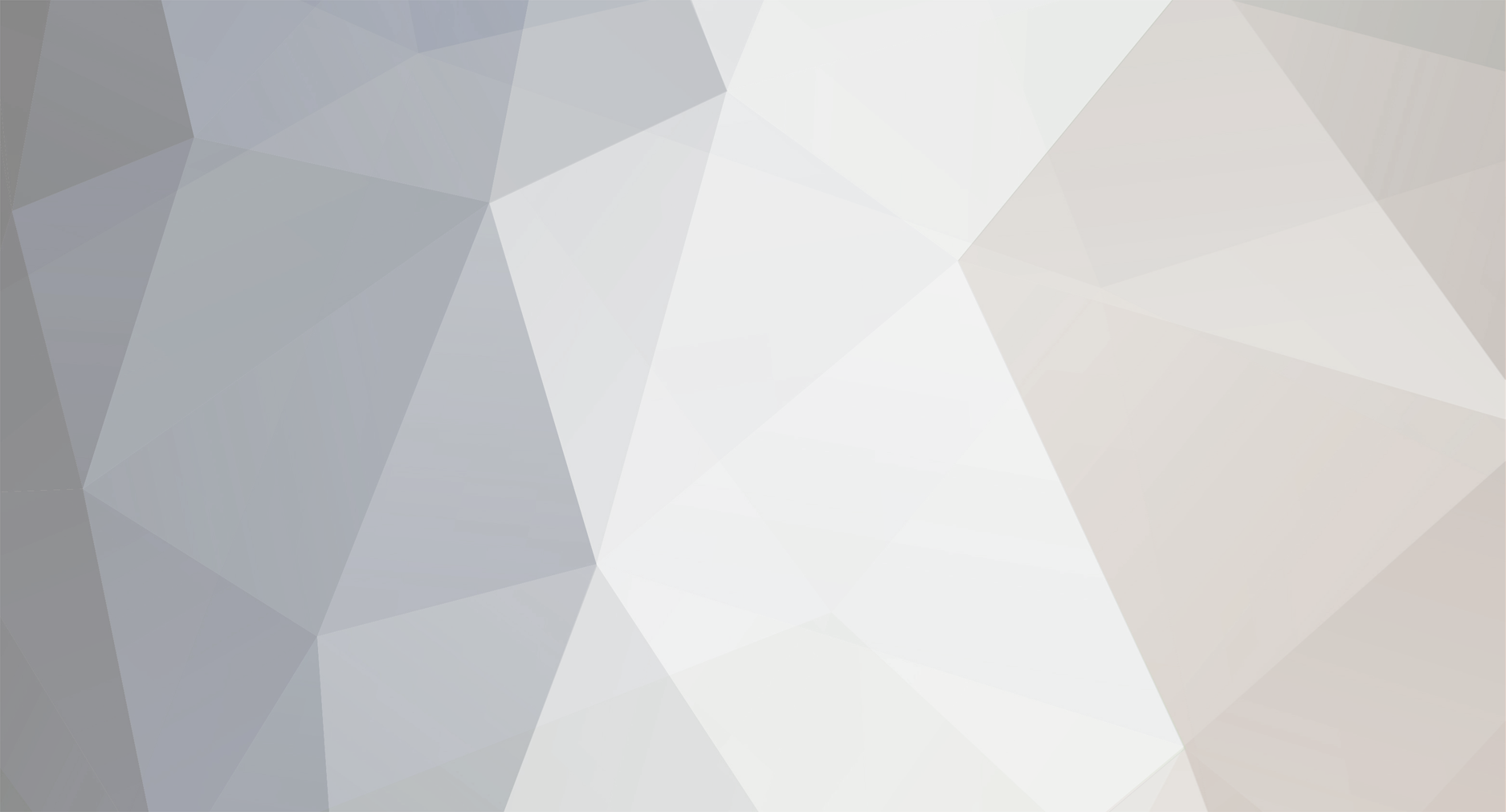
Fadion
-
Posts
1,710 -
Joined
-
Last visited
Posts posted by Fadion
-
-
When generating a contact form, appart from the typical "name, email, message", you can offer things such as dropdowns (<select>), checkboxes, etc. Visitors may need to generate a form that's more specific, where a "department" may be needed and stuff like that. Anyway, redixx's approach is a good start.
