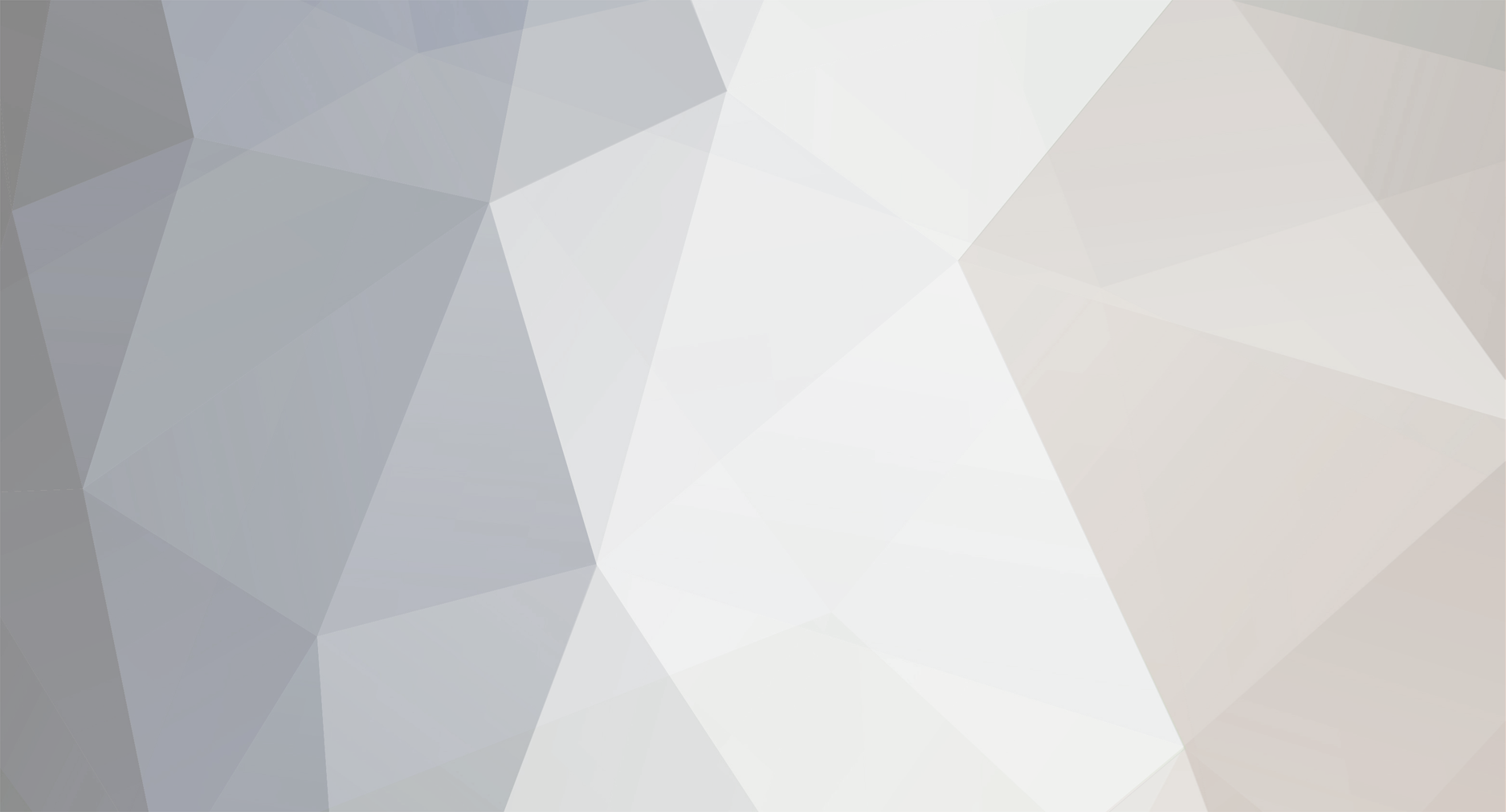
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
A class doesn't 'run'. A class is a blueprint for a custom type. An object is an instance of that class*. To create a new object, a special method known as a constructor is executed. A constructor is ALWAYS executed, regardless of whether or not you define one in your class. If you don't define one, PHP's default constructor is executed. All this does is inform the interpreter that a new object of that type now exists. *A type is generally described as data which adheres to certain criteria and the actions that can work on that data. An example would be integers. They represent data of a certain criteria (whole numbers) and allow certain actions to work on that data (mathematical operations). An instance of an integer would be 4.
