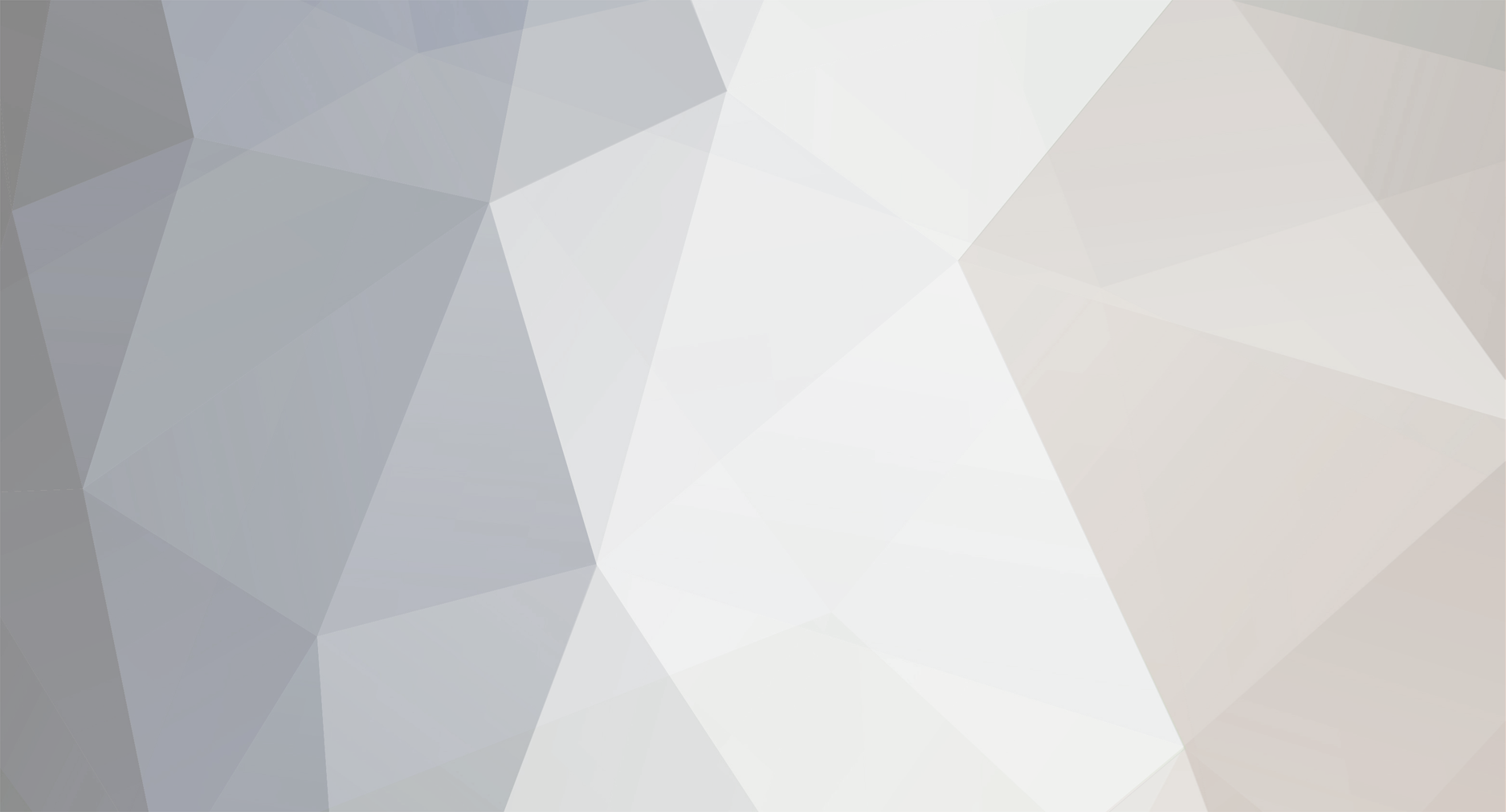
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Which is resume-speak for being skilled in those areas. I mean, how would one describe having mastered PHP? Do they have intimate knowledge of its internals? Have they written extensions for the language itself? Likely not. Similarly with 'complete knowledge' of JavaScript. It's bullshit buzzwords designed to look impressive, but has little actual meaning. And it's usually proven to be a lie during the interview phase anyway. Don't get hung up on it. And college doesn't suck. You just need to find a good one and pick the right major. Of course a major called 'Networking and Internet' isn't going to teach you much in the way of web programming. It's an IT major focusing on networking. I don't know where you live, but in the US there are plenty of schools that teach web development, and teach it well.
