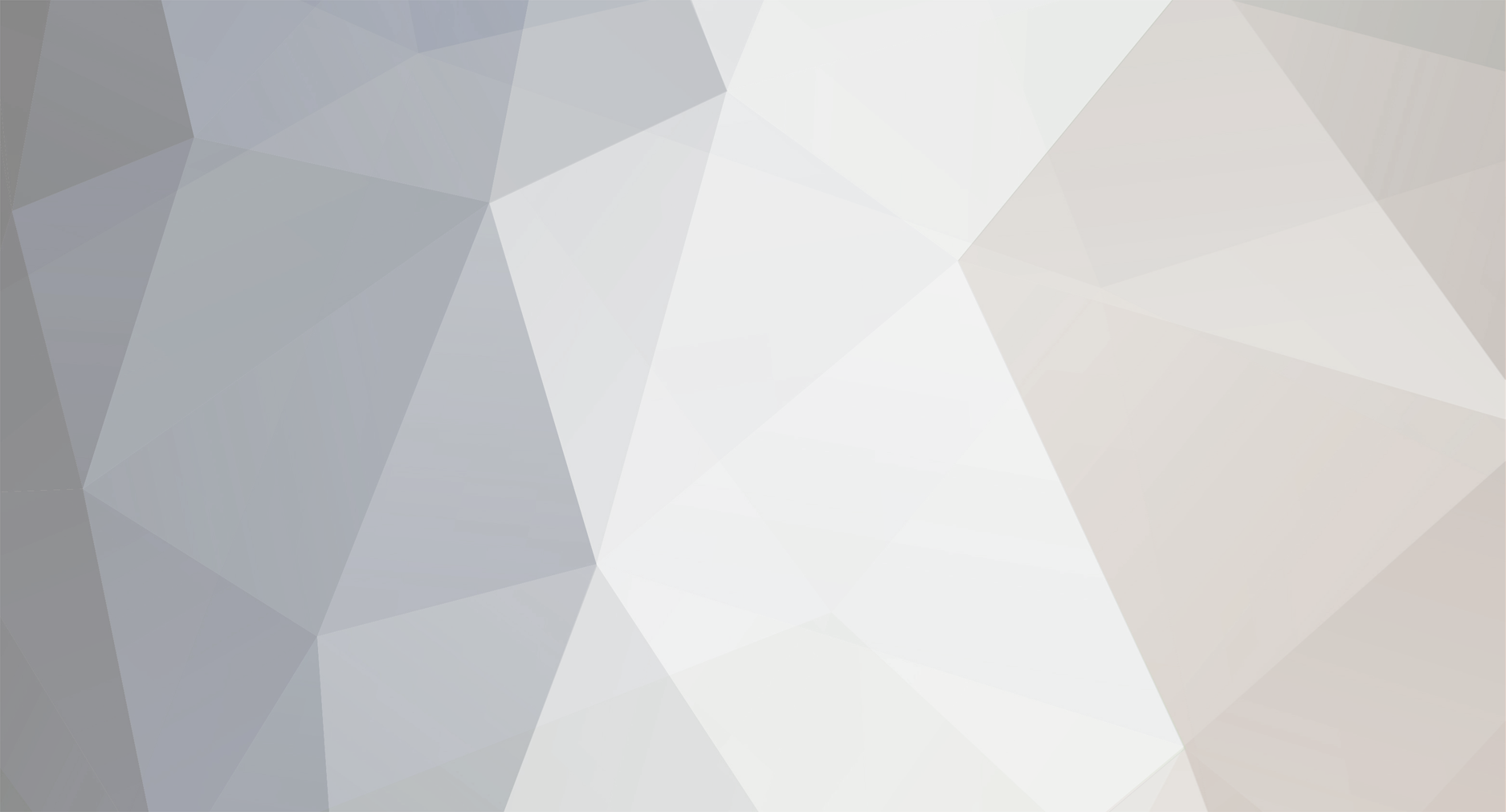
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
There is no pause in JS, but there is a time out function
use this in the onload event
<body onload="setTimeout('Function_Name()', 30000);" ....
This will run the Function_Name() function in 30 seconds.
