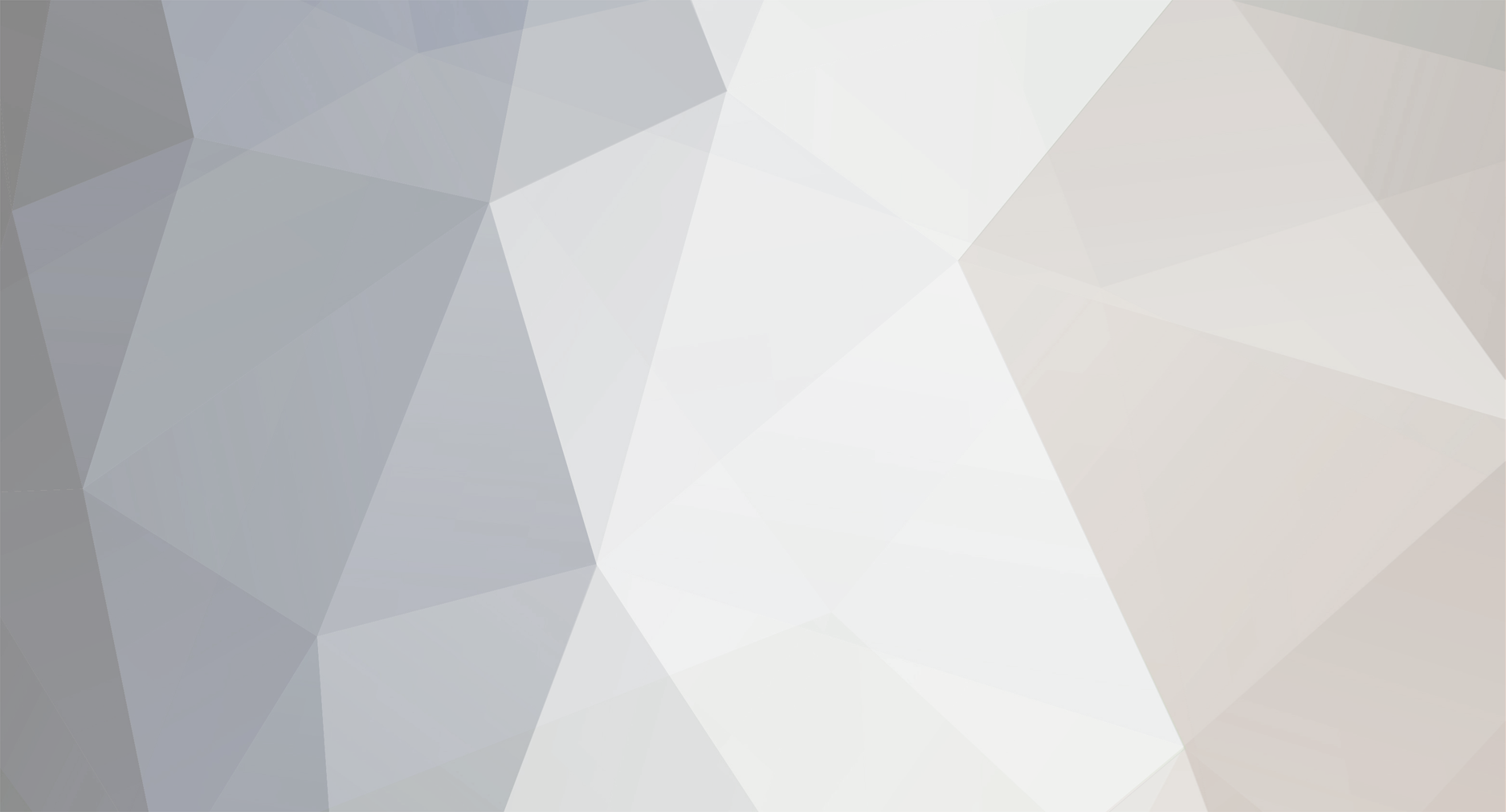
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Just run your script from the command line in windows; that's exactly what a cron is.