-
No one in web development gets hired without: 1. A portfolio of projects that showcases your abilities 2. Being able to pass interview tests #1 can come from a variety of sources. If you're a student, you may have had some projects that you think really represent you. If you're a freelancer, you may have had some particular challenges you overcame. No employer will hire you unless you can show them what you do. #2 is an assessment of the practical knowledge you've gained as you created your portfolio. Internships are becoming more rare because a lot of places don't want to waste resources training someone up to a passable level of skill only to potentially see them jump ship after they've learned what they came for. So, your best bet is to do some freelancing on the side until you feel confident enough to apply for a job with an existing development company. Small businesses exist everywhere. Many of them want a web presence. That's a service you can provide now. It will give you real life experience, not just in solving programming problems, but dealing with clients, communicating and collaborating with them, etc. Which will, in turn, give you something to add to your portfolio. But, yeah, I'd expect an intro-level developer to be comfortable writing procedural PHP. To be able to handle arrays, string manipulation, dates/times, basic database CRUD routines, etc. To understand the HTTP request/response cycle, and properly handle forms. To structure their code correctly so it's not a mishmash of HTML, PHP, and JavaScript. To understand how to debug and troubleshoot their own code.
-
Wow, there's a lot here that pretty much misses the point entirely. I'm not trying to be rude, but there's a definite lack of understanding as to why certain class structures are used. First and foremost, something like this would likely never be put into a class, at least, not by itself. It's too specific, too small to justify the overhead of writing a class and instantiating an object. OOP is primarily about dealing in abstractions. A more robust greeting system could warrant a class, but not as it stands now. Putting that aside, the class is overwrought. Why would you want to store the current hour when you can simply get it from PHP whenever you wanted? Why do you have a public getter, then a private getter that does the work for you? There's no need to go through so many hoops. Your constructor didn't work because you never created the property you're trying to assign the value to. The $this keyword means 'current' object, but you never declare a $hour_of_day property for the object to use. You need to declare something before you can attempt to use it, like: class Example { private $x; // <-- notice that we created a property here public function __construct($blah) { $this->x = $blah; // <-- the value of $blah is now contained in this object's $x property } } So, all that said, your class can be reduced to: class Greeting { public function getGreeting() { $hour = date('G'); if ($hour < 12 ) { // if it's before 12pm $greeting = "good morning"; } elseif ($hour >= 12 && $hour < 18 ) { // if it's after 12pm but before 6pm $greeting = "good afternoon"; } else { // what is left over - after 6pm until midnight $greeting = "good evening"; } return $greeting; } }Which isn't really useful. Classes are not intended to just be groups of functions.
-
Yup. It's a Fire tablet slimmed down to a phone form factor. The four front facing cameras strikes me as very gimmicky. The tech that scans everything and can match it to an Amazon item (or whatever... I didn't go through many of the details carefully) is kinda neat, but eh. It strikes me as an Android for people who don't like Android.
