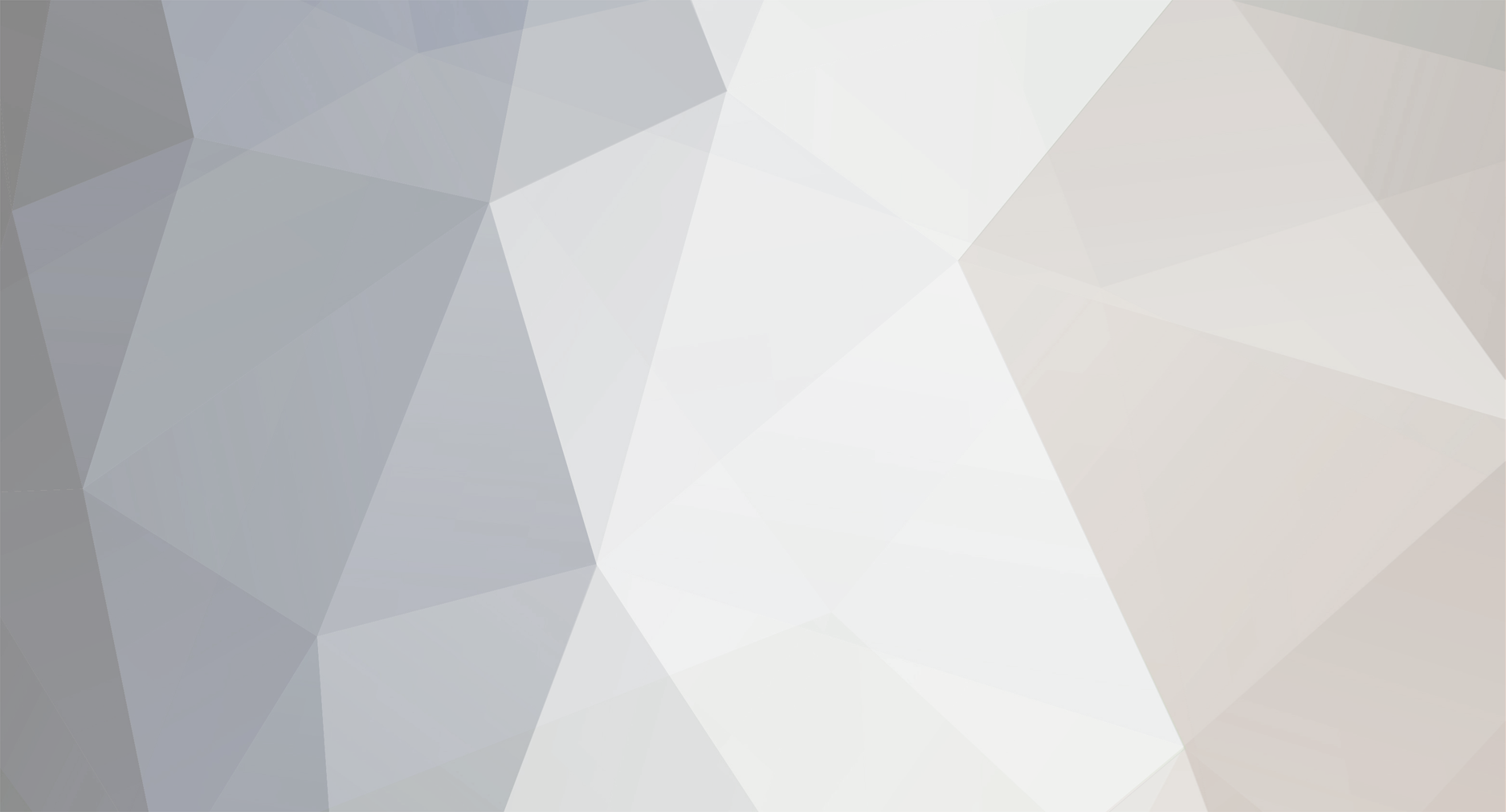
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
you can use the eval function
eval("document.formName."+myFields[i]+".focus();");
-
You can use the NoGray JS Library, it has an animation file that will do exactly what you want. I haven't wrote the docs for the animation library, but it's pretty easy to figure out if you know some javascript (you can ask any question if you have issues with it).
