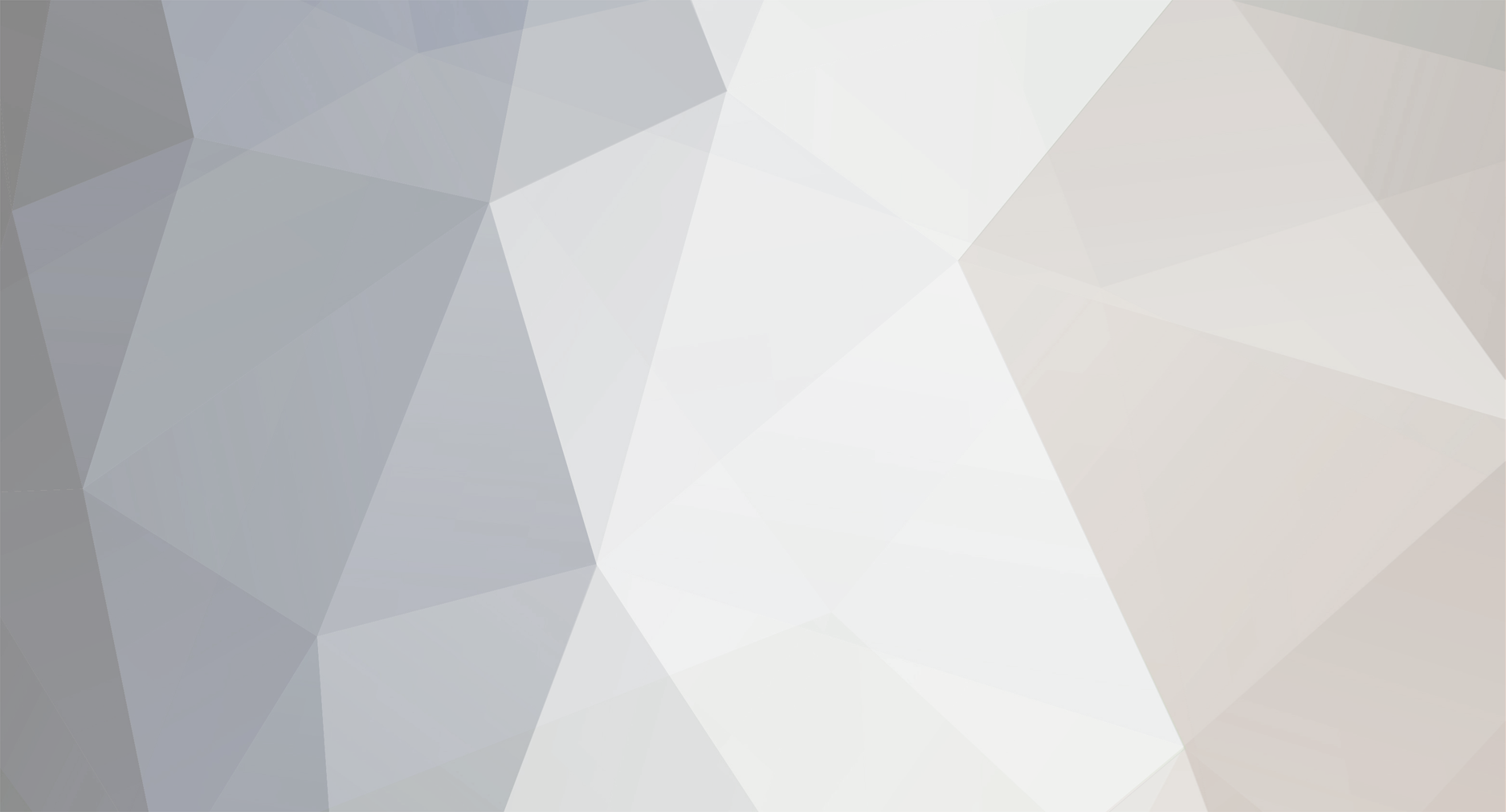
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
Actually, live search (with the result showing under the search field) is not usually a good feature. It's resource hog and no one really use it. If you want that feature, I would recommend you set a php file to put the top couple of hundreds search queries in an javascript array, and use that array to show the top searches on key press. This will speed up the list update and won't use a lot of resources.
-
just take this line out
[code]
window.onload=process_box('middle');
[/code]
and add the function to the body onload event
[code]
<body onload="swap(); process_box('middle');">
[/code]
and it should work. -
just use the same code I posted there, and instead of the div, make it a tr
[code]
<tr id="one" style="display:none;"><td>Hidden Text</td></tr>
[/code] -
check this page for full details on opening a window
http://msdn.microsoft.com/library/default.asp?url=/workshop/author/dhtml/reference/methods/open_0.asp
-
_TOP should be lower case _top
-
Hey there,
I notice this problem happens in Firefox, so here is how you can fix it.
add this to the top of the function
[code]
var div = document.createElement("DIV");
[/code]
replace this
document.getElementById('prot').innerHTML += txt;
with
[code]
div.innerHTML = txt;
document.getElementById('prot').appendChild(div);
[/code]
Let me know if this works -
I don't think you can have a div inside the select menu. If you using ajax, you can append the new options to the select using appendChild method.
-
Height and CSS are not very friendly, and I think your task is almost impossible using divs but I am not sure.
