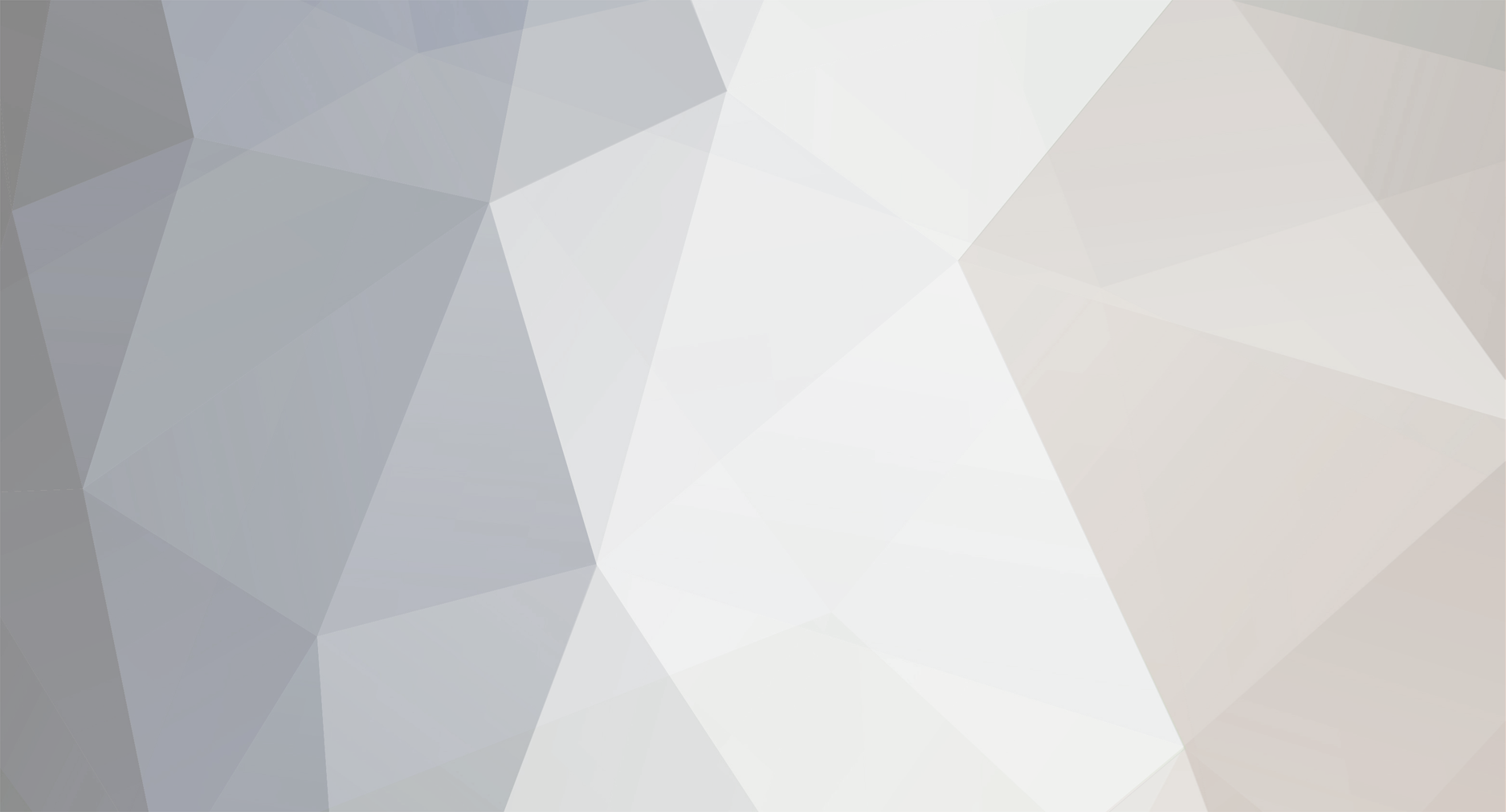
Fadion
-
Posts
1,710 -
Joined
-
Last visited
Posts posted by Fadion
-
-
The <select> dropdown looks correct. You have set the ID as the value and you're to go with form validation and processing. The code below will be run once the form has been submitted, will get the person's information from the database (based on the selected ID) and print it out. Easy enough.
