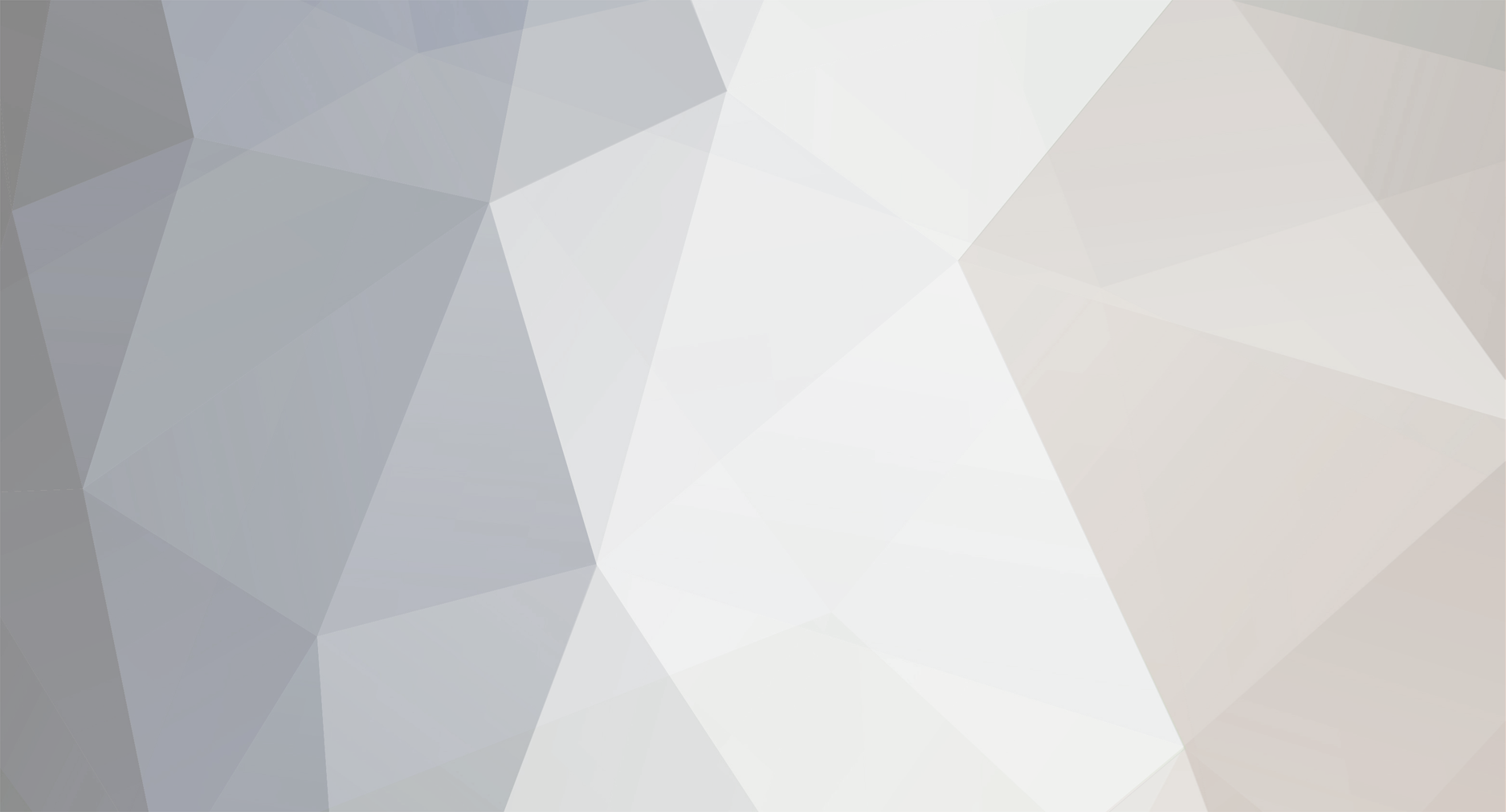
Fadion
-
Posts
1,710 -
Joined
-
Last visited
Posts posted by Fadion
-
-
There's no really a better way of doing it. The only thing I can think of is doing a subqyery in the WHERE clause, which will make the code shorter as you won't have to fetch the array. Take a look at the following code, try it and decide if it's worth it.
