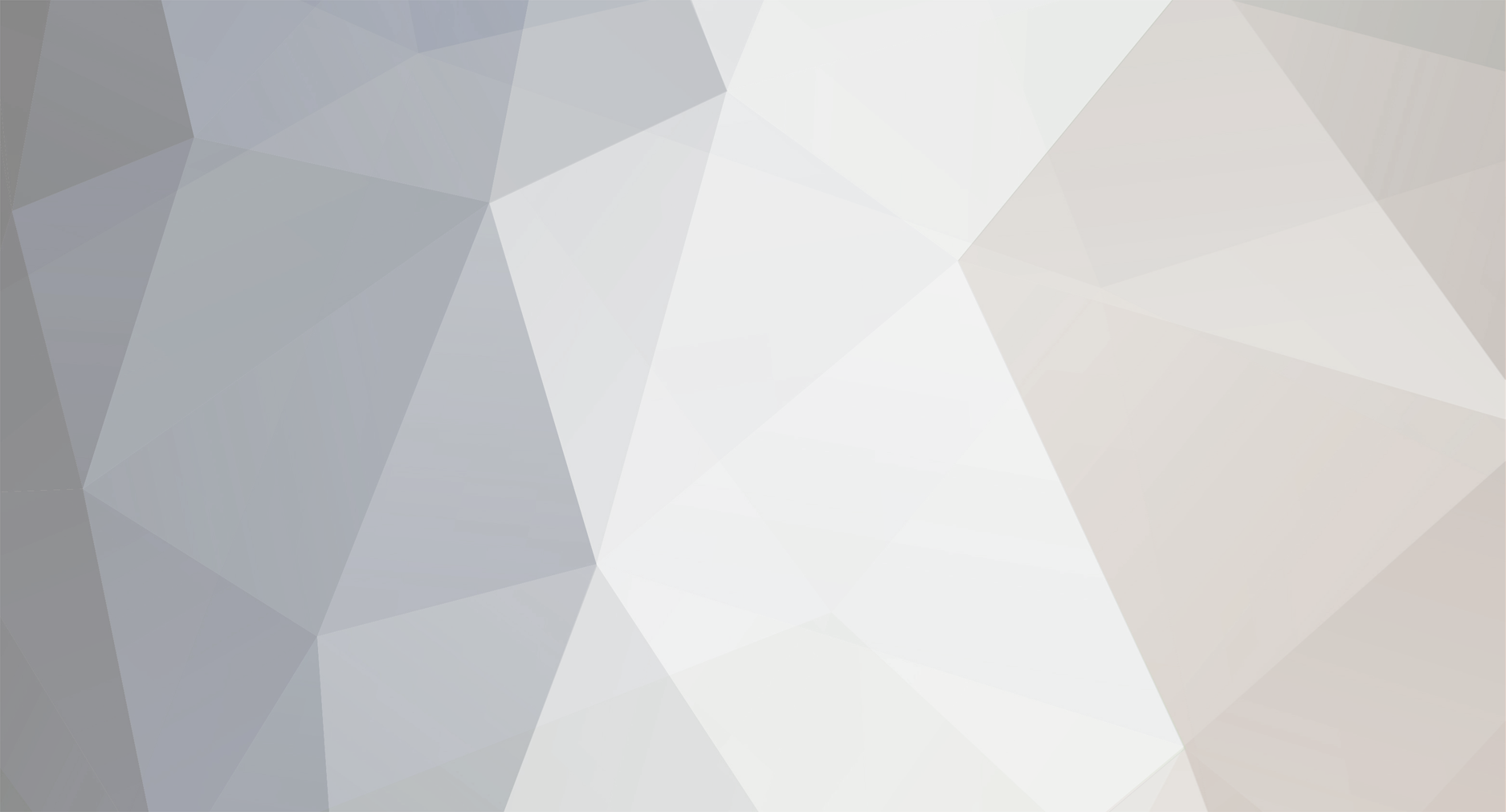
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I was considering the possibility. I would imagine I would be dumping all of the databases into some directory on our current host and then using a .tar and ftp'ing from one host to the other. Would I be faced with a lot of complications in directory permissions or would those be preserved? I know enough in *nix to be dangerous but by no means am I a proficient user.
