSE; } } } # Some below are from code igniter /* Instructions File Helper The File Helper file contains functions that assist in working with files. Loading this Helper This helper is loaded using the following code: $this->load->helper('file'); The following functions are available: read_file('path') Returns the data contained in the file specified in the path. Example: $string = read_file('./path/to/file.php'); The path can be a relative or full server path. Returns FALSE (boolean) on failure. Note: The path is relative to your main site index.php file, NOT your controller or view files. Code Igniter uses a front controller so paths are always relative to the main site index. If you server is running an open_basedir restriction this function might not work if you are trying to access a file above the calling script. write_file('path', $data) Writes data to the file specified in the path. If the file does not exist the function will create it. Example: $data = 'Some file data'; if ( ! write_file('./path/to/file.php', $data)) { echo 'Unable to write the file'; } else { echo 'File written!'; } You can optionally set the write mode via the third parameter: write_file('./path/to/file.php', $data, 'r+'); The default mode is wb. Please see the PHP user guide for mode options. Note: In order for this function to write data to a file its file permissions must be set such that it is writable (666, 777, etc.). If the file does not already exist, the directory containing it must be writable. Note: The path is relative to your main site index.php file, NOT your controller or view files. Code Igniter uses a front controller so paths are always relative to the main site index. delete_files('path') Deletes ALL files contained in the supplied path. Example: delete_files('/path/to/directory/'); If the second parameter is set to true, any directories contained within the supplied root path will be deleted as well. Example: delete_files('/path/to/directory/', TRUE); Note: The files must be writable or owned by the system in order to be deleted. get_filenames('path/to/directory/') Takes a server path as input and returns an array containing the names of all files contained within it. The file path can optionally be added to the file names by setting the second parameter to TRUE. Download Helper The Download Helper lets you download data to your desktop. Loading this Helper This helper is loaded using the following code: $this->load->helper('download'); The following functions are available: force_download('filename', 'data') Generates server headers which force data to be downloaded to your desktop. Useful with file downloads. The first parameter is the name you want the downloaded file to be named, the second parameter is the file data. Example: $data = 'Here is some text!'; $name = 'mytext.txt'; force_download($name, $data); If you want to download an existing file from your server you'll need to read the file into a string: $data = file_get_contents("/path/to/photo.jpg"); // Read the file's contents $name = 'myphoto.jpg'; force_download($name, $data); */ /** * Read File * * Opens the file specfied in the path and returns it as a string. * * @access public * @param string path to file * @return string */ function read_file($file) { if ( ! file_exists($file)) { return FALSE; } if (function_exists('file_get_contents')) { return file_get_contents($file); } if ( ! $fp = @fopen($file, 'rb')) { return FALSE; } flock($fp, LOCK_SH); $data = ''; if (filesize($file) > 0) { $data =& fread($fp, filesize($file)); } flock($fp, LOCK_UN); fclose($fp); return $data; } // ------------------------------------------------------------------------ /** * Write File * * Writes data to the file specified in the path. * Creates a new file if non-existent. * * @access public * @param string path to file * @param string file data * @return bool */ function write_file($path, $data, $mode = 'wb') { if ( ! $fp = @fopen($path, $mode)) { return FALSE; } flock($fp, LOCK_EX); fwrite($fp, $data); flock($fp, LOCK_UN); fclose($fp); return TRUE; } // ------------------------------------------------------------------------ /** * Delete Files * * Deletes all files contained in the supplied directory path. * Files must be writable or owned by the system in order to be deleted. * If the second parameter is set to TRUE, any directories contained * within the supplied base directory will be nuked as well. * * @access public * @param string path to file * @param bool whether to delete any directories found in the path * @return bool */ function delete_files($path, $del_dir = FALSE, $level = 0) { // Trim the trailing slash $path = preg_replace("|^(.+?)/*$|", "\\1", $path); if ( ! $current_dir = @opendir($path)) return; while(FALSE !== ($filename = @readdir($current_dir))) { if ($filename != "." and $filename != "..") { if (is_dir($path.'/'.$filename)) { $level++; delete_files($path.'/'.$filename, $del_dir, $level); } else { unlink($path.'