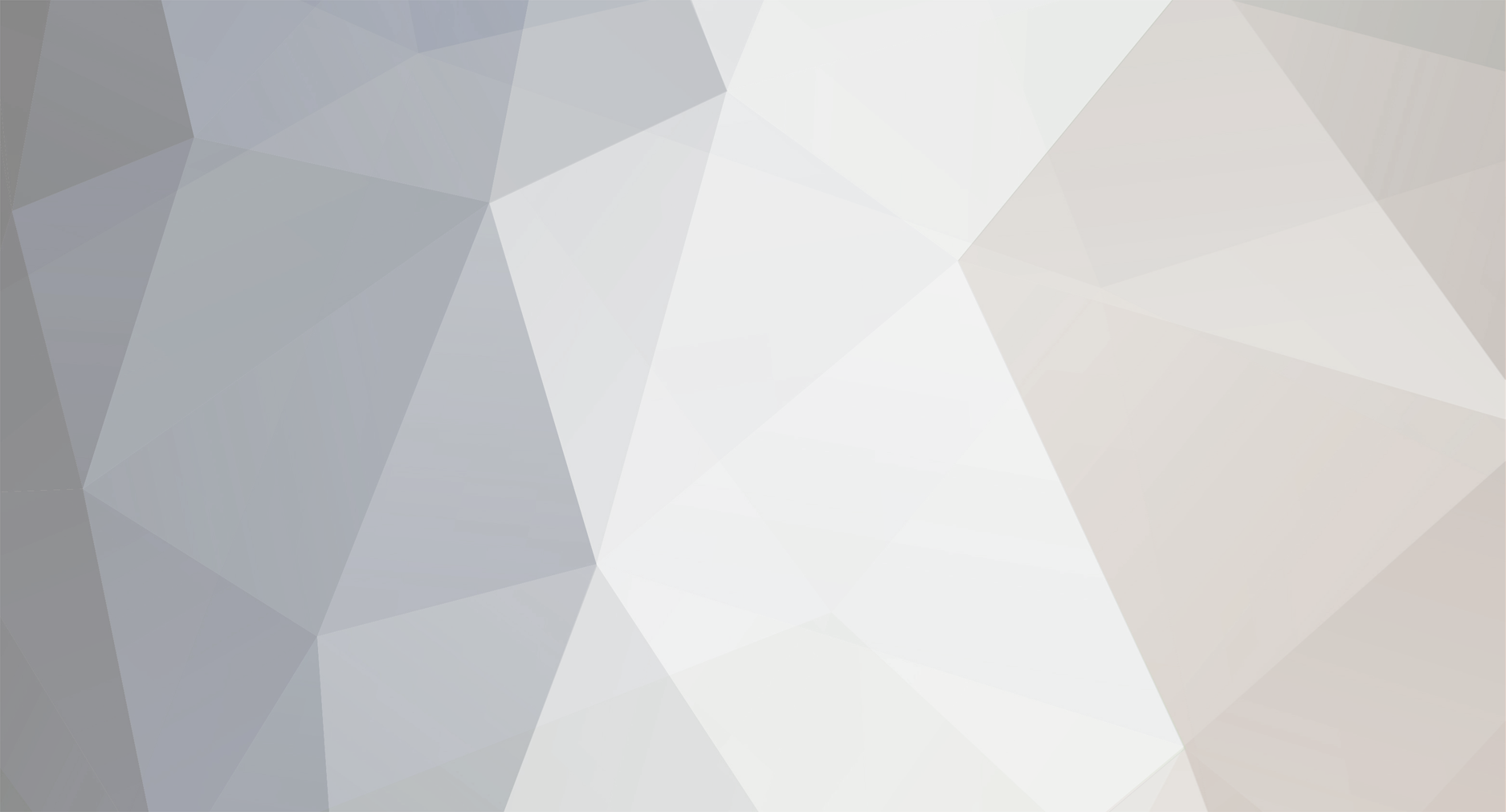
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Is there any reason you didn't just use __set & __get instead of __call for those types of methods. I can think of one, but I'm just curious as to the actual reason.
