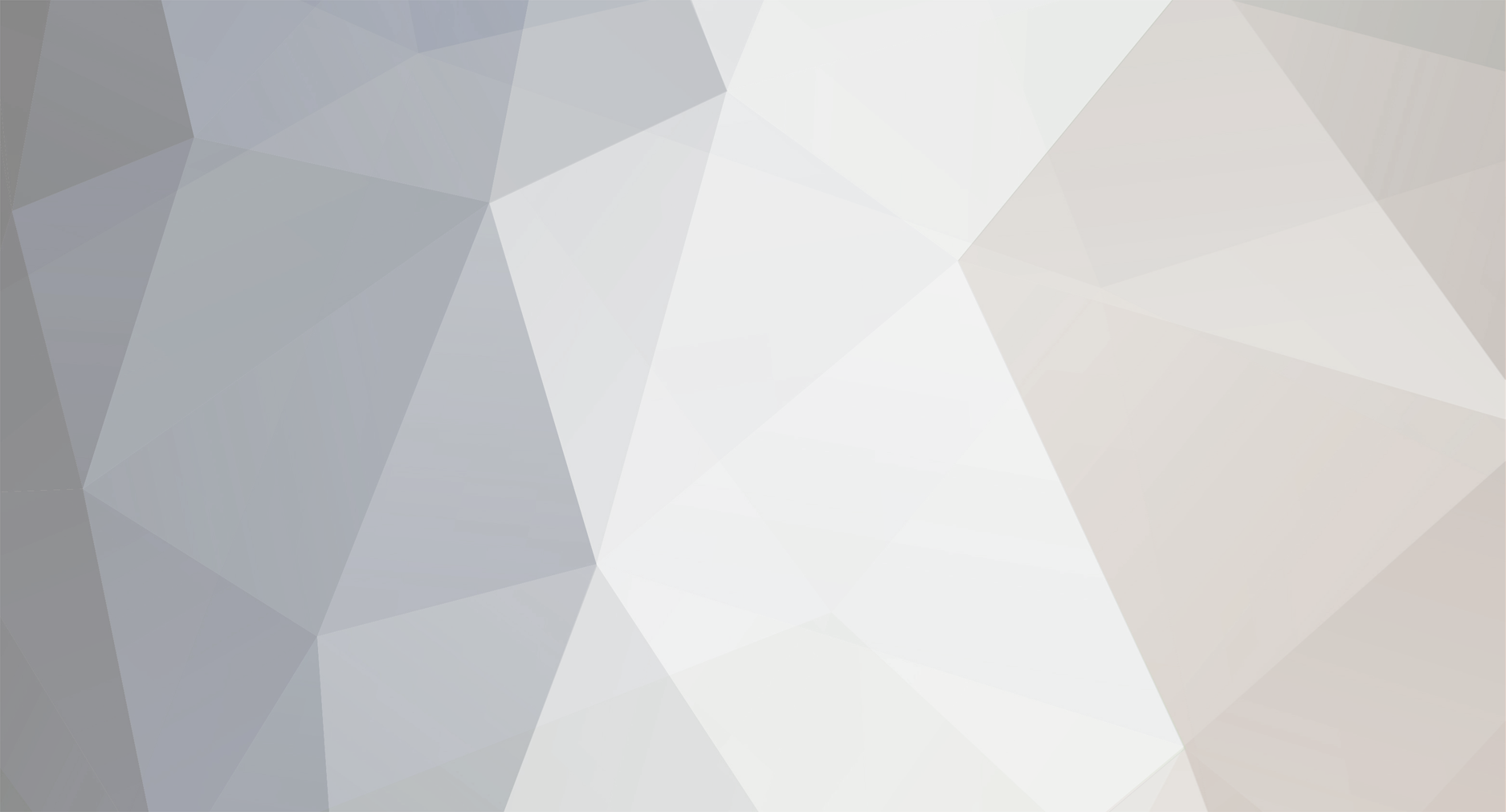
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Ok, here you go. I'm not even going to try and explain the logic <?php $generations = 3; //Create temp array to store results $genTableArray = array(); for($genCol = 0; $genCol <= $generations; $genCol++) { $genRowCount = pow(2, $genCol); $rowspan = pow(2, $generations) / $genRowCount; for($familyGenCount=0; $familyGenCount<$genRowCount; $familyGenCount++) { $personIndex = pow(2, $genCol) + $familyGenCount - 1; $rowIndex = $rowspan * $familyGenCount; $genTableArray[$rowIndex][] = "<td rowspan='{$rowspan}'>$personIndex - {$familyTreeArray[$personIndex]}</td>\n"; } } //Output the array into a table ksort($genTableArray); $familyTreeHTML = ''; foreach($genTableArray as $rowData) { $familyTreeHTML .= "<tr>\n" . implode("\n", $rowData) . "</tr>\n"; } ?> <html> <head> <style> td { text-align: center; } </style> </head> <body> <table border='1'> <?php echo $familyTreeHTML; ?> </table> </body> </html> I tried to write it to create the table directly, but couldn't figure out the formulas to determine which records to output on each row. So, I just processed the records similar to the original scripts but then dynamically populate an array.
