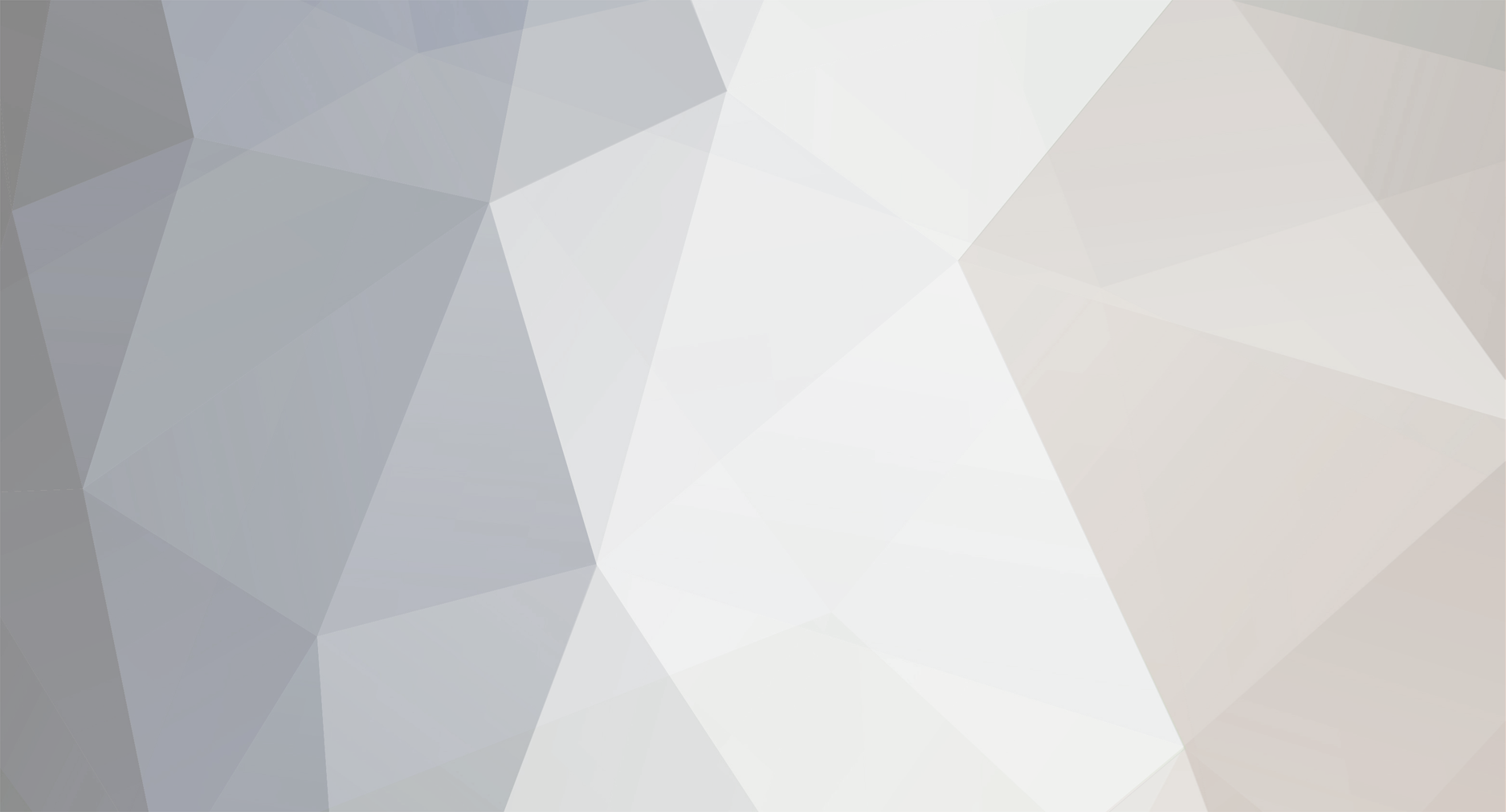
Fadion
-
Posts
1,710 -
Joined
-
Last visited
Posts posted by Fadion
-
-
You'll have to use the comparison operator: ==
//string datatype if ($row['relation'] == '') {} //numeric datatype if ($row['relation'] == 0) {}
-
That query makes no sense. What is the table structure? What value are you passing to $table1?
