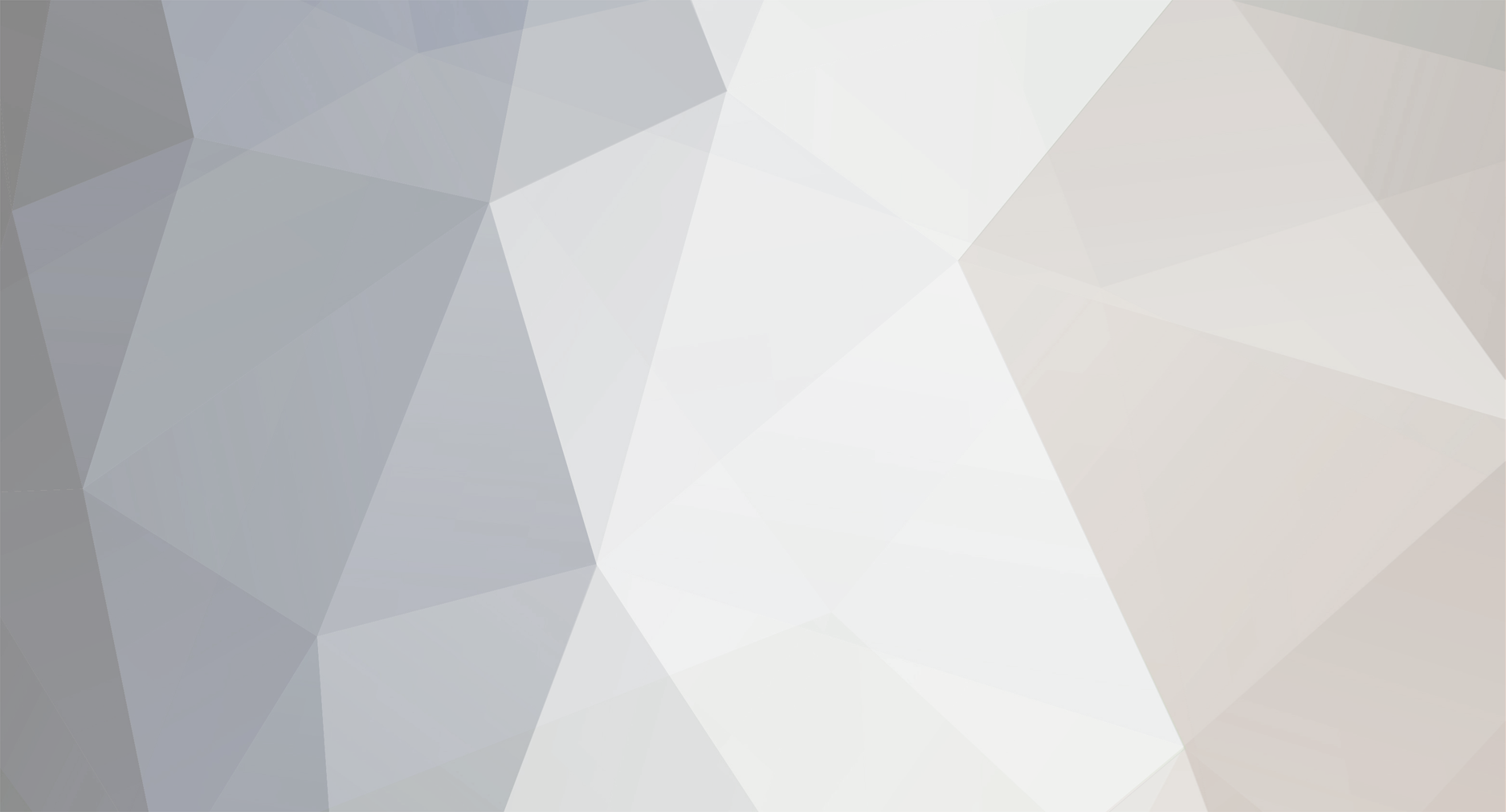
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Your best bet is to: 1. Do what I suggested before. I'm a big stickler for keeping data members private specifically for this reason. Named accessor methods make it easier to see where/how an object's data members are being used, and keeping them private protects them from stray overwrites. 2. Write some error handling code. Ensure that a Character exists. Ensure that the proper data members have values before accessing them. Code defensively. 3. Write debugging output. At each major step of the process, output the appropriate info. That all written, I think I may have just spotted your problem: it looks like your PACKET_WALK case is being invoked before you actually create a character. That explains why those secondary data members are filled, but not the ones you usually set in the constructor. $character becomes a default object at that point.