-
If I were to create such a device, I'd probably implement it like so: <?php class Loader { /** * Static var holds instantiated singletons for use in the site * 'className' => objectInstance */ private static $s_Singletons = null; /** * Magic method __call * Functionality depends on called name * getSingleton[Classname] - returns instantiated singleton * $a acts as constructor args * getInstance[Classname] - returns instantiated object * $a acts as constructor args * @param string called function name * @param array function arguments * @return mixed */ function __call($m, $a){ // Determine if we're instantiating an object $bSingleton = strpos($m, 'getSingleton'); $bInstance = strpos($m, 'getInstance'); if($bSingleton !== FALSE || $bInstance !== FALSE){ // Instantiating an object - get object name $name = $bSingleton !== FALSE ? str_replace('getSingleton', '', $m) : str_replace('getInstance', '', $m); // Actions differ depending on singleton or instance if($bSingleton !== FALSE && array_key_exists($name, self::$s_Singletons)){ return self::$s_Singletons[$name]; } $obj = new $name($a); if($bSingleton !== FALSE){ self::$s_Singletons[$name] = $obj; } return $obj; } } } ?> I left out error checking and other fancy features, like require_once()'ing the class file (if you're not using auto_load). Anyways, I'm sure that method adds some overhead, but it eliminates a lot of similar functions IMO and still gives you flexibility to use an existing object (like using an existing mysql connection) or requesting a new one. Opinions welcome.
-
Deep thought: is there such a thing as a completely new idea?
roopurt18 replied to neylitalo's topic in Miscellaneous
New ideas do occur but for the most part everything we have is just an extension of what already existed. Here are some things I consider to be new ideas: zero (or nothing), [non-]existence of powerful god(s), calculus, certain advancements in physics (theory of relativity, quantum mechanics, etc) Those are all items that to some extent were built on previous concepts, but required radically new thinking to carry the concept further. Things like computers, various machines that fly, are all innovations of previous technology, so aren't really new ideas. They're just more efficient methods of completing tasks that are thousands of years old. Also, IMO, human beings are fairly limited in their capacity for thought in that we all draw on the same resources for our thought process. Thus, any time one human has had an idea or innovation, there is a good chance someone else has thought of it before. Two classic examples: The European development of calculus - http://en.wikipedia.org/wiki/Calculus#Development Steam Power - http://en.wikipedia.org/wiki/Aeolipile -
You need to first research what image resizing capabilities are supported by PHP; for this, use Google. Next, try to get it working yourself. Start a new thread if you run into troubles with just the resizing as it's a new topic. Good luck.
-
Your code never returns zero for me. I see you have a curly bracket in there; I'll wager that curly bracket goes with an if that never gets entered.
-
I suspect that $q will always be set, since it is capturing the value of mysql_query(). You should run a test for: if(file_exists(...))
-
Essentially, yes this is what I meant. I have a bit more time today than I did yesterday, so this may get long, but here we go. In OOP you first must declare your object; you do this by writing the source code. Think of this like creating a blue print for a house. // Declares an object, shape // (not a very useful object though) class Shape { } There. I have just declared my object 'Shape.' However, I do not yet have a shape anymore than creating a blue print means I have a house. After you declare your object, you must instantiate (create an instance of) it. This is like building the house from the blue print. // elsewhere in the code, we instantiate // this is out-dated php4 syntax, php5 drops the & operator $obj = &new Shape; After that line of code, $obj is a variable that refers to an instance of the object. Since our declaration doesn't have any methods or properties, our instantiated object isn't of much use. For example, our declaration (the blueprint) doesn't include a Draw() method, so it's impossible to say: $obj->Draw();. Just to be clear: method : a fancy name for a function, just like any other program function you can write, that happens to belong to a class property : a fancy name for a variable, just like any other, that happens to belong to a class Now for a quick aside: You are familiar with the terms client and server, although up until now you typically think of computers over remote connections. You can essentially think of an instantiated object as a server and the code that uses it the client. So to answer your question, the interface is the set of methods and properties the object (server) exposes to the code that uses it (client). Typically, the interface consists entirely of methods. Methods do not necessarily have to access any of the properties, although they usually do. Now, it is possible that properties of the object will be part of the interface, as in this example: $obj = &new A; $obj->a = 'Hello'; // as opposed to: $obj->set_a('Hello'); You have to be very, very careful when doing this. You have to almost guarantee that property will never change in name, type, restrictions, constraints, etc. for all of eternity. This does happen in practice; a good example are the nextSibling and previousSibling properties of DOM nodes in Javascript. You will notice in PHP5 and other OOP languages there are reserved words: public, protected, and private. When you start using those keywords they define the level of visibility attached to a particular method or property. class A{ private $a; public set_a($val){ $this->a = $val; } public get_a($val){ return $this->a; } } // elsewhere in another file $obj = new A; // PHP5 now so I dropped the & $obj->set_a('Hello'); // works as expected $obj->a = 'Hello'; // a is declared private, can't be seen outside the class Above, because the property $a is declared private, that last line of code won't work. Not only that, the PHP interpretor is able to throw an error (since the interpretor read the definition and saw the private keyword). When using public, protected, and private in a class declaration you guarantee that the clients (code that uses it) adheres to the interface you intended. PHP4 has no such public, protected, and private reserved words. Therefore any method of a class is public, likewise with any variable. Due to this, even though PHP4 supports classes and objects, I wouldn't call it object oriented since it doesn't give the object designer the ability to enforce their interface. Think about what would happen if a PHP4 application constantly used what was meant to be a private method of a class. The server is then upgraded to PHP5. Now that the server runs PHP5, the private keyword is added to the function in the class declaration. Now all those calls that worked in PHP4 break. Oops.