-
Yeah, that is going to be much more complicated as you will need to build the rows in a more complex format. Not impossible, but will take some analytical and mathematical skills.
-
Here is a small script that will create a table in the general format of a family tree based upon the number of generations given. But, I would need to know the format of your input array to know how to populate the contents. <?php $generations = 5; $total_columns = pow(2, $generations); $familyTree = ''; for($gen = 0; $gen <= $generations; $gen++) { $gen_family_count = pow(2, $gen); $colspan = $total_columns / $gen_family_count; $familyTree .= "<tr>\n"; for($i=0; $i<$gen_family_count; $i++) { $familyTree .= "<td colspan='{$colspan}'>{$i}</td>\n"; } $familyTree .= "<tr>\n"; } ?> <html> <head> <style> td { text-align: center; } </style> </head> <body> <table border='1'> <?php echo $familyTree; ?> </table> </body> </html>
-
What is the format of the array and how do you want the output to look?
-
More advanced way to search for LIKE names...
Psycho replied to melting_dog's topic in PHP Coding Help
If you are exploding a name on spaces, using trim on the value would probably have no effect (unless the name has tabs in it). But, you should definitely use mysql_real_escape_string() as well as filter out empty values (in case the name had two or more successive spaces). Some slight modifications that I would make: $searchTerms = explode(' ', $productname); $searchTerms = array_filter($searchTerms); //Remove empties foreach ($searchTerms as &$term) { $term = "name LIKE '%" . mysql_real_escape_string($term) . "%'"; } $query = "SELECT * FROM product WHERE " . implode(' OR ', $searchWord); -
Have you tried reading the manual for those two functions? http://php.net/manual/en/function.imagecopyresampled.php http://us3.php.net/manual/en/function.imagecopy.php And I don't mean just skimming the description. Typically, if there are certain issues that can be encountered with a function the information is there if you take the time to read it. Specifically, I see this in the manual page for imagecopyresampled() I don't know if that is the cause of the problem you experienced, but it definitely sounds like a possibility.
-
if (isset($_POST['submit'])) { $query = "SELECT imgaddress, uploaddate FROM thumbs ORDER BY uploaddate"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { echo "<img src='{$row['imgaddress']}'/>\n{$row['uploaddate']}<br>\n"; } }
-
Why are you trying to use both file handler functions AND the database? If you want to use file handler functions, then use those to read the date of the file. Or, better yet, just use the database values to display the output. If you have records for all the images, there is no need to read the files from the directory!
-
This really isn't a PHP issue. You should first decide what you want your output to look like. THEN you should build the PHP code to generate that output. I would suggest building a basic HTML page with some mock data to put the content into the format you want. You can tweak whatever specifications you want until you are happy with the look. In my opinion: it is much easier to design outside of code and it is much easier to code when you have a set design. Trying to design within code can be done, but it routinely becomes more difficult than it needs to be.
-
Query "WHERE string is blank and date is at least 6 months ago.
Psycho replied to Ailis's topic in PHP Coding Help
The only line I see with mysql_query() is this one: $rs_specialty = mysql_query($query_rs_specialty, $LocalConnect) or die(mysql_error()); The $query_rs_specialty variable is just a string. If it was an invalidly formatted query it wouldn't generate the error. SO, the problem must with $LocalConnect. I assume that connection variable is set within the include file 'LocalConnect.php'. So, I'm guessing you are running a mysql_connect() function in there and assigning the result to this variable. So, open that file and see where you are doing that and add some error handling. E.g. $LocalConnect = mysql_connect('database', 'username', 'password') or die(mysql_error()); -
Well, you can dynamically find the start text using a regex and use your current logic //Find first instance of ( decimal ) preg_match("#\(\d+\)#", $content, $startText); $Start = $startText[0]; Or you can replace all of that logic with a single regular expression to return all the text that you need. I can provide that as well but need to know whether you need the start and end text in your results.
-
OK, so can you give a little more information. Is the value being saved incorrectly or is it a problem when the values are displayed?
