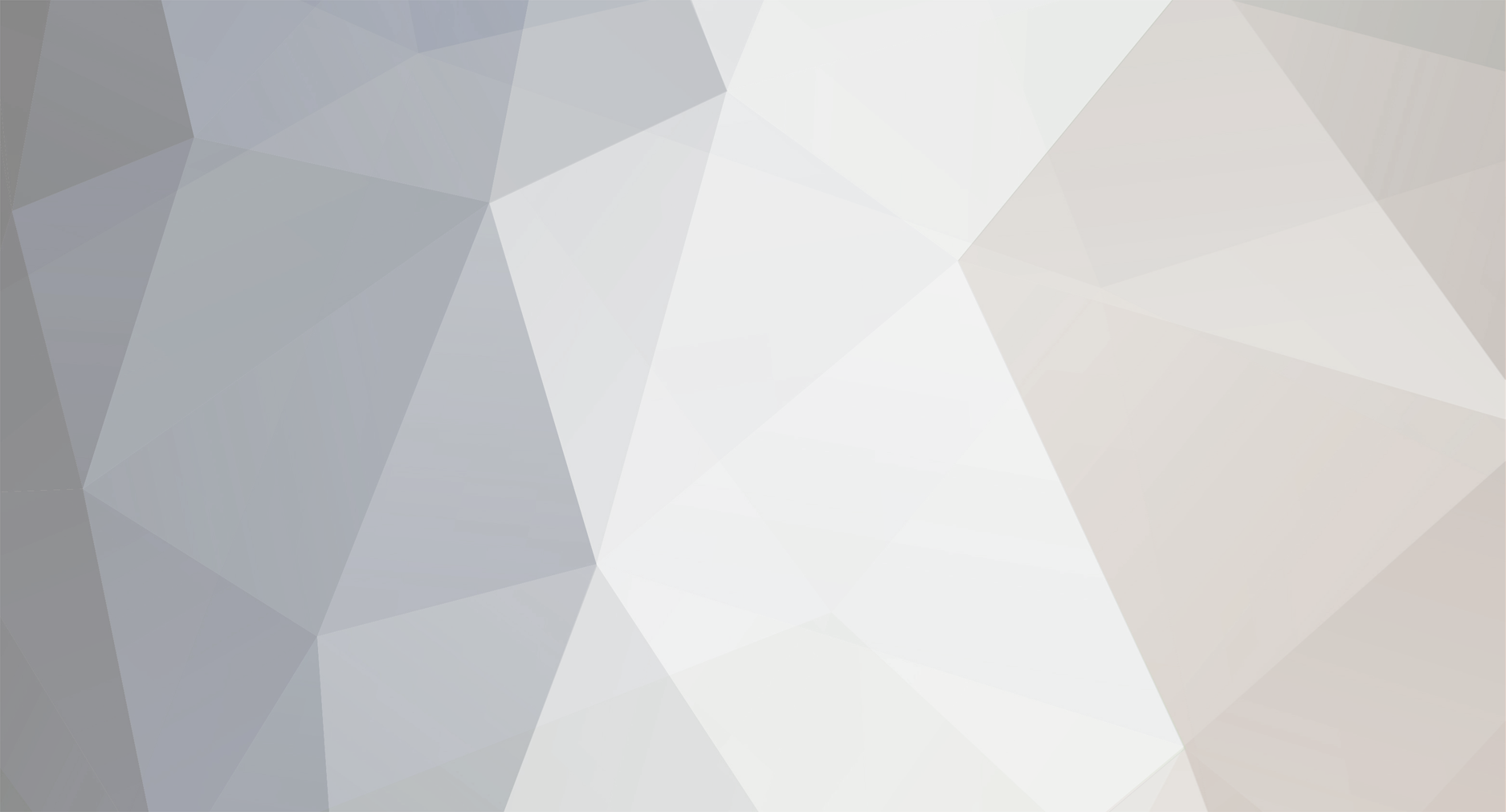
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I need a way to puch selected data to the beginning of an array.
Psycho replied to gibigbig's topic in PHP Coding Help
Then try the last query I posted. -
I need a way to puch selected data to the beginning of an array.