$shop_id = 1; $address = 'Some Address'; $results = mysql_query("UPDATE addresses SET address='$address' WHERE id=(SELECT address_id FROM shops WHERE id=$shop_id)");
I simplified column names to keep it shorter, but it's basically your 2 queries written in just 1.
-
You can use a simple code like this one:
<?php $ip = '11.11.11.11'; $lines = file('somefile.txt'); foreach ($lines as $line) { if ($line == $ip) { echo "$line OK<br />"; } else { echo "$line BAD<br />"; } } ?>
file() returns an array where each element is a line of the read file. I assumed your file contains just IP Addresses and no parsing was needed.
-
Your question doesn't make much sense. Explain better the workflow you're trying to achieve.
-
Everyone's been in your phase and there's nothing wrong with it. The important is to keep trying
I would rewrite your function code like this:
<?php function latestHunterNews () { //I put the or die(mysql_error()); part so you know if any error occurs $results = mysql_query("SELECT id, title FROM hunter_news ORDER BY id DESC LIMIT 1") or die(mysql_error()); //There's not need to run a while() loop on a result-set with only 1 row $row = mysql_fetch_assoc(); return $row; } ?>
While in main.php
<?php include('includes/connection.php'); //this is your standard db connection code include('includes/functions.php'); //your functions file $latest_news = latestHunterNews(); ?> <div id="news"> Latest News:<br /><br /> <span class="bolddark"><a href="hunters.php?id=<?php echo $latest_news['id']; ?>"><?php echo $latest_news['title']; ?></a></span> </div>
As a note on the code I wrote: database connection doesn't have scope. It's kept alive as long as the script runs and queries can be made drop-in inside functions and classes, as long as they're included in the main file. If there isn't any error in your query and you've set up the database connection correctly, the script should run fine.
-
I'm sure you will get along better with something like jQueryUI Auto Suggest or any jQuery plugin for that matter. The jQueryUI one is pretty simple to set up and works with a remote dataset that can be a PHP script outputting database words in JSON format. It handles the rest by itself.
-
Hmmm you mean:
<form method="post" action="action.php" target="_blank"> <input type="text" name="myfield" /> <button type="submit">My Button</button> </form>
The "target" attribute is deprecated in XHTML and will not validate with a Strict Doctype, but brought to life in HTML5. You can use it safely as browser support is assured.
-
Glad you fixed it up, but please mark the topic as "SOLVED" so others don't think you still need help.
-
Loading example.com/ and example.com/index.php should be the same, unless you have a "index.html" (typical in Apache installation: in DirectoryIndex, index.html is served first). Otherwise, session data should be available. Try posting some code.
-
Expert is big stuff, so my advice is to stay away from it for a while - unless you have good general programming knowledge and been on PHP for a few years. Anyway, this is a subject where's there's lots to learn, be it PHP-related or not, so using the term "expert" is very subjective.
The best way would be telling an experience, so I'm telling mine [hopefully] briefly. I did start PHP with an absolute beginner's book (can't remember the title) and that set me in for the basic principles. Then I started working on a few small projects just to test what I did learn. In the meantime I was active in this forum and I have to say it: it has helped me more than any book. I tried to help people by researching myself, read other's opinions and also asked questions when there was no way I could find an answer. When I felt confident enough, I started reading more advanced topics about objects, patterns, performance, the environment, etc, and tried writing more logical and modular code. As I said previously, there's a lot going on so you better start exploring it right now.
By the way, I started PHP by 2007 and have been working full-time (employed and from 1 and 1/2 years freelance) as a web designer and developer. It helps a lot being in the "field".
-
would rand be truly random and would it be impossible for a duplicate ?
mt_rand() will generally be a better idea, but in either case, it doesn't matter much. Random passwords, as the OP stated, are temporary solutions that are going to be changed in most cases. If he wants to increase security, in grahamb314's code he can increase the length, add some non-alphanumeric characters to the $chars list, hash it and finally drop in a salt. Collision in this case is not even an issue.
-
You'll need to target a specific key of the array. In your case, you have keys: 1 and 2. If you leave keys empty, they'll be generated starting by zero (0).