-
Sounds like you are parsing some text and are wanting to use the "(NUMBER)" as a reference point. You will likely want to use regular expression (preg_match() I'm assuming) You could either use a regular expression to extract out the content you want or, if you want to use string functions but just need to know the position, you can use a regex to find the "(NUMBER)" value and use that value in your string functions.
-
is there a way to echo out all the variables that are in use ?
Psycho replied to jasonc's topic in PHP Coding Help
Also, instead of moving the debugging code around, I would suggest creating a debugging function that you can reference many places in your script. Make the function so that you can set a value to determine whether debugging is on or off. If off,t he function does nothing. You could also have the function compare the GLOBAL values between calls so you can see where the value is getting set or changes. -
Your logic is currently looking for $_REQUEST['update'], but you have no field called that in the form. Look for $_POST['leaderboard'].
-
OK, I was wrong. There is a simple solution no matter if the string is at the beginning or within the value UPDATE wp_usermeta SET meta_key = REPLACE(meta_key, 'wp_1_', 'wp_') WHERE meta_key LIKE '%wp_1_%'
-
No, you can't use a LIKE in your set statement - at least not like that. I believe the regex functionality in MySQL is only used for matching, not modifying. Is "wp_1_" at the beginning of the field value or 'within' the field value. If it is at the beginning there is a simple solution. If it is within, you may need to create a PHP script to process the records individually. Ifi "wp_1_" is at the beginning then this should work: UPDATE wp_usermeta SET meta_key = CONCAT('wp_', SUBSTRING(meta_key, 5)) WHERE meta_key LIKE 'wp_1_%'
-
OK, you really could work on your 'questions'. What you stated above is very confusing. Instead of talking about multiple if() statements and such. Just state what you are trying to accomplish, which I believe is: When the page is loaded and the user has not performed a search you want the page to displays the user's info. If the user has performed a search, then you want the page to display the information for the search. If that is the case, then you only need one conditional statement. IF the search field was submitted, then do a search on that value. If not, then use the value for the current user (you would need to have this stored in a session or cookie). if(isset($_POST['search'])) { $search_value = intval($_POST['search']); $where_clause = " goauld = {$search_value}"; } else { $search_id = intval($_SESSION['user_id']); $where_clause = " id = {$search_id}"; } // searches goaulds then displays them $query = "SELECT goauld, id FROM users WHERE {$where_clause} LIMIT 1"; $result = mysql_query($search3) or die(mysql_error()); $search1 = mysql_fetch_array($search2); $grab_goauld = $search1['goauld']; echo '<table width="300" height="5" border="1" align="center">'; echo '<th><center>Goauld Statistics</center></th>'; echo "<tr><td height='340'>$grab_goauld</td></tr>"; echo '</table>';
-
Query "WHERE string is blank and date is at least 6 months ago.
Psycho replied to Ailis's topic in PHP Coding Help
If that PHP error is due to that query failing then you need to view the error generated by the query. Echo mysql_error() to the page after runnign that query. You might have a typo in a field name, maybe the 'Date' field in the database isn't an actual date type, or ??? -
EDIT: Kevin beat me to it, but I'm posting this anyway since I provided some additional info as well. Several problems there: 1. Do not use short tags (e.g. <? ?>), instead use the full php tags <?php ?>. I suspect this is your problem based upon your results 2. Do not use the $_REQUEST global variable. Use the appropriate $_POST or $_GET You should also consider separating the logic from the presentation. I think there are some unhandled scenarios, but it is difficult to determine in that code. It would also be good practice to modularize your scripts. For example, I would do something like this if(!$logged_in) { if(!isset($_POST['username']) || !isset($_POST['password'])) { header('Redirect: login_page.php'); } else { include('authentication_script.php'); } } else { if($mode == 'edit') { include('edit_script.php') } else { include('display_script.php') } }
-
Well, you can't get 'true' pantone colors on a monitor because colors on a monitor are created via the additive (RGB) color system whereas pantones are created via the subtractive (CMYK) color system. If you want pantone equivalents you would need to get the appropriate conversions. So, you would have to build a database of all teh colors you want to support. Here is one reference: http://goffgrafix.com/pantone-rgb-100.php Then you could use that to build a custom color pick if needed.