-
You seem to have a good idea of what's going on. Exactly. Tsk. Tsk. It's a good thing you left it there otherwise you may have let it go through your final code. We all do goofy things from time to time while coding; it is always best to paste your code directly as it is so that people may point out things you're not even asking about. Anyways, to help with the problem at hand, let's start with what we know. Also, since web applications are open to the public (even if password protected), we always want to give as little as possible about our application away to the user; always design your forms so that they send along just enough information to accomplish their task. Let's start with the process: User selects directory -> User selects files -> delete files Which translates to (substitute your own file names) list_dirs.php -> list_files.php -> delete_files.php This is exactly what you have (except the script names), so far so good. The root directories that you're listing to the user lives in: /usr/local/php_classes/forms/FORMS/ There is no reason for the end-user to ever know this directory exists. list_dirs.php exists and is working, so we skip that. Looking at list_files.php, our form is incorrect; all of the inputs have the same names, also we are using some incorrect input types. Think about what the user needs to do on this page: check off files from a listing. Thus our form should look like: Files: - [ ] file1 - [ ] file2 - [*] file3 - [ ] file4 - [*] file5 The brackets indicate a check box and the asterisks indicate that it is selected. There is no reason to place the file names in inputs with type="textbox"; indeed we do not want to invite the user to change file names (although a more experienced user can do so no matter what you do). This would indicate the HTML source we should be generating for our form should maybe look like: <p> Files: <ul> <li> <input type="checkbox" name="checkthis" /> file1 </li> <li> <input type="checkbox" name="checkthis" /> file2 </li> ... </ul> </p> We have two problems now: We still have duplicate name attributes for our input fields. How will we get the file names? We can fix both problems with a neat trick: You can always coerce $_POST to be in a format that is desirable for your application. By appending square brackets to the name attribute of the inputs, you will turn $_POST['checkthis'] into an array. Not only can we turn $_POST['checkthis'] into an array, but we can define the keys! For our purposes, setting the keys equal to the file names will be most convenient. This changes our form to: <p> Files: <ul> <li> <input type="checkbox" name="checkthis[file1]" /> file1 </li> <li> <input type="checkbox" name="checkthis[file2]" /> file2 </li> ... </ul> </p> Before we get back into the code, our final page that deletes the files will need to know which directory was originally selected in the drop down, so we will add that to the form as a hidden parameter. Thus our entire form should look like: <form action="delete_files.php" method="post"> <input type="hidden" name="directory" value="the_selected_path" /> <p> Files: <ul> <li> <input type="checkbox" name="checkthis[file1]" /> file1 </li> <li> <input type="checkbox" name="checkthis[file2]" /> file2 </li> ... </ul> </p> </form> Here is pseudo code to generate that form: list_files.php <?php $base_dir = '/usr/local/php_classes/forms/FORMS/'; $directory = $_POST['folder']; if(!is_dir($base_dir . $directory)){ // User has selected a directory that DOES NOT EXIST - aka they're // cheating. } ?> <form action="delete_files.php" method="post"> <input type="hidden" name="directory" value="<?php echo $directory; ?>" /> <p> Directory: <?php echo $directory; ?> </p> <p> Files: <ul> <?php // PHP PSEUDO CODE FOREACH $file IN $base_dir . $directory SKIP . AND .. echo " <li> <input type=\"checkbox\" name=\"checkthis[{$file}]\" value=\"1\" /> {$file} </li> "; ENDFOREACH ?> </ul> </p> <p> <input type="submit" value="Delete Selected" /> </p> </form> Moving along to delete_files.php, $_POST will have three keys: one for the submit button, one for the hidden field, and one for all of the checkboxes. The checkboxes key will be an array itself where each key is named after a file that was selected. Try this as a simple delete_files.php: <?php echo '<pre style="text-align: left;">' . print_r($_POST, true) . '</pre>'; ?> From there, it is trivial to write a loop that deletes the files that were selected: delete_files.php <?php $base_dir = '/usr/local/php_classes/forms/FORMS/'; $directory = $_POST['directory']; foreach($_POST['checkthis'] as $file => $empty){ if(file_exists($base_dir . $directory . '/' . $file)){ unlink($base_dir . $directory . '/' . $file); } } ?> There is one thing that I've noticed your scripts are missing (mine are as well), and that is proper checks to make sure the user isn't feeding you bad data. Remember that the user can try to be sneaky and traverse up your directory structure. So filter the user's incoming data, remove '.' and '..' strings from the paths they say they want to list, and any time you list or delete stuff make sure it's something the user originally had access to.
