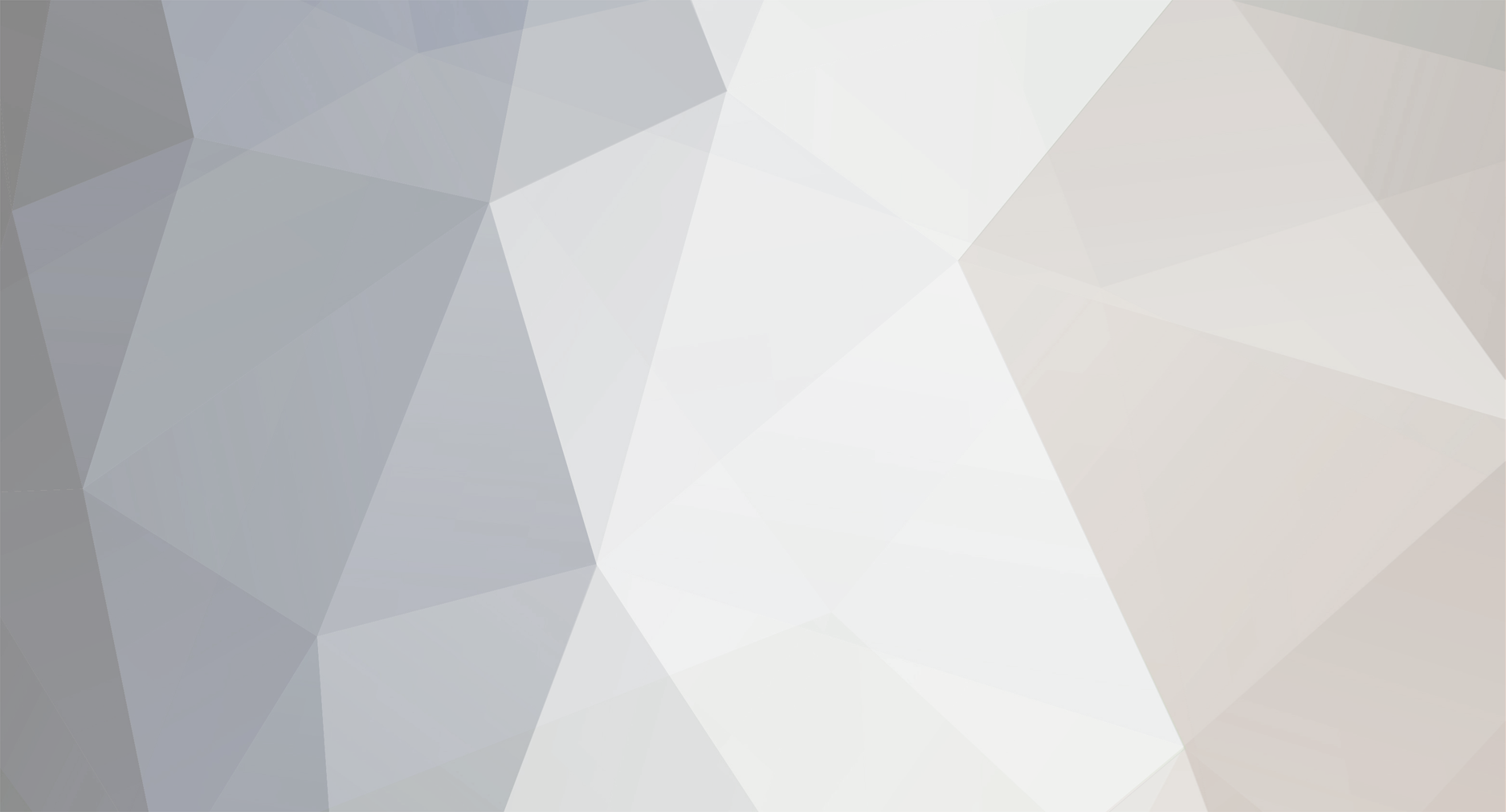
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
am i in the right direction with this function?
