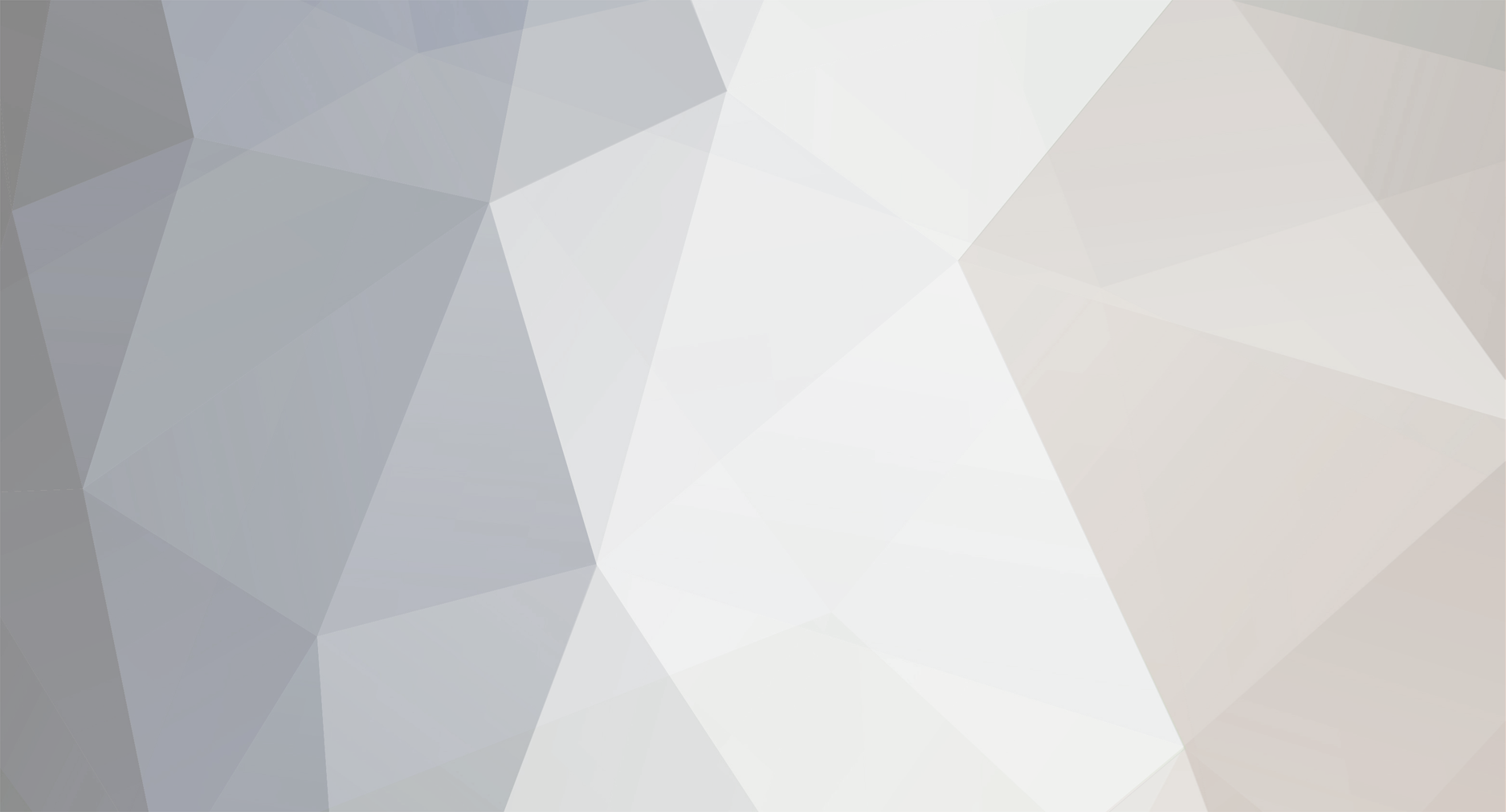
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
I am not sure if you fixed already or not, but for IE you'll need to set the overflow to hidden
[code]
newDiv.style.overflow = "hidden";
[/code] -
it's because of the paragraph tag <p> change your list to
[code]
<li class="style1">Professional web site design</li>
........
[/code] -
It's mainly firefox that doesn't cross session info between https and http, what you can do is set two sessions, one in the http side and one in the https side (two files with 2 redirection), maybe that'll work.