<?php $bellProductsArray=array(1=>"Apple_iPhone3GS.jpg", 2=>"Apple_iPhone4.jpg"); echo $bellProductsArray[1]; //will print "Apple_iPhone3GS.jpg echo $bellProductsArray[2]; //will print "Apple_iPhone4.jpg ?>
Or otherwise you can leave the keys empty:
<?php $bellProductsArray=array("Apple_iPhone3GS.jpg", "Apple_iPhone4.jpg"); echo $bellProductsArray[0]; //will print "Apple_iPhone3GS.jpg echo $bellProductsArray[1]; //will print "Apple_iPhone4.jpg ?>
By the way, arra_values() will just return an array holding the values of the original array and the keys will be numerical, starting from zero. There's no use of that function in this case.
-
Using fenway's query with PHP code:
<?php $results = mysql_query("SELECT COUNT(DISTINCT column) AS myCount FROM table"); $values = mysql_fetch_assoc($results); echo $values['myCount']; ?>
-
You have a missing brace, that's for sure, but your code is a mess, so I'm just guessing it's for the first if (if (isset($_POST['submit'])) { // Handle the form.). Just check if you've closed every brace and as you're on it, apply some indentation.
There are a few problems with your $theResults html output. I would put the whole HTML in a text or html file and use file_get_contents() to get it - it would clean the code a lot. Or better yet, I wouldn't print the whole HTML page with PHP - it is a very bad practice that makes code totally unreadable.
As for the header injection, try google as there are plenty of tutorials on that.
-
Included PHP files work as if they were actual PHP code in the same page, inheriting scope and the ability to use variables defined before the inclusion. So, the behavior, as shallow as you describe it, makes no sense.
I guess you're sure the path to the included file is correct, so try printing any of the variables to see if there's any output. Also, what do you mean by "no longer works properly"? It doesn't work at all, or not in the way you expect it to?
PS: There's no need to achieve what you're trying with Javascript. You can create a CSS class (ex: .red-border { border:1px solid red; }) and apply it to the elements. It will be faster and accessible to anyone.
-
The way I read it, it sounded as though you were implying a boolean value is never returned by mysql_query, which clearly isn't the case.
I've read the manual! The sentence I quoted previously is exactly what we're discussing about. While in the first part of it (mysql_query() doesn't return a boolean value), which I'm seeing now that it may be ambiguous, I was referring to the specific case mentioned in this thread. Anyway, it doesn't really matter.
Cheers
-
That's what I said: "instead it returns a resource identifier that can't be evaluated to true/false if there isn't any error in the query."
-
mysql_query() doesn't return a boolean value; instead it returns a resource identifier that can't be evaluated to true/false if there isn't any error in the query. As I said in my previous post, an empty result set doesn't trigger an error, but missing quotes can!
-
Try:
$query = mysql_query ($sql) or die (msql_error());
Check what error are you getting and post it here. I suspect the "K_Type" part is causing it, as it most probably is not numeric and you've not used quotes.
$sql = "SELECT Friendly, K_Type FROM SAYusersX WHERE UserKey = '$val' AND K_Type = '$type'";
-
If you want no errors at all, you can turn display_errors off in php.ini (or in a htaccess file if you don't have access to php.ini). That would be a must in a production server.
If a query returns zero rows, that doesn't trigger an error, so post how your query looks and any variable used in it.
-
Thanks guys , that worked fine.
Instead of using echo $_SERVER['PHP_SELF'] is it OK to just use the url of the same page the form is on?
You can leave the action attribute empty to submit the form in the same page. It is completely valid XHTML and also included in the form submission algorithm of HTML5 (look here, point 9).
-
3 bumps in less than a day? Who's willing to help, will help without bumps!
Explain your problem as we can't read minds. What are the "no images"?
-
This is a "Website Critique" forum, not a "Join my Team" one. It's like going to the coffee shop and asking for shoes. Live up with the suggestions given here, which I personally find realistic.
-
As you have set Rewrite Base to root (/), there's no need to write the whole url.
RewriteEngine On RewriteBase / RewriteRule ^partners/([\w-]+)/([\w-]+)/?$ channel-partners/en/index.php?location=$1&name=$2 [L]
-
Because the url doesn't contain any "username" parameter in the query string. Instead it contains a "boxerman" parameter.
It should be: members_profile.php?username=boxerman
Doing php/mysql the right way.
in PHP Coding Help
Posted
I would recommend Art of SQL. It's pretty much of a classic that goes into really deep details about schema and performance. It needs some experience though, but you can get the grasp of lots of stuff while reading it.