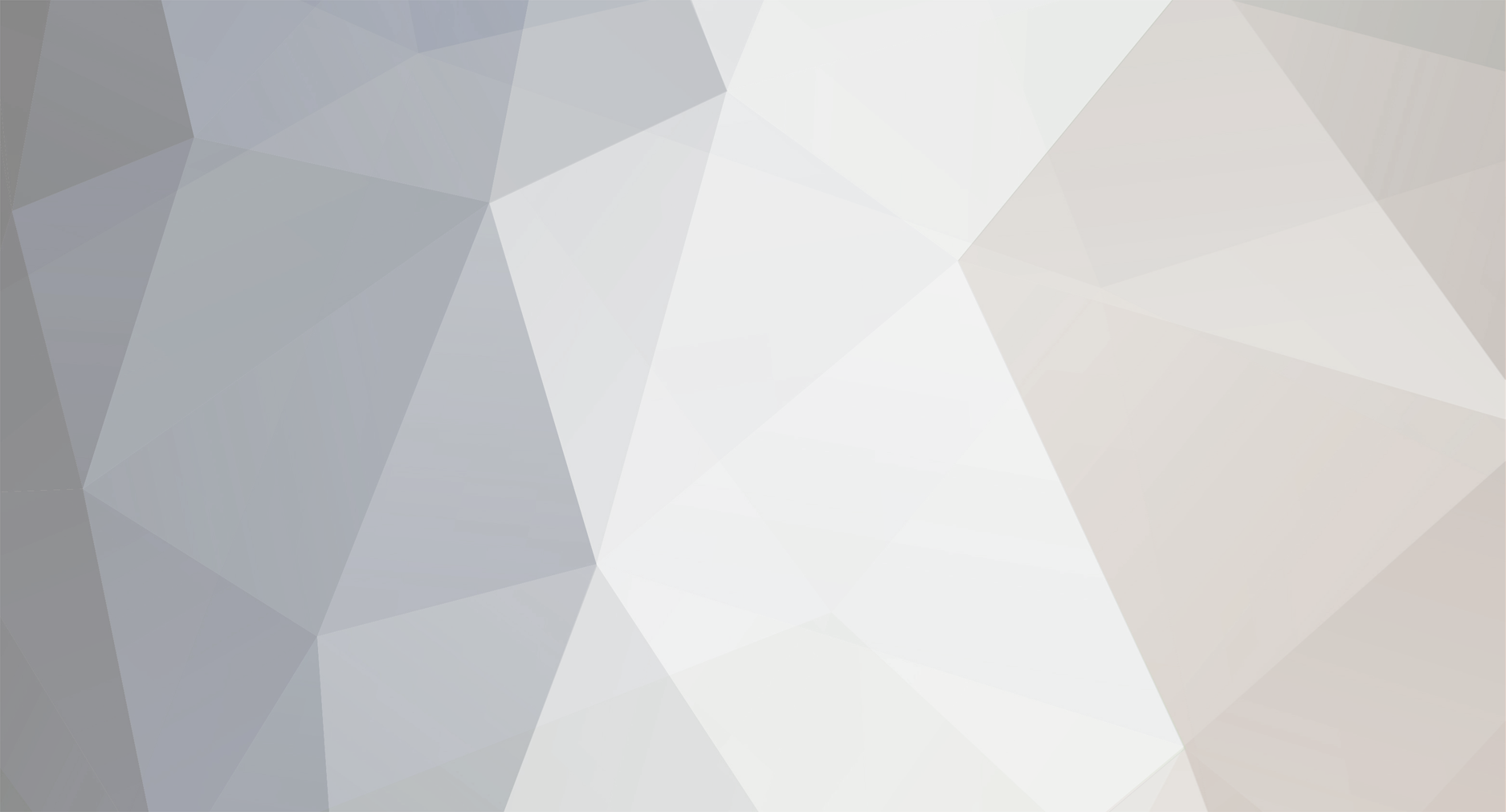
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I think you're having trouble understanding the distinct between development and production. development is the box you develop your code on. It might your local PC, i.e. the one literally sitting next to you. It might be a remote PC in your office, i.e. it's down the hall. This box will have a web server, PHP, and likely a database server installed on it (depending on your application's needs). The PHP environment will likely have error_reporting turned on at the most paranoid level. You perform all of your coding and testing on this server. production is the computer provided to you by your web host. The only thing you do on this box is upload your code to it. You don't edit code on this box. You don't test code on this box. You don't add new features on this box. You touch this box as little as possible. It sounds to me like your dreamweaver is configured to edit files in a remote location, i.e. on your production box. If this is the case, then you are busy editing files while people are also trying to use them (by browsing the site). This creates an extremely unstable environment. cron is a linux utility for running programs on a schedule. I don't know of any pre-built cron script that does what I described; you'd have to write your own.