Psycho replied to gibigbig's topic in PHP Coding Help
Again, I don't even know if this is correct for your situation. I asked you to provide details on your database to make this more efficient. After rereading your query it looks like you have a "host" field in the "episode_links" table. If that field actually holds the name of the host then the query would be much simpler: SELECT *, IF(host='Fileserve.com', 0, 1) as primary_sort FROM episode_links WHERE episode_id = '$episode_id' ORDER BY primary_sort ASC, el.host ASC -
No offense to shlumph, but I think in his attempt to impart the logic for solving such a problem it became overgeneralized. My take: simply query the min and max from the database. Then create an array of all possible values from the min to max. Next, do a second query to find all the used values. Then simply remove the used values from the list of total possible values. Sample code //Query db for min/max and create array of total possible $query = "SELECT MIN(number) as min, MAX(number) as MAX FROM TABLE GROUP BY number"; $result = mysql_query($query); $record = mysql_fetch_assoc($result); $all_numbers = range($record['min'], $record['max']); //Query db for all used values and create array of used array $query = "SELECT number FROM TABLE"; $result = mysql_query($query); $used_numbers = array(); while($record = mysql_fetch_assoc($result)) { $used_numbers[] = $record['number']; } //Determine unused values $unused_numbers = array_diff($all_numbers, $used_numbers); If you have a VERY large number of records and/or a large possible range the performance could be affected. Alternatively you could just query all the records sorted low to high and iterrate through each one to see if the next value is the incremented by 1 from the previous and determine the gaps that way. Not sure which would be more efficient.
-
I need a way to puch selected data to the beginning of an array.
Psycho replied to gibigbig's topic in PHP Coding Help
Here is an example of how you could change your query to get what you want. It is only an example since I don't understand your database structure, names, etc. SELECT el.*, IF(h.hostname='Fileserve.com', 0, 1) as primary_sort FROM episode_links as el JOIN hosts as h on el.hostID = h.hostID WHERE el.episode_id = '$episode_id' AND el.moderated='1' ORDER BY primary_sort ASC, el.host ASC -
I need a way to puch selected data to the beginning of an array.
Psycho replied to gibigbig's topic in PHP Coding Help
Not sure what you mean by "host table" since the name of the table is "episode_links". Do the records in the "episode_links" table have a foreign key back to a hosts table with the values specified? If so, please show the fields from both tables and the ones that are associated. Also, you state you want the fileserver records to display first, do you have any preference on how the rest are ordered according to their host? FYI: This has nothing to do with arrays. This is a database question. -
You should consider setting the domain in the cookie dynamically so it will work in any environment and you won't have problems with misspellings and such. Do you even use sub-domains that you need to do that?
-
There's your answer - or do you not see the problem?
-
You can have the form post to a processing page on Server 1. After that page does the necessary validation of the data the processing page would then send the data to a receiving page on Server 2. That page would then do whatever it needs to with the data (e.g. save to a db) then send back a response to the processing page from Server 1. The Server 1 processing page would then either display a success or failure message depending upon the response received from server 2. For sending the data from Server 1 or Server 2 you could either send it by adding the data to the URL (Server 2 would processign the data from the $_GET variable), you could use cURL, or you could even send it via POST. See this page for an example on how you can resend POST data using PHP (I have not tested this myself): http://wezfurlong.org/blog/2006/nov/http-post-from-php-without-curl
-
Put this in test2.php echo $_SERVER["HTTP_HOST"]; What is output?
