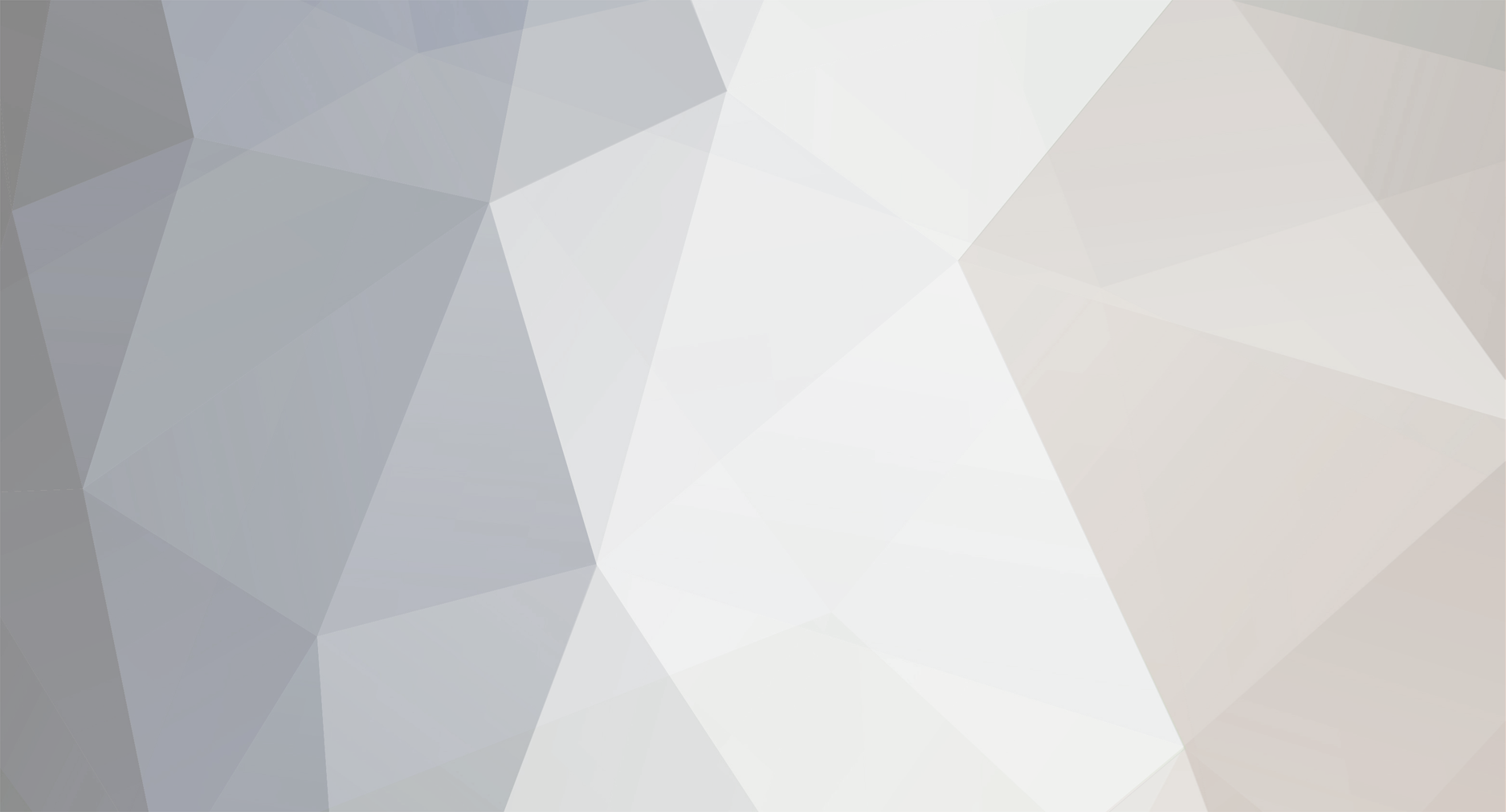
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Reading Material - General Computing not PHP
roopurt18 replied to GingerRobot's topic in Miscellaneous
I'd recommend any resource that deals with the basics of abstract data types and algorithms. You won't need to know them to program PHP, but learning about them will teach you to think effectively about implementing solutions to problems. The best example of where this pays off that I can think of is this thread: http://www.phpfreaks.com/forums/index.php/topic,155891.0.html Any programmer, through sheer determination, can create a program that parses and then evaluates an expression. I can guarantee that any implementation that doesn't use a tree will be messy and difficult to maintain though. I can't recommend any resources that don't read like text books, but here are some general data types / concepts I think every programmer should be vaguely familiar with: linked lists queues priority queues hash tables binary search trees Those are some basic data structures, but you can accomplish quite a bit with them. They also lead into more complicated structures such as red-black trees, oct-trees, or graphs. Other topics I would recommend: algorithm analysis (big-O) discrete mathematics languages / grammars software development processes / methods (test driven, uml, test cases, that sort of thing) That's all I can think of right now. Inevitably there is always one individual that says, "None of that stuff applies to web development so don't waste your time." It's still good to know IMO. -
[SOLVED] Arrays (3D array? or just value change?)