-
Another Android I don't care about
-
Making a program to automatically install PHP frameworks
KevinM1 replied to CrimpJiggler's topic in Miscellaneous
Composer is civilization. -
Yup, basically loops themselves have two parts: 1. The block of code that will be repeated 2. Some sort of condition to tell the loop to keep looping That's it. The big three are while, for, and do-while. While loops: while (/* some condition is true */) { // do stuff } The while checks the condition first. If it's true, it executes the block of code. If it's not true, it does not execute the block of code. That means you're not guaranteed that anything in the loop will actually execute. Do-while loops: do { // stuff } while (/* some condition is true */) This one isn't used very often. It's 99% the same as a regular while-loop except the part in the do block will be executed before the condition is checked. So, you're guaranteed at least one pass through the code. For loops: for (/* initialization */; /* some condition is true */; /* end-of-iteration statement */ ) { // do stuff } For-loops are the most complex, but they're still not hard. It can be translated into: /* initialization */ while (/* some condition is true */) { // do stuff /* end-of-iteration statement */ } So, with a concrete example: for ($i = 0; $i < 10; ++$i) { echo $i . '<br />'; } // is the same as: $i = 0; while ($i < 10) { echo $i . '<br />'; ++$i; } In PHP (and other languages), there's also foreach, which is special. It uses an internal structure called an iterator to go through a collection (usually an array) one element at a time. So, it always starts at the first element and ends at the last element, unless you do something like use break to end the loop prematurely. You've seen them before, I'm sure: $arr = array(1, '6' => 'monkey butt', 'Mississippi', 'orange' => 'is the new black'); foreach ($arr as $item) { echo $item . '<br />'; } So, yeah, that's it. Loops are not hard. You just need to practice them. Make a few test scripts and see how they work. Chances are, like I said before, you're freaking out because you're not breaking your problems down into manageable parts.
-
Regarding your first paragraph, are you saying a foreach is wrong 99% of the time, or a for-loop? It reads as a for-loop can break if you're not dealing with numeric keys, but I want to double check.
-
Yeah, it's not a problem with loops per se, but rather an issue with your approach to problem solving in general. You need to break the problem down into individual chunks, like: Can you obtain a single record that needs to be updated? Can you update that record? Can you expand that process to two records? Howabout three or more? Most problems are actually a collection of many smaller problems. If you can solve those, then you should be able to put it all together. That said, I recommend you do further research on arrays and loops. Regular for-loops aren't used much in PHP. Instead, foreach takes its place in most cases, and while-loops tend to handle everything else. It's not that you can't use a normal for-loop, it's just not nearly as common. The reason being that this: $arr = array(1, 2, 3, 4); for ($i = 0; $i < count($arr); ++$i) { echo $arr[$i] + 1 . '<br />'; } is the same as: $arr = array(1, 2, 3, 4); foreach ($arr as $num) { echo $num + 1 . '<br />; } but, as you can see, the second one is easier to read and write.
-
http://lmgtfy.com/?q=bytes+to+megabytes
-
What is the difference between a front end web developer and a
KevinM1 replied to lexijensjacejack's topic in Miscellaneous
A front end developer is someone who primarily writes HTML, CSS, and JavaScript. They may also have a hand in the graphic design for a site. An application developer is a person that, well, helps create the actual guts of the application. They create the part of the site that actually does the bulk of the work. So, that would mean a lot of work storing, editing, manipulating data, writing the processes that do all those things, etc. It's more generally server side work, with databases and languages like PHP, Python, Ruby, Java, C#, etc. Not always (Google uses a ton of JavaScript in their apps), but generally speaking. One isn't 'better' than the other. Both are necessary when creating websites. I'd expect application developers to be paid a bit more, since they handle more sensitive data and may have more managerial responsibility, but that's not a rule written in stone, and it's not like either is a bad job. And in America, at least, good luck getting your foot in the door of any company without some sort of degree. That degree doesn't necessarily need to be tech related, but a college degree shows that you can handle pressure and deadlines and assignments. A college degree today is what a high school diploma was to our parents - the minimum requirement in having most white collar companies even looking at you. A certificate can have value, depending on where it's from. No employer would give a w3schools certificate any weight, but something from Zend likely would have value. But, a certificate will never be as valuable as a solid portfolio. That's how an employer gauges whether or not your claims of experience match reality. You can say, "Oh, I've been building sites for five years," but if they suck that means it's not five years of professional grade experience, which is what they want. -