Psycho replied to justAnoob's topic in Javascript Help
So...create them as closed. Just include style="display:none;" in all of the divs by default. Then reverse the logic of your function so that it "assumes" the initial state is closed. The JavaScript style properties are not set via the inline properties. I would also modify the function to just two lines: function clicked(element) { var divObj = document.getElementById(element); divObj.style.display = (divObj.style.display=='block') ? 'none' : 'block'; return; } -
I don't think you are understanding/utilizing AJAX correctly. You cannot dynamically "run" PHP code client-side. The format you have will work - assuming you implement the AJAX correctly. Use a link such as you have above: <a href="javascript:ajaxpage('login.php','contentarea');">Link to login</a> Then, when you call the function ajaxpage(), it should make a request via AJAX to the provided page (i.e. the first parameter. That page should provide a response of the content you want displayed in the div. The javascript function should include an onreadystatechange() process to "capture" when the response is made. The javascript can then simply populate the div with the response. There are countless tutorials out there that can show you how to do this.
-
Disable a form by removing the submit function
Psycho replied to gerkintrigg's topic in Javascript Help
Well, there is nothing that will prevent it 100% since users may have JavaScript disabled. So, you'd better have some logic server-side as well. Anyway, all you need to do is have a global variable to track whether the form should be submittable or not. Then add an onsubmit() trigger to the form to call a function. That function will return true/false based upon the global variable. You can get more create and also disable the submit button or show other messages to the user. <html> <head> <script type="text/javascript"> var allowSubmit = true; function changeSubmit(allowState) { //UNCOMMENT NEXT LINE TO ALSO DISABLE SUBMIT BUTTON //COMMENTED OUT FOR DISPLAY PURPOSES // document.getElementById('submit').disabled = !allowState; allowSubmit = allowState; } function checkFormSubmitState() { //ONLY THE RETURN LINE IS REQUIRED //THIS LINE ADDED FOR DISPLAY PURPOSES alert((allowSubmit)?'Form will now be submitted\nReturn true':'Submit not allowed\nReturn false') return allowSubmit; } </script> </head> <body> <form onsubmit="return checkFormSubmitState();" id="test"> Field 1:<input type="text" name="field1" /><br /> Field 2:<input type="text" name="field2" /><br /> <button type="submit" id="submit">Submit Form</button> </form> <br /><br /> <button type="submit" onclick="changeSubmit(true);">Allow Submit</button> <button type="submit" onclick="changeSubmit(false);">Disable Submit</button> </body> </html> -
A google search for "PHP create thumbnail" came up with several tutorials ont he subject. Really too much to try and emaplain in a forum post. Try reading a tutorial and implementing it into your script. Then post back if you run into any problems.
-
From the OPs post I assumed that Yahoo mail was just one example. If you need to do this with multiple sites, you might want to look into using cURL: http://php.net/manual/en/book.curl.php
-
Your example doesn't jibe with your description. You state Then you state that in the example code player 1 should be paired with (300, 300). But, player 1's current position is 50, 50 which is ~353 units away. Aside from that problem, I think you are going to have a hard time getting the "perfect" solution. What should happen if there are three players which all have the same two available options? Which two get one of the two and what do you do about the third player? Assuming that when there is a comflict sach as the above that one player will not get a matching, here is the logic I would follow: Iterrate through the players finding ones with only one available match and make that match. When that is done, some players which had multiple possible matches may only have one available since some were used up on the first pass. So, repeat the process above until you get through one complete pass with no players with only one available match. Then do a pass looking for players with 2 or 1 available matches and assign one. Run that again and again until there are none left. Then do for players with 3-1 possible matches and so on. The problem with all of that is that it would not necessarily always do the optimal selection and you may get some players that do not get a match when they could of when matched int he "optimal" manner. Here's an example: Player / Possible matches Bob : A, B Tim : B, C Jim : A, C It is possible to match each of them to unique values such as Bob:A, Tim:B, Jim:C But, if you were to match Bob with A and Tim with C, you would have no available matches for Jim. Again, I think trying to get the perfect solution would be very complex as it would have to look at all conceivable pairings before determining the best matches.
