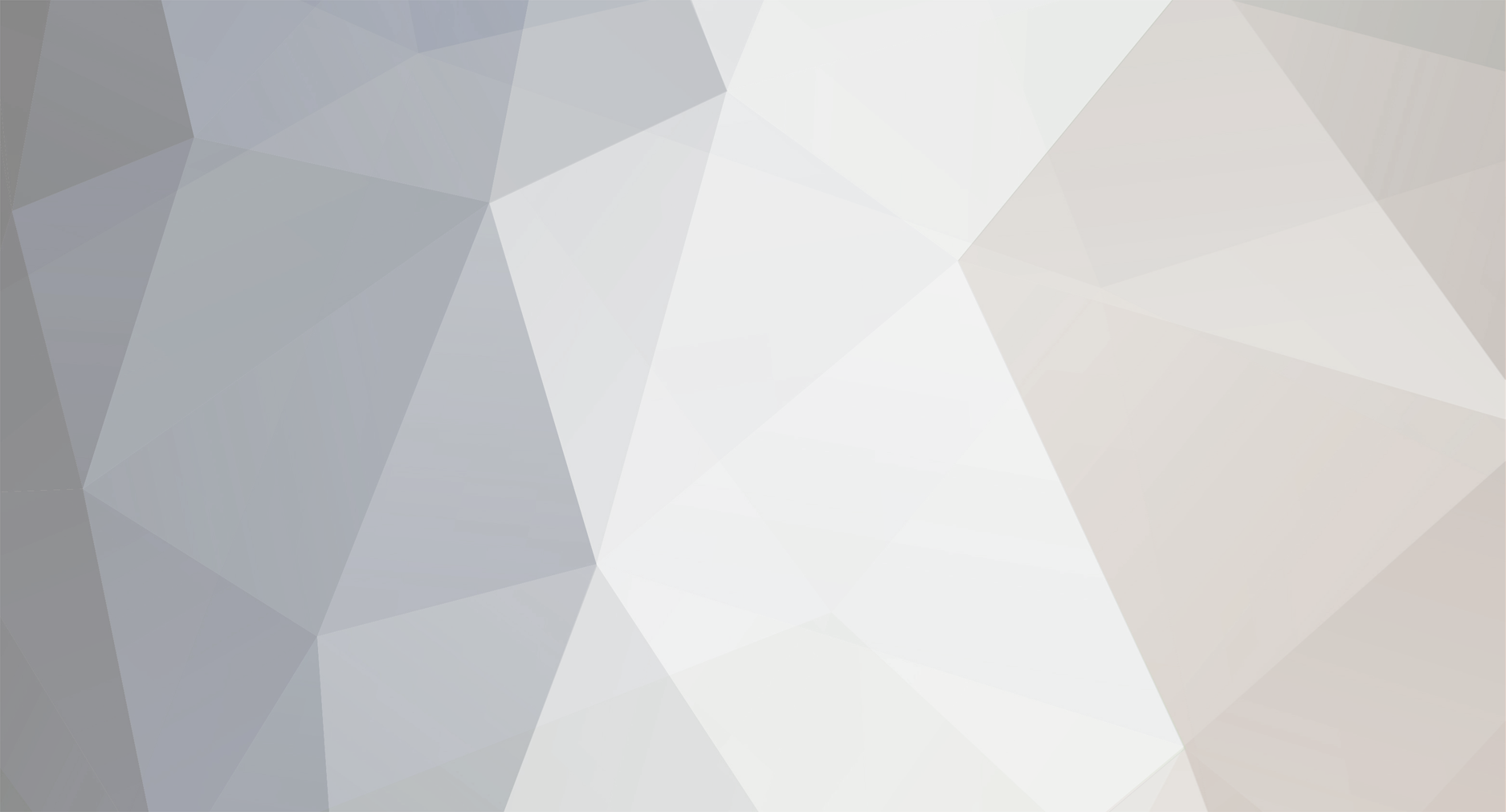
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
If you want to know which is most popular, slap: ORDER BY '%' DESC LIMIT 1 onto the end of your query.
-
The method proposed by thorpe would work if implemented correctly. What exactly are you having trouble with?
-
So put the lookup table into a database.