-
I tried your functions and they seem to be fine. Check if your query return any result or if you SQLQuery function is generating any errors.
-
Just use the "parent" window and it should work if both pages on the same domain
<a href="#" onclick="parent.document.getElementById('message').value = 'hello world'; return false;">Click here</a>
Change the "hello world" to anything you need, the return false will stop the page from jumping up.
-
you can do something like this
the did_load variable will stop the page from reloading once the page first load (so the user doesn't get unlimited loop)
<script language="javascript"> var did_load = 0; </script> <iframe onload="if(did_load > 0) window.location.reload(); did_load++;" ....
-
Not sure how will the newsgator output the HTML, but if the script prints out HTML code, you can load the output into a div, and fill a form field with the div innerHTML and submit the form to a php file.
<html> <head> <script language="javascript"> function submit_form(){ document.getElementById('html_output').value = document.getElementById('HTML_code').innerHTML; document.getElementById('html_form').submit(); } </script> </head> <body onload="submit_form();"> <div id="HTML_code"> <script src="http://services.newsgator.com/ngws/headlines.aspx?uid=XXXXXX&mid=XX"></script> </div> <form method="post" action="SOME_PAGE.php" id="html_form" name="html_form"> <input type="hidden" name="html_output" id="html_output" /> </form> </body> </html>
-
you can't use the if statement withing the echo statement, break it down like this
echo "<input type='text' name='first' size='10' value='"; if(isset($_POST['change'])==TRUE){ echo $_POST['first']; } else{ echo 'john'; } echo "' />\r\n";
-
for a line break on a browser, you need to use HTML < br /> tag instead of \n
Or you can put your output between < pre>< /pre> tag (spaces added to tag so they show up)
-
I tried this line in a simple loop and it worked fine
document.myform.subcat.options[count]=newop;
check if your loop and number variables are working through out the loop, or if there is anything else that's generating an error (you can use firefox error councel)
Here is the function I tried
<script> function loadit(){ var count=0; for(var number=0;number<5;number++) { var theText = "Hello World " + number; var theValue ="Hello World " + number; var newop= new Option(theText,theValue); document.myform.subcat.options[count]=newop; count++ } } </script> <form name="myform" id="myform"> <select name="subcat" id="subcat"> </select> </form> <button onClick="loadit()">Click</button>
-
add ids to your input fields
<input name="price" id="price" type="text" value="<? echo $price; ?>" readonly="readonly"> <input name="quantity" id="quantity" type="text" value="1" onChange="setTotal()"> <input name="total" id="total" type="text" readonly="readonly" value="">
and this should do it
-
add an id to the input field
<input type=text name=message id=message>
in the iframe, add an onload event
<iframe onload="document.getElementById('message').value=''; document.getElementById('message').focus();" .....
This should clear the input field and put the focus on it.
-
wow, that's very interesting.
Well, as long as you got it to work.
Wish you the best.
-
The issue is the with the scroll bar in Firefox
the bottom scroll bar appread because the div have the same width as the screen (wider than the page). In line 15 I changed it to this
myOverlay.style.width = pageWidth + 'px';
The easy way to fix this is the add 15 to the height if it's firefox and the scrolling is more than the page height. This will solve the issue.
-
you're checking if the length is smaller than 5 and smaller than 12
if((document.registration.username.value.length < 5)||(document.registration.username.value.length < 12)){
Notice the < sign before the 5
-
on this line
<area shape="rect" coords="17,133,59,180" alt="Southern California" title="Southern California" href="http://search.stores.ebay.com/MOD-ELEMENTS_southern-california_W0QQfcdZ2QQfciZQ2d1QQfclZ4QQfromZR10QQfsnZMODQ20ELEMENTSQQfsooZ1QQfsopZ1QQftsZ2QQsaselZ239020879QQsatitleZQ22southernQ20californiaQ22QQsofpZ0"onMouseOver="document.getElementById('testtext').innerHTML='Southern California <br> <div class=smalltext>Featured Designers<br>Charles and Ray Eames <br>Click to view item<br><br></div>' ">
change
Ray Eames
to <a href='link here'>Ray Eames</a>
-
They are using the Yahoo UI Library Container object,
Here is a link for it, http://developer.yahoo.com/yui/container/
You can find all the instructions on how to use it there.