-
help plz -----problem with header redirection --------urgent
Psycho replied to coolabhijits's topic in PHP Coding Help
The logic is messed up. For example if(!$result)//checking for null { go(); } 1. That does NOT check for null (i.e. empty results), that check to ensure there were no errors in the query. 2. You are not escaping the variables in your query and are leaving yourself open to SQL injection. 3. The go() function echo's text to the page before running the header() function. In which case you should have received an error stating that you cannot resend headers after content is sent. Wht is the purpose of the variable $i in that function? Makes no sense. <?php ob_start(); session_start(); function go() { header("Location : http://127.0.0.1/ajax/data_entry/"); exit; } if(isset($_POST)) { //if started if (!$con = mysql_connect("localhost","ultra","9968015024")) { die('Could not connect: ' . mysql_error()); } //echo "Connection established"; mysql_select_db("admin", $con); $name = mysql_real_escape_string($_POST["name"]); $password = mysql_real_escape_string($_POST["password"]); $query = "SELECT password FROM login where name='{$name}'"; $result = mysql_query($query); if(!$result) //Check for error { die("Error running query: $query<br>\n\n" . mysql_error()); } if(mysql_num_rows($result)===0)//checking for null { go(); } $row = mysql_fetch_assoc($result); if($row['password']==$password) //Checking the password { $_SESSION['admin'] = $name;//setting session for admin go(); }//password comparision if ending mysql_close($con); //echo "\n 3header redierection error"; go(); }//if check ending ?> -
You only need one function, just pass a true/false. Here is a working example -I'll let you convert it into the PHP code as needed <html> <head> <script type="text/javascript"> function checkAll(formObj, groupName, checkState) { var groupObj = formObj.elements[groupName]; for (var i=0; i<groupObj.length; i++) { groupObj[i].checked = checkState; } return true; } </script> </head> <body> <form> Box 1 <input name="picbigid[]" type="checkbox" value="value1" /><br> Box 2 <input name="picbigid[]" type="checkbox" value="value2" /><br> Box 3 <input name="picbigid[]" type="checkbox" value="value3" /><br> Box 4 <input name="picbigid[]" type="checkbox" value="value4" /><br> Box 5 <input name="picbigid[]" type="checkbox" value="value5" /><br> <input type="button" name="CheckAll" value="Check All" onClick="checkAll(this.form, 'picbigid[]', true);" /> <input type="button" name="UnCheckAll" value="Uncheck All" onClick="checkAll(this.form, 'picbigid[]', false);" /> </form> </body> </html>
-
Inserting data in an array into a mySQL table using php
Psycho replied to husslela03's topic in PHP Coding Help
Why explode $file_contents into an array? Just store it as-is in the the database and then explode when you use it later if needed. Otherwise, you can use serialize() and unserialize() to contert/unconvert an array. -
<?php function getHTMLTitleAndBody($fileName) { //Read the HTML content $content = file_get_contents($fileName); //Extract the title preg_match("/<title[^>]*>(.*)<\/title>/is", $content, $titleMatch); $result->title = $titleMatch[1]; //Extract words from body up to X number of characters preg_match("/<body[^>]*>(.*)<\/body>/is", $content, $bodyMatch); $result->body = array_shift(explode("\n", wordwrap(strip_tags(trim($bodyMatch[1])), 30))); return $result; } $file = "temp.htm"; $results = getHTMLTitleAndBody($file); echo "<b>Title:</b> {$results->title}<br><br>\n"; echo "<b>Body:</b> {$results->body}"; ?>
-
DELETE FROM not working deletes wrong row
Psycho replied to marshmellows_17's topic in PHP Coding Help
One more thing: The code should do the delete before it creates the delete links. Otherwise, when you do a delete, the page will display the record as still available for deletion after the record is deleted. -
DELETE FROM not working deletes wrong row
Psycho replied to marshmellows_17's topic in PHP Coding Help
The problem is that you first loop through ALL the records in the database to create the delete links. In that process you define two variables ($tenants_house_name & $tenant_house_id) based upon the values from each record. Fine so far... But, then at the end of the script you check if the user had submitted a delete request via the GET object (no problem there, either) The problem is that if the script does identify that a delete request was made it deletes the record with the ID from the variable from $tenant_house_id! That variable was only used in the loop to create the delete links - so it will always be the value from the last record in the database. You should be using $_GET['tenant_house_id'] which was the value passed in the delete request. In addition, you should be escaping that value before running it in a query. Also, you should not be using ectract() in the while loop. You already "extract" the values you need on the following two lines and extract() is considered unsafe by many. -
So, back up your database before you run your tests. If you run into problems restore your database. Basic development/testing processes.
