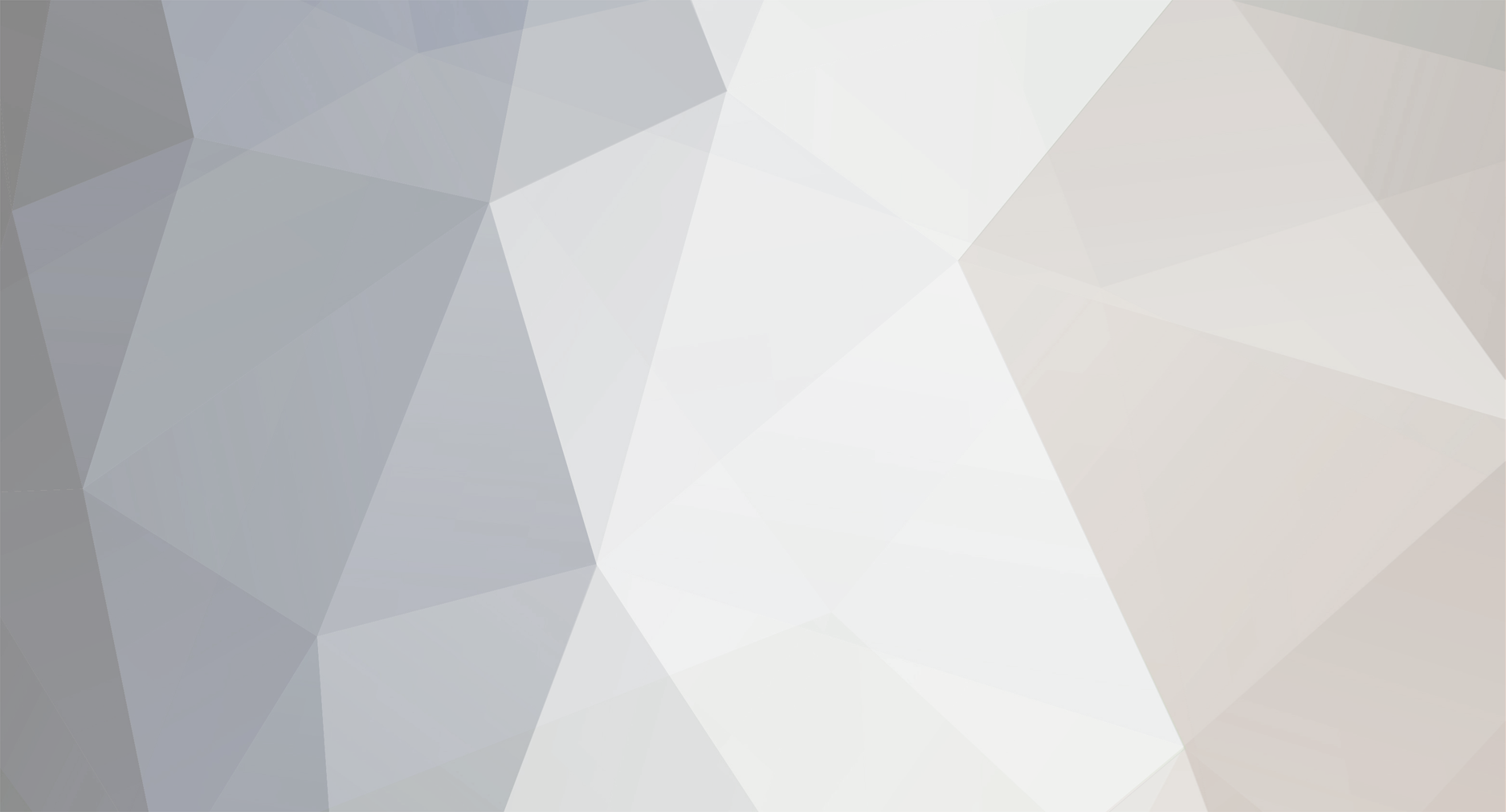
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I worked in a college computer lab as part of my work-study program. One day while working in one of the mac labs I was just walking around and I noticed a lot of people were typing into really small word processor windows, like 400 x 400 or smaller. Most of them didn't know you could resize it.