-
while (($file = readdir($dh)) !== false) { if($file !== "." && $file !== ".." ) //Get the short version of the file name //and store them in 2 arrays $short=shortimg($file); $aryRef[]=$short; $files[$short]=$file; } Did you intentionally leave off the curly brackets on the if statement? That closing curly bracket goes with your while loop. You should always include curly brackets around the body of your if statements, even if they're optional; trust me on that one. I've only glanced at your code, but if I understand it correctly you are grabbing all the files in the directory and generating: <input type='checkbox' name='checkthis' /> <input type='text' name='filename' value='the_path_and_name' /><br> The HTML this form generates will be similar to: <input type='checkbox' name='checkthis' /> <input type='text' name='filename' value='/the/path/file1' /><br> <input type='checkbox' name='checkthis' /> <input type='text' name='filename' value='/the/path/file2' /><br> There are two things very, very wrong with this: You are giving away the file structure of your web host - BAD! You have multiple inputs with the same name. If you can convince me that you understand what I've said so far, I can assist you with a good solution. But you have to convince me.
-
I just wanted to throw this out there, depending on how old your system is and what you're doing, it could be a hardware problem. A faulty power supply could cause a system to restart. If you play games and your system is very dusty inside, excessive heat can cause a restart as well. If you've added new hardware recently, it could also be driver incompatibilities as well. I'd go through the virus and spyware route first as that's most likely, but I wanted to give you some other alternatives to look into if you can't find anything wrong. P.S. Most computer hardware lasts for a very long time, but in terms of age most components fail around the 3 to 5 year mark when they start to go.
-
What about the HTML for the form where the user selects the files to delete? Also, on the form you posted, you can leave off the enctype attribute if you want, since you're not uploading any files with it.
-
Who on Earth would be naive enough to put a laptop in a locker, or bring it to high school (or lower) in the first place? I don't know about you guys, but where I went to school, anything valuable in locker == stolen. And who would want to stand there in front of their locker to use a laptop? ... Nothing ever gets stolen from our lockers. And you don't use it in front of your locker, you set some downloads in a cue and put it in your locker for downloading. Yes, I'm sure that would fly by the school's IT department just fine. I'm not trying to be a Negative Nancy or poo-poo ideas on a whim here, but one of the key points of designing anything is being realistic.
-
Who on Earth would be naive enough to put a laptop in a locker, or bring it to high school (or lower) in the first place? I don't know about you guys, but where I went to school, anything valuable in locker == stolen. And who would want to stand there in front of their locker to use a laptop? ...
-
Here we go again, curing the symptons and ignoring the disease. Get rid of all the books and BS busy work and you won't even need lockers anymore.
-
I don't see where you're setting the $id variable that is used in the query on detail.php
-
If I understand what you're saying, then you have something like: products product_id cost price pricing_brackets from to markup Your algorithm is then to pull everything from both tables, loop over the products, and determine which bracket it falls into. As I said before, in the worst case scenario, your code has to loop 21,000 times just to determine the pricing bracket of each product; now I know that's not the real-world case, but I'm talking about in terms of algorithm analysis. We can use a table join to return the product information and the matching pricing bracket in a single query; this means you effectively cut your loop down to iterating only as many times as there are items in the product table. SELECT i.`my_price`, i.`catalog_price`, i.`productCode`, m.`markup` FROM `CubeCart_inventory` i INNER JOIN `CubeCart_markup_table` m ON i.`my_price` BETWEEN m.`value_from` AND m.`value_to` That query uses the database to match the products with their appropriate markup; if you don't believe me, add m.`value_from` and m.`value_to` to the list of fields returned and run it in phpMyAdmin. Note also that since we are updating the `catalog_price` column, you probably don't need to return it as well. (The "best practice" when running queries is to ask for only the columns you are actually using.) This effectively changes your PHP code to: <?php $sql = " SELECT i.`my_price`, i.`catalog_price`, i.`productCode`, m.`markup` FROM `CubeCart_inventory` i INNER JOIN `CubeCart_markup_table` m ON i.`my_price` BETWEEN m.`value_from` AND m.`value_to` "; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ $row['catalog_price'] *= $row['markup']; // #1 Perform any other logic on the row // #2 Update the product row in the database } }else{ die('Error: ' . mysql_error()); } ?> We can take this one step further, we always want to push as much work into the DB engine as possible. Here is a query that should do everything your PHP code was doing. UPDATE `CubeCart_inventory` i, `CubeCart_markup_table` m SET i.`catalog_price` = i.`my_price` * m.`markup` WHERE i.`my_price` BETWEEN m.`value_from` AND m.`value_to` I used to do things the "long" way myself until I picked up a book on MySQL. I recommend following suit, you'll save yourself oodles of headache and complicated program code.
