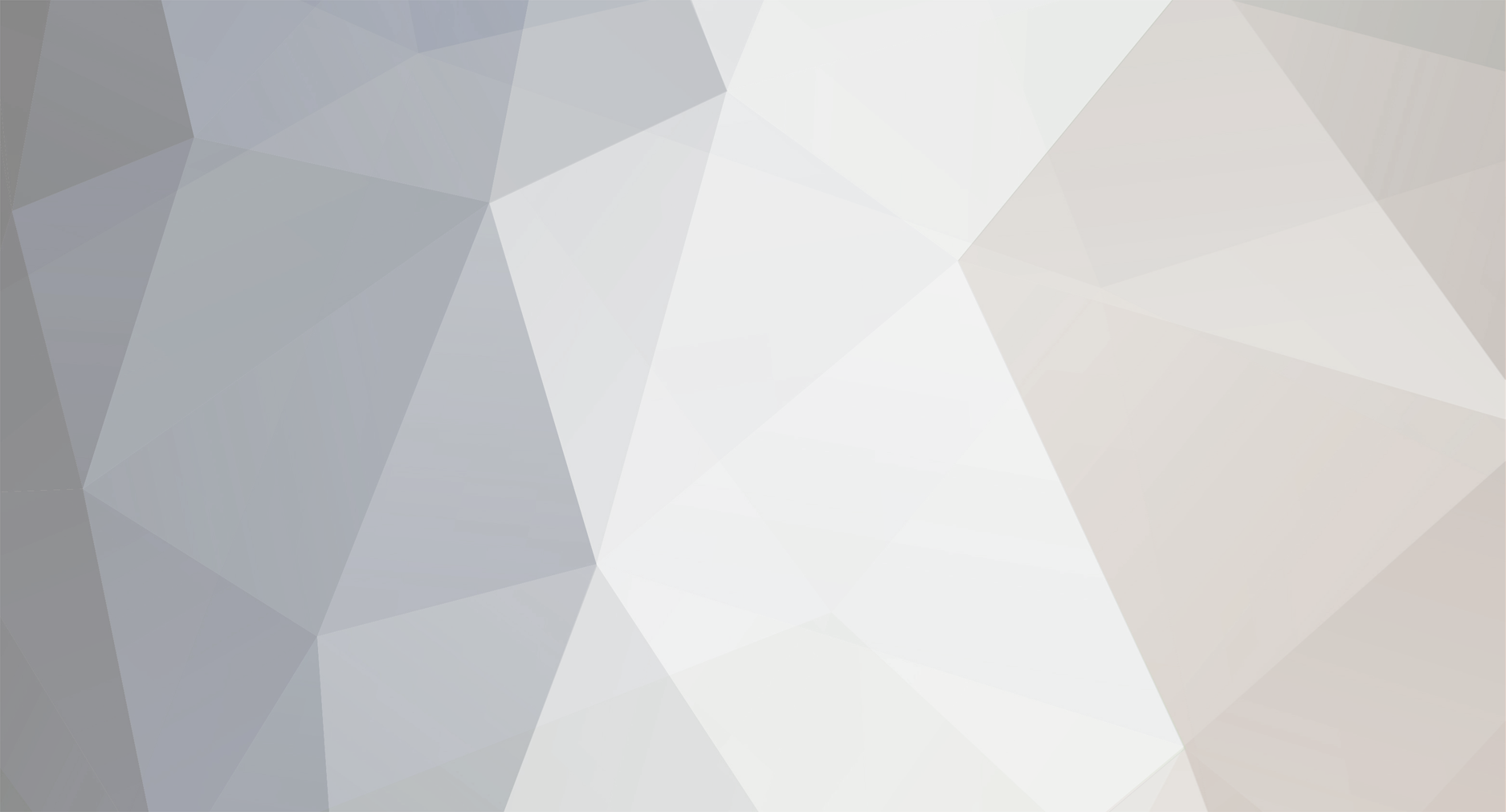
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
"I imported the mysql database from the win box to the linux box." It depends on your definition of import. If you went into phpMyAdmin, picked a database, and created a .sql (or .zip) which you then imported into the linux machine via it's phpMyAdmin, then the user privileges for the MySQL server are not intact. Is this linux box provided via a hosting company? If it is, then the e-mail they sent you when you signed up for their hosting will contain the host, user, and password information for the MySQL server.
-
The MySQL server on the linux host might have different values than the windows server for the values: host user password Businessman is stating that you should ensure that you are using the correct values.
-
Parse error: syntax error, unexpected ';', expecting
roopurt18 replied to suttercain's topic in PHP Coding Help
Are those typos actually in the book? -
http://www.phpfreaks.com/forums/index.php/topic,115254.msg469365/topicseen.html#msg469365 http://www.phpfreaks.com/forums/index.php/topic,114046.0.html Those should get you started.