-
Without seeing more code, I cannot give an answer.
-
I'm wondering if your data members are being overwritten somewhere, as nothing in your syntax jumps out at me. Try this: make your character data members private and give them appropriate accessor methods. For example - class Character { private $socket, $id, $x, $y, $direction; public function __construct($s, $id) { $this->socket = $s; $this->id = $id; } public function getSocket() { if ($this->socket) { return $this->socket; } else { return null; } } public function setSocket($s) //just in case you need to reset/change it { $this->socket = $s; } //etc for the rest of the data members } It's a bit of a hassle, but it ensures that your objects are properly encapsulated. You may have a conditional or something else in your system that's trying to assign to the socket data member rather than check for its value (in other words, a missing or extraneous '=' somewhere).
-
Are there any other places within your Server class where you instantiate and store Characters? Also, can you show us the loop that's giving the error?
-
The following works for me in IE8 and FF 3.5: <html> <head> <title>Blah</title> <script type="text/javascript"> window.onload = function(){ var oTextarea = document.getElementById('myText'); var oButton = document.getElementById('myButton'); oButton.onclick = function(){ alert(oTextarea.value); } } </script> </head> <body> <textarea id="myText"></textarea> <button id="myButton">Click</button> </body> </html>
-
I'm pretty OCD about file structure, so I like having folders for raw HTML includes, images, CSS files, etc. I find that a consistent file structure across projects makes it easier for me to port over various bits and pieces, as I know how my sites are laid out physically. In terms of site structure - how a script is organized programmatically to deliver the desired content to the user - it depends on how complex the site is. Bigger sites benefit from some sort of controller resting on top of everything, delegating request information to the parts of the script that need it. Smaller sites benefit from a more direct approach, without that kind of overhead. There's no one-size-fits-all solution. Instead, it all depends on what the site needs to do.
-
It works for me: <html> <head> <title>Blah</title> <script type="text/javascript"> window.onload = function(){ var oTextarea = document.getElementById('myText'); alert(oTextarea.value); } </script> </head> <body> <textarea id="myText">Hello world</textarea> </body> </html> How are you filling the textarea?
-
Why wouldn't it be? Jagged arrays (a.k.a., an array of arrays) are pretty common in programming.
-
Each item has a name, a price, and a quantity, correct? Store that info in an associative array: $item["name"] = $name; $item["price"] = $price; $item["quantity"] = $quantity; $_SESSION["cart"][] = $item //accessing the data.... foreach($_SESSION["cart"] as $item) { echo "Item: {$item["name"]} <br />"; echo "Individual price: {$item["price"]} <br />"; echo "Quantity purchased: {$item["quantity"]} <br />"; echo "Total for {$item["name"]}: " . $item["price"] * $item["quantity"] . "<br /><br />"; }
-
I think order of operations is still being performed correctly. Look at it in steps: if (5 or 0 || (5/0)) -> if ((5 or 0) || (5/0)) Like you said, the || is checked first. So, (5 or 0) is checked as the first part of that comparison, since logical operators have left associativity (i.e., left operand goes first). Within that comparison, 5 evaluates as true. Since PHP uses lazy evaluation, it doesn't even get to the illegal division.
-
There's also the Microsoft (*gasp* "They're evil!") route. Their free IDE - Web Developer Express 2008 comes with a built-in SQL server. C# is a pretty nice language (at least, from what I've experienced so far), and is entirely OOP as well. It's pretty much Microsoft's answer to Java (even though they're addressing the problem from opposite ends...go figure), and is a very marketable skill to have in my area of the world. And, since it's apparent that I like parentheses, here are a few more: (())(())(())(())(())(())
-
Think it out: If there's less than 50 seconds difference between the current time and the time in the db, deny access - if ((time() - $time < 50)) { //posted too soon } Make sure that $time is a Unix timestamp.