<?php if (isset($_POST['Persons'])) { //get the selected user ID $id = (int) $_POST['Persons']; $results = mysql_query("SELECT name, surname, age, email, phone FROM persons WHERE id=$id"); if (mysql_num_rows($results)) { $values = mysql_fetch_assoc($results); $name = $values['name']; $surname = $values['surname']; //and so on for every field echo "<b>Name: </b> $name<br />"; echo "<b>Surname: </b> $surname<br />"; //and so on for every field } else { echo 'No user found.'; } } ?>
-
From the PHP Manual (but slightly modified):
<?php // Order of replacement $str = "Line 1\nLine 2\rLine 3\r\nLine 4\n"; $order = array("\r\n", "\n", "\r"); $replace = ''; // Processes \r\n's first so they aren't converted twice. $newstr = str_replace($order, $replace, $str); ?>
That is removing them completely from the string. Instead, if you need to display them as new lines, you can use the nl2br() function, that will convert linebreaks to an HTML break (<br />).
<?php $text = "test\r\ntest\r\n\r\nhello\r\nhello"; echo nl2br($text); ?>
Your question wasn't quite clear as to remove linebreaks or show them correctly, so I gave both versions.
-
If you have no idea how to optimize your services, leave it to someone who knows. Server administration isn't something you can learn in a few days in forums.
And btw, if your social network isn't something that blows away everything in existence, I doubt you'll come to the point where traffic is an issue...
-
As there aren't many options involved, it looks more than feasible to me!
However, if you want it to be at least decent (speaking of 3 or less characters search), you'll have to build an index table. Basically, what I'm talking about is a table that holds all your pages content, but stripped from punctuation and stopwords (and, or, a, an, stuff like that). FULLTEXT itself uses a list of stopwords, which can be a good starting point. Wrapping it up: write some code that takes the content of your pages, strips it from punctuation and stopwords, adds it to the index table together with that page ID and finally modify that code to be run by a cron job so it updates the indexes periodically (for changes) and adds new pages. The "WHERE LIKE" search will be run on that table once you have it set up and if you like, you can run the FULLTEXT search on it too. It will surely benefit from it!
It isn't that complicated, really. Sure, sticking just to FULLTEXT would be easier, but when options are scarce, why not use what you have to the full potential?
-
That could be a problem actually. The limit is controlled by a MySQL system variable and if you aren't running it on a system where you have full access, chances are low. In a shared host or other limited environments, you can't edit such variables and I doubt the company will change it just for you.
Anyway, here is an article that describes FULLTEXT limits. Basically, you have to add "ft_min_word=3" (or 2) in my.cnf, restart the server and rebuild the FULLTEXT indexes. Hope it's something you have access to.
-
First let's try refactoring your code and make it more readable.