-
You have to declare the webSafeColor as a global array outside the colorDraw() function (so all functions can access it)
[code]
var webSafeColor = new Array();
function colorDraw(){ .....
[/code]
If you want, I wrote an artical a while back regarding color swatches at http://www.nogray.com/new_site/color_picker_javascript.php (notice I am not the best writer :D)
Also, if you want an advance color picker, you can download a copy at http://www.nogray.com/new_site/download.php which is a Photoshop style color picker, you can try it at http://www.nogray.com/new_site/nogray_app/color_picker/usage_example.html -
try to fix the TR> tag (make it <TR> with the <) in this code chunk.
[code]
<TD WIDTH=43 HEIGHT=32 COLSPAN=2>
<IMG SRC="images/30.jpg" WIDTH=43 HEIGHT=32 ALT=""></TD>
TR>
<TR>
[/code]
it may fix it, but I am not sure. -
The image will swap when you click on it, if you want the image to change when the link is clicked, you gotta add the onclick even to the link instead of the image.
[code]
<a href="#" class="faq_a" onclick="return swapImage(document.getElementById('IMG1'));"><img src="../images/plus.gif" align=left border=0 id="IMG1" name="IMG1">What Is ROM?<br></a>
<a href="#" class="faq_a" onclick="return swapImage(document.getElementById('IMG2'));"><img src="../images/plus.gif" align=left border=0 id="IMG2" name="IMG2">Is ROM FREE?<br></a>
[/code] -
[code]
<iframe frameborder="0" .....
[/code] -
child node for the table is <tbody><thead> and <tfoot> if you want to add elements using JavaScript.
-
what you need is offsetX and offsetY (you can read more about it in http://msdn.microsoft.com/library/default.asp?url=/workshop/author/dhtml/reference/properties/offsetx.asp)
but since firefox doesn't support it, you'll need to add a few function to emulate the results.
I did this after some research for a drag and drop library, and the function were from http://davidlevitt.com/2006/04/04/how-to-implement-offsetx-and-offsety-in-firefox.aspx
for IE and Opera, you can use
[code]
if (is_IE) e = event;
X = e.offsetX;
Y = e.offsetY;
[/code]
for firefox
[code]
X = window.pageXOffset + e.clientX - GetLocation(__getNonTextNode(e.explicitOriginalTarget)).x;
Y = window.pageYOffset + e.clientY - GetLocation(__getNonTextNode(e.explicitOriginalTarget)).y;
function __getNonTextNode(node) {
try {
while (node && node.nodeType != 1) {
node = node.parentNode;
}
}
catch (ex) {
node = null;
}
return node;
}
function GetLocation(el) {
var c = { x : 0, y : 0 };
while (el) {
c.x += el.offsetLeft;
c.y += el.offsetTop;
el = el.offsetParent;
}
return c;
}
[/code] -
you don't need to use setAttribute, just assign the values to the object properties directery
[code]
childdiv.id = divIdNameBtn;
childdiv.className = 'fenMaxBtn';
childdiv.title = 'Click to Maximize/Restore';
[/code]
and don't forget the ; after each line of code
[code]
childdiv.setAttribute('title','Click to Maximize/Restore') <--- missing a ;
[/code] -
This is pretty odd, but I think opera doesn't load the image for unknown reason. You can fix it by omitting the display:inline; all togather in the print style.
[code]
.print_only {}
[/code]
Let me know if this works. -
-
you'll need to change the image ids and names so each is uniqe (IMG1, IMG2, IMG3, etc..) then pass it to the function
[code]
<SCRIPT LANGUAGE=JavaScript>
intImage = 2;
function swapImage(img) {
switch (intImage) {
case 1:
img.src = "http://www.microsoft.com/library/toolbar/images/mslogo.gif"
intImage = 2
return(false);
case 2:
img.src = "http://msdn.microsoft.com/msdn-online/start/images/msdn-logo.gif"
intImage = 1
return(false);
}
}
</SCRIPT>
[/code]
and your onclick event will look like
[code]
<IMG id="IMG1" name="IMG1" src="http://www.microsoft.com/library/toolbar/images/mslogo.gif"
onclick="swapImage(this);">
[/code] -
this looks fine, post the code you use when you call this function.