-
It looks as if move_uploaded_file() is trying to move the file to another server. Your script is in: /home/runningp/public_html/members/ and your images directory is in: /home/runningp/public_html/members/images/ Try this: if(is_uploaded_file($_FILES['image']['tmp_name'])){ // set destination directory $dst = realpath(dirname(__FILE__) . '/images') . '/' . get_username($_SESSION['user_id']); if(move_uploaded_file($_FILES['image']['tmp_name'], $dst)){ // Success, file has been moved and renamed // now do whatever else is necessary } }
-
A parser is just a FSM that returns tokens based on syntax rules. They're already very easy to write. I really can't see how error checking would be made any easier: if($bad_thing_happened){ goto err_label; } // hundreds of lines of code err_label: echo "An error occured!"; vs. if($bad_thing_happened){ // throwing an exception // calling a error handler function // etc. }
-
I don't know. The way you go on about optimizing and not using white space because it's a waste of bytes, I would have thought you'd have been against a text string (the label) that doesn't perform any calculations.
-
Since NULL is not equal to NULL, it also disobeys UNIQUE indexes in most of the engine types. Declaring a column as able to contain NULL will also use a small amount of extra storage for that column.
-
Call mysql_query() inside the loop after assigning to $sql; that will make multiple calls to the database though. With a small amount of cleverness you can do it with a single call. This will insert three rows into the table `table`. INSERT INTO `table` (`col1`, `col2`) VALUES ('a', 'a'), ('b', 'b'), ('c', 'c')
-
Hmmm...I don't think I like the break label thing either; I hope they don't implement it. I will concede that there may be rare occasions where it might be useful, although I myself have never thought, "Hey I could really use a break label right now." The problem is that you can't prevent programmers from using it where it's not appropriate, which will lead to abusing the use of the construct and sloppy code will follow anyways.
-
There is no reason to use goto. Ever.
-
Another thing I'd like to add. I'm of the mindset that you get what you pay for, but when it comes to any sort of optical drive I buy the cheapest ones possible. I once spent a lot of money for two separate CD burners that worked great at first, but eventually went to crap. So next time I bought one I said screw it and dumped $20 on an OEM NEC (and later an Asus) DVD Burner. They still work great.
-
http://www.php.net/features.file-upload Scroll down to where it talks about $_FILES. Essentially, $_FILES is an auto-global like $_SESSION or $_SERVER. When you have an input of type="file" in an HTML form, $_FILES will have an index with the same key as the name attribute of your input. For example, you have: <input type="file" name="image"><br><br> Thus there will be a variable $_FILES['image'] that contains data about the image upload. Try adding this to your script in the processing portion: echo '<pre style="text-align: left;">' . print_r($_FILES, true) . '</pre>'; While $_FILES has a decent amount of information packed into it, I will warn you in advance that you should not trust the 'type' index. This is provided by the browser and can easily be faked.
-
Are you familiar with $_FILES?
-
You just change the property loop to include cases for: is_bool is_string is_int, is_float is_null etc. and print the appropriate thing for each one. The only reason you have to do this BTW, is because you want to make sure strings are escaped, null usually prints blank, etc. I noticed on mine I left out printing the <?php and ?> tags, nice catch. But on yours, did you forget a backslash in here: echo("<?phpn");
-
Can you post your current code? As well as the line where you are setting the $image variable? It seems your code thinks $image is pointing at a directory
-
Nah. I work for a small company and maintain a php / mysql website with a bit of fancy javascript thrown in, although the current user base is relatively small. I've been told I should consider a career in teaching on many occasions though, or a comedian, psychologist, or one of a few other things I can't think of right now. The one career no one has ever said I should consider is computer programmer, imagine that. I'm a nerd at heart, but I fool almost everyone into thinking I'm not!
-
You could try the doomsday algorithm.
-
What's the output from mysql_error()?