<?php if (isset($_POST['numUploadsHidden'])) { //chop() is just an alias of rtrim(). Better use the original $_SESSION['numUploads'] = rtrim($_POST['numUploadsHidden'], '/'); } if (isset($_SESSION['numUploads'])) { //you're missing the step where file submissions is checked? $num_uploads = $_SESSION['numUploads']; $xml_list = array(); for ($i = 0; $i < $num_uploads; $i++) { $xsd_file = $_FILES['uploadedXSDFile']['name'][$i]; $xml_file = $_FILES['uploadedXMLFile']['name'][$i]; $xsd_ext = pathinfo($xsd_file, PATHINFO_EXTENSION); $xml_ext = pathinfo($xml_file, PATHINFO_EXTENSION); //skip the loop iteration if the pair is not complete. //use "break;" if you want to show a message and stop the loop completely. //you can show a message before "continue" too. if (!$xsd_file or !$xml_file) { continue; } //same idea as above. //it should be "xsd" or "xml", not ".xsd" or ".xml". Pathinfo doesn't return a dot. if ($xsd_ext != 'xsd' or $xml_ext != 'xml') { continue; } //in_array() checks if a value exists in an array. In your case, it will check //if the XML file exists in the XML List you got from the DB. If true, skip the loop. if (in_array($xml_file, $xml_list)) { continue; } //make the upload and whatever else here } } ?>
It does all the checks of the original code, but without nested if()s and a bit cleaner. Just add some debugging messages in there to see what part works and what not. It looks right from here, assuming that the form is constructed well. I have to declare it "closed" for tonight so I can't help any further until tomorrow. Maybe someone else will in the meantime.
-
Try checking if you have created the FULLTEXT indexes on the columns you want to be searchable. If not, have a look at this article, which gives a lot more details on FULLTEXT and how to alter your tables to add indexes.
Basically, you have to run a SQL code like this:
ALTER TABLE table ADD FULLTEXT(scientific_name, common_name_english);
BTW, "table" is a reserved word in MySQL. Don't know if that's your real table name, but if it is, changing would be a good idea
-
Every PHP function runs by the Zend Engine (internal engine, PHP engine, whatever suits it). My point was that with pathinfo() you run a single PHP function which is interpreted by the Zend Engine and some C code is executed to get the file extension. By using a mix of string or vector manipulation functions, you run 2 or 3 functions which are also interpreted by the engine. The later will normally be slower (even if for not really significant values), because it triggers a greater part of C code in the engine. Usually, achieving something with native functions is faster than with a group of functions not really related to the subject.
I think I complicated this more than it really is
-
Why not try it in the first place?
<?php $x = 105.55; if ($x >= 100 && $x <= 1000) { echo 'x is within range'; } ?>
Be it an integer or a float, if it's within the range, it will return true. Dead simple!
-
On a user experience perspective, I think it would be better if there was an "Add XSD:XML Pair" button that adds inputs dynamically, instead of the first step where they have to type how many pairs they have. It can be easily done with Javascript, especially with the help of jQuery, which makes DOM manipulation a child's game.
On the real deal! File Inputs in PHP are treated with the $_FILES superglobal, which is an array. If square brackets are used in the name (like: photo[]), different file inputs with that same name are placed in the same array with different keys. It makes validation much easier. So, basically you'll need a form as the one below. You can omit the keys, but it will make things easier as by default vectors in PHP start with the index 0 (zero).
<form method="post" enctype="multipart/form-data"> <input type="file" name="xsd[1]"><br> <input type="file" name="xml[1]"> <br><br> <input type="file" name="xsd[2]"><br> <input type="file" name="xml[2]"> <br><br> <button type="submit">Send Files</button> </form>
Once submitted, the $_FILES superglobal will become a multi-dimensional array that holds the filename, size, temporary location, etc, for each file. Make a form like the one above and add in the end of it a simple: print_r($_FILES). Then select some files, submit the form and inspect the contents of the array to understand how it works.
You can check for individual names using a code like below:
<?php echo $_FILES['xsd']['name'][2]; //will output the second "xsd" filename ?>
You can check if a value is not empty to understand if a file was selected or not:
<?php if ($_FILES['xsd']['name'][2]) { echo 'Second XSD was selected'; } ?>
In the same fashion, you can check if pairs are complete (xsd and xml is set) by using a code similar to the one below. It's the answer for question number 3).
<?php foreach ($_FILES['xsd']['name'] as $num=>$xsd) { $xml = $_FILES['xml']['name'][$num]; //check for each one individually if (!$xsd) { echo "XSD $num was not selected"; } if (!$xml) { echo "XML $num was not selected"; } //or check for pairs if (!$xsd or !$xml) { echo "Pair $num is not complete"; } } ?>
To answer your question number 2), what kind of check you want to run, on filenames or content? Both aren't complicated, but depending on filesizes, checking on content can not be a very good idea. However, here you have the two options.
