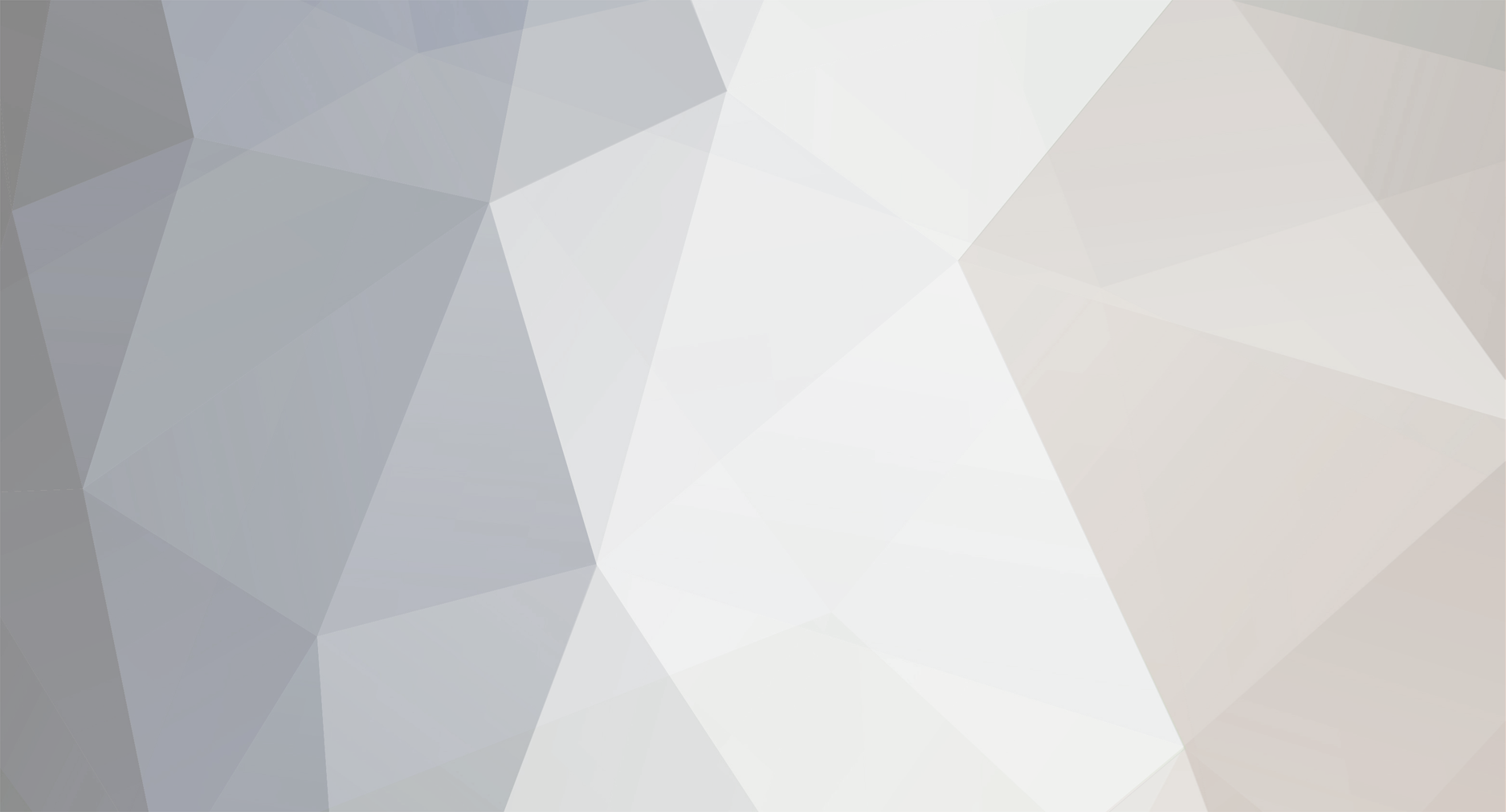
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
instead of using the variables name directly, use the $_POST array
so instead of this
$msg = "Sender Name:\t$sender_name\n";
You'll have this
$msg = "Sender Name:\t".$_POST['sender_name']."\n";
Also you have a security problem in this line
$mailheaders .= "Reply-To: $sender_email\n\n";
spammers could use your server to send tons of spam all over the place. You can find out more details on http://www.securephpwiki.com/index.php/Email_Injection
-
I think the easiest way to read a text file through iframe. Just call the text file in a hidden iframe and read the content using a script. Here is a quick example
<script language="javascript"> var did_load=false; function grap_cont(){ if (did_load){ var frm = document.getElementById('hidden_frame').contentWindow.document; var txt = frm.body.innerHTML.replace(/<[^>]*>/g,""); alert(txt); } } </script> <a href="txt1.txt" target="hidden_frame">First text file</a><br /> <a href="txt2.txt" target="hidden_frame">Second text file</a> <iframe src="blank.html" name="hidden_frame" id="hidden_frame" onload="grap_cont(); did_load=true;" style="width:0px; height:0px;" frameborder="0" scrolling="no"></iframe>
-
You have two ids with the same value (id="proof"), ids should be uniqe for each element. Also, use onclick event to capture the user selection and since you passing the object to the function, you can just use obj.value
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Untitled Document</title> <script type = "text/javascript" language = "Javascript"> function Proof(obj) { if (obj.value == "no") { // if you using class name to set the display property, use block. // if you set the style in the tag (e.g. <div style="display:none"> // use nothing "" // the second is a better option for layout. displayValue = ""; } else { displayValue = "none"; } document.getElementById('proof_type').style.display = displayValue; } </script> </head> <body> <form action="" method="post" enctype="multipart/form-data"> <input name="proof" type="radio" id="proof_1" tabindex="1" value="yes" onclick="Proof(this);" /> Yes <input name="proof" type="radio" id="proof_2" tabindex="2" value="no" onclick="Proof(this);" /> No <div id="proof_type" style="display:none;">Proof Type</div> </form> </body> </html>
-
Here is a quick demo on alerting using an image button (using the onsubmit event on the form)
<form onsubmit="alert('hello');"> <input type="text" /> <select><option>Select</option></select> <textarea></textarea> <input type="image" src="image_src" alt="submit" /> </form>
