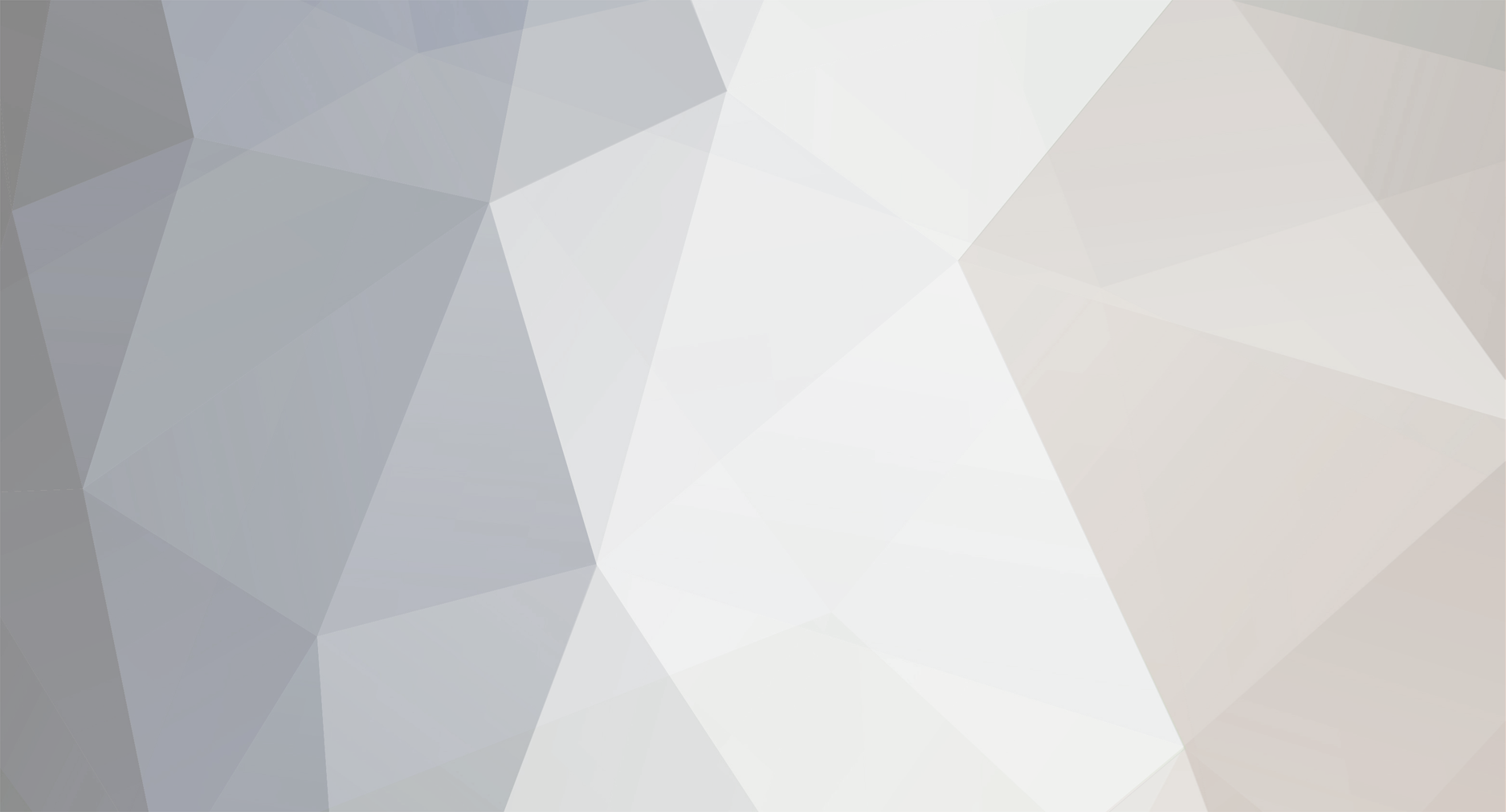
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Look up DATE_FORMAT in the MySQL documentation, not the PHP documentation.