Here is an example of how it works http://www.nogray.com/new_site/examples/animate_example.html
You can download the script from http://www.nogray.com/new_site/download.php
the function you'll probably us is animateSizeTo()
-
You just used a reserved word as the id 'choice'
change it to something else and it should work, like this
<script type="text/javaScript"> function checkDonation(id){ var amount = document.getElementById('donationAmount').value; var zeroField = id+"None"; //var should be equal to "choiceNone" if (amount > 0){ document.getElementById(id).checked = true; document.getElementById(zeroField).checked = false; }else{ document.getElementById(zeroField).checked = true; document.getElementById(id).checked = false; } } </script> <input name="choice" type="radio" id="chsNone"> <input name="choice" type="radio" id="chs" > <input name="donationAmount" type="text" id="donationAmount" onkeyup="checkDonation('chs')" />
-
you can use document.getElementById('ID_HERE') to get the object in any browser
<script type="text/javascript"> function papito(obj) { document.getElementById(obj).src="images/"+obj+"_on.jpg" } function papiton(obj) { document.getElementById(obj).src="images/"+obj+".jpg" } </script> <img name="logo2" id="logo2" onmouseout="papiton('logo2')" onmouseover="papito('logo2')" src="images/logo2.jpg" />
-
You just need to return false in the javascript so the link won't re-load the page
<a href="#" onclick="window.open ( blablabla); return false;">
-
this should do everything you need
[code]
<a href="#" onclick="window.open('test1.html', '', ''); selectLink(this); return false;">Link 1</a><br />
[/code] -
you can do this with some javascript and style. basiclly, when you click on a link the script goes through the links, find the higlighted link and unhighlighted. Than highlight the link you clicked on. If you have a lot of the links in the page, put the window.open before the selectLink (in the sample below)
[code]
<script language="javascript">
function hide_selection(){
var lnks = document.getElementsByTagName("A");
for(i=0; i<lnks.length; i++){
if (lnks[i].className == "selected_link"){
lnks[i].className = "";
break;
}
}
}
function selectLink(ob){
hide_selection();
ob.className = "selected_link";
}
</script>
<style>
.selected_link {background:#e9e5c6;}
</style>
<a href="#" onclick="selectLink(this); window.open('test1.html', '', '');">Link 1</a><br />
<a href="#" onclick="selectLink(this); window.open('test1.html', '', '');">Link 2</a><br />
<a href="#" onclick="selectLink(this); window.open('test1.html', '', '');">Link 3</a><br />
<a href="#" onclick="selectLink(this); window.open('test1.html', '', '');">Link 4</a><br />
[/code] -
you forgot the [b]px[/b] after the 2, you can use something like this
[code]
style="border:solid #00003B 2px"
[/code] -
ok, here is an easy way to achive what you want. (I removed the cookie stuff from this function for now). Instead of using height, you can use the style property like below.
[code]
...
function menu(varID) {
if (document.getElementById(varID).style.display == "none"){
document.getElementById(varID).style.display = "";
}
else {
document.getElementById(varID).style.display = "none";
}
}
...
[/code]
[code]
...
<div id="2" on onclick="menu('2_1')"><strong><a href="#">Departments</a></strong></div>
<div id="2_1" style="display:none;">
<table width="100%" border="0">
<tr>
<td width="10"> </td>
<td><a href="engine.php?varDisplay=&varPage=english001">English</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=citizen001">Citizenship</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=found001">Foundation</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=geog001">Geography</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=hist001">History</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=ict001">ICT</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=lang001">Languages</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=maths001">Maths</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=ssci001">Social Science</a></td>
</tr>
<tr>
<td> </td>
<td><a href="engine.php?varDisplay=&varPage=technology001">Technology</a></td>
</tr>
<tr>
<td> </td>
<td> </td>
</tr>
</table>
</div>
...
...
[/code] -
you can use span tag instead, or try this
[code]
div {display:inline;}
[/code]
not sure if it'll work though. -
If you look over the NoGray JS library, there is a getStyle, getHeight functions that will return the current style for any element.
Just go to http://www.nogray.com for more info. -
the php include file will take the code out of the top.php and place it in the other file (the files inside teams). Most like the image reference is relative to the top.php file, so when the file is included in another folder, it can't find the image. Try to use absolute paths
e.g. http://www.site.com/images/image1.gif instead of images/image1.gif -
There is a very easy way to do this. Firefox and IE have this cool function that will correct any HTML code that is inserted into the page using JavaScript. They'll close all the tags and fix the HTML code without you having to do anything. Here is a basic script that will take the input from a textarea and assign it to a div. The browser will fix the HTML. Finally, get the HTML value for that div and assign it the textarea value.