-
How about the PC in front of you?
-
It does not. Read the appropriate entry in the PHP manual to see what trim() does. There are third party programs that will encode your PHP scripts. Essentially your PHP scripts become random garbage that can only be run with the appropriate decoder. Let's say I create a nice PHP product and I want to sell it. I don't want my customers to easily reverse engineer my code so I will provide them with encoded files. This way my property is protected and they can use the program. It also helps with support requests because it is unlikely that your customers have tampered with your encoded files since they will be just garbage in their text editor. By "box" I mean server. You should develop your code locally on a PC or server designed for development. When you're ready to release your code, you should upload it to your production server. Once it's on the production server, you stop tampering with it. There is no such term as hash-map that I'm aware of; I made it up strictly for that post. Anyways, what I meant was you should take means to detect when your production code has been altered or tampered with. You could, using a PHP program, create an md5 hash of every file in your application and a map of the files and directories. You create this data set before deploying your application; this is your known-good configuration. On the production server you should set up a cron script that checks the current production hashes against the known-good configuration. If something doesn't match up, then someone has tampered with your files.
-
Your comment about using date( 's', $etime ) will not produce the desired result since $etime represents the number of seconds since the epoch. At this point the OP has plenty of solutions to choose from. The one he chooses boils down to how precise he desires to be.
-
Don't put anything in public_html that doesn't have to be, such as the sessions. You only need to use mysql_real_escape_string() when inserting into a MySQL database. Using it on values not going into the database is wasteful. addslashes() is probably unnecessary if you're using mysql_real_escape_string(). trim() doesn't have anything to do with security. Other security tips: * Don't store passwords in plain-text. * Encode your files. * Don't perform development and production on the same box (it sounds like you are from what you said about error_reporting). * Create a hash-map (for lack of a better term) of your deployed application and its directories. Compare regularly (using cron) with a known-good configuration to detect if someone is changing your files or directories (think root-kits).
-
Which PHP Editor do you think is the best? [v2]
roopurt18 replied to Daniel0's topic in Miscellaneous
Other - PhpEd from nuSphere. vim is nice as well. -
Fixed something with the seconds calculation: <?php function time_elapsed( $time ) { if( is_string( $time ) && is_numeric( $time ) === false ) { $time = strtotime( $time ); if( $time === false ) { throw new Exception( "Can not convert time string to timestamp." ); } } if( is_numeric( $time ) === false ) { throw new Exception( "Can't make any sense of the time value." ); } $curr = mktime(); if( $time == $curr ) { return 'right now'; } $tY = date( 'Y', $time ); $tm = date( 'm', $time ); $td = date( 'd', $time ); $tH = date( 'H', $time ); $ti = date( 'i', $time ); $ts = date( 's', $time ); $cY = date( 'Y', $curr ); $cm = date( 'm', $curr ); $cd = date( 'd', $curr ); $cH = date( 'H', $curr ); $ci = date( 'i', $curr ); $cs = date( 's', $curr ); $YD = $cY - $tY; $mD = $cm - $tm; $dD = $cd - $td; $HD = $cH - $tH; $iD = $ci - $ti; $sD = $cs - $ts; $values = array(); if( $YD >= 2 || ($YD == 1 && $tm <= $cm) ) { $values[] = array( 'year', $YD ); } $mod = $dD < 0 ? -1 : 0; if( $mD >= 1 ) { $values[] = array( 'month', $mD + $mod ); }else if( $mD < 0 ) { $values[] = array( 'month', (12 - $tm) + $cm + $mod ); } $mod = $HD < 0 ? -1 : 0; if( $dD >= 1 ) { $values[] = array( 'day', $dD + $mod ); }else if( $dD < 0 ) { $values[] = array( 'day', (date( 't', $time) - $td) + $cd + $mod ); } $mod = $iD < 0 ? -1 : 0; if( $HD >= 1 ) { $values[] = array( 'hour', $HD + $mod ); }else if( $HD < 0 ) { $values[] = array( 'hour', (24 - $tH) + $cH + $mod ); } $mod = $sD < 0 ? -1 : 0; if( $iD >= 1 ) { $values[] = array( 'minute', $iD + $mod ); }else if( $iD < 0 ) { $values[] = array( 'minute', (60 - $ti) + $ci + $mod ); } if( $sD >= 1 ) { $values[] = array( 'second', $sD ); }else if( $sD < 0 ) { $values[] = array( 'second', 60 + $sD ); } foreach( $values as $k => $v ) { if( $v[1] > 1 ) { $v[0] .= 's'; } $values[$k] = $v[1] . ' ' . $v[0]; if( $v[1] == 0 ) { unset( $values[$k] ); } } return implode( ' ', $values ) . ' ago'; } ?>
-
I believe this is more accurate, but it requires more testing than I have time to do right now: <?php echo 'Current: ' . date( 'Y-m-d H:i:s' ) . "\n"; echo time_elapsed( strtotime( '-1 year -12 months' ) ) . "\n"; echo time_elapsed( strtotime( '-4 year -6 months' ) ) . "\n"; echo time_elapsed( strtotime( '-12 months' ) ) . "\n"; echo time_elapsed( strtotime( '-11 months' ) ) . "\n"; echo time_elapsed( strtotime( '2009-10-18' ) ) . "\n"; echo time_elapsed( strtotime( '2009-10-04' ) ) . "\n"; echo time_elapsed( strtotime( '2009-10-29' ) ) . "\n"; echo time_elapsed( strtotime( '2008-02-18' ) ) . "\n"; echo time_elapsed( strtotime( '2008-02-04' ) ) . "\n"; echo time_elapsed( strtotime( '2008-02-28 09:21:00' ) ) . "\n"; echo time_elapsed( strtotime( '2008-02-28 09:35:00' ) ) . "\n"; echo time_elapsed( strtotime( '2008-02-28 08:00:45' ) ) . "\n"; echo time_elapsed( strtotime( '2008-02-28 19:15:23' ) ) . "\n"; echo time_elapsed( mktime() ) . "\n"; echo time_elapsed( strtotime( "-1 year -1 day" ) ) . "\n"; echo time_elapsed( strtotime( "-2 hours -34 minutes -20 seconds" ) ) . "\n"; echo time_elapsed( strtotime( "-2 hours -98 minutes" ) ) . "\n"; function time_elapsed( $time ) { if( is_string( $time ) && is_numeric( $time ) === false ) { $time = strtotime( $time ); if( $time === false ) { throw new Exception( "Can not convert time string to timestamp." ); } } if( is_numeric( $time ) === false ) { throw new Exception( "Can't make any sense of the time value." ); } $curr = mktime(); if( $time == $curr ) { return 'right now'; } $tY = date( 'Y', $time ); $tm = date( 'm', $time ); $td = date( 'd', $time ); $tH = date( 'H', $time ); $ti = date( 'i', $time ); $ts = date( 's', $time ); $cY = date( 'Y', $curr ); $cm = date( 'm', $curr ); $cd = date( 'd', $curr ); $cH = date( 'H', $curr ); $ci = date( 'i', $curr ); $cs = date( 's', $curr ); $YD = $cY - $tY; $mD = $cm - $tm; $dD = $cd - $td; $HD = $cH - $tH; $iD = $ci - $ti; $sD = $cs - $ts; $values = array(); if( $YD >= 2 || ($YD == 1 && $tm <= $cm) ) { $values[] = array( 'year', $YD ); } $mod = $dD < 0 ? -1 : 0; if( $mD >= 1 ) { $values[] = array( 'month', $mD + $mod ); }else if( $mD < 0 ) { $values[] = array( 