-
do you have any code
or you want us to
write it for you?
it can be easily
done in Javascript
the form
place it within the <body> section of your document
<form method="post"> <div> <input type="text" name="question[]" /> </div> <button type="button" id="add">Add Question</button> <button type="submit" name="submit">Submit</button> </form>
javascript code (with the jQuery library)
place it in the <head> section of your document
$(document).ready(function(){ $('#add').click(function(){ $('form div').append('<input type="text" name="question[]" />'); }); });
php code to process the form
place it within the <body> section
if (isset($_POST['submit'])) { //this will return an array holding all the values of the inputs $questions = $_POST['question']; foreach ($questions as $question) { $results = mysql_query("INSERT INTO questions (question) VALUES ('$question')"); } echo 'Your questions were added and will be responded soon. Bye!'; }
test the code and modify it
to your needs
you will need to add some
error handling to the php code
-
You'll have to create a relation between the 2 tables, otherwise you're using MySQL simply as a data storage solution, not as a RDBMS.
table "games"
--------------------------------
id | team1 | team2 | result
--------------------------------
1 | Barcelona | Manchester Utd | 3-1
2 | Milan | Inter | 0-2
table "bets"
-----------------------------
id | game_id | bet | won
-----------------------------
1 | 1 | X | 0
2 | 2 | 2 | 1
What you can do here is insert matches in the "games" table, together with the result (will be set when the match finishes, obviously). In the bets table, you have the played bets with a reference to the "games" table (game_id) that specifies what game has been played and the final bet (1, X or 2). You also have a field "won" that is set to "1" if the bet is a win (correct guess), or "0" if the bet lost - this field is set when the match finishes.
Writing PHP code to validate bets should be trivial right now. Take a look at the database scheme and return here if you have problems with code.
-
That form is quite complicated and will need some fair amount of client-side scripting (Javascript). What's more important in this stuff is the logic behind it, as to what that spell does, what that hero is and whatever. But this is a help forum though, not a "how much does this cost" one.
-
Something as simple as this should work:
<?php $s = '&Itemid=457'; if (preg_match('|&Itemid=(\d+)|', $s, $match)) { echo $match[1]; } ?>
You could even use PHP's string manipulation functions. They're not as flexible and scalable as regex, but if the case is simple enough, they get the job done.
<?php $s = '&Itemid=457'; $nr = substr($s, strrpos($s, 'Itemid=') + strlen('Itemid=')); echo $nr; ?>
-
Member AbraCadaver gave a good solution in this thread that will work in your case too.
<?php $str = 'some dynamic text'; $file = 'testFile.txt'; $lines = file($file, FILE_IGNORE_NEW_LINES | FILE_SKIP_EMPTY_LINES); if (!in_array($str, $lines)) { $h = fopen($file, 'a'); fwrite($h, $str . "\n"); fclose($h); echo 'Line written successfully.'; } else { echo 'Line already exists.'; } ?>
-
-
The $_FILES array is multidimensional and looks like this:
[key 'xxx'] => {
[tmp_name] => 'some_name'
[name] => 'some_name_again'
and so on...
}
When you loop through it, you're referencing keys with the $name variable and values with the $file variable. Trying to access $file['tmp_name'] makes no sense, because $file is a string. That's why copy() is giving an error, because it expects a string.
Said that, there's no need to loop through $_FILES unless you have a multiple upload form and for that, you'd have to iterate the array in a slightly different case. If you have just one upload input, just access $_FILES like this:
<?php echo $_FILES['input_name']['tmp_name']; ?>
where 'input_name' is the name attribute of your file input. If you still aren't clear enough, I will give you a full example.
-
You can use a session variable as an array to keep track of the replied questions and use that array to find a random question that has not been replied yet. The following code is not tested, but should give a general idea.
<?php session_start(); //processing the form if (isset($_POST['submit'])) { $id = (int) $_POST['id']; $answer = mysql_real_escape_string($_POST['answer']); $results = mysql_query("SELECT answer FROM questions WHERE id=$id"); if (mysql_num_rows($results)) { $values = mysql_fetch_assoc($results); if ($values['answer'] == $answer) { //add the question ID to the session array $replied = $_SESSION['replied']; $replied[] = $id; $_SESSION['replied'] = $replied; echo 'Correct answer. Moved to the next question'; } else { echo 'Incorrect answer. Please try again.'; } } } $replied = $_SESSION['replied']; $query_modifier = ''; //if any question has been replied if (count($replied)) { $query_modifier = "WHERE id NOT IN (" . implode(', ', $replied) . ")"; } //you could even get a random question, but that won't be much needed. It will always show 1 question //that has not been answered yet. $results = mysql_query("SELECT id, question, answer FROM questions $query_modifier LIMIT 1"); $values = mysql_fetch_assoc($results); ?> <form method="post" action=""> <h1><?php echo $values['question']; ?></h1> <input type="hidden" name="id" value="<?php echo $values['id']; ?>" /> <input type="text" name="answer" /> <button type="submit" name="submit">Reply</button> </form>
As you can see, the logic is quite simple. I used a session variable as array to hold the question IDs that are already successfully replied and made the query such that it doesn't show any of the replied questions. The same session is used when submitting a reply; it is added the question ID when the answer is correct.