-
Um, a database-driven web page? What you are asking is not a single function or process. It is several functions/processes to achieve the desired goal. If you have a form and can post the form to another (or the same page). You just need to check for the value passed in the form. In this case yor script would check for the value of $_POST['target2'] (or $_GET['target2'] depending on the method you set for the form). From there, you would want to validate/sanitize the value and then run a select query for the information you want to display. Then create the logic to output the data in the manner you want it. Also, some advice on the code above: If you are only using 'friend_id' and 'name', then you only need to include those in the SELECT query. Plus, I always suggest separating the logic of your code from the presentation. So, I would put the logic to generate the options at the top of the script and then output the options in the display portion of the code at the bottom of the script (or even in a separate file). Lastly, if you need to generate multiple select lists using database queries it would be advantageous to create a function that you can pass a query to. My take <?php $sql = "SELECT friend_id, name FROM contacts AS c JOIN players AS p ON c.friend_id = p.id WHERE c.player_id = $playerID AND is_active = 1 ORDER BY name ASC"; $que = mysql_query($sql) or die(mysql_error()); $target2Options = ''; while($list = mysql_fetch_array($que)) { $target2Options .= "<option value='{$list['friend_id']}'>{$list['name']}</option>\n"; } ?> <select name="target2" id="target2"> <option value=""></option> <?php echo $target2Options; ?> </select>
-
Outputting data from MySQL Table to columns in PHP
Psycho replied to Travis959's topic in PHP Coding Help
As I said, I only did all that because I was bored. It was a lot of work. If you want someone to DO this for you then you should be posting in the freelance forum. I'm certainly not willing to put more work into this out of the goodness of my heart. But, if you are willing to learn I will provide some guidance. 1. To get the Family total look at the logic I used generate the totals for the groups for field4. Create a temp array to store the totals for each family group until a change in family is detected. Then use that array to output the results. You can use the same display function I provided, but you will need to modify the last parameter. Right now, that parameter is a simple Boolean to determine whether you are displaying a 'normal' record or a summation for field4. Since you will want to use that same function for more than just two purposes you will want to change that parameter and the logic that uses it to use multiple values. 2. To get the subtotal of ALL families, just do the exact same thing as above. Create an array to store totals during the entire process. Once all records have been processed use the array to output the results. Again, you need to modify the function to output the results as you need them for the subtotal 3. I really don't understand your "requirements" regarding the "CUSTOMER ALW". 4. I also don't understand what you mean by "Adjustment Totals" need to be added. Where do these values come from and what are they added to? Just the ALW values? 5. For the grand total I would probably use an array for the ALW and adjustment totals (don't know if it would make sense to have one or two). BUt, then you would just create a foreach() loop to calculate the grand total for each month by comparing the values from the subtotal array and the adjustment arrays. And, again, I would put those value into an array (or more likely I would just change the values in the subtotal array). Then use the existing function to output the results from that array. EDIT: I just noticed that the format of the new tables is different than the originals. Average price and sales are switched. Is this correct? If so, then I would likely do more intensive modifications of the current process (the format of the temp arrays and the display function) to do that. -
If I have multiple parameters for my search clause I typically include logic for each clause and them implode them at the end. I usually use checks on the submitted values and not the variables that are set from those submitted values. Since you don't show where those variable are set I'll just use the variables. $whereParts = array(); if(!empty($low) && !empty($high)) { //Both low and high have values $whereParts[] = "se.age BETWEEN '$low' AND '$high'"; } elseif(!empty($low)) { //Only low has a value $whereParts[] = "se.age >= '$low'"; } elseif(!empty($high)) { //Only highhas a value $whereParts[] = "se.age <= '$high'"; } if(!empty($name)) { $whereParts[] = "se.ro='$name'"; } //Create the base query $sql = "SELECT private.username, se.age, se.ro, se.suc, se.bu, se.fu, com.in, com.ol, se.di, se.bo, se.uin FROM Reg_Profile_public AS se INNER JOIN Reg_Profile_Private AS private USING (uin) INNER JOIN Reg_Profile_public_Com AS com USING (uin)"; //Add the WHERE conditions if(count($whereParts)>0) { $sql .= "WHERE " . implode(' AND ', $whereParts); }
-
You will ONLY be able to do this if the value in the cookie ($_COOKIE['tntcon']) was set to lower or upper case before BEFORE creating the MD5() value. You MUST get the user input in the exact same case as was used to create the validation value. So, you will need to update the code that sets the cookie value AND update the code to check the POST value against the cookie value. Since you need to do the same thing in two different places you should create a function so you are guaranteed to be doing the exact same thing in both instances. function createChecksum($code, $salt) { return md5(strtolower(trim($code)).$salt); } Creating the value to store in the cookie $tntcon = createChecksum($captchaCode, 'a4xn'); setcookie("tntcon", $tntcon, time()+300); //5 minutes Verify user input if(createChecksum($_POST['security_word'], 'a4xn') == $_COOKIE['tntcon'])