'month', (12 - $tm) + $cm + $mod ); } $mod = $HD < 0 ? -1 : 0; if( $dD >= 1 ) { $values[] = array( 'day', $dD + $mod ); }else if( $dD < 0 ) { $values[] = array( 'day', (date( 't', $time) - $td) + $cd + $mod ); } $mod = $iD < 0 ? -1 : 0; if( $HD >= 1 ) { $values[] = array( 'hour', $HD + $mod ); }else if( $HD < 0 ) { $values[] = array( 'hour', (24 - $tH) + $cH + $mod ); } $mod = $sD < 0 ? -1 : 0; if( $iD >= 1 ) { $values[] = array( 'minute', $iD + $mod ); }else if( $iD < 0 ) { $values[] = array( 'minute', (60 - $ti) + $ci + $mod ); } if( $sD >= 1 ) { $values[] = array( 'second', $sD ); } foreach( $values as $k => $v ) { if( $v[1] > 1 ) { $v[0] .= 's'; } $values[$k] = $v[1] . ' ' . $v[0]; if( $v[1] == 0 ) { unset( $values[$k] ); } } return implode( ' ', $values ) . ' ago'; } ?> yields: Current: 2009-11-18 10:33:16 2 years ago 4 years 6 months ago 1 year ago 11 months ago 1 month 10 hours 33 minutes 16 seconds ago 1 month 14 days 10 hours 33 minutes 16 seconds ago 20 days 10 hours 33 minutes 16 seconds ago 1 year 9 months 10 hours 33 minutes 16 seconds ago 1 year 9 months 14 days 10 hours 33 minutes 16 seconds ago 1 year 8 months 19 days 1 hour 12 minutes 16 seconds ago 1 year 8 months 19 days 58 minutes 16 seconds ago 1 year 8 months 19 days 2 hours 32 minutes ago 1 year 8 months 18 days 15 hours 17 minutes ago right now 1 year 1 day ago 2 hours 34 minutes ago 3 hours 38 minutes ago
-
Negative times? There is no such thing and I have no idea what you're talking about. You provided a function that: 1) Takes a parameter: A time in the past 2) Returns: The elapsed time between now and the parameter, as a text string So how come when I give your function a timestamp that is exactly 2 years ago your function returns 2 year(s) 11 day(s) ago?
-
<?php echo time_elapsed_string( strtotime( '-1 year -12 months' ) ) . "\n"; echo time_elapsed_string( strtotime( '-4 year -6 months' ) ) . "\n"; echo time_elapsed_string( strtotime( '-12 months' ) ) . "\n"; echo time_elapsed_string( strtotime( '-11 months' ) ) . "\n"; function time_elapsed_string($ptime) { /* Elapsed time */ $etime = time() - $ptime; /* Less than a second so return that */ if($etime < 1) { return '0 seconds ago'; } /* Crate an array of all of the time values */ /* You could modify this function later on when it checks what the values are which could, */ /* add the (s) on automatically if needed as 1 would be 1 hour but 2 would be 2 hours */ $a = array( (12 * (30 * (24 * (60 * 60)))) => 'year(s)', (30 * (24 * (60 * 60))) => 'month(s)', (24 * (60 * 60)) => 'day(s)', (60 * 60) => 'hour(s)', 60 => 'minute(s)', 1 => 'second(s)' ); /* Create a blank output string ready for analysis */ $outputString = ''; /* For each item in the time values array */ foreach($a as $secs => $str) { /* difference is the time elapsed devided by the seconds */ $d = $etime / $secs; /* If the difference is larger than one */ if($d >= 1) { /* Round the difference */ $r = round($d); /* Add the value to the output string */ $outputString .= $r . ' ' . $str . ' '; /* Minus the time that we just analysed from the elapsed time so that when it gets analysed */ /* for the next variable in the array it does not return something like 61 second(s) */ $etime -= ($r * $secs); } } /* Add the ago onto the end */ $outputString .= 'ago.'; /* Return the output string. */ return $outputString; } exit(); ?> yields: 2 year(s) 11 day(s) ago. 5 year(s) ago. 1 year(s) 5 day(s) ago. 11 month(s) 5 day(s) ago.
