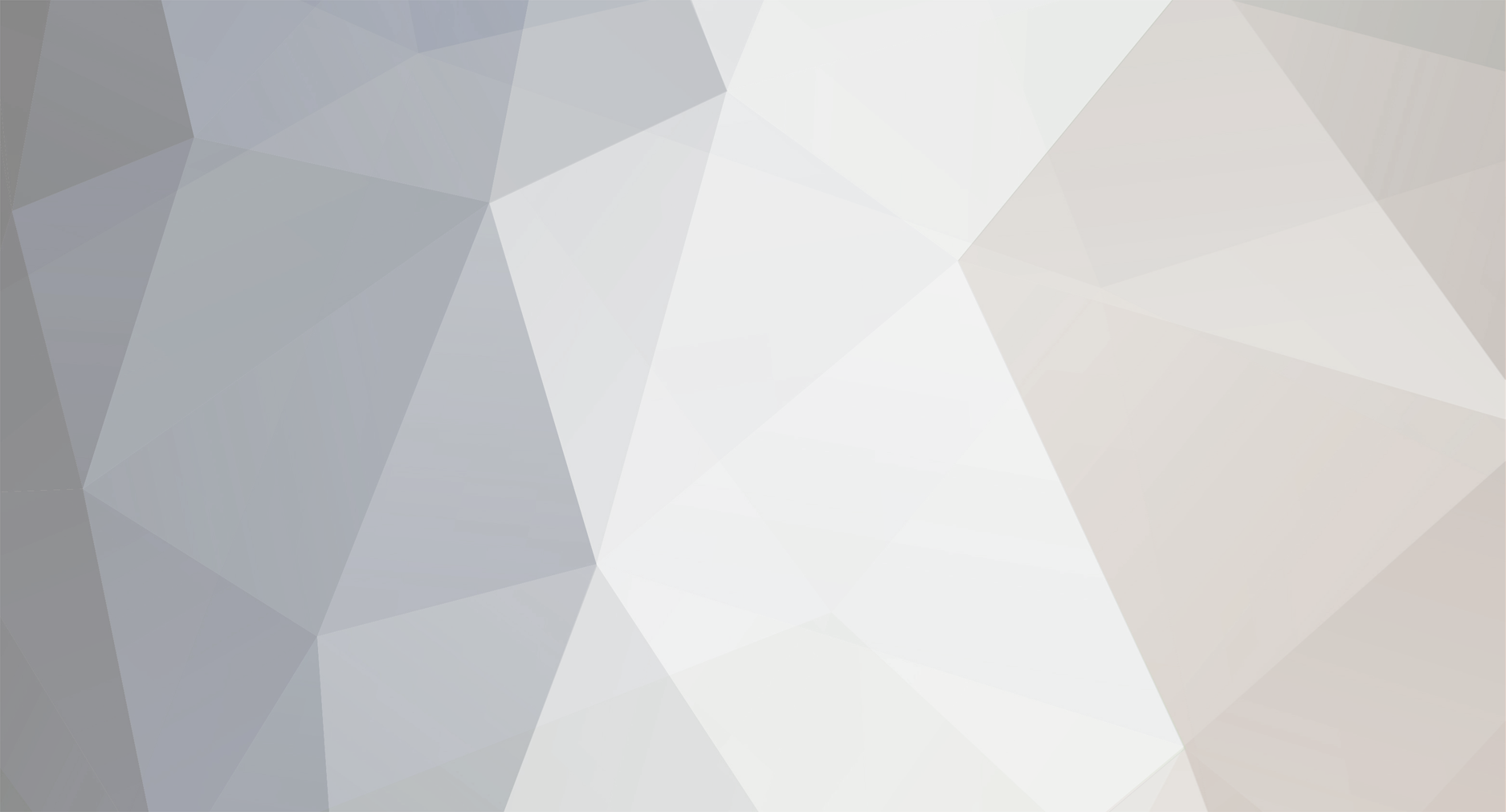
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
You forgot the z. tar xzf extracts a gzip'ed tar file, the p just preserves permissions. Show offs!