Try it and tell me if you have any problems.
-
You can get the last letter with:
<?php $s = 'some text'; echo $s[strlen($s)-1]; //will output: t ?>
and you can find if the last 3 characters are "ies" with:
<?php $s = 'bodies'; if (substr($s, strlen($s) - 3) == 'ies') { echo 'Ends with: ies'; } ?>
Not sure if any word that plural makes "ies" and with "y". Anyway if it isn't for anything really important, it doesn't matter much.
-
ok I can explain what he wants
he wants to upload image from a form
then let's say the image name is 123.jpg
he wants to make page names 123.php immediately(automatic)
how can he do that??????????????
I already posted some code that can help him do that.
-
If you're doing this for a class and need to save it as binary in a database, than ok. Otherwise, I highly suggest saving images just as paths in the database.
I'd suggest making another php file, let's say called image.php which servers the image. Your code will be much more tidy and you can reuse the code in several places.
image.php?id=10
<?php $film = $_GET['id']; $getimage = mysqli_query($connection, "SELECT * FROM Bild WHERE filmNr='$film'"); $image = mysqli_fetch_array($getimage); $filnamn = $image['filnamn']; $filtyp = $image['filtyp']; $fildata = $image['fildata']; $disposition = 'inline'; header("Content-type: $filtyp;"); header("Content-disposition: $disposition; filename=$filnamn"); header('Content-length: ' . strlen($fildata)); echo $fildata; ?>
using the image
<img src="image.php?id=10" />
You'll need to add error checking in your image.php file (if "id" isset and if "id" exists in the "Bild" table) and you're set. You can loop or whatever, just you'll need to pass the id to image.php.
-
What you're asking isn't the clearest thing on earth, so please give some more detail. What are you trying to achieve?
-
You can use data from a drop-down in the same way as in other form elements. In your case:
<select name="options"> <option value="1">6 disc DVD changer</option> <option value="2">TV function</option> <option value="3">DAB digital radio</option> </select> <?php echo $_POST['options']; ?>
The selected value of the drop-down will be echoed. You don't need to treat the <select> as an array (name="options[]") because it can return just one value. You will need that instead when working with checkboxes.
-
Is there any reason why you're saving images as binary data? It's not something of a great solution in most cases, because you can save in database just the image path and keep that image in a folder. The system will be easier to mange too.
-
You'd better go for a different approach on error handling. As you have it, you show just one error, even if there are more. Take a look:
<?php //check if the form has been submitted //$_POST['submit'] is the post value of the submit button (if you have one) if (isset($_POST['submit'])) { $errors = array(); if ($_POST['cartype'] != '') { $errors[] = 'Please select car type'; } if ($_POST['carcolour'] != '') { $errors[] = 'Please select car colour'; } //and so on for every input you need validated //if no error occurred, process the form if (count($errors) == 0) { //process the form } else { //print errors foreach ($errors as $error) { echo $error . '<br />'; } } } ?>
Also, keep in mind that isset() checks if a variable exists. In the case of POST, variables will be created and return true to isset(), even if they're empty. It's better to check for length with strlen() or value.
-
You have two errors I noticed immediately
First (double curly brace):
if($serverSettings['register_on'] && (!isset($_SESSION['user_admin']) && !checkInt($_SESSION['user_admin']) && !$_SESSION['user_admin']>=0)) { {
should be:
if($serverSettings['register_on'] && (!isset($_SESSION['user_admin']) && !checkInt($_SESSION['user_admin']) && !$_SESSION['user_admin']>=0)) {
and Second (missing semi-colon):
echo'<p class="meldung">Die Registration ist deaktiviert oder Sie sind bereits angemeldet. Es kann kein weiterer Account erstellt werden.</p>'
should be:
echo '<p class="meldung">Die Registration ist deaktiviert oder Sie sind bereits angemeldet. Es kann kein weiterer Account erstellt werden.</p>';
Syntax errors are what they say, syntax errors. In most cases they are missing braces, semi-colons, parantheses, quotes, etc.