/'.$filename); } } } @closedir($current_dir); if ($del_dir == TRUE AND $level > 0) { @rmdir($path); } } // ------------------------------------------------------------------------ /** * Get Filenames * * Reads the specified directory and builds an array containing the filenames. * Any sub-folders contained within the specified path are read as well. * * @access public * @param string path to source * @param bool whether to include the path as part of the filename * @return array */ function get_filenames($source_dir, $include_path = FALSE) { static $_filedata = array(); if ($fp = @opendir($source_dir)) { while (FALSE !== ($file = readdir($fp))) { if (@is_dir($source_dir.$file) && substr($file, 0, 1) != '.') { get_filenames($source_dir.$file."/", $include_path); } elseif (substr($file, 0, 1) != ".") { $_filedata[] = ($include_path == TRUE) ? $source_dir.$file : $file; } } return $_filedata; } } /** * Force Download * * Generates headers that force a download to happen * * @access public * @param string filename * @param mixed the data to be downloaded * @return void */ function force_download($filename = '', $data = '') { if ($filename == '' OR $data == '') { return FALSE; } // Try to determine if the filename includes a file extension. // We need it in order to set the MIME type if (FALSE === strpos($filename, '.')) { return FALSE; } // Grab the file extension $x = explode('.', $filename); $extension = end($x); // Load the mime types @include(APPPATH.'config/mimes'.EXT); // Set a default mime if we can't find it if ( ! isset($mimes[$extension])) { $mime = 'application/octet-stream'; } else { $mime = (is_array($mimes[$extension])) ? $mimes[$extension][0] : $mimes[$extension]; } // Generate the server headers if (strstr($_SERVER['HTTP_USER_AGENT'], "MSIE")) { header('Content-Type: "'.$mime.'"'); header('Content-Disposition: attachment; filename="'.$filename.'"'); header('Expires: 0'); header('Cache-Control: must-revalidate, post-check=0, pre-check=0'); header("Content-Transfer-Encoding: binary"); header('Pragma: public'); header("Content-Length: ".strlen($data)); } else { header('Content-Type: "'.$mime.'"'); header('Content-Disposition: attachment; filename="'.$filename.'"'); header("Content-Transfer-Encoding: binary"); header('Expires: 0'); header('Pragma: no-cache'); header("Content-Length: ".strlen($data)); } echo $data; } ?> <?php //---- //Personal Programmers Assistant //Created by Joyel Puryear (http://www.freelancebusinessman.com //---- //Copyright (c) 2006, "Freelance Businessman Network" //All rights reserved. //License Location: /ppassistant/userdocumentation/license.txt //---- // file functions // Function // Purpose: Get File Extensions // Parameter: Takes the full name of a file (file.ext). it strips // out the extension and leaves the .ext part. function getext($file) { $pos = strrpos($file, '.'); if(!$pos) { return FALSE; }else { $str = substr($file, $pos, strlen($file)); return $str; } } // Function // Purpose: Checks an extension for type // Parameter: You create an array of the extensions you want // to be approved, then you put them in array with the . // example .gif or .jpg. The array can be as many as you want // then you call the function and put the returned value from // getext() and the array. It returns true if it was on the // allowed list, and false if it was not (allowing you to // populate your error handler, or however you want to act on it.) function testext($ext, $allowedextensions) { if (!is_array($allowedextensions)) { return "2nd parameter has to be an array"; }else { foreach($allowedextensions as $k => $v) { if ($ext == $v) { $success = TRUE; return TRUE; } } if ($success !== TRUE) { return FALSE; } } } # Some below are from code igniter /* Instructions File Helper The File Helper file contains functions that assist in working with files. Loading this Helper This helper is loaded using the following code: $this->load->helper('file'); The following functions are available: read_file('path') Returns the data contained in the file specified in the path. Example: $string = read_file('./path/to/file.php'); The path can be a relative or full server path. Returns FALSE (boolean) on failure. Note: The path is relative to your main site index.php file, NOT your controller or view files. Code Igniter uses a front controller so paths are always relative to the main site index. If you server is running an open_basedir restriction this function might not work if you are trying to access a file above the calling script. write_file('path', $data) Writes data to the file specified in the path. If the file does not exist the function will create it. Example: $data = 'Some file data'; if ( ! write_file('./path/to/file.php', $data)) { echo 'Unable to write the file'; } else { echo 'File written!'; } You can optionally set the write mode via the third parameter: write_file('./path/to/file.php', $data, 'r+'); The default mode is wb. Please see the PHP user