-
Here is some sample code to get you pointed in the right direction. As I stated before, put the files in a directory that is not publicly available. I will assume that folder is one level up from the current working directory and it is called "secured_docs": Page to display the links to the documents" if($logged_in) { //Query list of documents $query = "SELECT id, name, FROM documents ORDER BY name"; $result = mysql_query($query); //Display links to download the documents echo "Here are the secured documents:<br />\n"; echo "<ul>\n"; while($doc = mysql_fetch_assoc($result)) { echo "<li><a href=\"download_doc.php?id={$doc['id']}\" target=\"_blank\">{$doc['name']}</a><br /></li>\n"; } echo "</ul>\n"; } Page to download the documents from secured location (download_doc.php) if($logged_in && isset($_GET['id'])) { //Query for selected document $docID = (int) $_GET['id']; $query = "SELECT name FROM documents"; $result = mysql_query($query); //if(mysql_num_rows($result)!==0) { $document = mysql_fetch_assoc($result); //Include function to download files include('downloadFunction.php'); //Download the document output_file($file, true); } } Here is the function to download the files. I had this lying around which I picked up somewhere and have made changes to (downloadFunction.php) function output_file($file, $download=false; $name=false, $mime_type=false) { /* This function takes a path to a file to output ($file), the filename that the browser will see ($name) and the MIME type of the file ($mime_type, optional). If you want to do something on download abort/finish, register_shutdown_function('function_name'); */ //echo "<br><br>file: $file <br>name: $name <br> mime: $mime_type<br><br>"; //if(!is_readable($file)) die('File not found or inaccessible!'); /* Figure out the MIME type (if not specified) */ $mime_types=array( 'pdf' => 'application/pdf', 'txt' => 'text/plain', 'html' => 'text/html', 'htm' => 'text/html', 'exe' => 'application/octet-stream', 'zip' => 'application/zip', 'doc' => 'application/msword', 'xls' => 'application/vnd.ms-excel', 'ppt' => 'application/vnd.ms-powerpoint', 'gif' => 'image/gif', 'png' => 'image/png', 'jpeg' => 'image/jpg', 'jpg' => 'image/jpg', 'php' => 'text/plain', 'rtf' => 'application/msword' ); $size = filesize($file); $output_name = ($name) ? rawurldecode($name) : rawurldecode(basename($file)); $mime_type = (isset($mime_types[$file_ext])) ? $mime_types[$file_ext] : 'application/octet-stream'; @ob_end_clean(); //turn off output buffering to decrease cpu usage // required for IE, otherwise Content-Disposition may be ignored if(ini_get("zlib.output_compression")) { ini_set("zlib.output_compression", "Off"); } header("Content-Type: {$mime_type}"); if ($download) { //Force download header("Content-Disposition: attachment; filename='$output_name'"); } header('Content-Transfer-Encoding: binary'); header('Accept-Ranges: bytes'); /* These three lines basically make the download non-cacheable */ header('Cache-control: private'); header('Pragma: private'); header('Expires: Mon, 26 Jul 1997 05:00:00 GMT'); // multipart-download and download resuming support if(isset($_SERVER['HTTP_RANGE'])) { list($a, $range) = explode('=', $_SERVER['HTTP_RANGE'], 2); list($range) = explode(',', $range, 2); list($range, $range_end) = explode('-', $range); $range = intval($range); $range_end = (!$range_end) ? ($size-1) : intval($range_end); $new_length = ($range_end-$range+1); header("HTTP/1.1 206 Partial Content"); header("Content-Length: $new_length"); header("Content-Range: bytes $range-$range_end/$size"); } else { $new_length = $size; header("Content-Length: $size"); } /* output the file itself */ $chunksize = 1*(1024*1024); //you may want to change this $bytes_send = 0; if ($file = fopen($file, 'r')) { if(isset($_SERVER['HTTP_RANGE'])) { fseek($file, $range); } while(!feof($file) && (!connection_aborted()) && ($bytes_send<$new_length) ) { $buffer = fread($file, $chunksize); print($buffer); //echo($buffer); // is also possible flush(); $bytes_send += strlen($buffer); } fclose($file); die(); } //Cold not open the file die('Error - can not open file.'); }
-
Are you testing this on a local install or on your production server?
-
Put the documents in a directory that is not publicly available and use PHP to deliver the documents to the user rather than providing them a direct link. What type of documents are they: pdf, doc, txt, ??? And, how do you maintain the document list: in a database, do you read the directory, hard-coded list, ???
-
I'll add that it is also used to simply end a function and does not have to return a value. In fact, I would say that is it's primary function with return-ing a value as a secondary. For example, let's say you have a function that processes an array or records. The records are sorted according to a date value (low to high) and you only want to process the records that are on or before the current date. The function might look something like this: function processOrder($dataArray) { $today = time(); foreach($dataArray as $record) { if($record['timestamp']>$today) { return; //Exit function } //Code to process record goes here } }
-
Actually, because constants aren't interpolated into strings like that, leaving out the quotes for associative array indices within double quotes is completely valid. Not saying you are wrong, but this is what the manual states: http://php.net/manual/en/language.types.array.php As the manual states it works, but is bad practice. Maybe there is some exceptions within a double-quoted string, but I prefer to adhere to a standard for my own sake.