-
If the only options are yes / no, it makes more sense to use a checkbox for your input. If you manipulate the name attribute of your form inputs correctly, then you can coerce $_POST into a format that's easier to work with on the server. Your form should look like this IMO: <form method="post" action=""> <p> <input type="checkbox" name="tasks[1]" value="1" /> finished_homework </p> <p> <input type="checkbox" name="tasks[2]" value="1" /> cleaned_my_room </p> <p> <input type="submit" name="Submit" /> </p> </form> When you process that form, $_POST will have an index 'tasks' which will be an array. Each item in the array will be of the form task_id => '1' if the task was done, otherwise it won't appear in the array. You can then create the values to insert into the DB very easily with: <?php foreach($_POST['tasks'] as $task_id => $val){ // assumes $user_id is set elsewhere // you MUST sanitize $task_id and $user_id, $val is unimportant $sql = " INSERT INTO `task_user` (`task_id`, `user_id`, `created`) VALUES ( {$task_id}, {$user_id}, NOW() ) "; } ?> That's not really optimal code (or how I would code it anyways), it's just meant to give you an idea.
-
You are determining which date (the DB date or today's date) to use based on another database field: erfasst. You can just put that field in the beginning of the order by clauses: if($sm == 'or') { $qs = "select * from job_post ".(($sch)?"where ".join(" or ", $sch):"")." order by erfasst, EXyear DESC, EXmonth DESC, EXday DESC limit $Start,$ByPage"; $qss = "select * from job_post ".(($sch)?"where ".join(" or ", $sch):"") . " order by erfasst, EXyear DESC, EXmonth DESC, EXday DESC"; } elseif($sm == 'and') { $qs = "select * from job_post ".(($sch)?"where ".join(" and ", $sch):"")." order by erfasst, EXyear DESC, EXmonth DESC, EXday DESC limit $Start,$ByPage"; $qss = "select * from job_post ".(($sch)?"where ".join(" and ", $sch):"") . " order by erfasst, EXyear DESC, EXmonth DESC, EXday DESC"; } If that doesn't work, replace erfasst with erfasst DESC.
-
Have you still not fixed the error? If not, then read the error: Parse error: syntax error, unexpected T_STRING in /home/runningp/public_html/members/upload.php on line 37 These are the key parts: unexpected T_STRING upload.php line 37 Here is line 37: $filename1 = "./images/" get_username ( $_SESSION['user_id'] ); You forgot the . between "./images/" and get_username $filename1 = "./images/" . get_username ( $_SESSION['user_id'] );
-
The form where you update the settings likely generates a config.php. At least, that's probably how I'd do it.
-
Try changing this line: addListener(obj, function() { self.doSomething(i); return; }); to: addListener(obj, function() { var save_i = i; self.doSomething(save_i); return; });
-
Your Desktop directory in Ubuntu is very likely: /home/<username>/Desktop move those *.tar.gz files to the other directory mv /home/<username>/*.tar.gz /usr/local/src/ uncompress those files gzip -d /usr/local/src/*.tar.gz untar those files tar -xvf /usr/local/src/*.tar However, if you are trying to set up a LAMP server, you can save yourself some trouble and just use synaptic. It's oodles easier.
-
I was under the impression that you had a `task` table full of all the possible tasks? Is this not the case? Can you use phpMyAdmin to export your `task` table? Or copy and paste a sample of the data contained within?
-
Your best bet would probably be to store each of the $dayXX variables as minutes, rather than HH.MM. To do that: list($h, $m) = explode('.', odbc_result($queryexe, 1)); $day01 = $h * 60 + $m; Then instead of comparing to 70 for the violation, compare to 70 * 60 (the amount of minutes in 70 hours). As for the 7 consecutive days changing to 8, just change the bounds on the loops.
-
So does that mean the violation occurs if any X consecutive days totals more than Y hours?