-
Backup / Restore MySQL Databases with PHP
roopurt18 replied to tawevolution's topic in PHP Coding Help
Well it copies it. So the original data is left intact. The main difference between the two approaches is mine uses shell commands. To do it strictly through PHP requires more queries and a little more programming effort on your part. Also, I'm not sure if the SELECT INTO OUTFILE copies table structure or not, but I know mine does. That may be something worth considering as well. -
Backup / Restore MySQL Databases with PHP
roopurt18 replied to tawevolution's topic in PHP Coding Help
Here is a script I used to move [i]every[/i] database on our old host to our new webhost. I'm sure you could modify the code to do what you wanted. [code]<?php // MoveDBs.php // Automated PHP script to move the databases from our old host // to our new host. $new_host = ''; $new_user = ''; $new_pass = ''; $Imported = Array(); $old_host = ''; $old_user = ''; $old_pass = ''; $DBs = Array(); set_time_limit(0); // Delete all current *.sql files $cmd = sprintf('rm ~/host_move/*.sql'); echo "\n" . $cmd . "\n"; system($cmd); // We need to retrieve the name of all our databases // from the old host mysql_connect($old_host, $old_user, $old_pass) or die("Error: Could not connect to database."); $q = mysql_query("SHOW DATABASES"); if($q){ while($row = mysql_fetch_array($q)){ $DBs[] = $row['Database']; } } mysql_close(); if(true){ // Set false to skip the import // Dump each database if(count($DBs)){ foreach($DBs as $DB){ // Dump a .sql for each database $cmd = sprintf('mysqldump --opt -h %s -u %s -p%s --databases %s > %s.sql', $old_host, $old_user, $old_pass, $DB, $DB ); echo "\n" . $cmd . "\n"; system($cmd); } } } // Now we need to open all of our .sql files and import // into our new database if(count($DBs)){ foreach($DBs as $DB){ // Check that the file exists if(file_exists($DB . '.sql')){ $Imported[$DB]['Msgs'] = "{$DB}.sql - File found."; $cmd = sprintf('mysql -h %s -u %s -p%s < %s.sql', $new_host, $new_user, $new_pass, $DB ); echo "\n{$cmd}\n"; system($cmd); }else{ $Imported[$DB]['Msgs'] = "ERROR - {$DB}.sql - File DNE."; $Imported[$DB]['Errors'] = "{$DB}.sql - File DNE."; } } }else{ echo "\nNo imported databases exist.\n"; } print_r($Imported); ?>[/code] -
I would recommend reading the documentation on preg_replace and then googling for regular expressions. Then try and code something and come back when you run into difficulty.
-
This sounds like something you'd be better off using a SQL join for, but I could be wrong. If you're aware of joins and how they work, nevermind. If you're not, why don't you tell us a little about your database or show us the two relevant queries.
-
Not to be mean, but it'd take about as long to create a form with a type="file" input and test it yourself as it did for you to ask if it's what you wanted. ;^]
-
You should keep in mind that valid XML requires attributes to be placed in quotes.
-
[code] while($myrow = mysql_fetch_assoc($mysql_result)) $file = $myrow['file']; $name = $myrow['name']; { $xml .= '<song name='.$name.' file='.$file.'>'. NEWLINE; } [/code] Your while loop is effectively: [code] while($myrow = mysql_fetch_assoc($mysql_result)) $file = $myrow['file']; [/code] Try this: [code] while($myrow = mysql_fetch_assoc($mysql_result)){ $file = $myrow['file']; $name = $myrow['name']; $xml .= '<song name='.$name.' file='.$file.'>'. NEWLINE; } [/code]
-
If your web accessible folder is public_html, then a non-accessible folder is anything outside of that folder. These are [b]not[/b] web accessible: ./uploadedfiles ./tmp ./hi2u These [b]are[/b] web accessible: ./public_html/uploadedfiles ./public_html/tmp ./public_html/hi2u Notice a difference?
-
lol Follow and read the link AndyB provided.