-
My company is talking about switching webhosts and I would be in charge of migrating our current website to the new host. Our site is actually a web product. Every time we pick up a new client they receive their own database and their own data directory. They have unlimited access to uploading files (through our site) as well as bandwidth. So in addition to the normal moving of PHP code from one server to another I'd also have to move client data directories and client databases. Can anyone offer some advice or suggestions to make such a move easier not only us but our clients? This would be the first time I've taken on such a task and would really like to not screw it up!
-
1) Yes you can. The method for doing so depends on what these many other things are and how your program or database is storing them. 2) You can do that also. [code]<?php $outer = 1; while($outer< 10){ $inner = 1; while($inner< 10){ echo "$inner * $outer = " . $inner++ * $outer . "<br/>"; } $outer++; ?>[/code]
-
I find it ironic you'd want to assign $_FILES['form_param'] to another variable to save typing yet you didn't want to use a single loop and would rather type the same code thirty times over. World works in mysterious ways I guess! ;)
-
I'll toss in that there's at least two philosophies that I know of when it comes to returning early from a function. One side will tell you to only return a single time at the very end. [code]<?php function SingleReturn(){ $Ret = NULL; // Our return value if(!$no_error_check_1){ // Our first test for an error showed no error, so we can continue // some statements if(!$no_error_check_2){ // Our second test for an error passed so we keep on going // some more statements $Ret = $SomeFinalValue; } } return $Ret; } ?>[/code] The other side will tell you to return whenever the function can no longer continue. [code]<?php function ManyReturns(){ if($error1){ return NULL; } if($error2){ return NULL; } // Now we're done with errors we can continue // some stuff return $SomeFinalValue; } ?>[/code] People who favor the first method will argue that it's better to have one and only return simply because you know that all of the code in the function is looked at. I.E. with early returns you run the risk of forgetting to do something integral to your application. Also, as you indent further and further you will be encourage to break your functions up into multiple functions which can help maintain readability. People who favor the second method will argue that the structure of the function is easier to follow because you know that once you reach a certain point you're free to continue processing. It also requires less indenting so can also be easier to read. I myself favor the first method. If I find myself indenting more than 3 or 4 levels in I usually create another function and it helps down the road when I return to my read my old code. It doesn't matter which one you choose though. Just pick one and use it consistently throughout your project. If you mix them up you'll find yourself having a harder time debugging because you may not notice an early return in one function when you thought it returned only once at the end. Hope that helps a bit.
-
Remove everything after a certain character in a string
roopurt18 replied to rossh's topic in PHP Coding Help
[code] <?php $string = 'Hello, World! How are you? It looks like you\'re fine!'; $string = explode('?', $string); $string = $string[0]; echo $string; ?> Outputs: Hello, World! How are you?[/code] -
You can't comma separate a WHERE clause as far as I know.
-
Every form field of the following type: <input type="file" name="some_name"/> creates a corresponding entry in the $_FILES array. You check if the user put something into the field by checking for the corresponding entry in $_FILES.
-
I understand that. The question I'm asking is how does your program know which IDs are the ones to hide? You can use this MySQL statement: SELECT title, tid FROM ibf_topics WHERE forum_id != 53 AND forum_id != 85 AND forum_id != 86 And so on. Or you can use: 'SELECT title, tid FROM ibf_topics WHERE forum_id NOT IN(53, 85, 86, 88, 90, 93) But my question is how does your program know to use 53, 85, 86, 88, 90, and 93? How does it know that 33 should or should not be hidden?
-
Anything uploaded will appear in $_FILES.
-
How does your application determine that 85, 86, 88, 90, 93, and 53 are the forum IDs to hide? I know you can look at the MySQL table and pick them out easily, but how does your program know?
