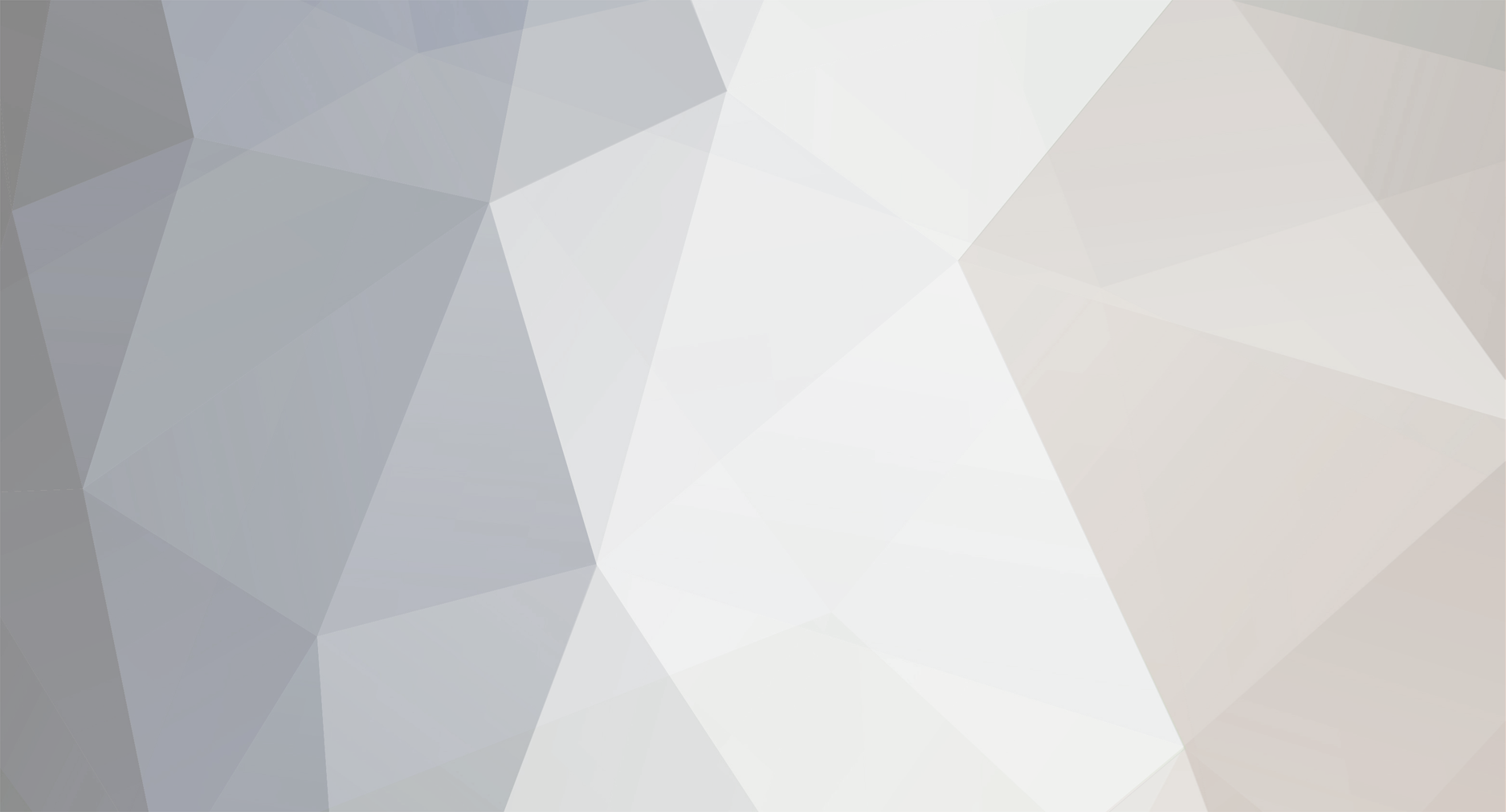
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
[code]echo "<a href = "recipes.php?id={$row['Recipe_Number']}">{$row['Recipe Name']}</a>";[/code] should be [code]echo "<a href=\"recipes.php?id={$row['Recipe_Number']}\">{$row['Recipe Name']}</a>";[/code]
-
Often times we get caught up doing things in the most efficient manner possible, but with a result set that small you could loop over it 4 or 5 times without dramatically reducing performance IMO. So it might be possible to come up with a working, albeit messy, solution if you really had to. Here is a little something I threw together that might help you out though: [code]<?php // test.php /* We have two tables like so: pages: page_id, parent_id, page_name, page_contents, order_num categories: cat_id, parent_id, cat_name, order_num ** We want a query to pull from each table and combine the results like so: page_id | page_name | cat_id | cat_name | order_num | 10 page10 1 15 cat10 2 11 page11 3 12 page12 4 16 cat_11 5 ** ** If I understand you correctly, a page can be in a category but so can ** a category. This would mean that, ideally, we'd want to list the cat_name ** for the rows coming out of the categories table. I'm going on the ** assumption that in each table, parent_id is a possible but not required ** relation to a cat_id in the categories table. ** ** I don't know of a way to do this through just queries in SQL, so we will ** use two queries to pull a result set from each table and then combine ** those in PHP. ** ** I'm also working on the assumption that a value for order_num in one table ** is not repeated in the other table. I.E. that if you have a 2 for ** order_num in pages, there is not a 2 for order_num in categories. */ $Final = Array(); // Init our final array /* First we pull information from the pages table. For this query we are ** going to left join with categories so that our returned rows will have ** a cat_name where there is a parent ID. We use LEFT JOIN because I'm ** assuming not all pages will have a category. */ $sql = "SELECT " . "p.page_id AS PageID, " . "p.page_name AS PageName, " . "c.cat_id AS CatID, " . "c.cat_name AS CatName, " . "c.cat_name AS ParentName, " // This will be more apparent in the next // sql statement, hopefully. . "p.order_num AS OrderNum " . "FROM pages p " . "LEFT JOIN categories c ON p.parent_id=c.cat_id " // If you have a WHERE, add it here . "ORDER BY