-
If you go to my website http://www.nogray.com and download the JS library. You can access all the URL variables by including the base.js file.
after you downloading the zip file, you'll need to include this in the header
[code]
<script language="javascript" src="nogray_lib/base.js" type="text/javascript"></script>
<script language="javascript" defer src="nogray_lib/IE_loader.js" type="text/javascript"></script>
[/code]
and your script will access the id by
[code]
_GET['id'];
[/code]
Here is a link to all the base.js functions
http://www.nogray.com/phpbb/viewforum.php?f=2
Here is where you can download it.
http://www.nogray.com/new_site/download.php -
[code]
function directions(id){
window.open("directions.html?id=" + id,"directions","toolbar=no, location=no, directories=no, status=no, menubar=yes, scrollbars=yes, resizable=no, copyhistory=no, width=500, height=400");
}
[/code] -
you gotta set the border property to 0 in the image, and add the alternative text to make it XHTML valid tag
[code]
<img src="resize.php?id='.$row[1].'" border="0" alt="Alt text" />
[/code] -
-
do you mean this?
[code]
<title>WWW.ALVAHARDCORE.COM | News | news</title>
[/code]
without the | -
onkeypress is better since it's supported by most browsers (I think). And you can find out users searches by recording the search terms and how many times. Usually people look for similar products when they visit a site.
-
You need to clear your floated divs,
whenever you have a div floated right or left, you need to add a div to clear the float
[code]
.clear_float{clear:both; overflow:hidden; font-size:1px;}
[/code]
[code]
<div id="main">
<div style="float:left;">text</div>
<div style="float:left;">more text</div>
<div class="clear_float"> </div>
</div>
[/code]
-
oh yeah, you are missing the values in your options
[code]
<option selected='selected' value=0>0</option>
<option value=1>1</option>
<option value=2>2</option>
<option value=3>3</option>
<option value=4>4</option>
<option value=5>5</option>
[/code]
notice how I added "value=#" in the option. -
as wildteen88 said
[quote]
change:
<input type="button"
to
<input type="radio"
[/quote]
should do the trick.
You may need to add some text next to the radio button. -
IE does support png but by using a filter
You can visit this page for more details http://msdn.microsoft.com/library/default.asp?url=/workshop/author/filter/reference/filters/AlphaImageLoader.asp
Basiclly, you gonna need to replace your images with divs and set the images as backgrounds. and for IE you can use _background:none; and add the filter.
There are tons of javascripts out there that can handle the whole process as well.
check to see if the mouse in a certain div
in Javascript Help
Posted
You'll need to create an array foreach span to hold the span top left corner x and y, the width and the height in an array (IE and Firefox will add the padding to the width, while Opera won't)
When you let the mouse go, you can go through that array (not all the spans because you may use other spans for something else) and check if the x and y position withen which span
Here is a sample code, just to show how to get the element position
[code]
<script language="javascript">
var spans = new Array();
var is_Opera = (window.navigator.userAgent.search("Opera") != -1);
var is_IE = ((window.navigator.userAgent.search("MSIE") != -1) && !is_Opera);
function createSpans(){
var widths = new Array(100,100,50,125);
var heights = new Array(50,100,50,150);
var bgs = new Array("#ffffcc","#cceecc","#33ffcc","#000000");
var x = new Array(25,150,250,400);
var y = new Array(10,125,300,500);
var sp = "";
for(i=0; i<widths.length; i++){
sp = document.createElement("SPAN");
sp.className = "floating";
sp.style.backgroundColor = bgs[i];
sp.style.width = widths[i]+"px";
sp.style.height = heights[i]+"px";
sp.style.top = y[i]+"px";
sp.style.left = x[i]+"px";
sp.id = "clr_"+i;
document.body.appendChild(sp);
spans.push(new Array(sp.id,widths[i],heights[i],y[i],x[i],bgs[i]));
}
}
function w_span(evt){
var right_x = 0;
var right_y = 0;
var id= "";
if(is_IE) evt=event;
for(i=0; i<spans.length; i++){
right_x = spans[i][1]+spans[i][4];
right_y = spans[i][2]+spans[i][3];
if ((evt.clientX >= spans[i][4]) && (evt.clientX <= right_x) && (evt.clientY >= spans[i][3]) && (evt.clientY <= right_y)){
id = spans[i][0];
}
}
if (id != ""){
alert(id+ " "+document.getElementById(id).style.backgroundColor);
}
}
</script>
<style>
.floating {display:block;
position:absolute;
border:solid #000000 1px;}
</style>
</head>
<body onload="createSpans();" onclick="w_span(event);">
</body>
</html>
[/code]
P.S. If you download the NoGray JS Lib there are a lot of functions you can use (such as the drag and drop lib)