-
Here is a small code to check which browser the user uses (from the NoGray Javascript Library)
// checking if the browser is Opera var is_Opera = (window.navigator.userAgent.search("Opera") != -1); // checking if the browser is IE var is_IE = ((window.navigator.userAgent.search("MSIE") != -1) && !is_Opera);
-
try moving the
onChange='autoSubmit2();'
to the select menu
<select onChange='autoSubmit2();' ....
-
The template you are using looks pretty simple and can be controled using javascript easily without overwriting the style.
Since you have a few elements (Header, navigation, side navigation, body, right side, footer) you can make the javascript to change these sections attributes. If you have a lot of links inside (like the navigation), just use the getElementsByTagName and loop through the objects the overwrite their style.
You still can overwrite the style tag (I never done that) or access it's childer (classes, definitions, etc)
You can view this for more details on the styleSheet
For colors, you can use the NoGray color picker
Good luck
-
That's a long script to read
You can use the drag and drop script in the NoGray javascript library or Yahoo UI library if you want something to run after the object is finished being draged.
When the dragging is over, retrive the x and y value using Javascript (the above libraries have built in values) and pass those values to php (either using ajax or iframes)
This should save the x and y coord
-
$labelchk=$_GET['chklabel'].value;
PHP doesn't have a value attribute on the variable, should be
$labelchk=$_GET['chklabel'];
-
There is very much "doable", just will take some JavaScript, naming structure and some logic.
What I did is each row of radio buttons will have the same name (option group) so when you select one, the rest will be unchecked.
I added some javascript to disable the radio buttons that are unchecked in the column, this way only one participant can have that placement.
If the use change their mind, the script will enable the other radio buttons. Also, I added a reset button to enable the radio buttons in case the user wants that.
You'll need to change the number of participants and the number of radio buttons in this script (I only made it for 5 participant and 5 placements), and keep the same structure
<script language="javascript"> // variable to set the number of participants (change this to the number of participants on the page) var par_num = 5; // variable to set the number of radio buttons (change this to 10 for your project) var rad_num = 5; function reset_radio(row){ // loop through the radio buttons on the row to see which // radio button is selected for (i=1; i<=rad_num; i++){ // if the radio button is selected, // uncheck it and enable the other radio buttons if (document.getElementById('par_'+row+'_'+i).checked){ // unchecking the button document.getElementById('par_'+row+'_'+i).checked = false; // turning the other radion buttons on change_disable_status(i, false); return; } } } // this function will change the status of the radio buttons function change_disable_status(ra, st){ for (i=1; i<=par_num; i++){ if (!document.getElementById('par_'+i+'_'+ra).checked){ document.getElementById('par_'+i+'_'+ra).disabled = st; } } } </script> <table> <tr><td> </td> <td>1</td> <td>2</td> <td>3</td> <td>4</td> <td>5</td> <td>Reset</td> <tr><td>Participant 1</td> <td><input type="radio" name="par_1" id="par_1_1" onmousedown="reset_radio('1');" onclick="change_disable_status('1', true);" /></td> <td><input type="radio" name="par_1" id="par_1_2" onmousedown="reset_radio('1');" onclick="change_disable_status('2', true);" /></td> <td><input type="radio" name="par_1" id="par_1_3" onmousedown="reset_radio('1');" onclick="change_disable_status('3', true);" /></td> <td><input type="radio" name="par_1" id="par_1_4" onmousedown="reset_radio('1');" onclick="change_disable_status('4', true);" /></td> <td><input type="radio" name="par_1" id="par_1_5" onmousedown="reset_radio('1');" onclick="change_disable_status('5', true);" /></td> <td><a href="#" onclick="reset_radio('1'); return false;">Reset</a></td> </tr> <tr><td>Participant 2</td> <td><input