-
My guess is the project manager said, "Worry about it later!"
-
How about pasting the text you want to capture from and telling which part you want to capture?
-
JOINs make it easier to normalize your database as tippy hinted at. Without JOINs, some operations would take several database queries to perform; with proper use of JOINs, you can perform most tasks in a single query and avoid placing unnecessary burden on the MySQL server.
-
Are you familiar with regular expressions? They will make this task much easier. // UNTESTED // Assumes $content has the content you wish to scrape $regexp_a = '/<a[^>].*<\/a>/'; // $regexp_a = '/([<]a[^>]*[>](.*)[<][/]a[>])/'; // try this one if above fails preg_match($regexp_a, $content, $matches); echo '<pre style="text-align: left;">' . print_r($matches, true) . '</pre>';
-
Google search: http://www.google.com/search?hl=en&client=firefox-a&rls=com.ubuntu%3Aen-US%3Aofficial&hs=KBO&q=mysql+create+sequence&btnG=Search According to the top couple hits, a sequence is an Oracle ID field that auto increments. In MySQL you would do something like this: CREATE TABLE IF NOT EXISTS `users` ( `id` INTEGER UNSIGNED NOT NULL AUTO_INCREMENT PRIMARY KEY, # OTHER FIELDS ) Can you elaborate more on what you're trying to do?
-
If you're using *nix you could just exec it to the shell: $dir = '/the/dir/to/delete'; exec('rm -r ' . $dir);
-
I also notice that you say you intend to delete multiple items at a time, but you are using your variables as if they contain a single value. $file = $_POST['filename']; $checked = $_POST['checkthis']; What does the HTML for your form look like that submits to that page?
-
If both the user name and password are md5'ed in the cookie, when the user returns how do you plan to determine which user it was? And yes, storing the user's password anywhere other than in your database is a bad idea.
-
I'd like to fix this bug I've been working on and convince my boss we could make much better software.
-
@runnerjp I don't have any experience resizing images with the built-in PHP functions; I use a utility named convert on my linux host. The best way to go about tackling new programming problems is to separate all other complexities from the problem at hand. Currently, your problem pertains to resizing an image and you are trying to solve it in an existing script. The danger in this approach is when it's not working, it's sometimes unclear if the problem lies in your image resizing code or somewhere else in the script. Create a separate test folder on your host, FTP a couple of images there, and use some tutorials from the PHP manual (or elsewhere on the 'net) to write a script that does one thing and one thing only: resizes an image that is already present in the directory. Work it through, be methodical, and think about your errors as you get them. When it's working apply it to your existing script. I know that's not the answer you were looking for, but it's the best way to learn.
-
Do you mean you want the overall layout to remain constant and just alter the order of the videos and associated text? Or do you want the entire layout to change (i.e. first load the videos are on the left, second load they're on the right)?