Notice the enter button only submit the button when it's clicked on the input type="text", it doesn't work from a select menu or a textarea.
You also can use a submit button if you want, just use CSS to add a background image, remove the borders and set the height and width. This will give you a graphical submit button.
-
-
hummmmm, this happens because the link is generated after the page is loaded (the lightbox script looks at links when the page loads). You'll need to assign the onclick event manully like this
<script language="javascript"> function show_img(src, lrg_src){ var txt = "<a href=\""+src+"\" rel=\"lightbox\" id=\"light_box_link\"><IMG src=\""+lrg_src+"\" /></a>"; document.getElementById('display_charts').innerHTML = txt; document.getElementById('light_box_link').onclick = function () {myLightbox.start(this); return false;} } </script> <a href="#" onclick="show_img('img0_src', 'large0_img_src'); return false">Image 0</a><br /> <a href="#" onclick="show_img('img1_src', 'large1_img_src'); return false">Image 1</a><br /> <a href="#" onclick="show_img('img2_src', 'large2_img_src'); return false">Image 2</a><br /> <a href="#" onclick="show_img('img3_src', 'large3_img_src'); return false">Image 3</a><br /> <div id="display_charts"> The images will show here </div>
P.S. I added a return false on the images list so the page doesn't jump up if you have too many links.
-
You'll have a hard time with this because firefox doesn't support the $1, $2, ... $9 which you'll need to use to replace in the string.
-
The image type doesn't have an onKeyPress event because it doesn't take any input.
Use onClick instead.
If you want the alert to show when the user click the enter button, add it to the onsubmit event in the form.
-
you can do something like this, just modify it to fit your needs
<script language="javascript"> function show_img(src, lrg_src){ var txt = "<a href=\""+src+"\" rel=\"lightbox\"><IMG src=\""+lrg_src+"\" /></a>"; document.getElementById('display_charts').innerHTML = txt; } </script> <a href="#" onclick="show_img('img0_src', 'large0_img_src');">Image 0</a><br /> <a href="#" onclick="show_img('img1_src', 'large1_img_src');">Image 1</a><br /> <a href="#" onclick="show_img('img2_src', 'large2_img_src');">Image 2</a><br /> <a href="#" onclick="show_img('img3_src', 'large3_img_src');">Image 3</a><br /> <div id="display_charts"> The images will show here </div>
-
Here is a sample fancy select menu, let me know if you have any questions.
Basiclly, the list is an array, when the user type something a function will go through the array and match the first characters with the user input. If it match, it'll add it to the drop down div.