-
<?php echo time_elapsed( '2001-01-01 11:34 AM' ) . "\n"; echo time_elapsed( mktime() ) . "\n"; echo time_elapsed( strtotime( "-1 day" ) ) . "\n"; echo time_elapsed( strtotime( "-2 hours -34 minutes" ) ) . "\n"; function time_elapsed( $time ) { if( is_string( $time ) === true && is_numeric( $time ) === false ) { $time = strtotime( $time ); if( $time === false ) { throw new Exception( "Can not convert time string to timestamp." ); } } if( is_numeric( $time ) === false ) { throw new Exception( "Can't make any sense of the time value." ); } $curr_time = mktime(); $time_diff = $curr_time - $time; if( $time_diff < 0 ) { return false; } if( $time_diff === 0 ) { return '0 seconds ago.'; } static $divisors = null; if( $divisors === null ) { $divisors = array( array( 'day', 24 * 60 * 60 ), array( 'hour', 60 * 60 ), array( 'minute', 60 ), array( 'second', 1 ) ); } $descriptions = array(); foreach( $divisors as $div ) { $val = time_elapsed_helper( $time_diff, $div[1], $div[0] ); if( $val !== false ) { $descriptions[] = $val['description']; $time_diff = $val['remainder']; if( $time_diff === 0 ) { break; } } } return count( $descriptions ) === 0 ? false : (implode( ' ', $descriptions ) . ' ago'); } function time_elapsed_helper( $num_seconds, $divisor, $string ) { $rv = false; do { $result = (int)($num_seconds / $divisor); if( $result === 0 ) break; if( $result > 1 ) { $string .= 's'; } $rv = array( 'description' => $result . ' ' . $string, 'remainder' => $num_seconds % $divisor ); } while( false ); return $rv; } ?>
-
The site owner should provide you with some sort of mechanism to write the file on their server. There are many methods in which they can do this and the method they decide to choose will dictate your next series of "How do I..." questions. If you're asking for help with writing a file on a server that you do not have permission to write to, then this is the wrong place to ask and I suggest you skip this endeavor altogether.
-
If this is your first programming language and you're already catching typos like that, then you're off to a good start.
-
This statement in and of itself is incorrect. A != B || A != C will always be true only if B != C
-
Neither of you read properly or understood what Gayner wishes to accomplish. I don't think it really makes sense to deal with years and months, as then you have to account for leap years and use slightly different logic. <?php echo time_elapsed( '2001-01-01 11:34 AM' ) . "\n"; echo time_elapsed( mktime() ) . "\n"; echo time_elapsed( strtotime( "-1 day" ) ) . "\n"; echo time_elapsed( strtotime( "-2 hours -34 minutes" ) ) . "\n"; function time_elapsed( $time ) { if( is_string( $time ) ) { $time = strtotime( $time ); if( $time === false ) { throw new Exception( "Can not convert time string to timestamp." ); } } if( is_numeric( $time ) === false ) { throw new Exception( "Can't make any sense of the time value." ); } $curr_time = mktime(); $time_diff = $curr_time - $time; if( $time_diff < 0 ) { return false; } if( $time_diff === 0 ) { return '0 seconds ago.'; } static $divisors = null; if( $divisors === null ) { $divisors = array( array( 'day', 24 * 60 * 60 ), array( 'hour', 60 * 60 ), array( 'minute', 60 ), array( 'second', 1 ) ); } $descriptions = array(); foreach( $divisors as $div ) { $val = time_elapsed_helper( $time_diff, $div[1], $div[0] ); if( $val !== false ) { $descriptions[] = $val['description']; $time_diff = $val['remainder']; if( $time_diff === 0 ) { break; } } } return count( $descriptions ) === 0 ? false : (implode( ' ', $descriptions ) . ' ago'); } function time_elapsed_helper( $num_seconds, $divisor, $string ) { $rv = false; do { $result = (int)($num_seconds / $divisor); if( $result === 0 ) break; if( $result > 1 ) { $string .= 's'; } $rv = array( 'description' => $result . ' ' . $string, 'remainder' => $num_seconds % $divisor ); } while( false ); return $rv; } ?>
-
$x = 1; $y = 1; if( $x != 1 || $x != $y ) { echo 'true'; }else{ echo 'not always!'; }
-
PREVENTING Piracy of web based applications
roopurt18 replied to canadabeeau's topic in Miscellaneous
nuCoder is another option. You still need to encode your source code if you want them to require_once() it from your server, as it will come over in plain-text just like any other PHP file. I saw a project out there once upon a time, the goal of which was to turn PHP code into compiled programs. I don't know if it's still active or not though. -
a && b === !a || !b a || b === !a && !b If you can remember that, then you're good to go.