-
I'm going to guess that the forum_id column is the id of the thread and 85, 86, 88, 90, and 93 are threads you'd like to hide?
-
If you have: if(true){ // 110 statements } You chould change it to if(true){ DoSomething(); } function DoSomething(){ // 110 statements } instead of if(true){ include('110statements.php'); } I find having include all over the place often sends you on a wild goose chase as to what file is crashing your script. And when you're passing data back and forth between files like this it can get really messy. I come from a C / C++ background where this type of stuff just wasn't used, so I've never been comfortable with it. The current project I've inherited is full of it and it drives me crazy.
-
What you should really be concerning yourself with is the algorithms you use to accomplish your tasks. Pretend you're a mechanic working on the four wheels of a car. Let's say you need to remove each wheel of the car, do some stuff, and then put the wheel back on. Consider the following procedures: Get out tools to remove wheel #1 Remove wheel #1 Put away tools to remove wheel #1 Get out tools to do procedure Do procedure to wheel #1 Put away tools to do procedure Get out tools to replace wheel #1 Replace Wheel #1 Put away tools to replace wheel #1 Move onto wheel #2 and repeat the whole process. Or are you going to do it like this: Get out the tools to remove a wheel Remove all four wheels Put away the tools to remove a wheel Get out the tools to do procedure Do procedure to all four wheels Put away tools to do procedure Get out tools to replace a wheel Replace all four wheels Put away tools to replace a wheel The choice is fairly obvious. The second process, or algorithm, is more efficient. It involves a lot less time walking back and forth in the garage getting out and putting away the same tools over and over again. It seems obvious in an example such as that, but a lot of times when looking at another person's code, you'll see that they're doing things as inefficiently as the first mechanic. If you really want to create fast loading pages, try to develop efficient ways to work with your data. It really doesn't matter how many includes, function calls, variable assignments or reads, or whether or not you use single or double quotes. You can streamline all of that as much as possible and you'll shave maybe a few milliseconds off your script's execution time. The stuff you really want to watch out for is repeated loops over the same data or horribly inefficient MySQL queries. It doesn't matter if your page is processed in less than a second if the 3 MySQL queries on it take a total of 30 seconds to execute. The key thing to remember is to use efficient algorithms but to maintain the readability of your code. It's not worth optimizing the bejesus out of your code to gain a millisecond of execution time if you can't easily tell what the code is doing 6 months down the road. You should try and apply that concept to 99% of what you write. After all is said and done, if you have a piece of your site that is performing much too poorly, you can go back and optimize just that portion of your code. As a final comment, if you have an if body that is really big, consider putting it into another function and calling just that function rather than creating another .php file to include().
-
You wanted it to not show rows that don't have fully complete data. Looking at what was output above, a lot of your records don't have fully complete data. (EDIT) That's just after a quick glance at your database records. The problem could very well be something else. Try putting more print statements in the code to see exactly what it's doing.
-
Store each piece of repeatable data in an array as you encounter it and then process the arrays.
-
It could be that I screwed up or that something else is wrong. It'd be nice to get a better look at the data you're pulling from the database. Can you change: [code]while($row = mysql_fetch_array($sql)){[/code] to [code] while($row = mysql_fetch_array($sql)){ echo '<pre style="text-align: left;">' . print_r($row, true) . "</pre>"; continue; [/code] Leave the rest of the code the same and copy and paste what the output of that looks like. Remember to remove any sensitive data!