-
table {field1, field2, field3, ...} elements {id, name, order, ...} Let them designate the order as an integer, but when you display the items don't display the order thats in the table, display an order from a counter variable. SELECT * FROM elements WHERE 1 ORDER BY order; <?php $q = mysql_query($sql); if($q){ $count = 1; while($row = mysql_fetch_assoc($q)){ echo $count++ . ": " . $row['name']; } } ?>
-
You might want to consider a different way of passing the language around from page to page, possibly with sessions. It's a little bit cleaner. Secondly, you have three sets of information which could potentially need to be in multiple languages. There's hard coded content, such as an actual .html file displayed by the site. For these you'll want to separate the files into directories where the base directory defines the language. i.e: en/home.html <- english sp/home.html <- spanish du/home.html <- dutch There's program output, which is basically strings of text in response to events. For these you'll want to use a string look up table: $lookup['en']['error'] = "Sorry, an error has occurred processing your request."; $lookup['sp']['error'] = "actual spanish"; Then there's potential database data. Not sure how to deal with that. I'd look up programming and internationalization through google.
-
I've read many, many programming books; I literally have shelves full of them. I've found the "Teach Yourself XYZ in " 21 days, 24 hours, 14 hour type books are actually full of good information and a good way to get off the ground quickly. If you're looking for a little more detail, I find the O'Reilly published books are a very good resource. After that google and these types of message boards are the best bet. I'd go down to your local, large book retailer and look at all of the available PHP books to start with. Pick up a couple, read some of each of them, and buy the one that's written in a way you can understand. Books are expensive so buy one at a time IMO. When you get most of the way through the first one, go back and look at books dedicated to MySQL. Not just a book that talks about using MySQL and PHP together, but one that will go into detail about the different features of MySQL itself. What types of common tasks you can accomplish with simple database queries, why choosing a good data type for a column is important, how to analyze your queries, make better indeces, etc. The most efficient PHP website will drop to its knees if the foundation is a poorly designed database. It's nice that a lot of free information is available on the web, but I still feel the best resource is a good book.
-
sort multidimentional array (or other best options)
roopurt18 replied to flash gordon's topic in PHP Coding Help
You should not be storing dates as separate fields in the first place. There's no sense in: CREATE TABLE table_one( month INT, day INT, year INT ) when this will do just fine: CREATE TABLE table_two( dateDT DATETIME ) Pick up a book on MySQL. You'll save yourself so much headache. -
You need to pull the data from the contacts table using a SELECT query. You then loop over the results and use each result to create an < option> item in a < select> input. This is the bare minimum you'd need to do and it makes some assumptions, such that DB_HOST, DB_USER, and DB_PASS are all defined as well as assumptions about your database table. <?php mysql_connect(DB_HOST, DB_USER, DB_PASS); $sql = "SELECT * FROM contacts WHERE 1"; $q = mysql_query($sql); if($q){ echo "<select>"; while($row = mysql_fetch_assoc($q)){ echo "<option value=\"{$row['fld1']}\">{$row['fld2']}</option>"; } echo "</select>"; } ?>
-
I recommend picking up a book on MySQL that goes more in depth on table design, MySQL features, etc. than a book that talks about MySQL and PHP. The very first database driven website I wrote didn't have a single table join in it because I didn't even know they existed, which I still get a good laugh out of. There's also a lot of common tasks you'll try and accomplish in PHP code that you can do more easily in MySQL; in other words, the amount of "programming" code you can move into database logic is astonishing and something every programmer should be aware of. The only reason I say this is because of this statement: "Since clans will have multiple members use the leaders id that registered it?" We all need to start somewhere, but a good book should help answer 95% of the initial questions such as those. However, if you don't want to buy a book, I suggest something like the following: table {field1, field2, field3, ...} users {id, login, password, ...} clans {id, name, ...} clan_member_ranks {id, name, ...} clan_members {clan_id, member_id, rank_id, ...}
-
When trying to trouble shoot a blank page, here's what I do. At the very top of the script before anything: echo "hi2u"; exit(); If I reload the page and don't see hi2u, 99% sure its a syntax error. If I do see hi2u, just cut the echo line, move down a small chunk, and re-paste it. Do this until you narrow down the one line that is causing it to blow up. You'll often find is a bad file path, undeclared function, etc.
-
You know how some people just don't get math...
-
http://www.php.net/setcookie setcookie() defines a cookie to be sent along with the rest of the HTTP headers. Like other headers, cookies must be sent before any output from your script (this is a protocol restriction).