-
Help with seperating data within MYSQL Fields???
roopurt18 replied to 00falcon's topic in Regex Help
Did you bother doing any research into what normalization is? -
I think his $_SESSION['user_id'] is the username, not the id field we all think it is... runnerjp, everyone has given you everything you need to get this working. You need to make some effort to connect the dots.
-
NP. Taking everything one step further, when writing OOP code you should be thinking in terms of interfaces. I'm not going to bother with a long, detailed explanation, you seem resourceful enough to find some of this on your own, but I'll try and get you thinking. Let's say you develop an object that acts as a session handler. You should be thinking of all the different ways in which sessions are used and provide the proper interface for your session object. What is an interface? The interface for the object is the functions and methods that expose it's functionality. There is a good chance your object will have properties (member variables), but any code that uses the object should not access those properties directly; instead it should access them via methods. Short example: class A { var $a; function set_a($val){ $this->a = $val; } function get_a($val){ return $this->a; } } $a = &new A; // DO NOT DO THIS $a->a = 'Hello!'; echo $a->a; // This is better $a->set_a('Hello'); echo $a->get_a(); Here's why the first example is bad. Say you develop that object. Again, you use it all over the place in your code. You later discover that the internals of the object should be redesigned, that internal variable 'a' should be an Array. Or you want to restrict it so that only numeric values can be set there. Well, if you were accessing that variable directly, you have a lot of code to change. If you were using the set* and get* methods, then you have very little code to change. When designing an interface, it boils down to this. As long as all client code (i.e. code that uses the object) respects and uses the interface, you can change the internal workings of your object all you want and all of your client code will continue to function. Hmmm. That wasn't as short as I intended.
-
Here is a website I frequently refer to when creating tricky regexps: http://www.regular-expressions.info/ There also exist sites where you can type a subject string in one field and a regexp in another and it will highlight the matching part; that makes it easy to test your regexp without having to edit code, load page, repeat. One tip for creating long regexps, separate them into more than one variable. For example: <?php // Create a regexp to match an e-mail -- THIS IS NOT A WORKING EXAMPLE, IT ONLY ILLUSTRATES USING SEVERAL // VARS TO CREATE A SINGLE REGEXP, WHICH INCREASES READIBILITY (sometimes) // An email is: <localpart>@<domain> $local = '...'; // part of the regexp that matches local part $domain = '...'; // part of the regexp that matches domain $email_regexp = "/{$local}@{$domain}/"; // Now we could easily create a regexp that accepts multiple emails separated by commas or semicolons $mult_email_regexp = "/({$email_regexp})([,;][ ]*{$email_regexp})*/"; ?>
-
Help with seperating data within MYSQL Fields???
roopurt18 replied to 00falcon's topic in Regex Help
PFM is describing the process called normalization. You may want to google it. -
From your example file. $query = "SELECT schedule.id, schedule.gig_date, schedule.start_time, schedule.end_time, location.name FROM schedule, location WHERE schedule.gig_date >= CURRENT_TIMESTAMP AND schedule.p_location = location.id ORDER BY schedule.gig_date"; Today that is the only file that uses that query. What if tomorrow you need to pull that same information on another page. No problem, you copy and paste the query, right? The day after that, you change the table structure, so now you have to change the queries. It's only two files and two queries, so it's not a huge deal, right? Now pretend your project is massively complex and encompasses several thousand (or several hundred thousand) lines of code. Those types of changes are not easily accomplished. What it boils down to is application design is about duplicating as little code as possible. If you have 10 files that all run the same query and there's a problem with the query, you have to fix 10 separate files. If instead you have 100 files that all call the same function in another file, and you find a bug in that function, you fix it in one place and you've fixed it in all of them.