-
For starters, you shouldn't be making so many damned variables. Look at how much you've typed! You must be exhausted. Secondly, the first part of your if statement is checking the variable $monday7 and [b]not[/b] $mondaypm7. Thirdly, try this: [code] <?php ?> <!-- Page Code --> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>City Response.com | Installation | Electrical Installation</title> <? include "../../includes/meta.php"; ?> </head> <body> <?php include "../db.php"; $thisweek = date("W"); $nextweek = $thisweek + '1'; $weekafter = $thisweek + '2'; // *** You don't need to separate the individual ORs with parens //$sql = mysql_query("SELECT * FROM electrical WHERE wn = '$thisweek' OR (wn = '$nextweek') OR (wn = '$weekafter') "); $sql = mysql_query("SELECT * FROM electrical WHERE wn = '$thisweek' OR wn = '$nextweek' OR wn = '$weekafter' "); // *** To help save yourself some typing below $days = Array( 'monday', 'tuesday', 'wednesday', 'thursday', 'friday', 'saturday' ); while($row = mysql_fetch_array($sql)){ // *** Save yourself some typing foreach($days as $day){ for($i = 1; $i <= 10; $i++){ ${$day . 'am' . $i} = trim($row[$day . 'am' . $i]); ${$day . 'pm' . $i} = trim($row[$day . 'pm' . $i]); } } $wn = $row["wn"]; ?> <center><b><font size="+3"> <?php // *** Get the first week of the year, someone might have a better way of // of doing this and honestly I have no idea if it'll work $Year = $wn >= 44 && $wn <= 52 ? 2006 : 2007; // Determine which year to use $Day = 1; // We will be starting with January 1st // Loop until we find the first monday of the year do{ $DateRet = date("D", mktime(0, 0, 0, 1, $Day++, $Year)); }while(strcmp('Mon', $DateRet)); // After the loop finishes, $Day should be 1 - 7 depending on which day in // January the first Monday of the year is // Now we update $Day to hold the number of extra days for the week number $Day += 7 * $wn; echo "Week Commencing " . date("jS F Y", mktime(0, 0, 0, 1, $Day, $Year)); ?> </b></font></center> <table width=100% border="1"> <tr> <td>Time</td> <td>Monday</td> <td>Tuesday</td> <td>Wednesday</td> <td>Thursday</td> <td>Friday</td> <td>Saturday</td> </tr> <?php $all = ''; // What we'll dump at the end // Do for am for($i = 1; $i <= 10; $i++){ $skip = false; $row = '<tr><td>a.m</td>'; foreach($days as $day){ // $days was set above $val = ${$day . 'am' . $i}; if(!strlen($val)){ $skip = true; // flag outer loop to skip this row break; }else{ $row .= '<td align="center"> ' . $val . '</td>'; } } if(!$skip){ // If we don't want to skip, append to $all $all .= $row . '</tr>'; } } // Do for pm for($i = 1; $i <= 10; $i++){ $skip = false; $row = '<tr><td>p.m</td>'; foreach($days as $day){ // $days was set above $val = ${$day . 'pm' . $i}; if(!strlen($val)){ $skip = true; // flag outer loop to skip this row break; }else{ $row .= '<td align="center"> ' . $val . '</td>'; } } if(!$skip){ // If we don't want to skip, append to $all $all .= $row . '</tr>'; } } echo $all; ?> </table> <br /> <? } ?> </body> </html> <!-- Page Code --> <? ?> [/code]
-
I'm more curious as to why 7 is appended to all of the variable names? Does this mean you have $tuesday1 through $tuesday7? Does this apply to every day of the week?
-
After the message is sent and everything, you can: [code]<?php // do everything that submits the message // redirect to the same page header("Location: " . $_SERVER['PHP_SELF']); exit(); ?>[/code] However, all they have to do now is hit the back button to accomplish the same thing. If you really want to stop them from spamming in this manner you really only have two choices I can think of: 1) Double check that this user hasn't recently, or ever, sent a message with the same exact body. 2) Limit the number of posts per segment of time. The reason every other community website uses one of those two features is they're the only real way of preventing it. A third possibility is to generate a unique token every time your page displays a form and save that token in a table with a timeout value. When processing the posted form, check if the token your user is submitting exists in this table, that it matches the user who created the token, and that it is within the timeout period.