<?php //check if filenames are different $xml = $_FILES['xml']['name']; $xml_unique = array_unique($xml); if (count($xml) != count($xml_unique)) { echo 'XML files should be unique'; } ?>
<?php //check if file content is different $xml = $_FILES['xml']['tmp_name']; $file_contents = array(); foreach ($xml as $file) { $file_contents[] = file_get_contents($file); } $file_contents = array_unique($file_contents); if (count($xml) != count($file_contents)) { echo 'XML files should be unique'; } ?>
For your question number 1), it is a simple procedure. You can use pathinfo() to get the extension of filenames and verify if they're .xml or .xsd.
<?php $xsd = $_FILES['xsd']['name']; $xml = $_FILES['xml']['name']; foreach ($xsd as $num=>$filename) { if (pathinfo($filename, PATHINFO_EXTENSION) != 'xsd') { echo "File $num is not a valid XSD file type"; } } foreach ($xml as $num=>$filename) { if (pathinfo($filename, PATHINFO_EXTENSION) != 'xml') { echo "File $num is not a valid XML file type"; } } ?>
It was a long one, but I hope you got things cleared up. It isn't very difficult really, but it needs some experience with multi-dimensional arrays and the $_FILES superglobal. Make some tests by yourself and by modifying the code I gave. You should get the thing you're working on up and running in no time
-
As you found out, using pathinfo() is much simpler than a mix of string or vector manipulation functions. It will also be a tad faster, because it uses the internal engine directly.
Anyway, you can also find the extension by passing an "options" parameter to pathinfo(), which should be simpler than accessing the vector's key.
<?php $file = 'hello.xml'; $ext = pathinfo($file, PATHINFO_EXTENSION); echo $ext; //will output "xml" ?>
-
Give a try to FULLTEXT indexing and searching. It will make search much better and results much more relevant. As far as I can tell, it should fix the punctuation problems, because the searching algorithm is a lot more complex than a simple WHERE LIKE.
-
I don't know your table structure, but you have an error in your SELECT statement. It should be:
SELECT * FROM table... or SELECT col1, col2 FROM table
Also, you can use the AS keyword to give the table an alias, as in: search_profiles AS sp. You can even omit it, as in: search_profiles sp, but personally I recommend it as it makes the code more readable. I would write your query like below:
SELECT reg.pref, search.search_small_image FROM search_profiles_up AS search INNER JOIN reg_profile_public AS reg USING (uin) WHERE uin='803272125132009';
-
I don't think it can be done. What htaccess does is just a redirect to the specified file when the error occurs, but it's the file's job to send the actual headers. If htaccess redirected a 404 error to 404.php, it's 404.php's job to construct a header("HTTP/1.0 404 Not Found"). There's no way, as far as I know, to check what type of error the visitor was redirected from.
Honestly, it's such an unimportant thing that you shouldn't stress yourself out. It just needs 4 or 5 files for the most common http headers.
-
Two different pages can't easily communicate with each other in real time. It would need a lot of work and in the end, would be totally pointless.
Instead of opening a new page, open the table diagram inside the main page with jQuery. It can be a modal window, a lightbox-like one, or whatever, as long as it's in the same page it's fine. It will be easy after that to modify with jQuery the table number based on the choice in the table diagram. You'll just need to set up an event handler for the table diagram click and write the choice in the input of the table number.
Hope you get what I mean.
-
There isn't any clue in your code where that functionality is trying to happen, or is it me? There should be a Javascript code that takes the username of the original author, prepends an "@" to it and writes it in the comment field. The following code, using jQuery for rapid prototyping, does all that. You'll notice that I've put the author in the "rel" attribute of the reply anchor, something that normally should be set dynamically for each reply button - that's the way I chose for this example, but you can retrieve it with other means, such as reading the html of the element (ex: $('p.author').html()).