You can use some javascript to postion the box div when the page load, something like this will work
[code]
<script language="javascript">
function process_box(align){
var white_height = document.body.scrollHeight - document.getElementById('container-foot').scrollHeight - document.getElementById('container-head').scrollHeight;
if (align=='middle') var h = parseInt((white_height/2) - (document.getElementById('box').scrollHeight / 2));
else if (align=='bottom') var h = white_height - document.getElementById('box').scrollHeight;
else h = -1;
if (h > 0) document.getElementById('box').style.marginTop = h+"px";
}
window.onload=process_box('middle');
</script>
[/code]
You can change the 'middle' to bottom if you want to align the box to the bottom. -
Your image should be first in the code
[code]
<li><img src="images/arrow-right.gif"><a href="#">test</a></li>
[/code] -
try this, may work
[code]
if ($row->new_window == "1") {
echo '<b class="linkhead"><a href="'.$row->url.' target=\"_blank\">'.stripslashes(htmlspecialchars($row->link_text)).'</a></b>';
} else {
echo '<b class="linkhead"><a href="'.$row->url.'">'.stripslashes(htmlspecialchars($row->link_text)).'</a></b>';
}
[/code]
Also, check the data in your database and check if it's the correct value. -
maybe this
[code]
$rand = ((float)rand()/(float)getrandmax()) + 1;
[/code] -
ok, let's say you generated the key (encrypt your string and save the output), and saved it in a config file (enc_config.php)
You'll need to put the file below the public_html folder (so it's not accessible via a browser), set the permissions to the file so only php files from you server can access it, and finally encrypt that file using a php encryption program (you can google for that). This way you have an encrypted key in an encrypted file which make it almost impossible for someone to steel your data. -
The key and iv you generate are different in every page, so the encryption will be different. You should generate the key and iv and store them in an include file (or something similar) then use them when you need.
Or you can use the encryption class from the tutorial and it'll do all the work for you. -
sorry, I was talking about IE (object) Firefox embed the media player in the page. If you find a solution, please let me know.
-
There is a great tutorial for encryption and decryption in this site
http://www.phpfreaks.com/tutorials/128/0.php
You always can store cc numbers, just always make sure it very secure and encrypted. -
this should solve your problem
[code]
<script language="javascript">
function show(){
document.getElementById('extra_content').style.display = "";
}
function hide(){
document.getElementById('extra_content').style.display = "none";
}
</script>
<input type="radio" onclick="show();" id="radio1" name="radio" /> <label for="radio1">Yes</label><br />
<input type="radio" onclick="hide();" id="radio2" name="radio" /> <label for="radio2">No</label>
<div id="extra_content" style="display:none;">
This text is hidden
</div>
[/code] -
if the page on iframe, try to use this
[code]
<script language=javascript>parent.location='index1.php';</script>
[/code] -
You can use the indexOf() method
[code]
<script language="javascript">
function check_field(){
val = document.getElementById('tst').value;
if (val.indexOf(".") < val.length - 3){
alert("error");
}
else {
alert("good");
}
}
</script>
<input type="text" id="tst" name="tst" />
[/code] -
I set it up for 9am, you can change it to 9 bm by changing the 9 to 21 (military time) and the 8 to 20
-
media player (or flash object) is a plug in object which means it'll always render on top of everything else (regardless of the z-index).
You may need to hide it while the images are shown and show it when they are hidden. -
try this
[code]
<body onload="show_time();">
<script language="javascript">
var seconds = 15
function show_time(){
if (seconds > 0){
if (seconds == 1) var s="";
else var s="s";
document.getElementById('url_msg').innerHTML = "Please wait "+seconds+" second"+s+" for download";
seconds--;
setTimeout("show_time()", 1000);
}
else {
document.getElementById('url_msg').innerHTML = "Please click here to download";
}
}
</script>
<div id="url_msg">
</div>
</body>
[/code] -
I don't see any errors in the code you posted. If you are getting an error, please post it here. You can see the actual JS errors in FireFox by clicking on "Tools"->"JavaScript Council"
for the bigpoppa
AJAX stands for "Asynchronous JavaScript and XML" and by definition from domscripting.webstandards.org it means
[quote]
A scripting technique for silently loading new data from the server. Although AJAX scripts commonly use the soon to be standardized XMLHttpRequest object, they could also use a hidden iframe or frame. An AJAX script is useless by itself. It also requires a DOM Scripting component to embed the received data in the document.
[/quote] -
this may be helpfull
http://www.phpfreaks.com/forums/index.php/topic,95972.msg384362.html#msg384362 -
something like this will help
[code]
<body onload="show_time();">
<script language="javascript">
function show_time(){
var today = new Date();
var time_dif = "";
if (today.getHours() > 8) var odt = new Date(today.getFullYear(), today.getMonth(), today.getDate()+1, 9, 0, 0);
else var odt = new Date(today.getFullYear(), today.getMonth(), today.getDate(), 9, 0, 0);
var d_time = odt.getTime() - today.getTime();
var f_minutes = (d_time/1000)/60;
var i_minutes = Math.floor(f_minutes);
var seconds = parseInt((f_minutes - i_minutes) * 60);
var f_hours = i_minutes / 60;
var i_hours = Math.floor(f_hours);
var minutes = parseInt((f_hours - i_hours) * 60);
if (i_hours > 0) time_dif = i_hours + " hours, ";
if (minutes > 0) time_dif += minutes + " minutes ";
if (seconds > 0) time_dif += "and " + seconds + " seconds ";
time_dif += "until " + odt;
document.getElementById('time_div').innerHTML = time_dif;
}
setInterval("show_time()", 1000);
</script>
<div id="time_div">
</div>
</body>
[/code]
script works in firefox but not in explorer
in Javascript Help
Posted
[code]
<!-- Begin
var interval = "";
[/code]