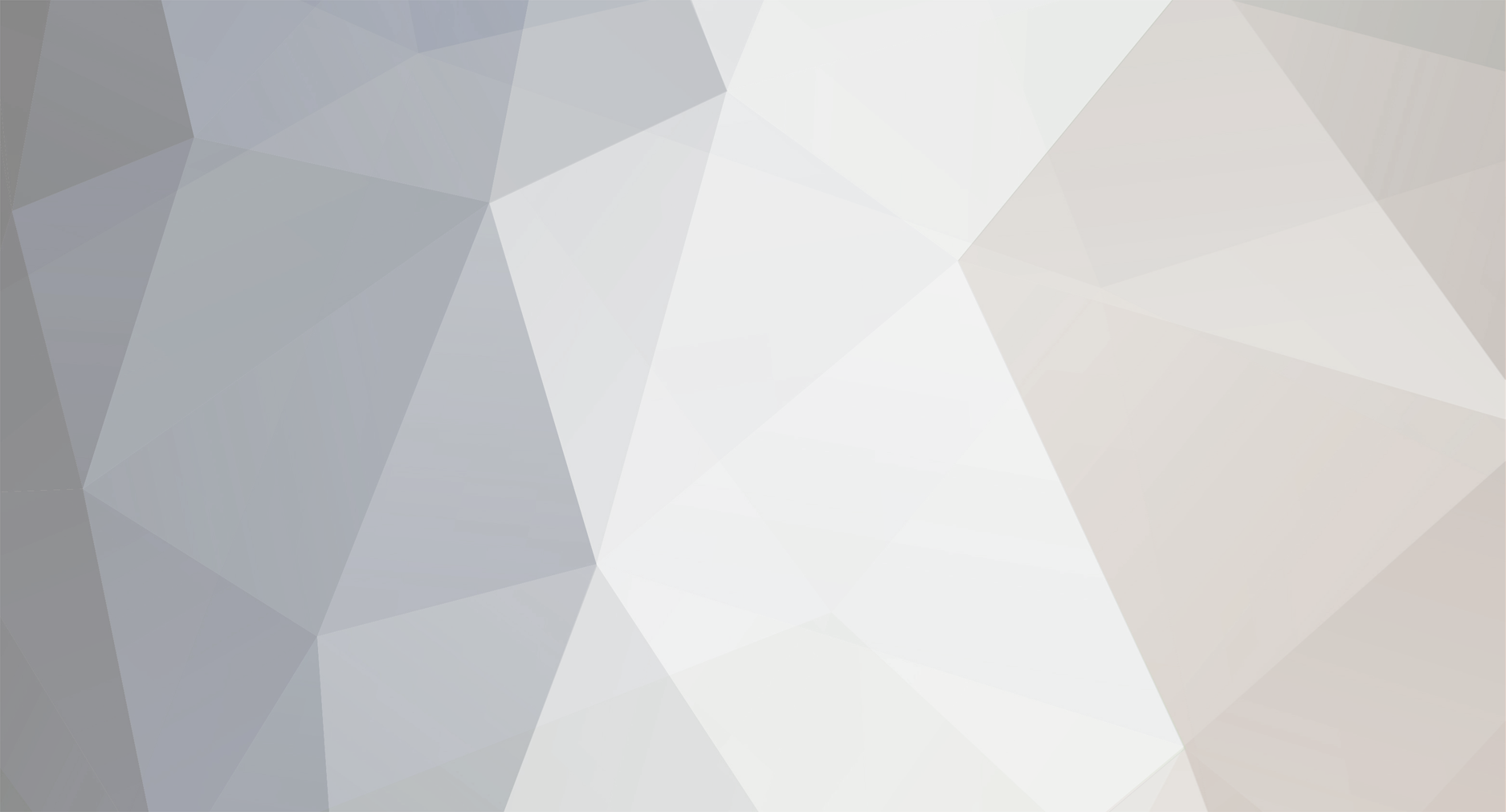
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
[SOLVED] Is this too much for PHP to handle or is it just my code?
roopurt18 replied to hyeteck's topic in PHP Coding Help
There error is in your SQL, not your PHP. Try echo'ing your SQL calls and then find the one that's wrong. -
[SOLVED] Is this too much for PHP to handle or is it just my code?
roopurt18 replied to hyeteck's topic in PHP Coding Help
It's either an error or a time out issue. -
You could always file_get_contents the thank you page and echo that. There's no reason you have to echo plain text; you can echo HTML.