-
That's why you should always test your code. You should have a separate development environment where you can back-up and restore the database. Besides, you can also test any DELETE as a SELECT first to validate it is selecting the correct records before you do a DELETE
-
You want to create ONE query with all the values for the record. Not run a query for each value mysql_connect("localhost", "root"); mysql_select_db("metacritic"); $regex6 = '/gave it a.*/'; preg_match_all($regex6, $curl, $done['comment']); $i = 1; $fields = array(); $values = array(); //Create arrays of the fields and values foreach ($done['comment'][0] as $comment) { $fields[] = "`comment{$i}`"; $values[] = "'" . mysql_real_escape_string(substr($comment, 50)) . "'"; $i++; } //Build the query $fieldList = implode(', ', $fields); $valueList = implode(', ', $values); $query = "INSERT INTO games ({$fieldList}) VALUES ({$valueList})"; //Run the query mysql_query($query);
-
You will definitely need to add a column or two to the table to accomplish this. Here is what I would do: Add an ID column that is an auto-increment field. Then when someone adds status(es) run a subsequent query to delete any that are more than the max you want available. Something like this should work: DELETE FROM table WHERE userid = '$userID' AND id NOT IN (SELECT id FROM table WHERE userid = '$userID' ORDER BY id LIMIT 0, 150)
-
I typically prevent double submissions by doing a header redirect right after I process the POST data. Once the redirect occurs there is no POST data, so the user can refresh all they want and there won't be a double submission. However, the user using the back button is a different story. In that situation I would create the form with a hidden field with some random value. When the form is submitted I would save that random value with the data. Then if the user hits the back button and resubmits I can check that the random value already exists and not allow the resubmission.
-
PHP Using either Dropdown or Text field if "other"is in dropdown
Psycho replied to jay7981's topic in Javascript Help
Where's your query to insert the record? I can only suspect that you are using the POST value ($_POST['access']) instead of the variable $access in your query. Also, since you have an option for "Select level" I hope you are implementing logic to prevent that value from being processed. -
PHP Using either Dropdown or Text field if "other"is in dropdown
Psycho replied to jay7981's topic in Javascript Help
Here's a quick example of how the form might work: <html> <head> <title></title> <script language="javascript"> function showOther(fieldObj, otherFieldID) { var fieldValue = fieldObj.options[fieldObj.selectedIndex].value; var otherFieldObj = document.getElementById(otherFieldID); otherFieldObj.style.visibility = (fieldValue=='--other--') ? '' : 'hidden'; return; } </script> </head> <body> <form name="form1" method="post"> Choose a color: <select name="color" onchange="showOther(this, 'other_color');"> <option value="Red">Red</option> <option value="Green">Green</option> <option value="Blue">Blue</option> <option value="Purple">Purple</option> <option value="Yellow">Yellow</option> <option value="--other--">--Other--</option> </select> <input type="text" name="other_color" id="other_color" style="visibility:hidden;"> <br /><br /> Choose a number: <select name="number" onchange="showOther(this, 'other_number');"> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="--other--">--Other--</option> </select> <input type="text" name="other_number" id="other_color" style="visibility:hidden;"> </form> </body> </html> Then in your processing page, you just check for the 'other' selection and set the value accordingly: <?php $color = ($_POST['color']=='--other--') ? $_POST['other_color'] : $_POST['color']; $number = ($_POST['number']=='--other--') ? $_POST['other_number'] : $_POST['number']; ?> -