p.order_num"; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_array($q)){ // Keep in mind this assignment assumes OrderNum is unique across tables $Final[$row['OrderNum']] = $row; } } /* Now we pull information from our categories table. For this query we are ** going to left join the table on itself in case any of the categories belong ** to a category. */ $sql = "SELECT " . "NULL AS PageID, " . "NULL AS PageName, " . "c1.cat_id AS CatID, " . "c1.cat_name AS CatName, " . "c2.cat_name AS ParentName, " . "c1.order_num AS OrderNum " . "FROM categories c1 " . "LEFT JOIN categories c2 ON c1.parent_id=c2.cat_id " // Insert your WHERE clause here . "ORDER BY c1.order_num"; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_array($q)){ // Keep in mind this assignment assumes OrderNum is unique across tables $Final[$row['OrderNum']] = $row; } } // Output what we have so far: echo "<pre style=\"text-align: left;\">" . print_r($Final, true) . "</pre>"; ?>[/code] Hope it helps.
-
Just curious, how large are the sets you're working with?
-
I'd like to query for the currently used database.
-
Disabling a user's ability to submit a form based on the date
roopurt18 replied to sws's topic in PHP Coding Help
First off, I'm going to assume your users have a UserID. I'm also going to assume the possible selections also have an ID, I'll call it PickID. I would use a table structure like this: table: [b]PickTrack[/b] TrackID INT AUTO INC etc UserID INT EnterTime DATETIME table: [b]PickTrackItems[/b] TrackID INT PickID INT UNIQUE(TrackID, PickID) When a user makes their selections, you make a single entry in [b]PickTrack[/b] with the times that the selection was made. You then enter all of the selected picks into [b]PickTrackItems[/b] and attach them to the single entry from the previous table. When a user visits this page, you can calculate the timestamp of the exact cutoff date for this week as well as one week ago. If no entry in [b]PickTrack[/b] between those dates exists for the user you can display the form. -
array not coming together (sql construction)
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
INT and VARCHAR columns do not sort the same. Since you are specifically writing this bit of code for sorting you might want to choose a datatype that sorts correctly for the type of data you will most likely be giving it. -
If its a MySQL function then yes. That doesn't mean there isn't an equivalent PHP function though, which I'm unsure of.