guide for mode options. Note: In order for this function to write data to a file its file permissions must be set such that it is writable (666, 777, etc.). If the file does not already exist, the directory containing it must be writable. Note: The path is relative to your main site index.php file, NOT your controller or view files. Code Igniter uses a front controller so paths are always relative to the main site index. delete_files('path') Deletes ALL files contained in the supplied path. Example: delete_files('/path/to/directory/'); If the second parameter is set to true, any directories contained within the supplied root path will be deleted as well. Example: delete_files('/path/to/directory/', TRUE); Note: The files must be writable or owned by the system in order to be deleted. get_filenames('path/to/directory/') Takes a server path as input and returns an array containing the names of all files contained within it. The file path can optionally be added to the file names by setting the second parameter to TRUE. Download Helper The Download Helper lets you download data to your desktop. Loading this Helper This helper is loaded using the following code: $this->load->helper('download'); The following functions are available: force_download('filename', 'data') Generates server headers which force data to be downloaded to your desktop. Useful with file downloads. The first parameter is the name you want the downloaded file to be named, the second parameter is the file data. Example: $data = 'Here is some text!'; $name = 'mytext.txt'; force_download($name, $data); If you want to download an existing file from your server you'll need to read the file into a string: $data = file_get_contents("/path/to/photo.jpg"); // Read the file's contents $name = 'myphoto.jpg'; force_download($name, $data); */ /** * Read File * * Opens the file specfied in the path and returns it as a string. * * @access public * @param string path to file * @return string */ function read_file($file) { if ( ! file_exists($file)) { return FALSE; } if (function_exists('file_get_contents')) { return file_get_contents($file); } if ( ! $fp = @fopen($file, 'rb')) { return FALSE; } flock($fp, LOCK_SH); $data = ''; if (filesize($file) > 0) { $data =& fread($fp, filesize($file)); } flock($fp, LOCK_UN); fclose($fp); return $data; } // ------------------------------------------------------------------------ /** * Write File * * Writes data to the file specified in the path. * Creates a new file if non-existent. * * @access public * @param string path to file * @param string file data * @return bool */ function write_file($path, $data, $mode = 'wb') { if ( ! $fp = @fopen($path, $mode)) { return FALSE; } flock($fp, LOCK_EX); fwrite($fp, $data); flock($fp, LOCK_UN); fclose($fp); return TRUE; } // ------------------------------------------------------------------------ /** * Delete Files * * Deletes all files contained in the supplied directory path. * Files must be writable or owned by the system in order to be deleted. * If the second parameter is set to TRUE, any directories contained * within the supplied base directory will be nuked as well. * * @access public * @param string path to file * @param bool whether to delete any directories found in the path * @return bool */ function delete_files($path, $del_dir = FALSE, $level = 0) { // Trim the trailing slash $path = preg_replace("|^(.+?)/*$|", "\\1", $path); if ( ! $current_dir = @opendir($path)) return; while(FALSE !== ($filename = @readdir($current_dir))) { if ($filename != "." and $filename != "..") { if (is_dir($path.'/'.$filename)) { $level++; delete_files($path.'/'.$filename, $del_dir, $level); } else { unlink($path.'/'.$filename); } } } @closedir($current_dir); if ($del_dir == TRUE AND $level > 0) { @rmdir($path); } } // ------------------------------------------------------------------------ /** * Get Filenames * * Reads the specified directory and builds an array containing the filenames. * Any sub-folders contained within the specified path are read as well. * * @access public * @param string path to source * @param bool whether to include the path as part of the filename * @return array */ function get_filenames($source_dir, $include_path = FALSE) { static $_filedata = array(); if ($fp = @opendir($source_dir)) { while (FALSE !== ($file = readdir($fp))) { if (@is_dir($source_dir.$file) && substr($file, 0, 1) != '.') { get_filenames($source_dir.$file."/", $include_path); } elseif (substr($file, 0, 1) != ".") { $_filedata[] = ($include_path == TRUE) ? $source_dir.