[code]
<script language="javascript">
function correctHTML(){
txt = document.getElementById('HTML_Code').value;
document.getElementById('fixing_div').innerHTML = txt;
document.getElementById('HTML_Code').value = document.getElementById('fixing_div').innerHTML;
}
</script>
<textarea name="HTML_Code" id="HTML_Code"></textarea>
<div style="display:none;" id="fixing_div"></div>
<button onclick="correctHTML();">Correct HTML</button>
[/code] -
The right floated image should come first in the code.
[code]
<a href="viewcat.php?cat=peripherals&PHPSESSID=99b9b7f7e7fa611cb34a2d4e6bf20799"><img src="images/store/printer.jpg" alt="" style="float: right;" /><img src="images/store/mp3.jpg" alt="" style="float: left;" /><img src="images/store/camera.jpg" alt="" /></a>
<a href="viewcat.php?cat=peripherals&PHPSESSID=99b9b7f7e7fa611cb34a2d4e6bf20799"><img src="images/store/mp3.jpg" alt="" style="float: left;" /><img src="images/store/camera.jpg" alt="" /></a>
[/code] -
No, there is no HTML alternitive. If you don't want them to type it in the first place, you gotta add an onkeypress event and check the value each time the key is pressed.
-
I know most people hate to use tables right now (CSS only approach) but tables can come in handy a lot of times.
You're issue can be solved if you put the navigation in a table with 100% width. You're client won't even know the difference if you use tables or lists, as long as it look like what he wants.
Otherwise, I assume you're use PHP to generate the menu. Just get the number of links that you have, and divide it by 100% using PHP. Finally, assign the output to the list items. -
You can use regular expressions for that, here is a small sample
[code]
<script type="text/javascript">
function cF(){
if (document.getElementById('input_field').value.search(/^[a-zA-Z0-9]+$/) == -1){
alert("invalid value");
return false;
}
}
</script>
<form method="post" action="#" onSubmit="return cF()">
<input type="text" name="input_field" id="input_field" />
<input type="submit" value="Click" />
</form>
[/code] -
just google "breakout of frame" and you'll find some usefull script. Alter them to do what you need.
-
on thing I notice is that you have an "onClick" event on the option. IE doesn't support events on the options, you'll need to set the event on the whole select menu and use "onChange"
You can add a function to the script like
[code]
function show_hide(val){
if (val == "Public"){
show('public'); hide('priv_p'); hide('private');
}
else {
show('private'); show('priv_p'); hide('public');
}
}
[/code]
and change the Type select menu like below
[code]
<select name="type" onchange="show_hide(this.value);">
<option value="Public">Public</option>
<option value="Private">Private</option></select>
[/code] -
If you want to keep it all CSS, the easiest way is to use a transparent png image on top of the navigation. For IE 6 and lower, you'll need to use "AlphaImageLoader" filter http://msdn.microsoft.com/library/default.asp?url=/workshop/author/filter/reference/filters/alphaimageloader.asp
Here is how it works, you set you navigation as it is right now (the normal status for the link shouldn't have a background color). Use the gradient background behind the whole navigation.
the Hover status will have the background color as the darker version.
Finally, add the png image over the background to create the shape you want. You can use a taller version of the shape and just set it as a div background. This way, if the menu grow, you can just grow that div.
Attached is a small illustration on how to make it.
[attachment deleted by admin] -
Actually, you would need to add action script in your flash movie to send to JavaScript and both the script and the swf file have to be on the same domain. Here is a link for more details
http://www.adobe.com/cfusion/knowledgebase/index.cfm?id=tn_15683 -
Try to use different names, "public" and "private" could be reserved by JavaScript. instead use something like "pub_tr" or something like that.
-
change the submit event in the form tag to
[code]
onsubmit="return submit_check();">
[/code]
this will return the function result to the submit event. -
you have a space before your div tag
[code]
valign=top> <div
[/code]
and you also have an extra table row tag
[code]
</tr><tr></table></div>
[/code]
remove them and see if that fixes the issue.
[SOLVED] Using a <select> to show/hide hidden layers
in Javascript Help
Posted
as "fenway" said, something like this