-
array not coming together (sql construction)
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
I noticed you said your order column for the images was a VARCHAR, I highly recommend changing this to INT or you'll have problems later. -
array not coming together (sql construction)
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
"I had forgotten foreach needed a key, because for some reason, lalely without the extra part, all the other things I was trying to do with an array, worked with foreach, just using foreach ($array as $val) or whatever, they were working exactly as expected, so I had forgotten that was needed." That's just it. It's [b]not always[/b] needed. Whether or not you include a $key value in your foreach is dependent on what you're trying to accomplish. -
array not coming together (sql construction)
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
Anothing thing: [code]$order = array($_POST['order']); $update = array(); foreach ($id as $var1) { $update[$var1] = "UPDATE oak_images SET order = '" . $order . "' WHERE id = '" . $var1 . "';"; } [/code] You declare $order as an array and then use it in your sql statement without providing a key. Your sql is always going to be: UPDATE oak_images SET order = '[b]array[/b]' WHERE id = 'array' You're trying to step through two arrays with a foreach. The only way that's possible is if both arrays have the same keys for the corresponding values. The fact that you don't even have a key variable being set in your foreach tells me, loud and clear, you have no idea what you're doing. Create a new file, and stick this in it: [code] <?php $arr1 = Array( 1, 2, 3, 4 ); $arr2 = Array( 'cat', 'dogs', 'horses', 'fish' ); foreach($arr1 as $key => $val){ echo "I have {$arr1[$key]} {$arr2[$key]}<br />"; } ?>[/code] -
array not coming together (sql construction)
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
I'm guessing that you're intending for your ID and Order arrays to be arrays of single valued entities and not arrays of arrays. The problem is that the ID and Order arrays themselves contain arrays. [code]foreach ($id as $var1) { $update[$var1] = "UPDATE oak_images SET order = '" . $order . "' WHERE id = '" . $var1 . "';"; }[/code] The fact that $id contains arrays means that $var1 [b]is[/b] an array. The error you're getting is stating that your offset into the $update array is illegal, in this case it's an array. Your code is essentially doing this, and it's illegal: $update[Array(1,2,3,4)] = "some sql statement"; -
corbin, what if your user is intentionally trying to add a double back slash?
-
Wasn't sure where to place this topic, but when I select [b]View -> Source[/b] from IE6 SP2 nothing happens. It only occurs on the PHP generate pages from my current site and only on some of them. Also, it appears to only affect pages that are visible after logging in. Any ideas?
-
Are you saying you're trying to prevent duplicate entries in your MySQL table? If so, why not just use a UNIQUEness constraint on the table?
-
http://dev.mysql.com/doc/refman/4.1/en/date-and-time-functions.html DATE([i]expr[/i]) SELECT DATE('2003-12-31 01:02:03') So if you have a table [i]the_table[/i] and the DATETIME column is named [i]the_date[/i]: SELECT *, DATE(the_date) AS the_date_disp FROM the_table WHERE 1 Also, if you want to format the date like you can with PHP's date function, you'll want to look up DATE_FORMAT in MySQL. SELECT * DATE_FORMAT(the_date, '%a. %b. %D, %Y') AS the_date_disp FROM the_table WHERE 1 Hope that helps.
-
Alternatively you can tell MySQL to return only that part of the field and avoid modifying it in PHP altogether.
-
Well, sessions are used to store values across pages transparently to the user. If you already have values in the session, there is no need to include them in a form in a hidden field. Now if you wanted to give the user a chance to change those values with the form, it'd be a different matter altogether.
-
When the form is submitted it will post to a specific page in your site. You just have that page do the two queries separately in succession.