-
I just have to ask, why not just use another while($row_inner = mysql_fetch_assoc($array)){ ? <?php //--------------------------- // Retrieve the markup table //--------------------------- $query = "SELECT * FROM CubeCart_markup_table"; $array = mysql_query ($query); // $array1 = mysql_fetch_assoc ($array); // print_r ($array1); //--------------------------------------------------------------------------------------- // Retrieve the "cost price", "retail price" and "Product Code" from the Inventory Table //--------------------------------------------------------------------------------------- $query = "SELECT my_price, catalog_price, productCode FROM CubeCart_inventory"; $results = mysql_query($query); //------------------------------------ // Get data for one product at a time //------------------------------------ while($row = mysql_fetch_array($results, MYSQL_ASSOC)){ $found = 0; //------------------------------------- // Filter to get correct markup variable //------------------------------------- while($row_inner = mysql_fetch_assoc($array)){ if ($row[catalog_price] >= $row_inner[value_from] && $row[catalog_price] <= $row_inner[value_to]) { $markup2 = $row_inner['markup']; $found = 1; } if (found == 1) {break;} } } ?> Depending on many records these tables have, your code has the potential to be very inefficient. If you are expecting either of these tables to have more than a couple hundred rows each, I suggest you give us the table structure, how they relate to each other, and describe your overall goal so we can help you optimize your algorithm. Here is your algorithm as it currently stands: M = SELECT FROM CubeCart_markup_table N = SELECT FROM CubeCart_inventory FOREACH M FOREACH N PROGRAM LOGIC ENDFOREACH ENDFOREACH Here is why it is inefficient. Let M' and N' (pronounced m-prime and n-prime) stand for the number of records in each set. Your code will essentially loop M' x N' times. If M' and N' are relatively small, say 200 records each, then you are already looping 4000 times, which isn't insignificant, but it's not terrible either. But if M' and N' are large, say only 1000 records each (and that's technically not large in terms of what MySQL can do), now you're code is looping 1,000,000 times. You can expect all of your pages that run that code to timeout.
-
@teng84 Don't get me wrong, I understand the difference between wild and domesticated cats, even though a cat is never truly domesticated. Wild cats are scary and dangerous; usually it's not a problem because they will avoid people but when cornered they'll scratch your face off. I can imagine they're even more of a nuisance when they're not afraid to enter your kitchen and steal your food right off the counter. I understand why she was afraid of them. But on the reverse side, I grew up with cats and let them climb on top of my head and bite my nose and stuff. So to see a grown adult (my fiance) trembling while holding a harmless little kitten is still funny, even if her behavior is justified. And for the record, large populations of wild cats are disease carriers; most often such populations are exterminated in the U.S. It just happens so quietly no one really gets a chance to hear about it.
-
My fiance is from the Philippines, where cats are mostly seen as giant, disease-carrying, food-stealing rats; there are many, many stray and wild cats there. When I first mentioned the idea of getting a cat, she rejected it. She continually rejected the idea. Sometimes when we were out I'd take her into a pet store so we could watch the kittens if they had any, but most pet stores in So. Cal. no longer have open pens where you can pet them. My parents live in Oxnard, which is still So. Cal. but a bit further North; once while visiting them my mom said there was a pet store that had a huge kitten pen and you could pet them. So we thought it would be a good idea to take her there so she could hold a kitten; she didn't like the idea but conceded to go along with it. A quick aside: my younger brother is dating my fiance's younger sister; it's a little strange when most people hear it, but it makes family gatherings more fun. So my dad, my mom, myself, my fiance, my brother, and the younger sister are all in the car on the way to the pet store. "We're just going to see the kittens." "No, we're not getting a cat." "I promise." We get to the pet store and go over to the kitten pen, on the way the younger sister is sidetracked by the shih tzu puppies. Immediately she grabs one in a bear hug and her eyes light up. The rest of us continue over to the kitten pen. The gal that runs the pet adoption comes over and we explain my fiance's background and that she'd like to hold a kitten. My fiance looks as if she's about to sky dive for the very first time; her face is complete worry. The lady explains to us there is only one kitten old enough to be held (out of the 20 or so that were there), that she had recently been spayed, and was even ready to go home. So here is my fiance sitting in a chair, the lady sets this kitten down in her lap, and my fiance has no idea what to do. The kitten immediately tries to take off, but I stop it by putting my hand on its chest; I explain to my fiance how to do this. Looking at her face, I see sheer terror and notice that she's shaking; psychologically, she feels like she's holding a rat that just crawled out of the sewer. She pets the cat briefly, holds it a little more, and then gives it over to me; her total time with the kitten, less than one minute. She wants to leave the store. But I haven't held a cat in years, let alone a kitten. So I sit down with it for a bit. My fiance wanders off to find her