-
When selecting data from the database, you can tell MySQL to order it for you with ORDER BY. Try changing these lines: to: if($sm == 'or') { $qs = "select * from job_post ".(($sch)?"where ".join(" or ", $sch):"")." limit $Start,$ByPage"; $qss = "select * from job_post ".(($sch)?"where ".join(" or ", $sch):""); } elseif($sm == 'and') { $qs = "select * from job_post ".(($sch)?"where ".join(" and ", $sch):"")." limit $Start,$ByPage"; $qss = "select * from job_post ".(($sch)?"where ".join(" and ", $sch):""); } to: if($sm == 'or') { $qs = "select * from job_post ".(($sch)?"where ".join(" or ", $sch):"")." order by EXyear DESC, EXmonth DESC, EXday DESC limit $Start,$ByPage"; $qss = "select * from job_post ".(($sch)?"where ".join(" or ", $sch):"") . " order by EXyear DESC, EXmonth DESC, EXday DESC"; } elseif($sm == 'and') { $qs = "select * from job_post ".(($sch)?"where ".join(" and ", $sch):"")." order by EXyear DESC, EXmonth DESC, EXday DESC limit $Start,$ByPage"; $qss = "select * from job_post ".(($sch)?"where ".join(" and ", $sch):"") . " order by EXyear DESC, EXmonth DESC, EXday DESC"; }
-
I'll admit I'm not up to speed on developing sites for hand-helds or other small displays, but here's my advice. You can be fancy and detect the browser via Javascript or PHP (via $_SERVER) as suggested, but some people will have Javascript turned off. Others might have their browser configured to tell sites it is a different browser (which would render PHP's ability to correctly identify the user agent in $_SERVER useless). So no matter what automatic detection you decide to use, it may fail, and you should still provide a 'Reload this page for a small display or hand-held device' link. No matter how you determine to display your alternate .css (automatic detection or user action), set a flag in $_SESSION that tells the site to use the alternate .css. Do it in $_SESSION because you can still use sessions even when cookies are disabled. Additionally, set a permanent cookie in the user's browser that says to use the alternate style sheet. On your visitor's next visit to the page, look for the permanent cookie and if it's present use the alternate stylesheet. If it's not present, revert back to your automatic or user specified action.
-
SHOW TABLES Will return all of the tables in a database. So you initially do that and build a link for each individual table, something like: http://www.domain.com/viewtable.php?table=table_name In viewtable.php, you get the table name from $_GET['table'] and run the query: SELECT * FROM {$_GET['table']} DO NOT FORGET TO SANITIZE ANY DATA BEFORE PUTTING IT IN THE DB, MY CODE IS JUST A SAMPLE, NOT MEANT TO BE USED AS IS. Since the columns will vary depending on which table is selected, when displaying the table you can do: $q = mysql_query("SELECT * FROM {$_GET['table']}"); if($q){ echo '<table>'; $bHdrs = false; while($row = mysql_fetch_assoc($q)){ // This IF will print the table headers on the first row encountered if(!bHdrs){ $bHdrs = true; $tmp = Array(); foreach($row as $k => $v){ $tmp[] = $k; } echo '<tr><td>' . implode('</td><td>', $tmp) . '</td></tr>'; } echo '<tr><td>' . implode('</td><td>', $row) . '</td></tr>'; } echo '</table>'; } Again, my code is just a sample. Remember to sanitize your data before using it in queries!
-
Out of curiosity, can you post that script?
-
Instead of 31 variations of the variable $day, next time use a single array named $day with 31 keys (0 through 31). It does simplify this sort of thing a little bit. Anyways, here you go: <?php for($i = 1; $i <= 25; $i++){ $total = 0; for($j = 0; $j < 7; $j ++){ $n = sprintf("%02d", $i + $j); $total += ${'day' . $n}; if($total > 70){ break; } } if($total > 70){ // VIOLATION } } ?> That's the inefficient, brute force method. (edit) Outer loop should be less than equal to 25, not 24 as in my first post.
-
The person you are changing the code for, do they wish to have click-able table headers that will cause the table to be sorted by that column in ascending or descending order?
-
[SOLVED] Problem With require() and header()
roopurt18 replied to centerwork's topic in PHP Coding Help
This is most likely correct. The "complete" db_config.php you posted is only 6 lines long, yet the error is referencing line 11 of db_config.php. Are you sure you have absolutely no spaces or newlines before the <?php or after the ?> in db_config.php? -
If you're not going to bother using code tags then I'm not going to bother reading your code. But, my browser's search function would indicate you don't have a single ORDER BY in your queries.