You don't need a contract in this case, but you need to make sure that the requirements and scope of the project are put in place before you start working on it to help guard against feature creep. Clients are notoriously capricious. They love adding "just one more thing," which becomes many small things, most of which are either useless or gaudy. And since clients think they have great ideas and design sensibilities, they tend to care more about the bells and whistles they suggest than the important things, like if the site fulfills its stated function, because that's boring. It's up to you to ensure that doesn't happen. The customer is not always right. So, like the others have said you need to do your homework and ask smart questions. Look at what other schools in your area, or schools that are of a similar size, have for their websites. Start thinking about the tech that they use - do they have a blog? Some kind of content management system (CMS)? - and research available solutions. Dale mentioned some in his post. Make sure you ask the client about how they envision using the site. It may sound obvious, but clients can get very picky about how certain things are done. They tend to like a workflow that mirrors how they work in real life, so be sure to get whatever mechanical parts (blog, lesson plan, grades, attendance, etc.) down and all the steps regarding their access and display. "How do you want it to work?" is a critical question. And, of course, confer on the site design. It would be beneficial for you to bring examples of those schools you researched (printouts of screenshots can suffice) to get the ball rolling. Be sure to ask them about not just what they like in terms of web design, but what they dislike. And be prepared to talk about fonts. It sounds like a lot, and it is. Your best bet is to treat it like your first professional gig. With this, you're not a student and this isn't a school project or favor. You're an intern/employee and you're working for a client. So behave as a professional would, and you'll be fine. Welcome to the real world
-
This. Polymorphism is about being able to have different classes that perform the same task at runtime. Take the following example: abstract class Person { private $name; private $salary; public function __construct($name, $salary) { $this->name = $name; $this->salary = $salary; } abstract public function calculateRaise(); } class Employee extends Person { public function calculateRaise() { $this->salary += 1000; } } class Boss extends Person { public function calculateRaise() { $this->salary += 100000; } } class PersonFactory { private $db; public function __construct($db) { $this->db = $db; } public function getPerson($id) { // query the db to get the person info // determine what kind of person to return based on a flag or something $person = new $row['role'](); // fill the person with the rest of the data return $person; } } $factory = new PersonFactory($db); // assuming we instantiated our db somewhere else $people = array(22, 54656, 99, 675, 1009); // person ids foreach ($people as $personID) { $person = $factory->getPerson($personID); $person->calculateRaise(); // save the person to the db }Yeah, the example is canned, and probably has some syntax issues, but you get the idea. The whole point of polymorphism is to hide implementation behind a common API. The loop that gives raises to those people doesn't care if they're an Employee or a Boss. Why should it? So long as a Person can get a raise, does it matter if they're an Employee, Boss, or something else, like a Contractor? No. At some point, you'll need to make a determination about what kind of object to construct. That's generally where factories come in. Their entire purpose is to return a concrete object for the program to use. But, with polymorphism, you'll only be seeing and dealing with the functionality laid out by the abstract base class or interface.
-
Yeah, same here. Mich, can you explain your roles a bit better? Can more than one be active at the same time (as in, can a person be both a Manager and Player, or Player and Referee, or any combination of them)? Is there any shared functionality between roles (as in, do they share properties, methods, etc.)?
-
We have a freelance section if you're looking to hire. This is not the place to be recruiting hired help.
-
Well, he did come here asking how to solve that particular problem of his Team object being tightly coupled to his db wrapper. And Ignace answered him. The problem is that sprinkled among Ignace's answer were suggestions that would do more harm than good. Which is what I was trying to address. Whether or not an ORM adds too much complexity is up for mich2004 to decide. But our position, as a site, is to inform and educate best practices. Ignace's answer was perfect because it provided two avenues: 1. Slap an enterprise-level solution into your code and call it a day. or 2. Learn how to do it yourself on a smaller scale so you understand the mechanics behind it. I don't see anything wrong with that. And OOP is confusing at first. It took me a long time to understand it. But I don't think withholding information, when it's something specifically asked for, is the way to go. "It's good enough" isn't what we do here when someone asks how to address a problem.
-
No, it's not a great place for a singleton. Remember, objects are passed by reference by default. If you create a database object (say, a PDO instance), and pass it around to other objects or methods, only references to that single instance are passed around. You're not passing multiple copies/connections around. It's just references to a single resource. That's why dependency injection is the way to go. You get your single instance, but without blowing apart scope/encapsulation to get it. Regarding the OP's code, yes, he used dependency injection, which is great. My comments were aimed towards adam_bray, who not only placed the database in a singleton, but also the Team, which is definitely not the way to go. His suggestion strikes me as coming from someone who only has a hammer, and thus sees everything as a nail. --- @mich2004 - Ignace had your solution. You need to use an ORM (Object Relationship/Relational (I've seen both) Mapper). It's what maps your database tables to an object representation (usually referred to as an Entity). You can either use an existing solution (his link to Doctrine 2, which is probably the most popular and widely used), or roll your own (the Sitepoint link). The point is that your Team object is supposed to be ignorant of the db. The mapper should do the heavy lifting of populating/editing/saving Team info. The Team object should be an in-code representation of your Team(s) and little else.