-
You could serialize and store the session in the DB every time the user requests a page as well as a cookie on their system. If they leave and come back you could reload the saved session from their last visit.
-
Try it like this: <?php // Update SQL $sql = "UPDATE ..."; $uq = mysql_query($sql); if(!$uq){ // Query failed, existing record must not exist // Insert SQL $sql = "INSERT INTO ..."; $iq = mysql_query($sql); if(!$iq){ echo "Error: Could not add your item to the cart."; } } ?> Or if you have the proper version of MySQL you can do an INSERT ... UPDATE query.
-
Also, WHERE number = '$number'"; If number is defined as INT, you're comparing it with a string by enclosing $number in single quotes. Use: WHERE number = $number";
-
$list = ".$row["number"]." ; $list = ".$row["first"]."; $list = ".$row["last"]."; echo( $list ); Should be: $list = ".$row['number']." ; $list .= ".$row['first']."; $list .= ".$row['last']."; echo( $list ); Take a good look at the use of .= instead of = on the 2nd and 3rd lines. Also take a good look at my use of the single quotes within the double-quoted strings.
-
$sql = "SELECT * FROM information `number`, `first`, `last` WHERE number = '$number'"; Unless I'm totally missing something, that query is all kinds of wrong. $sql = "SELECT `number`, `first`, `last` FROM information WHERE number = '$number'"; Also, the fine point that you're missing here is that $row will be an associative array of the values returned by the DB. Each key in the array will correlate to one of your DB rows. In the while loop where $row is set, try this: echo "<pre style=\"text-align: left;\">" . print_r($row, true) . "</pre>";
-
Use sessions for data you wish to store while the user is browsing your site. Use cookies for data you wish to preserve between user visits. Limit the amount of information you store in each to the bare minimum; it should only be enough to identify the user and pull data about them from the DB. My $.02 on the internal argument: sessions are not cookies, although they perform much of the same task. If a session was a cookie, there wouldn't be a sessions vs. cookie discussion IMO.
-
Masking URL without Mod_ReWrite in PHP
roopurt18 replied to phpBeginner06's topic in PHP Coding Help
Try e-mailing the support department of your host? -
Masking URL without Mod_ReWrite in PHP
roopurt18 replied to phpBeginner06's topic in PHP Coding Help
Well, the .htaccess file will only show up in your file manager if it exists. Try creating one and running some initial tests. -
"(Something like querying the db everytime a page is visited to make sure that the userid and auth level match? *gonna do that now actually*)" ^ Good idea. I'd create a unique hash when a user logs in and store that in their row of the users table. Then in the auto-login cookie I'd store the username and the unique hash. That way, the person who has the cookie can only log in as themselves.
-
Use sessions because they are better suited for what you want to do and easier to manipulate. Reserve cookies for when you want to save information between browser sessions, such as to provide automatic login on the next visit to the page (but of course, don't save the users login information in the cookie).
-
Masking URL without Mod_ReWrite in PHP
roopurt18 replied to phpBeginner06's topic in PHP Coding Help
I would have to say yes, I do think it's possible. Whatever path you choose has to point to a valid script and within that script you can just use $_SERVER['REQUEST_URI'] to get the full requested URI. You'd end up with URLs that look like this though: http://domain/some/path/script.php/param1/param2/param3 I've never tried it so I could be wrong though. (EDIT) NM. The server will just think script.php is a directory and give you a 404. You'd need to use something like http://domain/some/path/script.php?param1/param2/param3 -
<?php $result = mysql_query("SELECT * FROM `CATS` WHERE PING = '1'") or die(mysql_error()); $count=0; $PING = Array(); while ($row = mysql_fetch_array($result)) { $PING[] = $row[cat_name]; if($count % 3 == 0) { echo implode( ' | ', $PING) . "<br />"; $PING = Array(); } $count++; } echo implode( ' | ', $PING) . "<br />"; ?>
-
I responded to your other thread in the PHP Help area.