-
I would go for another approach.
First, make the link to twitter go into a social.php page like this:
<a href="social.php?p=twitter">Follow me on Twitter</a>
Then make social.php
<?php $social_sites = array('twitter', 'facebook', 'linkedin'); $site = $_GET['p']; if (!in_array($_GET['p'], $social_sites)) { die('What were you thinking?'); } $ip = $_SERVER['REMOTE_ADDR']; //or whatever u use to get the visitor's IP $results = mysql_query("INSERT INTO social_clicks (site, ip) VALUES ('$site', '$ip')"); $url = "http://www.$site.com"; header("Location: $url"); ?>
The script is very simple. Based on the "p" url parameter (passed by GET) it specifies what kind of social media the user wants to visit. It could be social.php?p=facebook for Facebook and so on. If the social media is correct (in the white list), you insert a row in the database with the right social media and IP, and finally redirect him to the correct url. It may certainly need some extra code, but it should give the general idea.
I assumed you needed a way to handle more than one social media (for scalability's sake), but if you want just Twitter, it can be easily modified.
-
You mean something like this?
<?php $template = 'templates/pictures.php'; $picture = 'lolcat.png'; //this could be the uploaded picture //we need just the filename - no extension $picture_name = pathinfo($picture, PATHINFO_FILENAME); copy($template, "templates/$picture_name.php"); ?>
-
The simplest solution that come to mind is printing PHP directly to the Javascript code, but that wouldn't be very clever. I'm talking about something like below, where POST data is printed directly in the Javascript code.
$(function(){ $(".tweet").tweet({ join_text: "auto", username: "<?php echo $_POST['username']; ?>", avatar_size: 68, count: 25, auto_join_text_default: "said,", auto_join_text_ed: "I", auto_join_text_ing: "I wase", auto_join_text_reply: "I replied", auto_join_text_url: "I was checking out", loading_text: "loading..." }); });
The best method would be using just Javascript and no PHP. You can make an input and a button, so when the user submits it, you catch that data with Javascript and pass it to the rest of the code you have. Something like:
//js code $('button').click(function(){ var username = $('#username').val(); //this is the username }); //html code <input type="text" name="username" id="username" /> <button type="button">Submit</button>
You'll need to provide alternate form submitting for non-javascript users, but as your functionality is based on Javascript, I guess you don't care.
-
Simply put, you can use file_get_contents() to get the page output and run a regex to filter out what information you want. That's basic scrapping. From what I saw, the season information are put in a structure like the following one:
<div class="filter-all filter-year-2001"> <hr /> <table cellspacing="0" cellpadding="0"> <tr> <td valign="top"> <div class="episode_slate_container"><div class="episode_slate_missing"></div></div> </td> <td valign="top"> <h3>Season 1, Episode 1: <a href="/title/tt0502165/">12:00 a.m.-1:00 a.m.</a></h3> <span class="less-emphasis">Original Air Date—<strong>6 November 2001</strong></span><br> Jack Bauer is called to his office because there's a threat on the life of a US Senator who's running for President... </td> </tr> </table> </div>
Running a regex to get what's inside <div class="filter-all filter-year-2001"> will return you all the seasons information. I'm not a regex expert, but I wrote just a simple one to get you that information. You'll have to figure out by yourself how to get the title and description without any html around it.
<?php $str = file_get_contents('http://www.imdb.com/title/tt0285331/episodes#season-1'); preg_match_all('|\<div class=\"filter-all filter-year-2001\"\>(.+)\</div\>|', $str, $matches); print_r($matches); ?>
EDIT: I noticed that <div class="filter-all filter-year-2001"> changes based on the season's year. You can easily run a loop from the starting year to the ending one. Scrapping is a b*tch
-
or
$date = date('Y-m-d H:i:s', strtotime('+2 Hours'));
-
Hmmm what?! I can't understand anything of what you've written...
Sessions and Cookies
in PHP Coding Help
Posted
No one will help you like this! Try debugging your code by yourself and post here only any part of it that is misbehaving. This is a help forum - no one will download a zip and waste his/her time with what's inside it.