$file : $file; } } return $_filedata; } } /** * Force Download * * Generates headers that force a download to happen * * @access public * @param string filename * @param mixed the data to be downloaded * @return void */ function force_download($filename = '', $data = '') { if ($filename == '' OR $data == '') { return FALSE; } // Try to determine if the filename includes a file extension. // We need it in order to set the MIME type if (FALSE === strpos($filename, '.')) { return FALSE; } // Grab the file extension $x = explode('.', $filename); $extension = end($x); // Load the mime types @include(APPPATH.'config/mimes'.EXT); // Set a default mime if we can't find it if ( ! isset($mimes[$extension])) { $mime = 'application/octet-stream'; } else { $mime = (is_array($mimes[$extension])) ? $mimes[$extension][0] : $mimes[$extension]; } // Generate the server headers if (strstr($_SERVER['HTTP_USER_AGENT'], "MSIE")) { header('Content-Type: "'.$mime.'"'); header('Content-Disposition: attachment; filename="'.$filename.'"'); header('Expires: 0'); header('Cache-Control: must-revalidate, post-check=0, pre-check=0'); header("Content-Transfer-Encoding: binary"); header('Pragma: public'); header("Content-Length: ".strlen($data)); } else { header('Content-Type: "'.$mime.'"'); header('Content-Disposition: attachment; filename="'.$filename.'"'); header("Content-Transfer-Encoding: binary"); header('Expires: 0'); header('Pragma: no-cache'); header("Content-Length: ".strlen($data)); } echo $data; } ?> There you go, that should help some. These functions here can do almost anything with files. For the zipping it's not that hard. The functions up there can easly get you directory listing of items in folders (as well as sub-folders) and everything else). You may even find one up there that deals with zipping, not sure if I had one for that, but it's not hard just wshow your code.
-
Opinions.
-
I found a solution. I got a class that turns xml into array. I was looking for xml parsing, and then found xml->array which they are all over the place. That's exactly what I needed.
-
I have been stuck on this issue for roughly 3-4 days. Basically I am querying amazon.com ecs system to get data from them in xml format (the only format they provide). What I am trying to do is this. * Make a request to there system * Set all the values in an array * make a second request * Add the values into the existing array * Continue that cycle until I am done * form a nice table using the array. I have tried everything. Expat, Simple XML, XML classes, and nothing is working. Can anyone point me in the right direction for this type of issue?
-
[SOLVED] Simple XML parsing problems (coming back blank)
Ninjakreborn replied to Ninjakreborn's topic in PHP Coding Help
Ah, to do multi levels inside an xml file using "simple xml" you do something like this. <?php // send request $request = 'http://ecs.amazonaws.com/onca/xml?Service=AWSECommerceService&' . 'AWSAccessKeyId=06NSVBP9J0TT6AXYGER2&Operation=ItemSearch&SearchIndex=DVD&Actor=Brad%20Pitt'; // get data from request $response = file_get_contents($request); // create temporary xml file based off data retrieved $fp = fopen("temp.xml", "w"); fwrite($fp, $response); fclose($fp); // parse/display the xml $file = 'temp.xml'; if (file_exists($file)) { $xml = simplexml_load_file('temp.xml'); echo $xml->getName() . "<br />"; foreach($xml->children() as $child) { foreach($child->children() as $childsecond) { foreach($childsecond->children() as $childthird) { echo $childthird->getName() . ": " . $childthird . "<br />"; } } } } ?> -
My xml file (example) is like this <?xml version="1.0" encoding="UTF-8"?><ItemSearchResponse xmlns="http://webservices.amazon.com/AWSECommerceService/2005-10-05"><OperationRequest><HTTPHeaders><Header Name="UserAgent"></Header></HTTPHeaders><RequestId>1QPP350WQXB5R08VSM7B</RequestId><Arguments><Argument Name="SearchIndex" Value="DVD"></Argument><Argument Name="Service" Value="AWSECommerceService"></Argument><Argument Name="Operation" Value="ItemSearch"></Argument><Argument Name="Actor" Value="Brad Pitt"></Argument><Argument Name="AWSAccessKeyId" Value="06NSVBP9J0TT6AXYGER2"></Argument></Arguments><RequestProcessingTime>0.174333810806274</RequestProcessingTime></OperationRequest><Items><Request><IsValid>True</IsValid><ItemSearchRequest><Actor>Brad Pitt</Actor><SearchIndex>DVD</SearchIndex></ItemSearchRequest></Request><TotalResults>179</TotalResults><TotalPages>18</TotalPages><Item><ASIN>B000TGGJKU</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B000TGGJKU%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B000TGGJKU%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Brad Pitt</Actor><Actor>Eric Bana</Actor><Actor>Orlando Bloom</Actor><Director>Wolfgang Petersen</Director><Manufacturer>Warner Home Video</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Troy (Director's Cut) [blu-ray]</Title></ItemAttributes></Item><Item><ASIN>B000TGJ8IU</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B000TGJ8IU%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B000TGJ8IU%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Brad Pitt</Actor><Actor>Eric Bana</Actor><Actor>Orlando Bloom</Actor><Manufacturer>Warner Home Video</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Troy - Director's Cut (Ultimate Collector's