-
A constructor is a function/method with an implicit return value - an object of that class. In order to capture and handle this new object, it needs to be stored in a variable, else it'll be inaccessible. It's no different than any other function that returns a value, with the exception that an explicit return statement isn't used. For that reason, version 1 is the way to go and version 2, while technically syntactically correct as well, is useless.
-
Do you want the user to remain on the same page after the email is sent?
-
Global scope is considered dangerous due to how easy it is to corrupt the values of a 'global' variable. There's no built-in check to see if a global has been changed, deleted, or even exists at all. Like I said earlier, 'global' tightly couples your functions to the global environment in which they're invoked. Any changes made to the global variable can spider throughout your code, often in ways you don't expect. Since PHP doesn't have a true global scope, functions have two ways to obtain variables from outside of them - by using the 'global' keyword, which simulates normal global behavior, or by passing them in through the argument list, which, by default, sends a copy of the variable to the function to play with. This is the preferred way to do it. Keep in mind, globals are considered dangerous regardless of language. It's one of the reasons why namespaces exist, and have been introduced in the most recent version of PHP. They're limiting, they lead to badly written code, and they cause problems by their very existence. This isn't entirely true. PHP has several pre-defined superglobal arrays, like $_GET, $_POST, and $_SESSION. You can't make your own superglobal, so if you try something like: $_PUPPIES, you'll simply be making a normal variable. Superglobals aren't ideal, but are a reasonable compromise since they're often used in a read-only manner, or used to persist data between HTTP requests. HTTP's request cycle makes them necessary. It's actually used quite often in frameworks when you need to pass an unknown number of values (think config settings) to a function. So, if I place any variables need by a function within those functions, my code should be okay? The reason I ask rather than debug the code on my host server, is because I want to make sure it works before I launch it (can't use wamp or xamp cuz my computer's really slow). So, to try to explain it another way, your current concern is "Where does the data that makes the function work come from?" In your case, from two places: 1. Outside the function. The errors you generate in process_form ($nm_errors) need to be sent to display_form. Since display_form needs the errors, and they're generated by an outside source, the only way display_form can get them is if they're passed in through display_form's argument list. 2. Inside the function itself. The error messages ($nm_error_messages) are created inside of display_form. Because of this, you don't need to add it to the argument list. It's already taken care of. Remember my analogy - the argument list acts like a bouncer. Only the people on the list go into the bar. If a person is already in the bar, they're crossed off the list. $nm_errors is a person waiting outside the bar. $nm_error_messages is already in the bar, so they're not on the list. And, for a general rule of thumb: where you'd use 'global', use the argument list instead. I hope this makes sense. I know I can be long-winded, but I really want you (and others) to understand what's actually going on. I've seen far too many cases where someone was told "Do X instead of Y," only for them to be frustrated when X doesn't work every time and they don't understand why.
-
Check out http://www.gliffy.com It's a free UML/database/general diagram web app.
-
What you should do is remove all internal and inline CSS in your files and work solely from external sheets. Putting CSS in one place - especially while developing and testing - is far more beneficial than having it strewn about in several areas. That way, if something goes wrong, you have at most two places (the HTML for the troublesome element(s) and the CSS for the styling) to look. Even better, you'll have a far more concise way of asking questions about any problems you can't fix. A good part of app development lies in organization. There's even a term for it - Separation of Concerns. This means that the different tiers of your app should remain as self-contained and separate from the others as possible, so a change in one level of your app doesn't necessitate a change in the others. From a practical point of view relevant to your current project, this means separating page structure (the 'physical' parts of a page, its HTML elements) from page styling (the way it looks and where things go, its CSS) from page behavior (how it acts when a user acts upon it, its JavaScript). This separation is achieved by using external stylesheets, external JavaScript libraries, and unobtrusive JavaScript techniques. If you do your best to adhere to this principle, your life will be made much simpler as each component will be self-contained rather than intertwined with the others. A failure/error in one will be far easier to spot and remedy. Even for a project as small as yours, better code organization can go a long way.