-
All I can tell you is when I used the line: [code] $files=explode("\n'-------------------------------[file]--------------------------------\n",$contents);[/code] it didn't explode properly. Removing the \n from the first parameter to explode did explode properly. Take a look at the note in the manual for fopen: http://us3.php.net/fopen
-
Just a general question on explode() [ANSWERED]
roopurt18 replied to ted_chou12's topic in PHP Coding Help
explode returns an array, not a string. -
$num=count($contents); should most certainly be $num=count($files);
-
A couple issues. First off, on windows a newline is \n\r or \r\n, forgot the order, so your explodes are not working properly. Remove the \n and trim the results. Secondly, the line: [code=php:0]$global_metadata=explode("\n",$contents[0]);[/code] is exploding on $contents[0], which is just a single character. So you're not going to get anything useful out of it. Perhaps you meant $files[0]? I'll post more after I play with it some more.
-
Does this work on any form of *nix based host?
-
Oops, I made a mistake. Don't enclose it in single quotes.
-
You should keep in mind that the server is not always in the same time zone as you and sometimes the mysql server is not the same machine as your apache server. For instance on the old web host we used at work, if I used date() from within PHP I got a time on the east coast of the United States. If I used NOW() in MySQL I got a time a few hours ahead of that. What I recommend is using a statement like: [code]SELECT DATE_FORMAT(NOW(), 'H:i:s') FROM table WHERE 1 LIMIT 1[/code] That will give you the time of the MySQL server. Let's say that time is 3 hours ahead of where you are, then you can add a line: [code=php:0]define('DB_NOW', '(NOW() - 3 * 60 * 60)');[/code] to your general DB.php file. Now whenever you need to insert the current DATETIME into a table you can just do: [code=php:0]$sql = "INSERT INTO table (fld1, fld2, the_date) VALUES (val1, 'val2', '" . DB_NOW . "')";[/code] If you ever move servers, you only need to adjust a single line of code to compensate for the new server's possible timezone difference than the old one.
-
Use an array?
-
Let the database perform your task for your: http://dev.mysql.com/doc/refman/5.0/en/string-functions.html Scroll down to SUBSTRING()
-
You should specify the [i]method[/i] attribute of your form to be [i]post[/i]. Here's a simple example: [code] <html> <body> <?php $form = '<form name="myform" action="" method="post">' . '<select name="mysel">' . '<option value="1">1</option>' . '<option value="2">2</option>' . '<option value="3">3</option>' . '</select>' . '<input type="submit" name="filter" value="Filter" />'; // Check if the filter button was pressed, if so we can display more fields if(count($_POST) && isset($_POST['filter'])){ $form .= '<select name="anothersel">' . '</select>'; } $form .= '<input type="submit" name="submit" value="Submit" />'; echo $form; ?> </body></html>[/code]
-
Just get the first character, build a regexp out of it, and look for any non-matching chars.
-
Have you gone through and looked at the contents of the directory where you were moving uploaded files? Chances are the attackers uploaded a multitude of PHP scripts and are only now starting to execute them through their browser. As far as filtering data is concerned, there is one rule to follow: Always, always, always.
-
If you're sticking with strictly PHP there is no way to know what they've selected in the first box [i]until[/i] they've submitted the form. Here is what you're looking at: 1) Display first drop down box on a form with a submit button 2) User makes selection and submits form 3) Take the user's selection and redisplay the page but add the new drop down box to it. PHP lives and runs only on the server, never on the client. If you want things to update / refresh / occur [i]without[/i] submitting and reloading the page, your solution lies in the domain of Javascript.
-
IMO you can't assume 1) that a file has an extension 2) that a file doesn't have more than one dot in it Here's what I would use: [code]<?php function filebits($file){ $bits = Array( 'name' => NULL, 'ext' => NULL ); if(strlen($file)){ $file = explode(".", $file); $bits['name'] = $file[0]; $bits['ext'] = ($num = count($file)) > 1 ? $file[$num - 1] : NULL; } return $bits; } ?>[/code]
-
Even though you set the time out to 0, I believe that has no bearing on database interactions. Do you have access to a CLI so you can test your cron jobs that way? It will make your life a little easier.