Edition)</Title></ItemAttributes></Item><Item><ASIN>B00003W8NM</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B00003W8NM%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B00003W8NM%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Edward Norton</Actor><Actor>Brad Pitt</Actor><Actor>Meat Loaf</Actor><Actor>Helena Bonham Carter</Actor><Actor>Zach Grenier</Actor><Director>David Fincher</Director><Manufacturer>20th Century Fox</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Fight Club (Two-Disc Collector's Edition)</Title></ItemAttributes></Item><Item><ASIN>B000062XHI</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B000062XHI%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B000062XHI%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Don Cheadle</Actor><Actor>George Clooney</Actor><Actor>Holly Marie Combs</Actor><Actor>Matt Damon</Actor><Actor>Michael de Lano</Actor><Creator Role="Cinematographer">Peter Andrews</Creator><Creator Role="Composer">David Holmes</Creator><Manufacturer>Warner Home Video</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Ocean's Eleven (Widescreen Edition)</Title></ItemAttributes></Item><Item><ASIN>B000TGGJKK</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B000TGGJKK%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B000TGGJKK%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Brad Pitt</Actor><Actor>Eric Bana</Actor><Actor>Orlando Bloom</Actor><Director>Wolfgang Petersen</Director><Manufacturer>Warner Home Video</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Troy - Director's Cut [HD DVD]</Title></ItemAttributes></Item><Item><ASIN>B000093FLA</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B000093FLA%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B000093FLA%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Ade</Actor><Actor>William Beck (II)</Actor><Actor>Andy Beckwith</Actor><Actor>Ewen Bremner</Actor><Actor>Jason Buckham</Actor><Creator Role="Primary Contributor">Statham, Jason</Creator><Manufacturer>Sony Pictures</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Snatch (Widescreen Edition)</Title></ItemAttributes></Item><Item><ASIN>B0007P0XBO</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B0007P0XBO%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B0007P0XBO%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Brad Pitt</Actor><Actor>Catherine Zeta-Jones</Actor><Actor>George Clooney</Actor><Actor>Ed Kross</Actor><Actor>Julia Roberts</Actor><Director>Steven Soderbergh</Director><Manufacturer>Warner Home Video</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Ocean's Twelve</Title></ItemAttributes></Item><Item><ASIN>B00004WG2F</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B00004WG2F%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B00004WG2F%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Brad Pitt</Actor><Actor>Anthony Hopkins</Actor><Actor>Aidan Quinn</Actor><Actor>Julia Ormond</Actor><Actor>Henry Thomas</Actor><Director>Edward Zwick</Director><Manufacturer>Sony Pictures</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Legends of the Fall (Special Edition)</Title></ItemAttributes></Item><Item><ASIN>B000MCH5P4</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B000MCH5P4%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B000MCH5P4%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Brad Pitt</Actor><Actor>Cate Blanchett</Actor><Actor>Mohamed Akhzam</Actor><Actor>Peter Wight</Actor><Actor>Harriet Walter</Actor><Director>Alejandro González Iñárritu</Director><Manufacturer>Paramount</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Babel</Title></ItemAttributes></Item><Item><ASIN>B00004RFFS</ASIN><DetailPageURL>http://www.amazon.com/gp/redirect.html%3FASIN=B00004RFFS%26tag=ws%26lcode=xm2%26cID=2025%26ccmID=165953%26location=/o/ASIN/B00004RFFS%253FSubscriptionId=06NSVBP9J0TT6AXYGER2</DetailPageURL><ItemAttributes><Actor>Tom Cruise</Actor><Actor>Brad Pitt</Actor><Actor>Kirsten Dunst</Actor><Actor>Stephen Rea</Actor><Actor>Antonio Banderas</Actor><Director>Neil Jordan</Director><Manufacturer>Warner Home Video</Manufacturer><ProductGroup>DVD</ProductGroup><Title>Interview with the Vampire</Title></ItemAttributes></Item></Items></ItemSearchResponse> PHP Parsing Page <?php // send request $request = 'http://ecs.amazonaws.com/onca/xml?Service=AWSECommerceService&' . 'AWSAccessKeyId=06NSVBP9J0TT6AXYGER2&Operation=ItemSearch&SearchIndex=DVD&Actor=Brad%20Pitt'; // get data from request $response = file_get_contents($request); // create temporary xml file based off data retrieved $fp = fopen("temp.xml", "w"); fwrite($fp, $response); fclose($fp); // parse/display the xml $file = 'temp.xml'; if (file_exists($file)) { $xml = simplexml_load_file('temp.xml'); echo $xml->getName() . "<br />"; foreach($xml->children() as $child) { echo $child->getName() . ": " . $child . "<br />"; } } ?> It returns the names of the elements as it should, but not the "data" that's suppose to be within them. Am I doing something wrong here. The xml file changes obviously each time, but that is the basic format that is always returned.