-
An extension to my reply above. When you're using bits to represent settings you're packing them into a variable. That variable might be 16, 32, 64, etc. bits in length. The size of that variable determines how many preferences or permissions you can pack in there. For instance, a 32-bit variable can represent 32 permissions with on / off settings. If you have a preference that is more than on / off, maybe it's: very low, low, avg, high, very high. That's 5 settings for one permission / preference. How many bits are necessary to store 5 unique combinations? The answer is 3 bits: 001 - very low 010 - low 011 - avg 100 - high 101 - very high Notice that we didn't use the values 000, 110, or 111. Going back to our 32-bit number, if we were storing the previous preference / permission, 3 of the 32 bits would be taken by that preference. This leaves us with 29 more bits to work with; we could store 29 more on / off preferences [b]or[/b] maybe 23 more on / off preferences and another preference that requires 6 bits (23 + 6 = 29). As for how we would go about storing and retrieving such a preference: [code]<?php define( 'PNAME_VLOW', 0x01 ); define( 'PNAME_LOW', 0x02 ); define( 'PNAME_AVG', 0x03 ); define( 'PNAME_HIGH', 0x04 ); define( 'PNAME_VHIGH', 0x05 ); define( 'PNAME_ALL', 0x07 ); $prefs = 0x00; // Set initial preferences // A lot of code that modifies the value in $prefs // Now determine which of PNAME the user has permission to do switch( $prefs & PNAME_ALL ){ case PNAME_VLOW: // very low break; case PNAME_LOW: // low break; case PNAME_AVG: // average break; // And so on... } ?>[/code] Notice now that instead of checking if the bit is turned on (Using $prefs & PNAME_const), we're checking which out of [b]all[/b] the bits for the preference are on [b]and[/b] which value they're equal to.
-
It [i]is[/i] the way [b]chmod[/b] works: [b]chmod[/b] gives you four columns, each of which can be a bit combination of the following: 4 : 0100 2 : 0010 1 : 0001 Since the highest bit possible is the (counting from the right) 3rd bit, we can ignore the 4th bit and drop it. 4 : 100 2 : 010 1 : 001 Now, [b]chmod[/b] has 4 possible settings, each setting is a combination of those 3 bits. So all total we need [i]at least[/i] 4 * 3 = [b]12[/b] bits to represent a [b]chmod[/b] setting. chmod columns: [code] SUI Owner Group Others 000 000 000 000 [/code] [b]Counting Problems[/b] [i]Q:[/i] How many combinations exist for each [b]chmod[/b] column? [i]A:[/i] 3 binary digits, each with 2 possible (0 or 1) values minus the number of impossible values (000): 2 * 2 * 2 - 1 = 7 [i]Q:[/i] How many possible [b]chmod[/b] permissions exist? [i]A:[/i] Our answer from above multiplied by the number of columns (4), or: 28
-
One of the things you're missing Zanus is in your hex values you're setting multiple bits as ones for a single permission: Ignoring leading zeros, 0x00000128 in binary is: 0001 0010 1000 You don't need 3 ones in your permission constant to specify 1 setting. So let's say you have the following: 0x01 -> 0000 0001 0x02 -> 0000 0010 0x01 & 0x02 -> 0000 0001 & 0000 0010 -> 0000 0000 (none of them have a 1 in the matching column of the other) 0x01 | 0x02 -> 0000 0001 | 0000 0010 -> 0000 0011 (the result has a 1 where any of the inputs had a 1) 0x01 & ~0x02 -> 0000 0001 & ~0000 0010 -> 0000 0001 & 1111 1101 -> 0000 0001
-
Why not do something like: [code]<?php $sql = "INSERT INTO TableName (Col_1, Col_2, ..., Col_n) VALUES "; $extras = Array(); foreach($DataSet as $Data){ $extras[] = "({$Data['Col_1']}, {$Data['Col_2']}, ..., {$Data['Col_n'})"; } $sql .= implode(", ", $extras); ?>[/code] You said this is to process a very large file, it might be best to use ini_set to turn off the script time limit and the memory limit and run it from a command prompt. If this is an operation that needs to run frequently consider setting it up as a cron.
-
Your password field is going to be VARCHAR. md5 is a php function you call on the password [i]before[/i] inserting it into the database.