sister, who is now in a puppy play pen with the shih tzu she had originally grabbed. The lady that runs the adoption comes over and starts talking to me. She says that in order to adopt a pet we have to fill out paper work and it usually takes a day or two to approve people; I tell her we'll be leaving the next day and we live a few hours away. The kitten is put away and I go to find everyone else, still with the puppy. It is very obvious the younger sister is in love with this puppy; my brother, who has always loved dogs, is now playing with the puppy as well. My fiance is distracted with watching her sister and my brother. My mom and I start talking amongst ourselves about the kitten. Unknown to my fiance, I wander off and fill out the kitten adoption paperwork. Now remember, cats are disgusting, vicious, and scary animals in the mind of my fiance. Up until that day, her imitation of a cat was a scratching and hissing animal, "We can't have a cat, it'll rip my face off *HISS* *HISS*" She also always said things like, "I don't have time to take care of a cat," which I always thought funny considering how easy they are as pets. The best one was, "I don't know if I can be a good kitty-mommy!" So I've gone and filled out the paper work to adopt the kitten and am now looking at supplies that we'll need if we're approved; also keep in mind the lady had told me approval takes a day or two. In all honesty I didn't expect what had happened next. So I'm looking at the supplies, pricing things out, and finish. I go back over to the puppy pen where everyone is and my fiance is crying. I mean almost bawling. Tears are all over her face and it's the most miserable I've ever seen her look since we started dating about 4 years earlier. Me: "What the Hell happened?" My Mom: "The lady came over and said you're approved, you can take the kitten home today." Me: "Wow! Baby isn't that great news?" Fiance: "Waaaaaaaaaaaaaaaaaagh!" So now I go back over to putting the supplies into a shopping cart. Eventually my brother joins me, only he's picking out dog stuff. Me: "What's going on?" Him: "Apparently we're getting a shih tzu." Me: "You don't sound happy, I thought you liked dogs." Him: "I do. But all she's going to want to do is hug it; I'll have to do all the work." Before we left the store, we took a couple pictures. We took a picture of my fiance holding the cat carrier with the kitten in it; she is trying very hard to not look seriously depressed. Then we have a picture of my brother holding his new shih tzu and he has annoyed written all over his face. It's impossible to not look at those photos and not laugh. *** Fast forward until the next day and my fiance and I are back home with our new kitten. Our kitten is named Rita because the first thing my fiance did when we got back from the pet store is drink a margarita. We're not sure how trained Rita is, so for the first night we lock her in a big crate with food, water, and a litter box. She doesn't like the crate. She latches onto the door, screams, shakes it, and is thoroughly displeased; this does not help in convincing my fiance that cats are sweet and loving animals. In the morning, I see that the cat has used her box properly. The next evening I figure she can roam loose in the condo. We get into bed, the lights are off, the cat leaps into the bed and runs across my fiance. My fiance screams in terror. "It tried to attack me!" "Baby cats are nocturnal, they play more at night." She lies back down, the cat jumps back into bed, my fiance is trembling. After 10 minutes of this, "I can't do it she can't stay in our bedroom." I put the cat outside our bedroom door and shut the door; she sits at the door and screams, she shoves her paw under it and tries to open it. Clearly this kitten does not like to be left alone. The next evening we let the cat into our room and make it through the night. My fiance didn't sleep very well, but she did better. Eventually my fiance is no longer terrified of the cat. She pets it, picks it up, and plays with it using cat toys. She still thinks I'm crazy for letting it bite and scratch my hand; "Doesn't that hurt?" "lol no, it tickles." It took about two weeks for my fiance to work up the courage to tickle the cat's belly until it bit her. *** Fast forward a few weeks, my fiance has gone to a coworker's baby shower. There is a cat there that bee lines for my fiance. Apparently the cat thinks she's a cat person. *** Almost two months later, my fiance has warmed up to the cat. She's starting to admit to herself how wildly wrong she was about them and that she might even be a cat person. We're buying stuff at PetSmart and look at the cats they have. We see these two snowshoe kittens that are 10 weeks old. My fiance immediately decides that one of them is absolutely adorable. We leave the store. Throughout the next couple of days we talk about possibly adopting it. Eventually I decide to do it, make the appointment, and pick the cat up on my way home from work. I get home late, my fiance has been wondering what I was up to, and is happy when she sees the cat. "I was hoping you were adopting him." *** Now she loves them. She buries her face in them and kisses them and Bandit (the lighter cat) is always on her lap. Rita (the charcoal) is more independent, although she doesn't like to be left alone. Rita is not cuddly but she will sit near you and follow you around. Whenever the younger sister comes over, she is not scared of the cats. When my fiance's older sister visits us, she is still terrified of the cats and screams whenever they get near her; my fiance now recognizes how silly that behavior looks over a cat. Long story!