-
Take a look at the text highlighting in the code you just posted. You're missing a semicolon at the end of your $message = ... line. Also, your switch shouldn't be working, as location isn't a variable, it's a string. 'location' will never be 'location1'.
-
Please re-read what I wrote and be sure to also read the examples I linked to. I never said that arrays shouldn't be used as parameters. In fact, I explicitly wrote code showing that they could, which, in turn, is the way I'd go about solving your problem. What I actually said is that if you're creating an array for use in a function WITHIN that same function that's going to be using it (like what you do in display_fotm where you create your error message array), then you don't need to list it in the function's argument list. Why? Because it's already there. You just created it. You should do some research on scope (a Google search along the lines of "PHP function scope" or "PHP block scope" should do the trick) and really brush up on functions. Not just their syntax, but what they are, why we use them, and why they should be made as abstract as possible. I'm getting the feeling that you're lacking some fundamental knowledge, and you're going to keep running into problems if you continue this way. It's difficult to efficiently teach this on a forum.
-
The CSS I found is in the internal stylesheet for your page (you'll have to scroll a bit to see it). Also, border=10 isn't a style, it's an HTML attribute. An inline style would look like: <table style="border: 10px solid #000;"></table>
-
Your CSS is: table,th,td { border: 1px solid black; /* other rules */ } It's obviously overriding what you want. If you're going to play with border thickness, do it in CSS, not HTML.
-
By reference or not by reference... That's the question
KevinM1 replied to Firemankurt's topic in Application Design
...can't believe I forgot that. -
Close. If you're creating an array within a function, it doesn't (and shouldn't) be listed in its argument list. A function's argument list is like a bouncer at a bar - it expects certain guests. If a guest is already in the bar, it doesn't need to be on the bouncer's list. See more at (examples 3 and 4): http://php.net/manual/en/functions.arguments.php Also, stop using global!. Pass in ALL of the necessary data necessary to execute your functions. This includes your db connection. I don't know where you learned to use global - a book, an instructor, whatever - but it's a real bad habit to get into. You're only hurting yourself when you use it. Here's why: Functions have a signature, which is both their identification and a summary of what they are. It consists of three parts - the function's return value type, the function's name, and the type and number of the arguments the function expects to receive in order for it all to work. The signature literally says "Function function name will return a value of type type when n arguments of the following types (types) are passed into it." PHP glosses over this a bit because it's dynamically typed, so many coders - especially newbies - don't really have a grasp on what type is. That doesn't mean it doesn't exist, or isn't important. Other, statically typed languages make it much more clear. It's not uncommon to see something like: bool IsEqual(string str1, string str2) { // ... } Which says "The IsEqual function returns a boolean and requires two strings to be passed in." When you use 'global' you do a few things: you muddy the function's signature, you introduce a hidden requirement to the function, and the function makes an assumption about the environment in which it's executed. In short, you couple (tie together) the function to the environment in which it's called, which negates the entire point of functions. Functions are supposed to be modular. Their requirements are explicit, and known ahead of time. Even if you never work with someone else's code (which, if you're going to do this for more than a hobby, you will at some point), your own coding life is made worse by using 'global'. When trying to debug your code, you'll be required to look into the guts of your function(s), then at the context in which it was called in order to see if the environment was set just right. You'll have to scan your code line-by-line to see if the environment variable exists at all, or has the right type (there's that word again) of value. And what about nested functions? This thread highlights some of the issues there. It's normally around this point that two counter arguments pop up: 1. Popular PHP software X uses 'global' in its code! 2. If 'global' is so bad, why is it in the language? 1. An app's popularity speaks nothing about its craftsmanship. Wordpress is probably the most popular blogging software on the planet, but it's a steaming pile under the hood. 2. Many questionable constructs exist in many languages. They're often remnants of an earlier time, when modern best practices weren't known. Look at 'goto': it's widely considered a four letter word. It leads to spaghetti code (not unlike 'global'), and with modern control structures and design techniques, is hardly ever necessary. At my university, a student would automatically fail if they used it. Just because a language provides you with something, that doesn't mean it's wise or even necessary to use it. There are better ways. Sorry about the rant, but I see this sort of thing all the time on here, and I have no idea where it comes from.