-
Do you understand what $_GET is? How it works? And how it is used? If you dont, I suggest you start here: http://whn.vdhri.net/2005/10/how_to_use_the_query_string_in_php.html
-
It won't work because the URL looks like this: http://www.runningprofiles.com/members/test Where if you're going to use $_GET it needs to look like this: http://www.runningprofiles.com/members/test?id=<users_number_id> So either you change the URL or you change the script to not use $_GET. I recommend changing the script to not use $_GET since you are already passing the username into it.
-
What does the URL look like?
-
What does this print: <?php session_start(); require_once '../settings.php'; // see if a name was passed via the url. if (isset($_GET['username'])) { $username = mysql_real_escape_string($_GET['username']); } elseif (isset($_SESSION['username'])) { $username = $_SESSION['user_id']; } else { die("No profile to search for"); } $query = "SELECT * FROM users WHERE Username = '$username' LIMIT 1"; if ($result = mysql_query($query)) { if (mysql_num_rows($result)) { $array = mysql_fetch_assoc($result); $pemail = $array['Email']; $puser = $array['Username']; } else { echo "No users found with id $id<br />"; } } else { echo "Query failed<br />$sql<br />" . mysql_error(); } echo '<pre style="text-align: left;">id: ' . $_GET['id'] . '</pre>'; $sql = 'SELECT `ext` FROM `user_image` WHERE `user_id`=' . $_GET['id']; $q = mysql_query($sql); echo '<pre style="text-align: left;">sql: ' . $sql . '</pre>'; echo '<pre style="text-align: left;">q: ' . $q . '</pre>'; echo '<pre style="text-align: left;">error: ' . mysql_error() . '</pre>'; if($q){ $row = mysql_fetch_array($q); echo 'emma'; if(!empty($row)){ echo '<img src="http://www.runningprofiles.com/members/images/' . $_GET['id'] . '.' . $row['ext'] . '" />'; } }else{ echo 'Error: Image not found.'; } ?>
-
That error message is telling you that this is your query: '<?php $sql = 'SELECT `ext` FROM `user_image` WHERE `user_id`=' . $_GET['id']' Whatever you are doing, mysql is running something like this: mysql_query("<?php\n$sql = 'SELECT `ext` ..."); which is surely wrong. So if this code is in a single file that you are referring to in your browser, yah it should work. But if you are including() or requiring() that code I gave you, that could explain it.
-
It seems that the PHP snippet I gave you is somehow being sent as the query. How are you calling this code?
-
Try echo'ing the SQL statement and running it directly in phpMyAdmin. Try echo'ing mysql_error(). At some point you need to learn how to debug your application. Other than copying and pasting what I put, running it once, and then coming back to say it doesn't work, what have you done to try and troubleshoot it yourself?
-
If it were correct it would be working, which you already said it wasn't. You're using a variable $image in your header() call but I don't see where you're setting it. Anyways, you don't have to make any calls to header(). The file has the extension attached so the browser will know what to do with it. All you need to do is look up the record in the table for the appropriate extension. <?php $sql = 'SELECT `ext` FROM `user_image` WHERE `user_id`=' . $_GET['id']; $q = mysql_query($sql); if($q){ $row = mysql_fetch_array($q); if(!empty($row)){ echo '<img src="http://www.yourdomain.com/members/images/' . $_GET['id'] . '.' . $row['ext'] . '" />'; } }else{ echo 'Error: Image not found.'; } ?>
-
Well these are avatars. I assume they're meant to be displayed while viewing a member's profile or forum post. In order to do so you have to SELECT the data from the database and then create an HTML img tag.
-
Would you be willing to provide an example? I've barely scratched the surface of MVC myself and I think it's pretty clear you have extensive experience in this area.
-
That's it as far as uploading is concerned. The next part is to display the avatars where appropriate. That should be as easy as selecting a user's avatar from the database and creating an img tag.
-
Be careful when doing that. Sometimes an author will take the time to point out a very particular nuance of a language in a section you think you already know and thus skip over. The best way to get a leg-up on the MVC approach is to download an existing PHP framework and see how they do things. I myself have been fiddling with cakePHP and so far the experience has been enjoyable.