I was bored and found this an interesting activity. Here is a rewrite of what you have above in what I believe is a more efficient manner - especially for the logic of parsing the external page. <?php //This function is called when the cached data is nonexistent or is stale //Will screen scrape the URL and parse the data function getWeatherData() { $url = "http://www.weatheroffice.gc.ca/city/pages/on-69_metric_e.html"; $urlContents = file_get_contents($url); preg_match('/weathericons\/(\d*)\.gif/', $urlContents, $idNumMatch); $idNumber = $idNumMatch[1]; preg_match('/<dt>Condition:<\/dt>.*?<dd>(.*?)<\/dd>/s', $urlContents, $conditionMatch); $condition = $conditionMatch[1]; if ($idNumber=='50') { $idNumber = '31'; } if (is_numeric($idNumber) && $idNumber>0 && $idNumber<50) { $output = "<img src=\"images/weather/{$idNumber}.png\" style=\"border-style:none;\">";; } else { $output = ' '; } $output .= " <span id=\"number\">$condition</span> $iden3"; return $output; } //This function is "generic" in that you can use it in any situation //where you would like to store cached data for a period of time function getCurrentData($cacheFile, $getDataFunction, $secondsToCache) { //Check if cache file exists & is current if (file_exists($cacheFile) && (time()-filemtime($cacheFile))<$secondsToCache) { //Read data from cache $cacheFileHandle = fopen($cacheFile, 'r'); $currentData = fread($cacheFileHandle, filesize($cacheFile)); fclose($cacheFileHandle); } else { //Get current data and write to cache $currentData = call_user_func($getDataFunction); $cacheFileHandle = fopen($cacheFile, 'w'); fwrite($cacheFileHandle, $currentData); fclose($cacheFileHandle); } return $currentData; } //Get the "current" data by calling the function passing the following: // - cache file name // - function to refresh the cache data // - seconds for the cache file to persist $weatherData = getCurrentData('cache\weather.txt', getWeatherData, 2000); echo $weatherData; ?>
-
The logic seems a little odd. I would create a function to return the content and have that function do the logic of pulling from a cache file or getting new content. Also, no need to have a log file with the time when the buffer (should be called cache) file was last updated. Just check the last modified time of the cache file. However, your current code should be producing an error because of this line elseif { $buffer = file_get_contents($lastRunBuf); There is an elseif with no condition.
-
counting specific words in string (reliably)
Psycho replied to gerkintrigg's topic in PHP Coding Help
??? How are "heard" or "hearing" not words? If I was manually doing a word count I would count them as words. If you want code to not count different tenses of words you have a very, very long project on your hands. You will need to build an extensive dictionary of words and their tenses. As for the html tags, I would suggest using preg_replace to remove any tags before doing the word counts (and also use it to remove any multiple spaces). Then do an explode to create an array of each word in the string. Then use array_unique() to end up only with the unique words. You will then need to create a custom function to remove different tenses of words. -
What do you think of the new look of these forums?
Psycho replied to smerny's topic in PHPFreaks.com Website Feedback
No, it's just not you. One workaround is to first paste into an application that recognizes formatting, such as MS Word. You can then copy out of word and into another application and the line-breaks will be there. -
What? How do you get a rating of two would display three stars? It's simply an average. You simply add up ALL the ratings and divide by the number (i.e. count) of ratings to get the average. Then round as needed. Ex: Ratings of 3, 4, 3, 2, 1, 5, 2 Sum = 20 Count = 7 Average = ( 20 / 7 ) = 2.86 Rounds to 3
-
array_unique()