-
You're confusing what the database's COUNT function is doing with what PHP's mysql_num_rows is doing. mysql_num_rows gives the count of how many rows your query will return. Your query will only return one row, which has one value - the number of rows in the 'totals' table that fit your criteria. So, long story short, the result that's being echoed is correct.
-
You can't use a MySQL function on a fetched result. Also, $result itself isn't a row of data you can directly manipulate. It's a resource which must be used by one of the fetch functions (like mysql_fetch_assoc) in order to actually retrieve data. If you need to count results on the db, do it in your query.
-
The '&' denotes an argument being passed in by reference. The problem is in your while-loop. You want $info['email'], not $data['email'], and the same goes for all the rest. Also, you never store the return value generated by decode_variable. You need: $var = decode_variable($sitename); (obviously rename $var to something more meaningful)
-
The best way to do it, IMO, is to use a handful of views/templates for each different kind of page. For example, I'm currently working on a couple of small media review sites (one for video games, one for TV shows and movies). It makes sense to create a review template, and then simply stick the info taken from the db into the appropriate places. This allows me to only have to worry about a handful of actual html files (in this case, ASP.NET MVC 2 view engine files), even though I can have an infinite amount of 'pages' online. I wouldn't go as far as crmamx has and swap out the entire body region of one template page with a mix of new html and PHP, mostly because people generally lose all sense of visual structure when they do something like that, and it also runs the risk of mixing the concerns of information processing with information display. To crmamx: you should probably take a closer look at your site design. Well-designed PHP apps have all information/form processing done at the top, with the code to display the results of that processing below. So, if you follow this general outline, you'd be able to dynamically change your meta tag info based on your incoming $_GET data, as you'd handle/process it all first, and then you could simply modify the tags in any way you wanted.
-
By reference or not by reference... That's the question
KevinM1 replied to Firemankurt's topic in Application Design
Not unless Core changes state and you need all of the objects that contain a reference to Core to have those changes reflected within. -
I tend to start with the domain model - what entities do I need? How will they interact with each other? How will the 'outside' interact with them for CRUD purposes? I then build a mockup of the various pages I need and play with them to see if the process I thought was good in my head makes sense in practice. I modify either the mockups or domain model as necessary. Once I get the domain locked down, I translate it to the db for persistence. Entities have to be stored, after all. Then, finally, I finish the pages themselves and add any JavaScript bells and whistles I need.
-
Using $_SESSION is still an ugly way to do it. It's actually simple to do it the right way: function display_form($errors = null) // setup the function to anticipate an incoming array of errors, but have it's default value be null for those times we're SURE there can't be any errors (first time looking at the form) { //... if ($errors != null) { // display errors } } function process_form() { //... // if there are errors from form processing, store them in an associative array, like $errors['name'] = "Must enter in a correct name"; display_form($errors); } There's no reason for this error data to be global, and contorting your code to make it deal with globals is the wrong way to go.
-
Sounds like your overall design may be flawed. Can you give a relevant example of what you're trying to do, and why you need these variables?
-
You'd have to use Ajax to send the name to your PHP script, then handle the return value.
-
Stop posting non-PHP questions in the PHP Coding Help section. We have many sub-forums, including both a JavaScript forum and one dedicated entirely to Ajax. This topic has been moved to Ajax Help. http://www.phpfreaks.com/forums/index.php?topic=327454.0
-
I'm still not understanding, though. I know that if you have a variable outside a function, you have to declare it "global" within a function to use it in that particular function, but how would you use a variable in the main script that's inside a function, and how would you use a variable in 1 function that's value is declared in another? No, you don't need to declare it global at all. Functions have argument/parameter lists, which allow you to pass in variables. function myFunc($arg1, $arg2) { echo "Hi, these values were passed in through my argument list: $arg1 - $arg2"; } $val1 = "Forrest"; $val2 = "Gump"; myFunc($val1, $val2); Globals are the exact opposite of what you should be using, for many reasons. They make code hard to read, difficult to maintain, and introduce hidden requirements for the function itself.
-
This topic has been moved to Ajax Help. http://www.phpfreaks.com/forums/index.php?topic=327462.0
-
You may want to add a border to the left side of your content area to reinforce the appearance of structure. Nothing too big, maybe just a 1px solid white or red border. Play with it, and see if it adds or detracts to the overall look.