-
everybody with a black suit! if this was the matrix that is. Hello, Mr. Anderson....
-
To the OP, imagine you're creating your Person objects from data stored in the database... how likely is it that all of those people will be named Mr. Smith?
-
[SOLVED] Using JSLint to check Javascript code?
KevinM1 replied to random1's topic in Javascript Help
JSLint is very clear regarding semicolons: Why not write your code as a human would write it (multiple lines), then run it through JSLint? Compression should be the final step, after code validation. -
Remove the '.changer' portion of the code, so it reads as 'document.getElementById("reply").value += newtext;'
-
Well, stuffing a bunch of reusable functions into a class != OOP. You're treating it the same way as a normal library file that you'd wind up including/requiring. Having it in a class doesn't make it magically better. Like CV said, OOP is a design methodology - a way of coding. It focuses on making code modular, reusable, and extensible. I always enjoy the LEGO analogy. Objects are like LEGO bricks. There are some that serve special purposes (like those cool slanted pieces in the Space sets), but most are pretty generic. They all have their own properties - size (a 2x2 brick is different than a 3x2 brick), color, thickness (plates vs. bricks) - and when used together can create any kind of shape. More importantly, a brick used in one shape can most likely be used in another. The same goes for objects in OOP - the objects used in one system can most likely be used in another. OOP describes how to make those bricks, and how to combine them to do something useful. Nothing more.
-
Add a counter to the id name. $count = 0; while (/* your db condition */) { . . . echo "<div id='chosen" . $count . "'>"; ++$count; . . . } Obviously this is just a sketch, but you initialize a counter outside this loop, add the counter to the id name, and finally increment the counter for the next iteration. Starting with the counter at 0 smooths things for the JavaScript, based on the way that script will be constructed.
-
Perhaps even. <?php echo {$_GET['pg']}; ?> Actually, Mark has a point. Short tags may not be turned on. It's always best to use the standard and always supported: <?php ?>
-
A few things: 1. Remember that an ID is supposed to be unique. The while-loop that you use creates several divs with an id attribute of 'chosen'. This is logically wrong. You'll either need to create a unique id for each, or give them all the same class name. 2. The easiest way for this to work would be to give each unordered list (ul tag) its own id. That would greatly simplify the JavaScript, as it would make it easier to find which elements should trigger the display when clicked. Tying it to all hyperlinks is a bad idea. 3. The basics of kratsg's approach will work, but you should change the display to block rather than inline. Divs are block elements. A final solution depends on what you do in steps 1 and 2.
-
Extending a class is used often in the Command pattern. abstract class Command { private $request; public function __construct(Request $request) { $this->request = $request; } public abstract function execute(); } class Login_Command extends Command { public function execute() { //assume we already have a db connection //request validation goes here...if valid $user = $this->request->getValue('user') and $pass = md5($this->request->getValue('pass')) $result = mysql_query("SELECT * FROM users WHERE username = $user AND pass = $pass"); if (mysql_num_rows($result) == 1) { /* success */ } else { /* error */ } } } Rough sketch, but you should get the idea.
-
Any reason why you're using classic ASP instead of ASP.NET?
-
I remember coding BASIC on my old VIC-20.... Man, I'm old.
-
Reading from stdClass Object within an array
KevinM1 replied to saucypony's topic in PHP Coding Help
Arrays are 0-indexed, which means your first object with an id of 1 is array element 0. If you're merely trying to get the unitprice based on the id, try: echo $array[$id - 1]->unitprice; Of course, it's best to use an accessor method to obtain an object's data members' values rather than leaving them publicly accessible. -
[SOLVED] C++ Multidimensional Array Issues
KevinM1 replied to seventheyejosh's topic in Other Programming Languages
Well, first, you're going outside of your array boundaries. something[2][50] is actually something[0-1][0-49]. Just like in PHP, arrays are 0-indexed. The number of elements you specify are indexed as num-1. Also, remember that C-style strings are null-terminated, so you have to account for that as well. I'm wondering where the char[5] part of the error is coming from. Can you show more code?