-
The main issue is that singletons are Bad. They're just another global which leads to tight coupling, which leads to rigid code, which leads to spaghetti-flavored hacks to work around it. Singletons are wholly unnecessary in PHP where a script's lifespan generally lasts just for one request and objects are passed by reference by default. Generally, if you think you need a singleton, what you really want is dependency injection.
-
Every time I look at WordPress code, I have a mini-stroke. So much crap under the hood, with more crap bolted on top in the form of plugins.
-
Potential Script Conflict - How to get both functions working?
KevinM1 replied to excelmaster's topic in Javascript Help
You need to post only the relevant parts of your code. You refer to two PHP blocks, but there are four in the code provided. Also, please double-check your formatting. I know that the editor here can mess with code that's pasted in, but try to remove extraneous line breaks. Beyond that, as a general tip, it's almost never a good idea to mix and match PHP and HTML like you have. It gets confusing and hard to debug. Instead, you should do all of your PHP work first, including database manipulation and anything that sets values, then use incredibly simple PHP to display those values in your HTML template. -
Before you do anything with a database, you need to ensure that your table structure is sound. Otherwise, basic operations become difficult to do properly, to say nothing of more complex actions. So, read through this tutorial first. It will get you on the right track, which will then make your queries easier to write. http://mikehillyer.com/articles/an-introduction-to-database-normalization/
-
Josh was referring to the '$' here: html_generator::$input_class
-
Was recently hacked and could use advice on some code I found
KevinM1 replied to coresash's topic in PHP Coding Help
If you think someone injected code into your .htaccess file, then it should be considered compromised. Which means you don't just keep it, but you delete it and replace it with a clean copy (you do have a locally saved version of your site, right?). Furthermore, Jacques is correct. FTP is NOT a secure way to transfer files. And if the hacker had access to your host, then you should assume that they have access to your FTP credentials. Change those immediately and use a more secure file transfer method (one of the options Jacques suggested above will suffice). Finally, WordPress is notorious for being insecure. You need to keep on top of all their updates, and be weary of plugins. Ideally, you shouldn't use WP at all as it's a train wreck under the hood. If you're serious about security you won't just say "I don't see anything else weird, so I must be good now." You'll work to plug the holes that created the issue to begin with. -
+1 with these sentiments.
-
You shouldn't be using globals at all, in any context. You should, instead, use a function's/method's argument list (the part between the parentheses). That's why it's there. The whole point of functions, and especially objects, is to create reusable pieces of code that work when certain conditions are met. Object methods and functions are supposed to be considered black boxes, where data is passed in, something happens, and a result is returned. The 'something happens' phase is not supposed to be aware of the context outside itself. A global creates a tie to that outer context. How is that different than passing in values through the argument list? Every function and method has a signature comprising that function's/method's name, the values it expects to be passed in, and the expected result. Everything required for a function/method to work properly is explicitly mentioned upfront, and it creates something of a contract. "I will only work if the following values are given to me." Globals create an implicit requirement that's essentially hidden because it's not part of the function/method signature. It says something vastly different - "I will only work if the calling environment contains the following values." That implicit connection to the outer environment creates what's known as tight coupling within code. A change to one thing necessitates changes to many other things since they're all so tightly connected. Modularity and reusability (the entire purpose of functions and objects) goes out the window, and you're left with a hard to edit/debug/modify mess. Especially since global variables/values are notoriously easy to overwrite/delete. If you're learning from a resource that uses 'global', ditch it. It's crap, and everything within should be considered suspect. If you're learning in a class, point your instructor to this post. And if your potential instructor balks, they should realize that everyone you/they see with a green, blue, or red badge under their picture is a professional that actually writes code for a living. So, to conclude, never, ever, ever use 'global' or globals. You'll write much better code, and anyone you might work with will be grateful.