-
I'm going to assume that $image is the uploaded file then. If that's the case, don't use the copy() function. Use move_uploaded_file(). If you reread my first post in this thread:
-
[SOLVED] Incremented Variable Problems... BIG ones.
roopurt18 replied to vozzek's topic in PHP Coding Help
I used to do things the way you were doing them, by appending the ID or whatever to the end of the name. I did it that way for a very long time. Then one day while working with a select with multiple="yes" I realized something, even though I'd used them before: $_POST can contain arrays! So unbelievably simple and yet so hard to miss. Anyways, since then I always write my name attributes in such a way that the incoming data in $_POST is already attached where ever it needs to be. At least as much as possible; sometimes it's just simpler to do it the other way. Also, I notice you have an id attribute on your inputs. Are you using any Javascript? If you aren't, then you can safely drop the id attributes. If you are, I can show you another "trick." -
[SOLVED] Incremented Variable Problems... BIG ones.
roopurt18 replied to vozzek's topic in PHP Coding Help
With your current set up, you could do this: <?php foreach($_POST as $key => $val){ if(substr($key, 0, 3) == 'qty'){ // quantity field, get the id $id = str_replace('qty', '', $key); // get the matching hidden id field $hidden = $_POST['hidCartId' . $id]; // Now $hidden is the hidden field and $val is the qty field // Do whatever } } ?> The better approach is to name your inputs so that you can do away with this altogether: <?php echo "<input type='text' size='1' maxlength='2' id=qty".$itemct." name='qty[{$row['ct_id']}]' value=".$row['ct_qty']." />"; ?> This will cause $_POST to have an array named 'qty'. Each entry in $_POST['qty'] will be of the form 'ct_id' => 'qty'. Basically you are putting the id field directly into the input's name attribute, so you no longer need a hidden field and you no longer need to match them up. <?php // processing foreach($_POST['qty'] as $id => $qty){ // $id is the id and $qty is the quantity, much easier! } ?> -
Eh that's more than I can help with at the moment. Your best shot is to ask in the CSS forums. The other thing you can do is use FireFox with the web developer and firebug extensions. Firebug will let you inspect page elements and the attached styles while web developer will let you edit your CSS on the fly. It's easier than editing the file, reloading the page, etc.
-
What's the CSS problem?
-
What are the possible values for `erfasst`? If the only possible values are say "fake" and "real", using ORDER BY `erfasst` will put the fake ones before the real ones. Did you see this part of my post:
-
Well, it doesn't help me! But I don't do this for personal gain, so sure, help the community instead.
-
The error is complaining about line 31: if (!empty($image)){ I do not see $image being used before that line. Place this before your current line 31 and tell me what it outputs: echo '<pre style="text-align: left;">' . print_r($image, true) . '</pre>';
-
Remember that PHP primarily outputs text. Just because we mostly use PHP to output [X]HTML doesn't mean there's any reason we can't use PHP to output other types of plain text, including a new PHP file. write_config.php <?php /** * write_config.php * This PHP file outputs a new PHP file that defines a CConfig object. class CConfig { var $property1 = 'a value'; var $property2 = 'another value'; var $property3 = true; // etc. } * In order to do this, the file expects a $properties array where * 'property_name' => 'config_value' */ // First we must detect that $properties is an array and contains values if(empty($properties)){ return; } // Begin our class echo 'class CConfig {' . "\n"; // Here we loop over each value and convert it into something we can place // into a class definition. For simplicity's sake, I'm only handling bool // properties, anything else will just be skipped. You can work on that as // an exercise of your own. foreach($properties as $prop => $val){ if(!is_bool($val)){ continue; // only handling bools } echo 'var $' . $prop . ' = ' . ($val ? 'true' : 'false') . ';' . "\n"; } // End our class echo '}' . "\n"; ?> sample script <?php /** * Here is a sample of how to exec our write_config.php */ $properties = Array(); $properties['require_login'] = true; $properties['write_logs'] = true; // Next property is a string, so will be skipped, you'll have to modify // write_config.php to make it work. $properties['board_name'] = 'Developer\'s Board'; // We can execute the file by include()'ing it, but that will cause it to // write output to the screen. So we will capture it in a buffer ob_start(); include('write_config.php'); // this writes to the buffer $file_contents = ob_get_contents(); // this gets buffer contents ob_end_clean(); // this ends and erases buffer // $file_contents now contains our class definition, we can write it to // a file // file_put_contents is PHP 5, use fopen, fwrite, and fclose if php4 file_put_contents('config.php', $file_contents); ?> That should help.