-
The problem is the column's data type. TIME is a 24 hour format that looks like: HH:MM:SS. If you look back at the information from the debugging statement earlier, attack_time is equal to 19:04:21. Using just that and the $attack_limit you echo'ed as example data, your SELECT statement looks like this: select * from attack_timers where attacker_id='$account_id' AND '19:04:21' > '1196281486' My guess is that MySQL is comparing these values as strings, not as timestamps. Rather than compute the timestamp in PHP, let's do it in MySQL: select * from attack_timers where attacker_id='$account_id' AND attack_time > (NOW() - INTERVAL 60 SECOND)' There are two reasons to do this. The first is that it cleans up your PHP; why do work intended for the database in PHP when you can just perform it in the database itself. The second is more subtle. There is a good chance your MySQL server and apache server are on the same computer, which means time() in PHP and NOW() in MySQL should return the same values. But what happens if the servers are separated to two separate computers, time() in PHP and NOW() in MySQL may no longer return the same value. Uh oh!
-
That simple debugging statement tells us several things. First is that up until that point, it appears your code works. (Although you should always confirm that's the record you expected to be pulled from the database). Second is that right before the if($row == '0') statement, we know that $row is an array. That would explain why your else condition is always triggering. Looking more carefully at your code, you made a simple logic mistake. In your SELECT statement, you are asking the DB to give you any records from this user within the last 60 seconds. So far so good. You test the result in an if, again so far so good. You then grab the number of rows returned in the result set; again so far so good. Then you use mysql_fetch_assoc() to get the first record in the result set, but why? You don't need it. You've already asked the database for the records within the last 60 seconds and then you asked how many there were, that's all the information you need. If there's zero records, you can process the user's data. Change: $num_rows=mysql_num_rows($result_1); $row=mysql_fetch_array($result_1); if ($row == '0') to: $num_rows=mysql_num_rows($result_1); if ($num_rows == 0) You should also note that in your SELECT query in the beginning, the most records you should ever be able to pull is 1; you can therefore place a LIMIT 1 on the end of the query. On the other hand, you may not want to limit the query because a result set larger than 1 record would be indicative of either a program bug or cheating, and you can have your script notify you (the admin, not your users) if this happens. Also, just for curiosity's sake, what's the column data type for attack_time? If its DATE, DATETIME, or TIME, you may have a bug on your hands.
-
Make sure you're ordering by sched_date and sched_time in the schedule table and NOT the gdate and gtime fields you created, as they are formatted and will be sorted as text. If you're using phpMyAdmin, if you click on the database name so that all of the tables are listed in the content frame, there is an export tab. You can choose to export the two tables (and only the two tables, I don't care about your other data) into a .gzip file and attach them to a post (or send them to me via IM). That's only if you want it done in a single query.
-
Which PHP-Editor do you think is the best?
roopurt18 replied to briananderson's topic in Miscellaneous
Some time ago I knew the basics of emacs, but I can't do crap in it now. Vi is what I use now when forced to edit files in a terminal, simply because I remember the basic commands from when I was first introduced to it years ago. I think the thing that makes Vi difficult, and possibly Vim, is the idea of jumping in and out of text-editing mode. It seems so unnatural if you grew up in the windows world, but you do get used to it. I use Zend for work by the way. It's great except for one minor quirk, which irritates the Hell out of me. It has back and forward buttons on the toolbar which would be awesome if they just cycled through files in the order you viewed them. But instead they go through every. single. cursor. position. If you just type out a string, you do not want to click a button 30 times to get back to the previous file you were editing. If the tabs for open files never changed positions, it wouldn't be half bad because you would always know where the file's tab was, but they move around. If there was a keyboard shortcut to jump back and forth between two files it would be forgivable as well (editplus has this feature and I love it). I use eclipse at home and it runs great under linux; it was very sluggish under windows. The only complaint I have with it is the intellisense (yah I know thats the MS version, I can't think of the other name right now) doesn't close after certain character strokes, like } ), etc. What happens is I type a variable name in an if statement, the popup list appears, I finish typing the variable and close the if statement, but the list is still there. Then because I'm not longer editing a variable or function name, the list goes to the beginning and I have to remember to hit escape before I hit enter or it plugs an unwanted bit of text into my code. Then I have to go back and delete it before I can continue typing. So far the best IDE I've ever used is the one that came with MS Visual C++ 6.0. God I miss that IDE. ARRRRRRRRRRRRRRRRRRRRRRRRRRRRRRGH! -
The change I suggested merely prints debugging information. If you copy and paste that information it will help determine what to do next. Debugging is a simple process that somehow eludes a lot of people. When your program doesn't work, the only way to fix it is to make it give you meaningful information about why it's not working. You're some of the way there already, as you're printing mysql_error() when things fail.