type="radio" name="par_2" id="par_2_1" onmousedown="reset_radio('2');" onclick="change_disable_status('1', true);" /></td> <td><input type="radio" name="par_2" id="par_2_2" onmousedown="reset_radio('2');" onclick="change_disable_status('2', true);" /></td> <td><input type="radio" name="par_2" id="par_2_3" onmousedown="reset_radio('2');" onclick="change_disable_status('3', true);" /></td> <td><input type="radio" name="par_2" id="par_2_4" onmousedown="reset_radio('2');" onclick="change_disable_status('4', true);" /></td> <td><input type="radio" name="par_2" id="par_2_5" onmousedown="reset_radio('2');" onclick="change_disable_status('5', true);" /></td> <td><a href="#" onclick="reset_radio('2'); return false;">Reset</a></td> </tr> <tr><td>Participant 3</td> <td><input type="radio" name="par_3" id="par_3_1" onmousedown="reset_radio('3');" onclick="change_disable_status('1', true);" /></td> <td><input type="radio" name="par_3" id="par_3_2" onmousedown="reset_radio('3');" onclick="change_disable_status('2', true);" /></td> <td><input type="radio" name="par_3" id="par_3_3" onmousedown="reset_radio('3');" onclick="change_disable_status('3', true);" /></td> <td><input type="radio" name="par_3" id="par_3_4" onmousedown="reset_radio('3');" onclick="change_disable_status('4', true);" /></td> <td><input type="radio" name="par_3" id="par_3_5" onmousedown="reset_radio('3');" onclick="change_disable_status('5', true);" /></td> <td><a href="#" onclick="reset_radio('3'); return false;">Reset</a></td> </tr> <tr><td>Participant 4</td> <td><input type="radio" name="par_4" id="par_4_1" onmousedown="reset_radio('4');" onclick="change_disable_status('1', true);" /></td> <td><input type="radio" name="par_4" id="par_4_2" onmousedown="reset_radio('4');" onclick="change_disable_status('2', true);" /></td> <td><input type="radio" name="par_4" id="par_4_3" onmousedown="reset_radio('4');" onclick="change_disable_status('3', true);" /></td> <td><input type="radio" name="par_4" id="par_4_4" onmousedown="reset_radio('4');" onclick="change_disable_status('4', true);" /></td> <td><input type="radio" name="par_4" id="par_4_5" onmousedown="reset_radio('4');" onclick="change_disable_status('5', true);" /></td> <td><a href="#" onclick="reset_radio('4'); return false;">Reset</a></td> </tr> <tr><td>Participant 5</td> <td><input type="radio" name="par_5" id="par_5_1" onmousedown="reset_radio('5');" onclick="change_disable_status('1', true);" /></td> <td><input type="radio" name="par_5" id="par_5_2" onmousedown="reset_radio('5');" onclick="change_disable_status('2', true);" /></td> <td><input type="radio" name="par_5" id="par_5_3" onmousedown="reset_radio('5');" onclick="change_disable_status('3', true);" /></td> <td><input type="radio" name="par_5" id="par_5_4" onmousedown="reset_radio('5');" onclick="change_disable_status('4', true);" /></td> <td><input type="radio" name="par_5" id="par_5_5" onmousedown="reset_radio('5');" onclick="change_disable_status('5', true);" /></td> <td><a href="#" onclick="reset_radio('5'); return false;">Reset</a></td> </tr> </table>
-
you can use the eval in the if statment as well
if(eval("document.formName."+myFields[i]+".value") == ""){
-
this should be the same concept as the drop down list you asked about
All you need to do is create the image layer (hidden with absolut position) on top of the image. once clicked, show the large image. Once the large image is clicked, hide it.
-
IE won't show the entier text in the select menu width is smaller than the option, you need to use a script to resize the width to "auto" when the menu is open using onclick events. It's a bit complicated since you'll need to track if the user clicked on the menu to open it or to close it.
Something like this
When the user click on the menu, a click counter will recorde the click to 1 which means the menu is open.
If the counter is 1 set the width to auto
the user make a selection, set the counter to 0
if the counter is 0 set the width to 150
Things you need to worry about is if the user click outside the menu to close (you'll need an onclick event on the body)
[SOLVED] form stuff
in Javascript Help
Posted
let's say your input field id is "name"
use this
make sure the field is not hidden by CSS.