roopurt18 replied to colombian's topic in PHP Coding Help
http://www.php.net/extract -
[SOLVED] how to check what the number is
roopurt18 replied to nathanmaxsonadil's topic in PHP Coding Help
A single = would have been even more wrong in this context. <?php $i = 0; while($row = mysql_fetch_assoc($result)){ if($i == 4){ // Rows 1 - 3 } $i++; } ?> Are you just blindly copying and pasting code or are you making any attempt to understand what's happening here? -
http://manual.phpdoc.org/HTMLSmartyConverter/HandS/phpDocumentor/tutorial_tags.pkg.html
-
[SOLVED] Combining two variables together to make a new one?
roopurt18 replied to hatrickpatrick's topic in PHP Coding Help
This is called concatenation: $var = $var1 . $var2; -
Hello, how to make this go from one item to the next?
roopurt18 replied to 11Tami's topic in PHP Coding Help
You can't use PHP to continuously update a user's browser, you'd need to use Javascript. -
[SOLVED] how to check what the number is
roopurt18 replied to nathanmaxsonadil's topic in PHP Coding Help
You missed a less than sign, Wuhtzu. -
[SOLVED] how to check what the number is
roopurt18 replied to nathanmaxsonadil's topic in PHP Coding Help
$i = 0; while($row = mysql_fetch_assoc($result)){ if($i < 3){ // Rows 1 - 3 } $i++; } -
[SOLVED] how do I show if something is new?
roopurt18 replied to acidglitter's topic in PHP Coding Help
Don't do this with PHP at all; use MySQL to do the work for you. Assuming the MySQL records have a DATETIME column named entered_dt: SELECT *, IF( NOW() - INTERVAL 1 WEEK < entered_dt, 1, 0 ) AS `NewPost` FROM <table_name> WHERE <where_condition> This will return a column named 'NewPost' that is a 1 if the post is new, 0 if it is old. This reduces your PHP code to: <?php $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ if($row["NewPost"] == 1){ // New post }else{ // Old post } } } ?> I wish people would stop recommending to use PHP's date / time functions on data that is coming from MySQL. -
[SOLVED] Arrays (3D array? or just value change?)
roopurt18 replied to colombian's topic in PHP Coding Help
<?php $forms = array ( 'fname' => Array( 'text' => 'First Name' ), 'lname' => Array( 'text' => 'Last name' ) ); ?> -
Hello, how to make this go from one item to the next?
roopurt18 replied to 11Tami's topic in PHP Coding Help
Are you wanting the user's browser to update a value every 1 second? -
[SOLVED] how to check what the number is
roopurt18 replied to nathanmaxsonadil's topic in PHP Coding Help
Do you want to echo the fourth row at all? -
If you have shell access you could run the script from the command line, then it can't timeout.
-
i was not aware that you can chain methods in php
roopurt18 replied to emehrkay's topic in Miscellaneous
I'm only saying this for the newer OOP programmers, be very careful when using this kind of syntax. You must be certain that the functions you are chaining return the proper object types or you will run into problems! -
[SOLVED] What is wrong with this conditional?
roopurt18 replied to tlavelle's topic in PHP Coding Help
You left an open paren off of one of your isset expressions and a closed paren off of the other. When you get parse errors, look on and around the line the error is complaining about and look carefully. -
I can't remember all of the rules for derivatives, but I don't think this problem is terribly complicated. My recommendation is to take the input and build an expression tree out of it the same way that compilers do *hint* *hint*. Some example expressions and their expression trees: 2x^3 + 5 +-- 5 +-- 3 | | + --+ +-- ^ --+ | | | +-- * --+ +-- x | +-- 2 ***************************** ***************************** 3ln(1 / (2x^3 + 5)) +-- 5 +-- 3 | | +-- ( -- + --+ +-- ^ --+ | | | | +-- ln -- / --+ +-- * --+ +-- x | | | * --+ +-- 1 +-- 2 | +-- 3 So the first task is writing a parser that can take an expression as a string and convert it into such a tree. You'll notice that orders of operation with high precedence are deeper in the tree; this gives us a hint with our next task. From here, you need to create a function that takes this tree structure and creates a derivative string of it. We'll call the function create_derivative_string(); it takes two parameters, the tree structure and the value we're solving for. <?php $expression = "2x^3 + 5"; $tree = parse_expression($expression); $dExpression = create_derivative_string($tree, "x"); // $dExpression now contains: "3*2x^(3-1) + 0" ?> You must now pass $dExpression into a regular expression parser that evaluates an expression: <?php // cont'd from above $dTree = parse_expression($dExpression); $output = evaluate_expression($dTree); // $output now contains: "6x^2" ?> The function that converts the input expression into a tree is nothing more than a finite state machine. If you want to do individual research on this stuff, there is no better resource than "The Dragon Book": http://en.wikipedia.org/wiki/Compilers:_Principles%2C_Techniques%2C_and_Tools Otherwise, googling for "computer languages" or "computer grammars" might pull up some decent hits. Also, feel free to PM me if you get stuck anywhere. I've written a basic C compiler and I worked on another project where we were given a fake language and had to create a parser for it; that was a while ago though so I may be a bit rusty!
