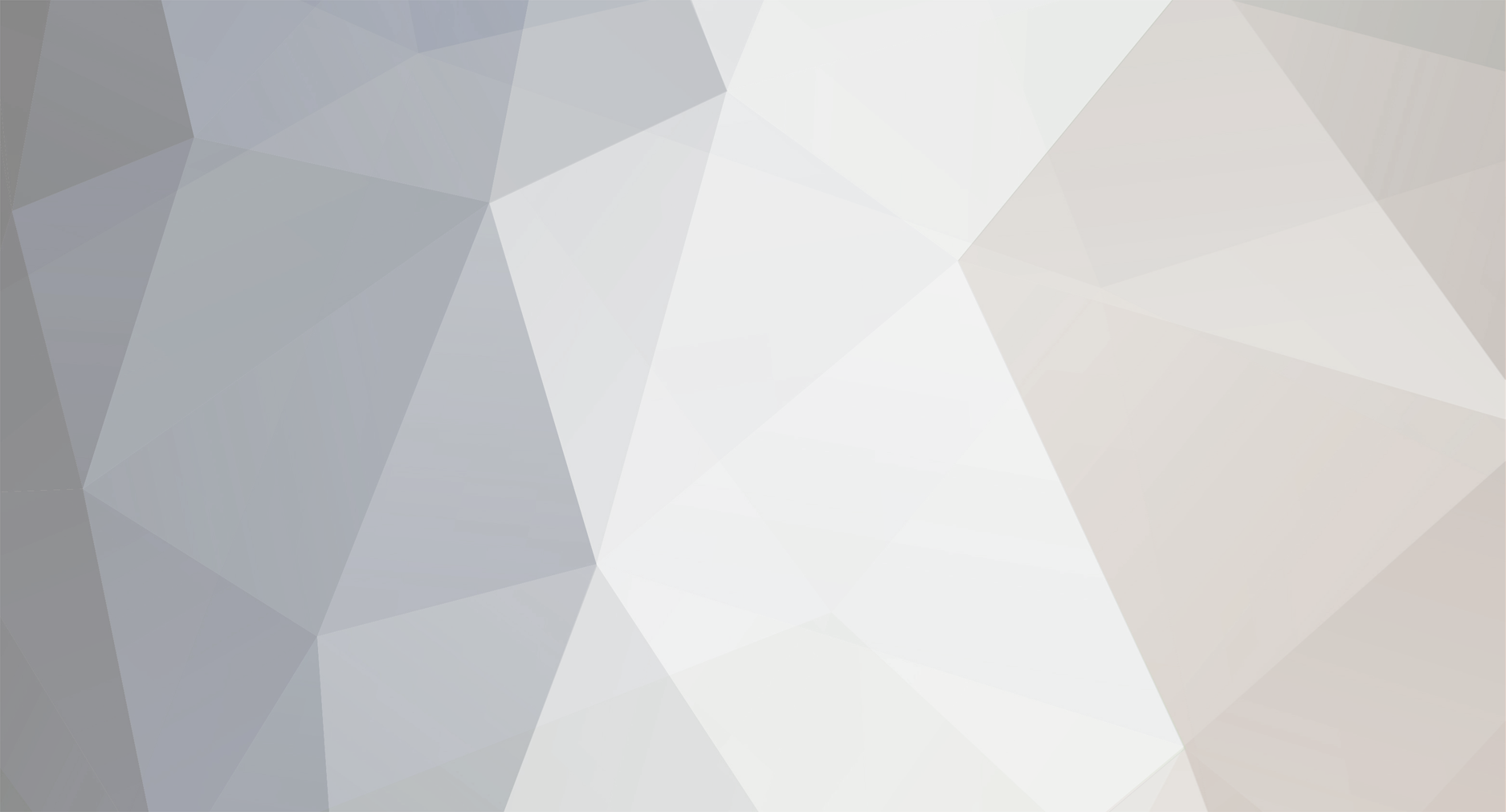
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
+1 for prepared statements, which should really be standard operating procedure for any query run.
- 10 replies
-
- injection
- sql-injection
-
(and 3 more)
Tagged with:
-
Pretty much. They make their money off of an all-out advertising blitz. A lot of newbies aren't even aware of other registrar/hosting options.
-
Deathbeam is actually explaining the 'internal' keyword wrong. C# has what are known as assemblies, which are either executables (actual .exe) or dynamically linked libraries (.dll). These assemblies can be created by/contain multiple files. So, what he wants is a way to say "these files belong to a framework assembly, so most of what's in them should be hidden from the public." 'Internal' says that only code that's in the same assembly can access it.
-
They turn up the graphics on level 6.
-
That is the class name. It's its fully qualified name.