-
If it is the page that is running then, most likely you can't use PHP anyway since it is an html page not a php page. Of course, it is possible the server is configured to process html pages for php code anyway, although it is not common that I am aware. So, on any page where PHP is interpreted you could do this: preg_match('/([\d]+)\..{3,4}(\?.*)+$/', $_SERVER['REQUEST_URI'], $match); $number = $match[1]; I modified the regex expression provided by Solarpitch to allow for pages with different extensions: html, htm, php, etc. as long as they are 3 or 4 characters. Also, the URL may optionally have additional parameters, such as http://www.mysite.com/our-company-t-2.html?id=34&cat=6
-
http://code.google.com/apis/ajaxlanguage/
-
Right you are! I misread your previous post where you used strip_tags(). I'm using IE on this PC and sometimes small code blocks are partially hidden where I have to scroll up and down to only see one line at a time. Sorry 'bout that. Although, I would think you would only use strip_tags() in the SELECT query if you used it on the INSERT query.
-
@rwwd: You are absolutely correct that any data from the user must be sanitized. However, your usage of stripslashes() and mysql_real_escape_string() is backwards. mysql_real_escape_string() will add slashes for characters that need to be escapes and then stripslashes will remove it. So, you would end up with the original unsanitary data. You would want to use strip slashes first ONLY if the input is on a server where POST/GET data has slashes automatically added because of magic quotes being enabled (which it shouldn't be). This is detailed in the manual. http://us2.php.net/manual/en/security.magicquotes.php http://us2.php.net/manual/en/security.magicquotes.disabling.php
-
Yes, of course. As I stated above, the "pages" are accessible to the user with direct URLs. It's just that those pages (files) don't include any real logic - they simply call secured files that have all the logic. There is no way a browser or search engine can know that the page was created with files in the public space or not. They only react to the final content that is delivered.
-
I have a project where ALL of the files with any logic are secured in non-public folders on the server one level up from the public folder. My homepage (index.php) only has a couple of lines of code to point it to the real files with the PHP Logic: <?php error_reporting(E_ALL | E_STRICT); $_PATHS['root'] = dirname(dirname(__FILE__)) . DIRECTORY_SEPARATOR; include ($_PATHS['root'].'main.php'); exit(); ?> Of course I do have other pages that are publicly available. But, all they do is set parameters for the modules to load then call the index.php page. Here is the page to access the management functions of the site. It would be accessed at the url: http://www.mysite.com/manage/index.php <?php $module = "manage"; include("../index.php"); ?> As you can see, all it does is set a value for the $module and then calls the index.php page at the "web" root. That page, then calls the logic files that are secured in non-public directories.
-
The problem with that approach is that if there is ever a PHP parsing error, the raw code *could* potentially be exposed. That exposed code could then be used to find weaknesses in the site and infiltrate it. This has happened in the past with some well-known sites. Instead, if you have files that should never be accessed directly, there is a very simple, fool-proof technique: don't include them in the public directory! In other words, do not point the root of the web address to the root of your folder structure. For example, you could create a directory structure such as this: filesystem root | --classes | --common | --htdocs (public folder) | --inlcudes | --Templates The index.php file for the home page would go into the htdocs folder and you would make that the root for the website. There is no way for users to access the other folders above. But, the PHP code could access those files via include() or other means. However, you have to include files such as images, javascript, etc, in the htdocs folder or subfolders because those are "requested" through the browser not the PHP code.
-
PHP is executed server-side. You can't put PHP code into a link expecting it to be executed when the user clicks the link. The link needs to point to a php on the server that will get called when the user clicks a link. So, you could create a page (say deleterecord.php) then for each link have the href pointed to that page and add an additional parameter to include the record id. So the link would look something like this <a href="deleterecord.php?id=123">Delete this record</a> Then you need to create that page to take the id passed on the query string and perform the delete operation.
-
This topic has been moved to CSS Help. http://www.phpfreaks.com/forums/index.php?topic=309496.0
-
This is not a PHP problem, but an HTML/CSS one. (Moving to CSS forum) I suppose you could make list items work for this, but I don't know why you would want to. They would probably take more style attributes to get what you want. I would suggest that you use DIVs or even tables to display the data in that manner. Here is one possible solution: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Index</title> <style type="text/css"> #latest_media div { text-align: center; margin-left:110px; background-color:#F00; float: left; } </style> </head> <body> <div id='latest_media'> <div><img src="images/2.PNG" /><br />Description</div> <div> <img src="images/1.PNG" /></div> </div> </body> </html>
-
That is not a PHP4 vs. PHP5 issue. Your query is using strait-quotes for what appears to be a field name. You need to use backquotes for field names. Plus, you have the array index (category) as a constant instead of a string. You should use single quotes for the array index and enclose the array variable inside curly brackets Try this: $sql = "SELECT * FROM products WHERE `{$_REQUEST['category']}` = 'Y' ORDER BY price ASC";