What you'll need to generate is PHP code too. If the contact form is supposed to be a copy-paste solution, it must have some PHP code that actually processes the user generated form and sends an email. Having some validation fields for each form element (required, email, phone number, etc) will help too. Finally, generate the form from the filled-in values and some PHP code too that validates the form (based on validation fields) and sends the email. It should be fairly easy with the code you got.
-
You better limit the string first and than wordwrap it. That way you won't be truncating line breaks.
Adding to it, word wrapping is better done with CSS than PHP. You can limit the string with PHP and than wrap it with a fixed-width container and a word-wrap:break-word CSS property. It's safe enough.
-
Actually no. Because the 'id' GET variable is converted to integer, it won't break the query. What it may do however, is get 0 results from the query if it is manipulated, something taken care of the: if(!mysql_num_rows($result)) part. You're safe to go.
-
<?php mysql_connect('localhost', 'ash', 'lash') or die('Connection Failed'); mysql_select_db('lash') or die('Database not found'); $id = (int) $_GET['id']; $result = mysql_query("SELECT title, dtl, meta_description, meta_keywords FROM page WHERE id=$id"); if (!mysql_num_rows($result)) { header('Location: 404.php', true, 404); die(); } $row = mysql_fetch_array($result); $title = stripslashes($row['title']); $dtl = stripslashes($row['dtl']); $meta_desc = stripslashes($row['meta_description']); $meta_keys = stripslashes($row['meta_keywords']); ?> <html> <head> <meta name="description" content="<?php echo $meta_description; ?>" /> <meta name="keywords" content="<?php echo $meta_keywords; ?>" /> <title>MySite.com - <?php echo $title; ?></title> </head> <body> <h1><?php echo $title; ?></h1> <?php echo $dtl; ?></body> </html>
As the script is intended for production, I added some control if the row exists. You can't show an article when it doesn't exist, right? In that case it does a redirect (with a 404 status code) to a "404.php" page. The best way to do that would be just sending a 404 header and creating an .htaccess file with an ErrorDocument for 404. Anyway, it will work the same.
I added the variables too (ie. $title = stripslashes($row['title']))
-
The script you have finished using a tutorial can be used in the same page you have the mail form. It's pretty much the same thing, just that when the captcha is correct, you send the mail.
reCAPTCHA is a service that generates captchas and offers a developer's API to be easily used in your website. It's like your normal captcha thing, but a lot more difficult to crack and by using it you help digitalizing books
-
As every page will need to have it's own title and meta tags ("description" I guess), your best bet is adding them to the article rows. In example:
Table "articles"
--------------------------------------------
id | title | content | meta_description
--------------------------------------------
1 | Apples | Apples are healthy to eat everyday | Not all apples are red
<?php $id = $_GET['id']; $results = mysql_query("SELECT title, content, meta_description FROM articles WHERE id=$id"); $values = mysql_fetch_assoc($results); $title = $values['title']; $content = $values['content']; $meta_description = $values['meta_description']; ?> <html> <head> <meta name="description" content="<?php echo $meta_description; ?>" /> <title>MySite.com - <?php echo $title; ?></title> </head> <body> <h1><?php echo $title; ?></h1> <?php echo $content; ?> </body> </html>
The code is just to give you a basic idea of how to handle it, but you'll need to validate input, check if the article exists, stripslashes, etc.
-
Integrate the captcha code into the mail page, otherwise you'll need to pass email information using GET variables or SESSION (which won't be very great). If everything goes wrong with your captcha integration, you can even use reCAPTCHA.
-
Do you have any example of what you need to do? Unfortunately, not everyone knows guitar and even less can read minds.
-
This question has been asked a dozen times recently. Anyway, you have 2 options to retain the selected value.