-
Perhaps if you told us what the error was? My guess is you want to do: SELECT DISTINCT employee.itemNo, employee.itemDesc, employee.date, employee.outlet, stockmovement.barcode, stockmovement.quantity, stockmovement.date FROM employee, stockmovement WHERE employee.itemNo = stockmovement.barcode AND MONTH(stockmovement.date) = $month_number GROUP BY employee.itemNo Where $month_number is an integer between 1 and 12, inclusive. @8ball, You have an error in your MySQL query. Check the manual for the correct syntax to use near "WHERE employee.itemNo = stockmovement.barcode, GROUP BY" ;^]
-
$outs = Array(); while($row = mysql_fetch_array($result)){ $outs[] = $row[PING_NAME]; } echo implode( ' | ' , $outs);
-
It makes the Email column in the table unique, which means you can't have two rows in the table with the same Email address.
-
Just to throw one more wrench into things, I wouldn't really consider it secure or good practice to have the user, password, and cookie name hard coded like that. A much better approach would be to define those elsewhere in a small .php file with 3 define statements. Then you can create a cron job on the server that runs once a month or whatever and changes them to random values. That way if you lay someone off within your company, you can run the script on the server and you have new debugging information. You set it up to run automatically just in case an outside source happens to discover them, that would (hopefully) limit the window of time they could use it to do anything to your system.
-
Learn how to make a column unique within the database itself. CREATE TABLE users( ... Email VARCHAR(255) NOT NULL DEFAULT '', ... UNIQUE(Email) ) Now when you try and insert a duplicate user with the same e-mail address, the database will refuse to do so. So in your script, if the INSERT statement fails you know the user wasn't registered. Note that a VARCHAR(255) is not necessarily sufficient for all possible e-mails, I just used that column for simplicity here.
-
BakeDebugCookie.php <?php // BakeDebugCookie.php // Create a debugging cookie that enables developers to enter the // site when it's down, or areas of the site not open to the general // public. $user = 'username'; $pass = 'password'; if(count($_POST)){ // Form submitted $gooduser = isset($_POST['user']) && !strcmp($_POST['user'], $user); $goodpass = isset($_POST['pass']) && !strcmp($_POST['pass'], $pass); if($gooduser && $goodpass){ setcookie('DebugCookie', 'On', time() + 60 * 60 * 24 * 14); $form = "Cookie set"; }else{ $form = "Try again, suckafoo."; } }else{ // Display form $form = <<<FORM <form action="" method="post"> User: <input type="text" name="user" value="" /> Password: <input type="password" name="pass" value="" /> <input type="submit" value="Submit" /> </form> FORM; } echo $form; ?> EatDebugCookie.php <?php // EatDebugCookie.php // Delete the debug cookie setcookie('DebugCookie', 'On', time()); ?> MyDebug.php <?php // MyDebug.php // Define simple debugging stuff // isDebug // RETURN: true if debug is on, false if off function isDebug(){ return isset($_COOKIE['DebugCookie']) && $_COOKIE['DebugCookie'] == 'On'; } ?> Then in your scripts, you just check if the site is down. If the site is down and the user doesn't have the debug cookie ( !isDebug() ), they get redirected. Just make sure you write your logic in a way that if the site isn't down they still don't get redirected.
-
I tend to write my SQL queries like so: $sql = "SELECT " . "fld1, fld2, fld3 " . "BIG_FUNCTION(fld4, param1, param2) AS OutFld " . "FROM table1, table2, table3, table4 " . "WHERE " etc. Works well except sometimes I spend forever trying to debug a query only to realize I forgot a space between my last table name and FROM. Argh!
-
If your IP address changes periodically that won't be very convenient. Why not set up a debug cookie?
-
I recommend storing dates as a DATETIME or DATE column type, which makes other manipulations with them easier in the long run.