-
[quote author=kenrbnsn link=topic=116597.msg475136#msg475136 date=1164733632] But why are you using hidden fields when the values are already in the SESSION? [/quote] Good question.
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
What exactly are you asking? :D -
I don't know what you guys are complaining about, I always look forward to his posts. Array indexes start at [b]zero[/b]. This means they end at [b]count($array) - 1[/b]. [code] <?php // build query $names = array("name", "t_number", "flighttype", "aircrafttype", "aircraftnumber", "eventdate", "eventtime", "flightnumber", "flightnumberselect", "scheduleddepartureairport", "scheduledarrivalairport", "captain", "firstofficer", "flightattendant", "additionalcrewmembers", "weather", "flightplan", "pax", "lapchildren", "actiontaken_abortedtakeoff", "actiontaken_canceledflight", "actiontaken_declaredemergency", "actiontaken_delayedflight", "actiontaken_diversion", "actiontaken_goaround", "actiontaken_groundaircraftatoutstation", "actiontaken_inflightshutdown", "actiontaken_missedapproach", "actiontaken_returnaircrafttomaintenance", "actiontaken_returntogate", "actiontaken_turnback", "phaseofflight", "classification_aircraftdamage", "classification_aircraftonrunway", "classification_aircraftlogsmanualsorphase", "classification_airportclosure", "classification_alternatorfailure", "classification_atcdelay", "classification_avionicsfailure_communication", "classification_avionicsfailure_navigation", "classification_battery", "classification_birdstrike", "classification_brakefailure", "classification_collision", "classification_crewincapacitation", "classification_debrisonrunway", "classification_deviationofatcinstructions", "classification_disruptiveorsickpassenger", "classification_doorwarning", "classification_doorwarningwhichone", "classification_dooropening", "classification_dooropeningwhichone", "classification_electricalfailure", "classification_emergencyevacuation", "classification_enginefailure", "classification_enginefire", "classification_fireorsmokeincabin", "classification_flapfailure", "classification_flattire", "classification_flightcontrolfailure", "classification_fuelleak", "classification_fuelquantity", "classification_hardlanding", "classification_icing", "classification_flightinstrument", "classification_flightinstrumentwhich", "classification_engineinstrument", "classification_engineinstrumentwhich", "classification_landinggear_blowdown", "classification_landinggear_failtoextend", "classification_landinggear_failtoretract", "classification_landinggear_hydraulicleak", "classification_landinggear_hydraulicsystemfailure", "classification_landinggear_falseindication", "classification_lighteningstrike", "classification_magnetofailedorrough", "classification_nearmidaircollision", "classification_paxinjury", "classification_propstrike", "classification_roughengine", "classification_runwayincursion", "classification_starterfailureorwarning", "classification_tailstrike", "classification_turbulence", "classification_vacuumfailure", "classification_waketurbulence", "classification_weatherbelowminimums", "classification_weatherbelowhighminimums", "classification_weightandbalance", "classification_windshear", "classification_other", "classification_othertext", "description"); $values = mysql_real_escape_string($_POST['search1field']); $values = "'%{$values}%'"; $select = "SELECT * FROM eventreport WHERE "; for ($i=0;$i<=count($names) - 1;$i++) { // - 1 // if ($i == 94) { // <-- WHY DO YOU INSERT RANDOM INTEGERS INTO YOUR CODE? if($i == count($names) - 1){ $select .= $names[$i] . " LIKE " . $values; } else { $select .= $names[$i] . " LIKE " . $values . " OR "; } } $select .= "LIMIT $rownumber, $limit;"; // end building query ?>[/code] I already told you once about inserting random integers into your tests when you were doing your form validation, which I assume is still broken. /popcorn
-
You can leave the parentheses off of statements like this: $Keyword =($row['Keyword']); You must always put $ in front of variable names. When posting your code, can you please enclose it inside of [ code ] [ / code ] tags, but without the spaces in the tags.
-
It stands for whatever alias you gave another of your tables in the SQL statement; in this case it'd be either m or b. I have no idea how you're matching the data in the third table with whats in the other two, so I don't know which table or field to use.