-
While I'm quite proud of my little concoction of SQL that I just came up with, after reading effigy's post I think something like (edit - nm, I'll have to play with it more when I have free time.) is a lot easier to read and use.
-
Got one more for you: Where: fld is the character field len is the length of the string you wish to return SELECT TRIM( REVERSE( SUBSTRING( REVERSE( SUBSTRING( fld, 1, len ) ), LOCATE( ' ', REVERSE( SUBSTRING( fld, 1, len ) ) ) ) ) ) AS SmallField FROM table WHERE 1 LIMIT 1 <?php // Sample use of the function below $sql = "SELECT id, name, " . makeShortField('description', 30, 'ShortDesc') . " FROM someTable WHERE 1 LIMIT 1"; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ echo '<pre style="text-align: left;">' . print_r($row, true) . '</pre>'; } } // makeShortField // $field - the name of the db field // $len - the maximum number of characters from $field to display // $name - the name you'd like the field to be returned as in the query // Create the string of text that will extract a limited number of characters // from a DB field and cut off any impartial text // i.e: makeShortField('field1', 15, 'ShortDesc'); // On a DB field containing 'abcd efgh ijkl mnop qrst uvwx yz' // Will make $row['ShortDesc'] contain: 'abcd efgh ijkl' // RETURN: the text string to insert into your DB query function makeShortField($field, $len, $name){ $query = <<<QUERY SELECT TRIM( REVERSE( SUBSTRING( REVERSE(SUBSTRING($field,1,len)), LOCATE(' ',REVERSE(SUBSTRING($field,1,len))) ) ) ) AS $name QUERY; } ?>
-
Perhaps if you told us something of the file we could guide you better.
-
Nevermind, don't think that was it.
-
Sorry. The CSS in my previous post was from the link provided by obsidian about the clearfix method. Anyways, the only point I intended to ever make with this whole discussion was that we're all in the same boat here. We'd all like the layout to be separate from the data. We're on our way there, but we haven't quite made it yet.
-
.clearfix:after { content: "."; display: block; height: 0; clear: both; visibility: hidden; } .clearfix {display: inline-block;} /* Hides from IE-mac \*/ * html .clearfix {height: 1%;} .clearfix {display: block;} /* End hide from IE-mac */ So we "save," in the sense of saving from danger, our document layout at the expense of our CSS due to browser inconsistencies. There is also the final warning of, "Just watch out for previous external floats triggering the IE Float Model, as mentioned earlier;" so you may still wind up bit in the ass. I consider myself a fairly intelligent and savvy guy, but you are right. When it comes to floating CSS elements, I'm not in the know. I've done table-less sites in the past and I did get them to work. But every time I set out to find the new, "best" multi-column layout method, something's changed. Here's the way I see it. Sure, tables are fine for tabular data and not layouts, as jesirose mentioned. But I could very well argue that a 3 column layout is a table. Hell, I could argue that a paragraph is a table with 1 column and 1 row. I understand that designers, for the sake of simplicity and different mediums (PDAs, cellphones) would like to eliminate all of their tabular layouts. I even agree that it's the right thing to do. But in order to do so still requires major work to make it consistent in multiple, and not even all, browsers as far as I can tell. In fact, the process is so counter-intuitive and has so many nuances that there are no less than 20 pages of google results for 'css column layout.' So until the major players get their [MOD] in gear and support the standard, the problem isn't eliminated, it's only moved from one file to another.
-
"I POSTED about this on the CSS forum. I find it kind of creepy that you're reposting my blog entries over here instead of on the existing thread, but okay." "Again, the fact that you posted this here, addressing it to me, just creeps me out..." Um, wtf? I tend to read the PHP Help forum during bits of downtime at work. You had posted in a thread I was reading and I saw your signature had a synopsis of "How to ask questions, the smart way" so I decided to check that out. As a web developer myself, of course I'm interested in any website I'm looking at. While checking out your site I saw the table-less layout entry. As I stated in my original post here, I dealt with the same issue this past Sunday so the article was of interest to me. I gave up in frustration on Sunday and I sensed a general feeling of dismay from the last line of your entry. I think the topic is of interest to us all so I decided to copy it here. I addressed it to you because, um, you wrote the original. I included the expanded part of my subject line so that others who found it interesting might chime in. I fail to see how that's creepy, although with that sense of paranoia, you may want to consider the field of cryptography. ;^] Now, if I were a true internet stalker, I'd probably have known that you originally posted this in the CSS forum in the first place, now wouldn't I?