-
I am having problems with this. I don't program java (yet) I go to the website and download the classic versionand I have a java version. But if I try to open files with another extension it tries to open them in another editor. I read in the sticky where oblivion spoke about using it,and said it was great. I can't seem to figure out which one is the proper download. I just need a general editor for anything. .php, html, xms, xsl, xhtml, css, whatever. With hopefully some decent syntax highlighting, which eclipse download do I need to get for this?
-
I finally got one. It took me forever, and I found it, finally. Thanks for all the feedback, demonoid is the best torrent site I have ever found.
-
Crap they look pretty good. I am hoping they open up soon, I tried everyone out there, and after awhile I kept seeing demonoid as a tracker and eventually visited it as a site. Did some searching throughout there torrents. That site is amazing.
-
You could work up a factoring system possible. No-one can learn everything, but someone can certainly learn almost everything about 1 thing. You can pick up a list of subjects related to php. Basics - (all basic stuff, variables, control structures, array setting). Advanced Arrays File handling Directory handling function memory so forth, and work up a list of like 10-15 different categories. With that, you can rate your skills in each. 0 = never done anythign with it 100% know everything about it. Then factor up a total percentage. That would not be that hard, and it could be made logical if thought out well enough.
-
I have recently gotten involved in torrents. Why do they have closed registration. Does anyone know how to get into demonoid.com
-
I have created a table that contains tabular data. I had the need to make it hide/show columns (using jquery). Now, It is working perfectly in IE, but there are problems in firefox. I am basically doing the sections in t-bodies. It took me quite awhile to get the hide/show features working right, and they are perfect in ie, but there messing up in firefox. There are gaps in between the sections and other small issues. I have used FF developer tools, to disable all css one at a time. Inline, internal, and each external one (one at a time), and I haven't found any issues that was actually causing the problem. Now I need to figure out what's causing it but I am having no luck. This project is from a private client so I can't show a lot of "project specific" stuff, but I can post some of the 'non-related' code. For example, the whole thing is done with a giant table. It has each hide/show section in a t-body. It is then powered by a few basic functions which show/hide the sections as needed (with programming here and there throughout the view page in order for it to work properly. Below I have included the basic javascript function, with project specific relations taken out. This will show you what I am trying to do nevertheless. var selected_tab = ""; function hide_element(elementName) { var c = $('#'+elementName+'_collapsable'); if (c) { c[0].app_width = c.width(); c.hide('slow'); } c = $('#'+elementName+'_title'); if (c) { var a = $('a:first', c); if (a) { a.before('<a href="javascript:void" onclick="return show2_element(\''+elementName+'\');">{{$html->image('plus.gif')}}</a>'); a.remove(); } } return false; } function show2_element(elementName) { var d = $('#' + elementName + '_collapsable'); if (d) d.show('slow', function(){ var d=$('#'+elementName+'_collapsable'); d.width(d[0].app_width).css("display", ""); }); c = $('#'+elementName+'_title'); if (c) { var a = $('a:first', c); if (a) { a.before('<a href="javascript:void" onclick="return hide_element(\''+elementName+'\');">{{$html->image('minus.gif')}}</a>'); a.remove(); } } return false; } function show_third(elementName) { var d = $('.' + elementName + '_expand'); if (d) d.show('slow', function(){ var d=$('.'+elementName+'_expand'); //d.width(d[0].app_width); d.css("display", ""); }); c = $('#'+elementName+'_title'); if (c) { var a = $('a:first', c); if (a) { a.before('<a href="javascript:void" onclick="return hide_third(\''+elementName+'\');">{{$html->image('minus.gif')}}</a>'); a.remove(); } } return false; } function hide_third(elementName) { var c = $('.'+elementName+'_expand'); if (c) { //c[0].app_width = c.width(); c.hide('slow'); } c = $('#'+elementName+'_title'); if (c) { var a = $('a:first', c); if (a) { a.before('<a href="javascript:void" onclick="return show_third(\''+elementName+'\');">{{$html->image('plus.gif')}}</a>'); a.remove(); } } return false; } That code will show you exactly what is causing the hide/show. I just need to figure out what I need to do in order to remove the css issues in firefox, or how to make it work right in firefox. Also it uses jquery as the javascript framework.