-
Requiring JavaScript before executing PHP
roopurt18 replied to Ryan Williams's topic in PHP Coding Help
Do spambots work with sessions? i.e. if a spambot is browsing pages on a site, will $_SESSION store values across pages? If that's the case I may have a solution. -
I'd do the following: 1) First, change your login mechanism so that a user may be logged in once and only once. This will prevent them from using one window of IE and another of FF, which will then allow you to use $_SESSION to solve the problem. 2) You undoubtedly have a function that handles the form input. Modify the function like so: [code] // At the top of the function if(isset($_SESSION['lock_bank'])){ return; } $_SESSION['lock_bank'] = true; // Do the regular processing // At the bottom of the function unset($_SESSION['lock_bank']); [/code] I guess there is still a risk of two requests being handled at once, although I think it's a much, much smaller risk. The only other suggestion I could make is to switch the table to type InnoDB and use transactions.
-
Because it looks bad is a perfectly valid reason.
-
It took me a while to get to this point, but I like to break things down into small components that pop data in place using the <?=$var?> syntax. I also allow for simple and extremely limited logic / program control within components, but the idea is 99% of the time that is handled elsewhere and the component merely slaps data into place. As another general rule, I try and keep component files small; generally I can see the entire code contained in a component file without scrolling the text editor.
-
Reading dynamically created fields. $_POST does not work
roopurt18 replied to mweinberger's topic in PHP Coding Help
All of those \t's are giving me a headache. -
That particular method gives the would-be hacker a little bit of extra information. Namely that you handled the particular attack that they tried, which is bad practice IMO. In addition, you run the risk of a legitimate user viewing that message, which is something you don't want to spring on a legitimate user.
-
I personally never use die in my code. The type of information programmers tend to place in their die statements is exactly the type of information you don't want non-developers to have access to. It's a much better practice, IMO, to program your application to handle failure in a graceful manner. For example, if someone attempts to hack my site, I prefer the product page to come up with a message stating, "Sorry, but no products found." instead of "die: You have an error in your MySQL statement. Check the syntax for the correct usage near your_sql_query."
-
1) Sanitize all data going into the DB: <?php // my_clean // $data - data to clean before inserting into db // $add_sq - true to add single quotes, false to just clean // $force_string - true to treat the data as a string // RETURN: properly escaped and db safe data function my_clean($data, $add_sq = true, $force_string = false){ if(is_array($data)){ foreach($data as $key => $val){ $data[$key] = $this->my_clean($val, $add_sq, $force_string); } }else{ if(!is_numeric($data) || $force_string){ if(get_magic_quotes_gpc()){ $data = stripslashes($data); } $data = trim($data); if($add_sq){ $data = "'" . mysql_real_escape_string($data) . "'"; }else{ $data = mysql_real_escape_string($data); } } } return $data; } ?> 2) Use a clean array when passing parameters into the DB: <?php function insertDB($val1, $val2, $val3){ $Clean = Array(); $Clean['Val1'] = my_clean($val1); $Clean['Val2'] = my_clean($val2); $Clean['Val3'] = my_clean($val3); $sql = "INSERT INTO table (fld1, fld2, fld3) VALUES (" . "{$Clean['Val1']}, {$Clean['Val2']}, {$Clean['Val3']})"; $q = mysql_query($sql); // Do error checking and return } ?> 3) I've never done this, but I've toyed with the idea of creating regexps to match the various types of SQL statements. So let's say you're using SELECT, after building your SQL statement you could match it against the regexp for a SELECT and if it fails, don't run the query. Let's say you're creating a delete SQL statement. If the statement is always to look the same, you could create a regexp that matches the statement and prevent a crafty hacker from appending a dreaded OR 1=1 onto the end of you WHERE clause. Just some suggestions.
-
Here's one I made last year when the WoW servers were down. Took about an hour if I remember correctly and I turned my mouse sensitivity way down, which helped a lot. I should try and do one in paint sometime, for shits 'n' giggles.
-
add a query string with the time to the image url
-
What kind of element is txtHint? And are you sure you don't mean: if(xmlHttp.readyState == 4 && xmlHttp.status == 200) I only ask the second question because I'm new to AJAX and that's what the tutorial I read had in it.
-
I arbitrarily decided to use POST for all of my requests; looks like I finally beat the 50/50 odds and saved myself a small headache. Thanks to both of you though, for the extra information.
-
Just don't forget to periodically check your work in IE. A lot of times I'll do something using firebug that works great in FF and then won't work for crap in IE. Then I have to modify it until it works in both. So far I've been able to do it without testing against a specific browser though. Also, I pull out a lot less hair now that I have firebug. If you don't have it, there's another great plug-in called Web Developer or something to that effect. Adds another toolbar with all sorts of handy features.