Good luck
<script language="javascript"> var list = new Array("James|jousting","Bob|jousting","Emily|jousting","James|email","James|filing","someone|else","kaaa?|where","why|any"); function update_list(){ var txt = ""; var str = document.getElementById('sel_search').value.toLowerCase(); for (i=0; i<list.length; i++){ list_arr = list[i].split("|"); if ((list_arr[0].substr(0, str.length).toLowerCase() == str) || (list_arr[1].substr(0, str.length).toLowerCase() == str)){ txt += "<a href=\"#\" onclick=\"document.getElementById('sel_search').value=this.innerHTML;\">"+list[i]+"</a><br />"; } } if (txt == "") txt = "No options were found"; document.getElementById('sel_div').innerHTML = txt; } </script> <input type="text" style="width:200px;" id="sel_search" onkeyup="update_list()" onfocus="update_list(); document.getElementById('sel_div').style.display='';" onblur="setTimeout('document.getElementById(\'sel_div\').style.display=\'none\'',100);" /><br /> <div style="width:200px; height:300px; overflow:scroll; display:none;" id="sel_div"></div>
-
can you post some of your html as well?
-
instead of going back and forward, this will get you started.
<script language="javascript"> function fill_name(val){ document.getElementById('to_name2').value = val; } </script> <a href="#" onclick="fill_name('Joe Dirt'); return false;">Joe Dirt</a><br /> <a href="#" onclick="fill_name('Joe Dirt 1'); return false;">Joe Dirt 1</a><br /> <a href="#" onclick="fill_name('Joe Dirt 2'); return false;">Joe Dirt 2</a><br /> <a href="#" onclick="fill_name('Joe Dirt 3'); return false;">Joe Dirt 3</a><br /> <a href="#" onclick="fill_name('Joe Dirt 4'); return false;">Joe Dirt 4</a><br /> <a href="#" onclick="fill_name('Joe Dirt 5'); return false;">Joe Dirt 5</a><br /> <input type="text" name="to_name" id="to_name2" size="30">
-
not sure which part didn't work, I just copied this code and it worked for me,
<select name="fl" onChange="pickedFile(this.value)" > <option value = "index" selected>index</option> <option value = "general">general</option> <option value = "test">test</option> </select> <input type="text" name="f" id="fileinput" value="" /> <script language="javascript"> function pickedFile(pickd) { document.getElementById('fileinput').value = pickd; } </script>
Let me know which part didn't work.
-
options doesn't have an onclick event, only the select menu with an onchange event. This might work
<select name="fl" onChange="pickedFile(this.value)" > <option value = "index" selected>index</option> <option value = "general">general</option> <option value = "test">test</option> </select>
-
you can't sleep in JS, use setTimeout instead
parent.document.getElementById('BUT01').value='ELABORAZIONE... '; setTimeout("parent.document.getElementById('BUT01').value='FINITO'", 2000);
-
actually, don't use display:block;
Not sure you set the script up, but here is what works
Let's say this is your html (notice the id in the tr tag)
<tr id="TR_ID" style="display:none;"> ..... </tr>
Your button will call the following Javascript function once clicked
<script> function show_tr(){ document.getElementById('TR_ID').style.display = ""; } </script>
You can add an if statment to toggle the display between "none" and "";
-
put the style="display:'none;'" in the tr instead of the td
-
That's way too much code, the select menu have the value of it's selected option. You can do something like this
<select onchange="location.href='family.php?search&view='+this.value;"> <option value="[iD]">option</option> .....
-
just use something like this
document.getElementById('itemone').id = "active";
-
for that, you would need to create a function in Mainpage A to handle the redirection like this
<script language="javascript"> function redirect_page(url){ location.href=url; } </script>
and in you pop up, you'll have something like this
<script language="javascript"> function redirect_parent(url){ try { opener.redirect_page(url); } catch(e){ // if no redirect function is around } } </script> <a href="test2.html" onclick="redirect_parent(this.href); return false;">Click here</a>
this function will try to use the Mainpage A redirect function, if it's not there nothing will happen.
Note, only the window that opened the pop-up will be the "opener", if you want to have both A and B open and the B generate the pop up, A won't be effected. For that you would need to have the main page that generate pop-ups for A, B, and the pop-up and assign names to them. All the functions will be in the main page to handle the different windows.
-
you can use the opener attribute to redirect webpage (A) to the URL
<a href="URL_GOES_HERE" onclick="opener.location.href=this.href; return false;">Click here</a>
-
You would need to store the messages somewhere (either text file or database) so the other users can get the data. Also, you might wanna use the encodeURI() function when creating the URL for your ajax.
I also think you might want to make your http variable global so you can use it in other functions other than the ajaxfunction.
-
you can use the eval function to run a the code stored in string
eval(params+"();");
-
the class might give you a problem with the script you have, you might wanna try this
<div style="display:none;" ...
Character count/restriction in IE
in Javascript Help
Posted
just add var infront of length