-
PHP Classes - Tricks for loading classes within classes
KevinM1 replied to CrimpJiggler's topic in PHP Coding Help
Objects can contain other objects. This is known as composition, and is used far more frequently than inheritance because, as Jacques says above, it creates a has-a relationship between objects rather than an is-a relationship that's formed with inheritance. It's more flexible. The code above is simply doing something like this: class Battery { // stuff pertaining to a battery } class Device { private $battery; private $name; public function __construct(Battery $battery, $name) // the uppercase Battery is a type hint, which makes the code more explicit { $this->battery = $battery; $this->name = $name; } // setters and getters }The part that's tripping you up isn't actually all that special. It's functionally the same as: $battery = new Battery(); $name = 'something'; $device = new Device($battery, $name);The only thing that's different is that instead of assigning the new Battery object to a variable, the code's author just creates it when they pass it as an argument to Device's constructor, likely because they won't need to access the Battery outside of the context of being a part of the Device. -
Basic question about the capabilities of PHP.
KevinM1 replied to claudiogc's topic in PHP Coding Help
It's important to understand the HTTP request/response lifecycle. User sends a request to a server | V Request is delivered to the proper place (PHP script, something else) based on the structure of the request | V The server processes the request based on the info provided. So, your PHP script will fire, maybe work on form data or some other info, interact with a database if necessary, and send a response | V Response sent back to the user. This will generally be in the form of a completed HTML document in our case, since we're interested in creating/modifying/editing web sites | V JavaScript is run in the browser, which is why it can manipulate rendered HTML directly. It's the last step of the process So, as you can see, PHP and JavaScript can't do the same things because they serve different purposes and are used at different times in the HTTP request/response cycle. Now, you can use JavaScript to send a request to a PHP script that resides on your server, and then dynamically apply the results to the screen without a page refresh. That's what AJAX is, and it's how web apps like just about everything Google does works. But, outside of a sophisticated testing framework that can simulate a web browser, PHP can't directly access a rendered page. By the time a page is rendered, PHP is done working. -
Help for writing code without mysql_result
KevinM1 replied to yathrakaaran's topic in PHP Coding Help
You should use prepared statements, and fetch the results from that. Look at the procedural example in the docs: http://php.net/manual/en/mysqli.prepare.php -
Keeping a value stored in a variable after reloading the class
KevinM1 replied to Noskiw's topic in PHP Coding Help
PHP is essentially a fire-and-forget environment. Once a particular script has executed, it's done. So, there's no shared memory or whatever for you to store values in. Because of that, you're left with the following options: Sessions Cookies Flat file Database -
Huh? If you're building an app that will eventually be placed on the web, you're going to have to use HTML and CSS to display results to the screen. Even with the various template engines available. Doesn't matter if it's backed by PHP, Java, Python, Ruby, C#, etc. To claim that it's somehow an integral flaw to PHP is simply not correct. Similarly, if you're going to work with a database, SQL is going to be written somewhere. You can make your own query language to run on top of it, like what you're trying to do (although, Lisp? Really?), or like Doctrine's DQL language like Jacques mentioned. But at some point, the conversion needs to be made, and the programmer that's using this new language still needs to have some idea of SQL to make it work.
-
GoDaddy sucks. They're the McDonald's of domains/hosting.
-
Yup. Despite PHP being dynamically typed, type still matters. Every class is a type. Inheritance creates a new type, yes, but an instance of that subclass is considered an instance of the parent. Quick example: class Shape { // some base code } class Square extends Shape { // Square-specific code } class Triangle extends Shape { // Triangle-specific code } Both Triangle and Square are Shapes. An object of type Square can be considered a Shape, and be passed into functions and methods as a Shape. Example: class Shape {} class Square extends Shape {} class Color {} function test(Shape $shape) { echo "Success"; } $sq = new Square(); $co = new Color(); test($sq); test($co);The first test will pass, even though we're passing in a Square. The second will fail. So, since inheritance means that an instance of the child type is also considered an instance of the parent type, that means you need to be very careful about what you choose to extend. Is a View an Action? No, of course not. One may have to refer to the other, but they shouldn't be considered the same thing. Which is what's happening when you decide to use inheritance. If you need to use or refer to an object of a different class, just compose them: class Action { private $view; public function __construct(View $view) { $this->view = $view; } }Composition - including other objects in your current object - is generally the way to go in most cases. Inheritance gets a lot of attention because it's an OO-specific functionality, but it has limited usefulness. It's unfortunate that so many resources seem to oversell it.
-
Why would a crate knowing its own physical properties create two responsibilities? I mean, yeah, you can get pedantic and separate the properties in terms of being a measurement of length (dimensions) and mass (weight), but that's way overthinking it. Don't get hung up on following SOLID to the nth degree. SOLID principles naturally follow from these two adages: 1. Code to an abstraction, not an implementation 2. Each class should focus on one thing A crate has physical properties. Weight is one of them. Unless including its weight in a class dramatically changes how that class behaves (i.e., if handling the weight is special/different from everything else), then don't sweat it.