-
http://www.w3schools.com/ajax/default.asp
-
Solution: Convert string of HTML to DOM node / object.
roopurt18 replied to roopurt18's topic in Javascript Help
Quick update. The function should probably be changed to: [code] // htmlDom_html2dom // html - the html string // Convert a string of html text to a dom object function htmlDom_html2dom(html){ if(html.length > 0){ var obj = document.createElement('div'); if(obj){ obj.innerHTML = html; return obj.firstChild; } } return null; } [/code] Also, keep in mind that it only returns the first child. I had originally wrote this for an HTML string that started with a [i]table[/i] tag. If you tried it with the following HTML string: [code] <div> 1 </div> <div> 2 </div> [/code] You would only get the first div back from the function. Just keep that in mind when using it or tailor it to suit your needs better. -
Darkstar, if you don't currently use it, get Firefox with the Firebug plug-in. I'm still fairly new to Javascript and always found it a pain to figure out which properties and methods were supported by my javascript variables. Firebug is a very useful plug-in with a great javascript debugger. It's made my javascript development so much easier. (EDIT) There are a couple noteworthy items about that function and the original thread has been updated to reflect them.
-
I have an AJAX application that receives strings of HTML from the server to plug into the document. I know there's a big debate between creating DOM objects the "correct" way vs. using innerHTML. I myself prefer to use the "correct" way when possible, but when the server is returning a long string of HTML it's much more convenient to use innerHTML. However, part of my current application is a form and FF has the odd habit of resetting the form when you append to the innerHTML of the form. Rather than write a long sequence of code to save the values and restore them afterwards, I came up with a quick and easy way to convert a string of HTML into a proper DOM object that I could append to the form, thus preventing FF from resetting the user's information. Enjoy: [code] // htmlDom_html2dom // html - the html string // Convert a string of html text to a dom object function htmlDom_html2dom(html){ var obj = null; if(html.length > 0){ obj = document.createElement('div'); obj.innerHTML = html; } return obj.firstChild; } [/code]
-
[SOLVED] Sending variable to 'stateChanged' function
roopurt18 replied to DaveEverFade's topic in Javascript Help
I think this is exactly what zeon just said, but more elaborate. I just got hit with the same problem. The scenario is I have a [i]table[/i] with a [i]select[/i] in it; when the [i]select[/i] is changed it should fetch an appropriate set of data from the server and display it in the sibling [i]td[/i] to the [i]td[/i] that contains the drop down. The catch is this is a dynamic form with multiple, identical [i]select[/i]s that all perform the same action, so using [i]id[/i] attributes is inconvenient. I have a DOM helper function that I wrote that given a node will search for the next sibling of a certain tag type. So the ideal solution would be to have my AJAX callback function take the [i]select[/i] that triggered the event as an input, locate the sibling [i]td[/i], and update the content there. The select control starts like this: [code]<select name="whatever" onChange="selOnChange();">[/code] Here is a basic outline of my original javascript code: [code] // cbDataReady // callback function for when the data is ready from the server function cbDataReady(){ if(xmlHttp.readyState == 4 && xmlHttp.status == 200){ alert(xmlHttp.responseText); } return; } // selOnChange // When this selection control changes, fetch data from the server because it's cool // and looks good on my resume function selOnChange(){ // ajaxRun is an AJAX utility function that takes: // url - url to open // cb - callback function // method - "POST" or "GET" // data - parameter to the send method ajaxRun(path, cbDataReady, "POST", null); return; } [/code] Now, like I said, the ideal solution is to pass in the [i]select[/i] that triggered the onChange to the cbDataReady function. Here is how I accomplished that: Changed the [i]select[/i] HTML to: [code] <select name="whatever" onChange="selOnChange(this);"> [/code] Changed cbDataReady to: [code] // cbDataReady // sel - the select that triggered this callback // callback function for when the data is ready from the server function cbDataReady(sel){ if(xmlHttp.readyState == 4 && xmlHttp.status == 200){ alert(xmlHttp.responseText); alert(sel); } return; } [/code] Changed selOnChange to the following: [code] // selOnChange // sel - the select that triggered the event // When this selection control changes, fetch data from the server because it's cool // and looks good on my resume function selOnChange(sel){ // Build our own callback function var cb = function(){ cbDataReady(sel); } // ajaxRun is an AJAX utility function that takes: // url - url to open // cb - callback function // method - "POST" or "GET" // data - parameter to the send method ajaxRun(path, cb, "POST", null); return; } [/code] I've not thoroughly tested this as of yet, but so far it appears to be working. An alert(sel) in cbDataReady comes up with a select control. That should help anyone hit with this same problem. -
[SOLVED] Personal Programmer Assistant 0.2
roopurt18 replied to Ninjakreborn's topic in Beta Test Your Stuff!
Here's a link: http://dn.codegear.com/article/31863