-
Depends really. When you're a teenager and dependent on your parents, any amount of money can be hard to come by. I read books not because I don't like online resource, but because after 8 hours of computer time at work, another 1 or 2 at home playing video games, sometimes I want to give my eyes a rest from the monitor. Also, I don't really condone reading online resources from your laptop in your bathtub. You could always print them, but I've always liked the compactness and longevity of a printed book to that computer paper. Books can also save time after the initial investment of reading them. For example, you could learn everything you need to know about the different Javascript event models online. But you'd be hard-pressed to do it as quickly as I can read chapter 14 of Javascript: The Definitive Guide.
-
It depends on the context. function someFunc($arg){ if($arg){ // Test that the value exists } } The example above doesn't make any sense. The argument $arg isn't optional so it has to exist. if($value){ // Ensure a value is true } This will work as expected, the if body will only execute if the variable is true. However, if the variable is not set PHP will raise an error, possibly silently. Use isset() when there is a possibility the variable is not set and then test it's value. if(isset($var) && $var){ // If the var exists and evaluates true }
-
AFAIK isset() is a function while if is a language construct, which means you can use isset() in places where you can't use if.
-
Never, ever, under any circumstances use the type field provided in the $_FILES array; it is set by the browser and not PHP or the web server. This means the value can be easily faked. Refer to this: http://www.php.net/manual/en/ref.fileinfo.php
-
To steer this back on topic, I doubt many people on this forum have read that particular book. Most of us are college educated or self taught through Google searches or online tutorials. If you want a review of a book, why don't you look for the title on Amazon.com and then read the user reviews there? I will tell you the very first programming tool I ever bought was Sams Publishing Teach Yourself C++ in 21 Days, it came with MS Visual C++ 2.0. I was around your age when I bought it and it gave me a great step in the right direction. In general, I think the Teach Yourself XYZ in LMNOP books are great for giving you a quick overview; but most of them avoid complicated tasks. For example, such a book will spend an entire chapter on loops. What they are, why we have them, the different syntaxes for creating one, explaining how you can use any type of for loop even though one might be better suited to the task, etc. I don't need such an explanation anymore; I've written thousands of loops, even a few I didn't intend! For me now, the best books are the real thick ones that dive deep into a topic. They spend a small amount of time explaining the syntax because syntax is easy to learn. They spend more time on the little nuances of a technique or particular construct within a language. I probably buy on average one programming book per month and read it leisurely just to become familiar with topics. I don't try to learn every detail because I know I can always pick up the book and use it as a reference when I'm confused about a subject. If you're the type of person that can sit down, read a book, and learn from it, then by all means by that Teach Yourself... book. Since you're still new to programming, I recommend typing out every single example and ensuring that they work. This will get you even more familiar with the syntax, teach you subtle things, and make you familiar with debugging. After that, I recommend working on your own mini projects, whatever they might be. Create a simple shopping cart where the user can select items and enter shipping info and stuff, but don't concern yourself with details like payment processing. After you develop a few such projects and gain a better understanding, I then recommend buying a more "heavy duty" book. Don't buy another beginner book; look at the back of it and make sure it says its for intermediate or advanced developers. You won't need another Building Websites with PHP & MySQL book, you'll want something more like PHP: The Definitive Guide; BTW I recommend books from O'Reilly. Other than that, ronald hit the nail on the head. You spent too much time too soon in the Misc. section of this board posting nonsensical crap. You're going to find out quick that computer people are an odd, peculiar breed. Also, most of us are working professionals. We don't come here to socialize or make friends; we come here to talk about all things related to web development. Sometimes someone will make a post about an odd topic, but it's maybe once every two weeks per person, if that. You were running an average of one or two per day. I would also recommend not responding to help threads if you have nothing new to offer; replying to a thread and just regurgitating what someone else said is a sure-fire way to piss them off to no end. Someone in here made a post about life experience. You're getting some now. Try too hard and / or come off too strong and people will shun you.
-
There is always a small chance that his experience with PHP and plumbing could allow him to create the most awesome, web-based plumbing software on the market. Then LiamProductions will make millions while the rest of us argue about the benefits of a CSS based layout and all that sort of thing! Buahahaha
-
I almost hate to encourage you, but I started programming as a hobby when I was around 14 or so because I wanted to make video games. So here I am now, over a decade later, programming, but not video games. So there is a possibility that what you want to do now is what you want to do later. However, I did take an extensive break from programming during my junior and senior years of high school to go out and have more fun. Also, you should note that X years programming as a hobby does not count as experience. The only thing that counts as experience as far as someone who is hiring is concerned are real projects made for real companies and / or clients.
-
Doing some reporting I found an article I found interesting on cross-tabulation: http://dev.mysql.com/tech-resources/articles/wizard/index.html