-
Hammers are better than screw drivers are better than wrenches. You gotta pick the right tool for the job. Average user, day-to-day business unrelated to video or CAD: Windows Average user, day-to-day business in video or CAD: Mac Average user, general purpose multimedia machine: Windows or Mac Gaming rig: Windows Novice user, non-business: Mac Stable server: Linux For the extreme enthusiast that likes to tweak every thing and doesn't mind spending 24 hours on something that should take 10 minutes or less, it doesn't really matter. My parents rendered their Windows PC inoperable three times in a row in less than three months. The number of phone calls I received from them about the PC and the weekends I spent reinstalling Windows were a real pain. On the fourth time they destroyed it, I said, "That's it. We're going to CompUSA and you're buying a Mac." That was like three years ago and their Mac is still running like a champ. I haven't had a "Help me with my computer" phone call in months either.
-
Why people store lists in databases like this is beyond me. Anyways: <?php $val = '[Trees][300][boxes][4000][Gizmos][3]'; // remove trailing [ and ] $val = trim( $val, '[]' ); $values = explode( '][', $val ); $max = count( $values ); for( $i = 0; $i < $max; $i += 2 ) { echo sprintf( "There are %s %s\n", $values[$i + 1], $values[$i] ); } ?>
-
The manual is always a good place to start: http://php.net/manual/en/book.mcrypt.php Right on that page is a link that says examples: http://www.php.net/manual/en/mcrypt.examples.php
-
Which PHP-Editor do you think is the best?
roopurt18 replied to briananderson's topic in Miscellaneous
[quote]I dont see why java is such a rave among academics[/quote] Because academics don't write production code. IMO Java is great for server apps, not so much for client stuff. -
It seems some how your browser is sending the form data as the request type. Can we see this in action online somewhere?
-
What's to stop someone from changing cookie/session value
roopurt18 replied to 9three's topic in PHP Coding Help
You need to store two values in the cookie for a remember me feature: 1) username 2) a unique key (not their password!) When a user requests to use the remember me feature, you need to generate a unique value and store it in both their user record in the database and in the cookie. When a user visits your site, look up the username and remember_me_key from the cookie and then look for a matching row in the database. If a matching row is found, log the user in and replace the remember_me_key with new values. In this way, an attacker needs to guess both the username and the remember_me_key in order to log in as another user. This still doesn't protect from session hijacking, which is a different matter altogether. And there is still nothing you can do if your client's machine is compromised. -
What's to stop someone from changing cookie/session value
roopurt18 replied to 9three's topic in PHP Coding Help
There's nothing to stop them from doing so. I don't store any information in cookies, except for the php session id (which happens automatically), for this very reason. Any user specific information should be kept in the $_SESSION array (which never travels to the client machine) or inside the database. If you follow that guideline, then the only danger you have is session hijacking. If the client machine, server machine, or the network connection between the two is compromised then it would be possible for a third party to extract the session id information and impersonate that user. You can protect the server by following best practices in server administration and code development. You can ignore insecurities that occur over the network (to some extent) by using an HTTPS connection. There's not much you can do to prevent your clients / users from compromising their machines though, as they're typically used by average people. One thing you can implement, is to prompt for a password that only the account holder would know when making extremely important updates or changes to account data.