-
I just like naming them myself like redbully does except reverse. math.class.php pdf.class.php For all of my classes. files.function.php whatever.function.php
-
I got it. Basically to simplify my original question. "How do you merge heavily multi-dimensional arrays?" To answer my on question in one sentence. "Look for a function on php.net under "array_merge()" in the users comments that is called "multimerge()"."
-
I can't mess with the models. Only the controllers and view files. I have another developer that handles all the models. Basically (I can't reveal much) but basically there are 3 databas calls. Which gather data. The query calls provide all the data that i need to form the arrays. It's just I am having a hard time getting them to fall into place correctly. Because of my lack in information I might be on my own. I just don't want to accidentally reveal anything that might get me in trouble with the client. The system, code, and app are all closed source and for internal usage. So I can't accidentally reveal anything that will get me screwed.
-
It is, there are multiple arrays. The main array contains a list of customer keys with information in that. Then a products array inside of that with a list of product information. Now, I am trying something(getting 3 different arrays and adding them together with +) I hope that works.
-
I have all of this in the $products array. If I do $products for the first one $products1 for the second loop $products2 for the third and then do array merge at the end will all the data filter in together as I described?
-
I have a really large system that pulls lot's of stuff from a database. From about 4-5 different queries it runs arrays of data into arrays from the fields. I have a products array on the first one which collects customers, products and other information. As follows [customerid] [customername] [customerid] [products] [productid and information in this array] Basically this is the array setup that is created at first. However when it goes through future database structures new customers might get added entirely (which should add a new customer id and customer details). Or some NEW products might get added(which need to add without having a chance of overwriting current projects. Then again some products might have data that need's to be added to that specific product. Does anyone have a tutorial, tips, tricks on writing "intellegent" arrays?
-
http://www.google.com/search?hl=en&q=basic+cronjob+setup Look at the second link there.
-
[SOLVED] Help with posting to a database.
Ninjakreborn replied to gareth886's topic in PHP Coding Help
Just grab request 1 2 and 3. <?php $request2 = $_POST['request2']; ?> Just as an example. Then just database it like you would a normal field. -
Yes there are a few options. * Cron Job * External Cron Job umm. You going in an manually clicking a button to run the procedure. Probably other's that's all I have off the top of my head.
-
[SOLVED] Help with posting to a database.
Ninjakreborn replied to gareth886's topic in PHP Coding Help
You just grab the post value of the checkbox the same you would anything (unless the form is get then you use get). -
Regular Expressions.
-
Just check for the time. If it's 12:00 or after then change it out. However database a temp field stating what "date" it changed. That way it won't try to re-change it multiple times per day.
-
Let's see let's cut up your code and see if we can find out what the problem is.... Ok you can't use it like that. The database queries inside of javascript are not going to work. They are php not javascript that is impossible. You can pass variables "to" javascript though and vice versa in a few situations (very few). You are going to need to look into ajax to do a call to a server side php page to perform the actions. Do some checking on ajax and get the basic concepts then post back here when you have something basic to look at.