<html> <head> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $('a.reply').click(function(){ comment = $('#comment'); author = '@' + $(this).attr('rel'); comment.val(comment.val() + author); return false; }); }); </script> </head> <body> <a href="#reply" rel="php_begins" class="reply">Reply</a> <textarea id="comment"></textarea> </body> </html>
Try it and if it's what you're seeking, feel totally free to use and modify it. The only thing it's missing is giving focus to the textarea after the reply button is pushed. I intentionally left it that way because simple and plain focus() will place the caret (cursor) at the beginning of the textarea and it's ugly. It can be easily coded to place the caret at the end of the text with a jQuery plugin.
-
<form method="post" action="index.php?status=done"> <!-- rest of form elements --> </form>
-
As thorpe mentioned, the functionality in here is very limited to be a class of it's own. If you have a user class or whatever, just stick the salting in there. Anyway, to get to the question, I rewrote your class to the one below:
<?php class PHash { private $salt_length = 20; public function makeSalt ($password, $salt) { if ($salt === NULL) { $salt = substr(md5(uniqid(rand(), true)), 0, $this->salt_length); } else { $salt = substr($salt, 0, $this->salt_length); } return sha1($salt . $password); } } //object initialization $phash = new PHash; $new_pass = $phash->makeSalt('myPASSword2011', 'phpfreaks.com'); ?>
Simple enough, but at least it works. Keep in mind that a class is just a definition and it's not supposed to process data outside its scope (as the POST superglobal is). An object does that! Plus, you have set a superglobal array element as a method parameter, which adds to the confusion.
-
You can check if $_GET['status'] isset() or even if $r['post'] is not empty. I would go for the first choice, because it make more sense in your scenario.
<?php if (isset($_GET['status'])) { ?> <div class="display-status"> <h3><?php echo nl2br($r['post']); ?></h3> </div> <?php } ?>
-
If you could post your script, everyone could get a better idea. Anyway, you can use output buffering to send headers whenever you need to.
An example of output buffering:
<?php ob_start(); <html> <head> <title>My Site</title> </head> <body> <?php $somevar = 'some value'; header("Location: page.php?somevar=$somevar"); ?> </body> </html> ob_end_flush(); ?>
-
Whatever you're trying to do, if you need duplicate keys, the logic is wrong. If you have 2 "a" indexes (a=>10, a=>20), how are you going to distinguish between different "a" key elements?
Show us the code of the inputs and how you get data from them, and I'm sure there is a way around.
-
You can't have an array with duplicate keys. Don't know if the indexes are build with any logic in it, but I would let them auto generate. In that case, there's no risk of duplication and will be combined without truncation. For example:
<?php $arr1 = array('a', 'b', 'c'); $arr2 = array('x', 'y', 'z'); $mix = array_combine($arr1, $arr2); print_r($mix); ?>
-
Try this code.
<?php $page = 1; $total = 37; $range = 3; if ($page > 1) { echo 'Previous '; } for ($i = $page - $range; $i < $page + $range; $i++) { if ($i > 0 and $i <= $total) { if ($i == $page) { echo "[$i] "; } else { echo "$i "; } } } if ($page < $total) { echo 'Next'; } ?>
-
You can do a simple search:
<?php $banned_words = array('hhhhh', 'aaaaa'); foreach ($banned_words as $word) { if (strpos($word, $text)) { echo "$word is not allowed! Don't asked me why!"; } } ?>
or replace those words directly:
<?php $search = array('hhhhh', 'aaaaa'); $replace = ''; $text = str_replace($search, $replace, $text); ?>
EDIT: Now I'm seeing I totally misunderstood your question. Sorry.
how to get rid of \r\n\ ??
in PHP Coding Help
Posted
@tomfmason, doesn't trim() just remove them in the beginning or end of a string? The OP's string had linebreaks in the middle...