-
Can you show us your entire code (especially if it's in separate files), and the exact error message? Also, are you using an autoloader?
-
I think your issue is with how you approach problem solving in general. If your previous posts are any indication, you're one of those people who sees an entire forest but can't focus on an individual tree. So, it's not the loops that are the root of your issue, but rather that you think you need to solve whatever problem you have with just a loop. That there's some magic combination of statements in a loop that will solve everything, and you're beating yourself up for not seeing it. That's not how programming is actually done. Break your problem into parts. Solve each manageable piece, then combine them. Over time you'll be able to skip steps, just like in math. Patterns will emerge, and your own experience will inform you of the route to take because you've done it before. That's why 'everyone else' seems to create these things seemingly from thin air. You're not there yet, and you shouldn't expect to be. You need to take baby steps and, again, solve your problem by chopping it up and attacking those individual pieces. EDIT: Here's a test - Given the following array: $array = array(1, 2, 3, 4, 5);Write some code that will go through the array and echo whether the value is even or odd. How you approach this will inform me whether or not I'm right about how you approach problems.
-
No, because there are laws involved. It's not the kind of thing you want to get into without having a good financial lawyer and a copy of your country's banking software requirements (there's usually various forms of compliance you'll need your system to adhere to). It's not something you should think you can just code up on a whim. Bank accounts, in particular, are tightly regulated. More so than credit cards. This has Bad Idea written all over it.
-
It's simple. 404 means not found. Can the product be found at that address? No? 404.
-
PHP apps are web apps. They require a server to run, whether it's some host or your own machine. You cannot make standalone desktop software with it. Look into Java, .NET, or C++ if that's what you're aiming for.
-
To put it in real world terms, APIs are generally used to create services for other programs/sites to use. Look at Twitter - it has an API that allows other programs/sites the ability to display and post tweets remotely. It's how all those 3rd party a Twitter apps/browser plugins work. You wouldn't expect those apps/plugins to actually contain all of Twitter's code, would you?
-
We can't give a firm answer because we don't know what you want to do with the data that's being passed in by the user. Any danger is predicated on the data's intended use. Like Jaques colorfully said, form data is simply data. It's not inherently dangerous, even if it contains a string that could potentially be used as a SQL injection or XSS attack. It's how that data is used that determines whether you're flirting with danger. Put another way, there's no one-size-fits-all rule to making something safe. It depends on what you want your code to do.
-
OOP definitely incurs the cost of overhead. Not only do objects themselves have overhead, but your conceptual structure can get more complicated. OOP is a different way of thinking. The currency of OOP is objects. There's no way around that. The whole idea is that, outside of initialization, you have a potential network of objects that are passing messages back and forth to each other. That's why documentation, project structure, and meaningful errors/exceptions are paramount. That said, I understand your pain. I've been using Symfony 2 for all my projects for the last year+, and the online documentation is woefully bare and incomplete beyond the basics. So I've often had to dive deeper than I've ever wanted to figure out why certain errors have popped up. But that's been an issue of poor documentation and bad naming practices (why is the symfony bundle buried so deep?), and not of OOP in and of itself. So, if you're going to go full OOP, be sure to document as you go. Document not only the classes themselves, but the app's workflow so you and other people can follow the chain.
-
Well, first, you shouldn't be using the mysql_* functions any longer. They've been deprecated and are not safe. Use either MySQLi or PDO. Beyond that, it's basically a matter of using the right tool for the job. I would never touch WordPress, since it's absolute crap under the hood, but beyond that, if the job calls for a 3rd party app, use it. If it calls for a framework, use a modern one that you like the best (I've been using Symfony 2 a lot lately). If it's something small, just write what gets the job done. There's no set in stone correct answer, as it depends on what problem you need to solve. Your current structure in funneling things through index.php is known as a Page Controller, and is absolutely fine for small projects. Just be sure to code defensively, and don't blindly pass along the values sent via GET to your database.
-
How do you keep bookkeeping records of transactions?
KevinM1 replied to floridaflatlander's topic in Miscellaneous
Then do that? I have a filing cabinet with hard copies of most things, and I can print out the receipt of just about anything since I purchase 99% of what I use for work online, and have an email record that lasts years. -
You can just pass the value through the method's argument list. There's nothing saying that getters can't receive an argument. Example: public function getGreetingPhrase($hour = null) // we set the default to null { $hour_of_day = ($hour) ? $hour : date('G'); // if something IS sent through as a parameter, use it, otherwise, use the current hour // do stuff with $hour_of_day }The above isn't really OOP specific, but it should illustrate a cleaner way to do what you want. What resources are you using to learn OOP? I have a couple of books I generally recommend: PHP Objects, Patterns, and Practice Design Patterns: Elements of Reusable Object Oriented Software They should get you on the right track. Start with the PHP-specific one first.
-
Yeah, I work with one other developer as well. We create/destroy feature branches as needed, but really only have a master branch. It works fine.