<select name="defpt"> <option value=".9" <?php if ($_POST['defpt'] == '.9') { echo 'selected="selected"'; } ?>>attacker</option> <option value=".9" <?php if ($_POST['defpt'] == '.9') { echo 'selected="selected"'; } ?>>Economist</option> <option value="1.15" <?php if ($_POST['defpt'] == '1.15') { echo 'selected="selected"'; } ?>>Miner</option> <option value="1.5" <?php if ($_POST['defpt'] == '1.5') { echo 'selected="selected"'; } ?>>Explorer</option> <option value="1" <?php if ($_POST['defpt'] == '1') { echo 'selected="selected"'; } ?>>Standard</option> <option value="1.1" <?php if ($_POST['defpt'] == '1.1') { echo 'selected="selected"'; } ?>>Researcher</option> </select>
What it does is simply check if the POST value of 'defpt' is equal to an option and if yes, put a "selected" attribute. Beware that you have two options with the same value.
The second way would be much easier to create and handle by using PHP arrays.
<select name="defpt"> <?php $options = array(0.9=>Attacker, 1.15=>Miner, 1.5=>Explorer, 1=>Standart, 1.1=>Researcher); foreach ($options as $value=>$name) { $selected = ''; if ($value == $_POST['defpt']) { $selected = 'selected="selected"'; } echo '<option value="' . $value . '" ' . $selected . '>' . $name . '</option>'; } ?> </select>
What I did was put all the options in an array ("value" as key, name as value) and printed all them in a loop. Used the $selected variable to check if the option was selected or not.
-
-
The approach is quite simple. You can treat values from inputs as array elements and manipulating them is pretty easy. Take a look at the following code:
<form method="post" action=""> <?php //Input Generation $nr_passengers = 3; for ($i = 0; $i < $nr_passengers; $i++) { ?> <input type="text" name="full_name[]" /><br /> <input type="text" name="date_birth[]" /><br /><br /> <?php } ?> <button type="submit" name="submit">Submit</button> </form> <?php //Form Validation if (isset($_POST['submit'])) { $names = $_POST['full_name']; $dates = $_POST['date_birth']; if (count($names) == count($dates)) { $passengers = array_combine($names, $dates); foreach ($passengers as $key=>$value) { echo $key . '->' . $value . '<br />'; } } } ?>
I made 2 inputs that were added to the POST superglobal as arrays (the square braces in the name attribute allow that). In the form validation code, I got those arrays from POST and combined them with array_combine where the passenger name is the key and the date of birth the value. You can further print that combine array ($passengers), manipulate it or whatever you need to do.
-
Just a quick code that gets the job done. It's tested, but you can take a look for any possible optimisation.
<?php /* ** Date Conversion */ $dates = array(array('2011-05-26', '2011-05-28'), array('2011-05-28', '2011-05-30')); function convertDate (&$array, $key) { foreach ($array as &$v) { list($year, $month, $day) = explode('-', $v); $v = $month . '/' . $day . '/' . $year; } } array_walk($dates, 'convertDate'); //print_r($dates); /* ** Dates Printing */ $dates_print = ''; foreach ($dates as $array) { $values = array_values($array); $dates_print .= implode('-', $values) . ', '; } $dates_print = trim($dates_print, ', '); //echo $dates_print; ?>
-
A 301 header and a redirect would do the job perfectly. Just create a blank .php file in your old website and write:
<?php header('HTTP/1.0 301 Moved Permanently'); header('Location: http://www.yournewsite.com/'); ?>
or the shorter (by 1 line
) version
<?php header('Location: http://www.yournewsite.com/', true, 301); ?>
-
As you only need to check if a string contains another string, stripos will be faster than stristr().
An example:
<?php $myurl = 'http://mydomain.com/abc/moretext.html'; $search = 'abc'; if (stripos($myurl, $search) !== false) { echo 'This URL contains useful data.'; } ?>
However, just a simple string search won't work very well in your case. Imagine having a url: "http://www.abcdomain.com/cde/sometext.html" and you're searching for "abc". In this case stripos() would return true because it finds the string (no matter where it is) and it may not be much of help. You could just search for with stripos() for "abc/" or better, use a code like follows:
<?php $myurl = 'http://mydomain.com/abc/moretext.html'; $search = 'abc'; /* ** Used parse_url() here just to make the output cleaner. ** It's used to get the path (everything after the domain name), ** but it can be ommited and skipped directly to explode(). */ $path = parse_url($myurl, PHP_URL_PATH); $path = trim($path, '/'); $pieces = explode('/', $myurl); if (in_array($search, $pieces)) { echo 'This URL contains useful data.'; } ?>
The code explodes the path to an array, where each individual path element is an array item. In this way you check for actual path data, not what a string contains.