-
"Yes, but once a substantial amount of layouts are created, they can be re-created for other purposes." That statement is no more or less true than any layout, including the one with the small table that I presented. At present, there always seems to be a "gotcha" with any multi-column CSS layout. This ranges from different browsers [not] supporting certain CSS properties, different browser behavior when certain events occur, the desire to add padding to the columns, wanting same-height columns, and the list goes on. The last article on CSS multi-column layouts likened them to the Holy Grail, with good reason IMO.
-
I happened to come across your blog in your signature and saw your table-less, CSS multi-column layout entry. I figured I'd respond here so everyone else can chime in. Blog Entry - http://jesirose.com/2007/02/11/equal-hight-columns-css/ "Most layouts use columns. Most designers want those columns to look like tables. Most visitors use IE. If we want to use a table-less layout, we run into problems. When you create your two or more columns, you’ll likely float them to make them side-by-side. Now, whichever has more content will be longer. This doesn’t always look great. People have come up with many approaches to fixing this issue. The way I’ve always done it is the “Faux Columns” approach. There’s a javascript approach, which would work if you knew for sure all your visitors would have javascript enabled. There’s a way to do it using just CSS, but it doesn’t work in IE. I have yet to find a CSS, non-graphical approach that works in all browsers " I can't tell you how many hours I've pissed away in the past trying to solve the same problem. There's as many solutions found through google as there are browser-specific caveats. After spending about 5 hours dealing with this same exact issue this past Sunday, I came up with a reasonable solution - to use a table. I can already hear the guns being loaded and cocked, but honestly, I consider my time to be valuable. I just don't see the point in spending several hours making small adjustments within the CSS, adding IE-specific crap, and special wrapper divs to make the content do what you want. I've recently switched to a design approach in which much of my HTML entities are broken down into small PHP files that I call components. One of the components is the general structure for all of the pages within a site, call it format.php. <?php // format.php // Define page layout // Expects vars: // $Title - Page title // $LCol - Left column content // $MCol - middle column content // $RCol - right column content ?> <!DOCTYPE ...> <html> <head> <link href="style.css" type="text/css" media="all" /> <title><?=$Title?></title> </head> <body> <div id="Header">The header</div> <table id="Main"> <tr> <td id="LCol"><?=$LCol?></td> <td id="MCol"><?=$MCol?></td> <td id="RCol"><?=$RCol?></td> </tr> </table> <div id="Footer">The footer</div> </body> </html> I'm all for standards across browsers, table-less layouts, and a whole slew of other would-be best practices. But until the "standard" is better supported, I don't think it's worth the headache. Just my $.02
-
I'm going to set up a little scenario here. Let's say you type something in notepad on your computer at home. Now let's say you want what you typed to be permanent. Now I'm going to ask a stupid question. Do you save the file to disk or do you just walk away from the computer and hope that what you typed will still be there 30 days later? Keep in mind that this computer might be used for many, many other tasks that could potentially warrant a system restart. In addition, environmental conditions could cause it to be turned off for hardware maintenance or repairs. If you want the information to be permanent, you have one choice. Save it to the server's hard disk. You have two choices on how you do that: 1) Creating your own files with fopen, fwrite, fread, etc. 2) Database, MySQL seems to go hand-in-hand with PHP.
-
You're including the word 'new' in your regexp so it's going to be returned in $result. Try this, going from memory so might need some adjustment: preg_match('/Array\(.*\);/i', $data, $result); Or this: preg_match('/new (Array\(.*\);)/i', $data, $result); echo($result[1]);
-
Headers are automatically sent the moment you start outputting anything to the browser. Almost at the bottom of your code you have a statement trying to modify the header: header ('content-type: image/png'); Now look at your error message: Warning: Cannot modify header information - headers already sent Putting 2 and 2 together gives...
-
Make sure that each user has a unique identifier and give them a link such as: http://www.domain.com/referral.php?id=unique_identifier to give to others. When someone visits the referral.php script, pop the id into the $_SESSION array and redirect to the home page. Then on site registration, check if the referral ID has been set in $_SESSION. If it has, you can credit the referrals properly.