Hope it helps
PS: Others have posted about stripos() while I was typing, but anyway.
-
This has nothing to do with PHP obviously. Javascript can achieve that (a simple google search tells you that), but it would be totally unnecessary. When visitors open a page, they don't expect it to resize or do whatever a page normally doesn't do. It would be a lot better to place the flash movie in the center with a dark background.
-
Using a config file is alright. Personally I like using MySQL to store config data, as it's a cleaner and easier method to manage. For example:
table "config"
----------------
name | value
----------------
site_url | mysite.com
site_title | My Site Title
It has just a bit of overhead compared to just including a PHP file, but that shouldn't be a problem for most sites.
-
When the form is submitted, check if the email isn't empty (via $_POST or $_GET) and if not, update the data in database. There's no need to compare the form value with the actual value from database, because if the email isn't empty, it is supposed to be changed.
<?php $email = trim($_POST['email']); if ($email != '') { $results = mysql_query("UPDATE users SET email='$email' WHERE user_id=10"); } ?>
Hope I got it right, anyway.
-
You can use Exceptions to catch errors and log them in a database.
-
The process you're referring is called scraping (web scraping, screen scraping, whatever). Basically, you read the page's html content and parse that data to filter what you need. Depending on the website's structure, it may be difficult to parse and slow as hell (read, parse, insert into db, repeat).
From what I know, the easiest way to go is using Regular Expressions - quite confusing for experienced coders, let alone new ones. A lot easier could be using an already built parser that filters data with only a few lines of code. Take a look at PHP Simple HTML DOM Parser. Have tried it once and it worked nicely.
Anyway, tell us what the site is and I'm sure someone will help.
PS: Check if the website has any API. It will make your life a lot easier. Also, if you need to post data to forms (search forms for ex), you may have to take a look at cURL.
-
You can use mkdir to dynamically create directories. Keep in mind that you can't just use any name for files and directories. Different filesystems have specific rules on file/folder names and you have to clear those characters out. Here is a round-up on different systems (just a random google search).
-
Hmm... you need a search engine for PHP files? What in the world you need it for? You mean filenames or file contents?
Anyway, you better explain what you're trying to do here, because the logic seems quite off in the first place.
-
I don't know for sure what you're trying to achieve, but from your description, the code below is much simpler and a lot faster.
<?php $arr = array('One', 'Two', 'Three', 'Four', 'Five'); shuffle($arr); foreach ($arr as $a) { echo $a . '<br />'; } ?>
-
Are you displaying data appart from the form and you need the see the changes when the form submits? If so, place the form submit code before the code that displays data. Example:
[php code to display some data]
[form][/font]
-
You are storing mp3 files as binary data (blob)? I've never tried and seen such an approach, but I'm guessing it's not the best. What if there are thousands of mp3s downloaded by hundreds of users? I doubt reading that much data from a database will perform well.
I would go for a normal approach, where you save the mp3 as a normal file and store in a database just the path. After that, you can use pretty much the same code as you have, but you'll need to use readfile instead of just echo()ing the content.
Finally, if you're serving big mp3 files, you'll have to split them in chunks or better, use an apache module (if you're using apache) to serve downloads via apache, not PHP. I've been using the X-Sendfile (download and a simple guide here) apache module for a while in a site that servers files as big as 3GB. It works like a charm.
templating system, inserting php code into the page...
in PHP Coding Help
Posted
Basically, PHP is a templating system for HTML. Adding another layer of abstraction will only get things more complicated. With just a little caution, you can use standart PHP code as a template. Anyway.
What I don't get in there is the use of eval. Isn't your templating system a pseudo-code based one, where each is replaced with an actual value? As I'm not quite sure if you understand templating systems, I wrote a very quick and basic one just to give you the idea.
First I made 2 files called "header.tpl" and "content.tpl" in a folder called "tpl".
header.tpl
content.tpl
The simple templating class
How to use it