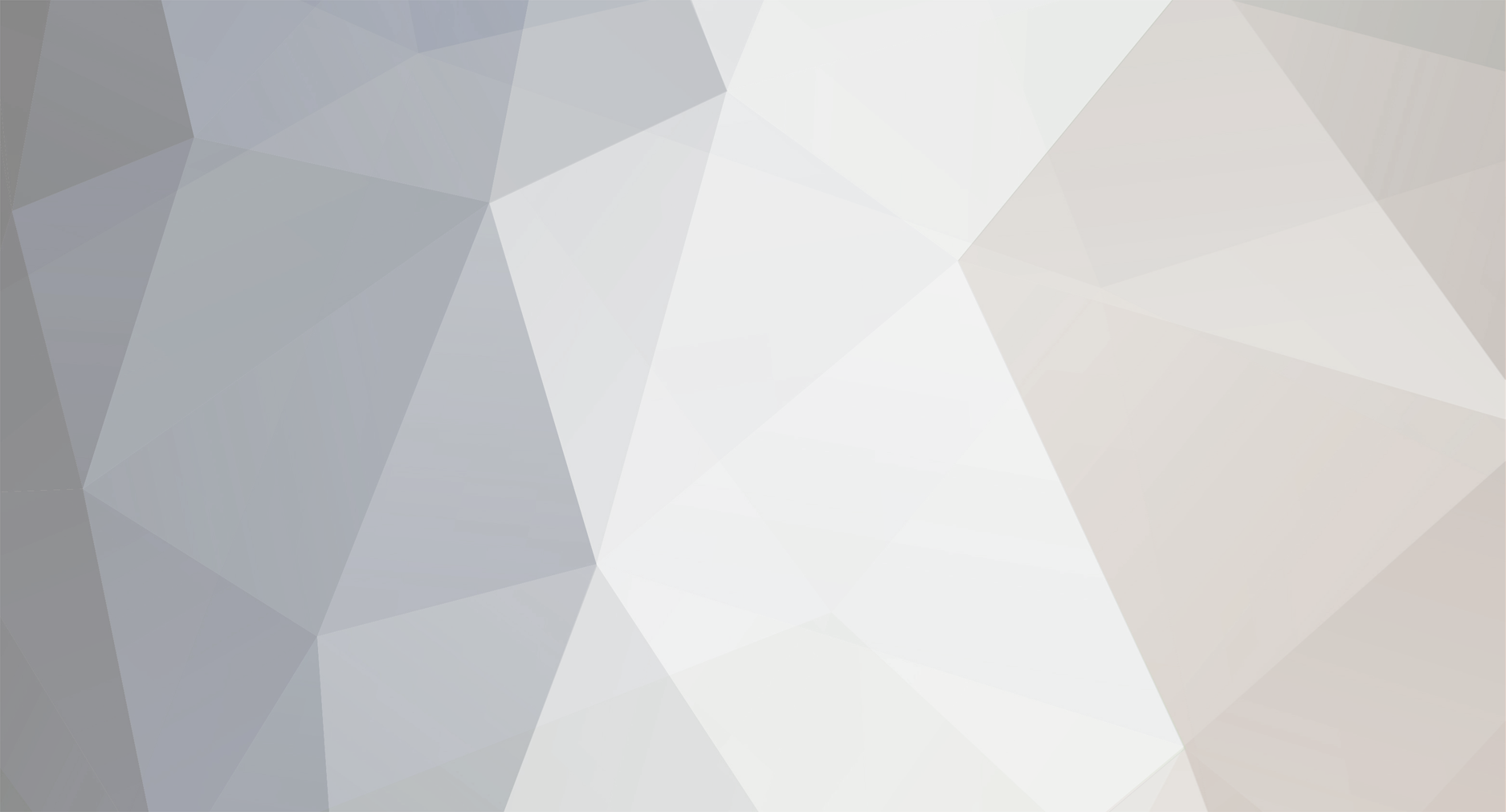
premiso
Members-
Posts
6,951 -
Joined
-
Last visited
-
Days Won
2
Everything posted by premiso
-
You will want to use is_null to test if the value is null, that is for PHP. For the SQL check you can easily do: SELECT * FROM table_name WHERE value IS NULL Or SELECT * FROM table_name WHERE value IS NOT Null If that does not answer your question, let me know. Example of the php code: <?php // mysql connect info: $res = mysql_query("SELECT * FROM table_name"); while ($row = mysql_fetch_assoc($res)) { if (is_null($row['field_name'])) { echo "It is null!"; } } ?>
-
TIME_FORMAT(TIMEDIFF(`period_length`, `clock`), '%k:%i') AS timepassed Should work. No need to do a "timepassed2" as you can just format it like above.
-
ini_set("display_errors", 0); Set the display_errors to off and it will stop errors from displaying.
-
One way to do this, is add another column "urlname" in the database. And in the urlname make it a unique key and change any special characters to something manageable, like spaces to _ or - etc. This way you do not have to worry about encoding etc and it should work. Not sure if you were looking for an alternative, but yea. That is what I would do
-
Ok, first up, your $timepassed should only return a Resource, if the query executed successfully. Second up, you are cacluclating the TIMEDIFF if a string value (the single quotes). Third, why not just calculate the timediff in the original query? <?php $sql = "SELECT period, team, goal, assist1, assist2, type, TIMEDIFF(`period_length`, `clock`) AS timepassed FROM scoresheet WHERE period='1'"; $rs = mysql_query($sql,$dbc) or trigger_error("Query Failed: " . mysql_error()); $matches = 0; while ($row = mysql_fetch_assoc($rs)) { $matches++; echo "{$row['period'}] {$row['timepassed']} {$row['team']} {$row['goal']} ({$row['assist1']} {$row['assist2']}) {$row['type']}<br />"; } echo ""; ?> Give that a try, notice that I encapsulated the $row items in the echo with { and } and I added the single quotes for the associative indexes, this will cause less notice errors from PHP as just doing $row[index] causes a notice of undefined constant where as $row['index'] will not since that is how it should be done.
-
mysql_query($query, $conn) or die ('Error: Der er opstået en database fejl, og det var derfor ikke muligt at gennemføre aktiveringen<br />MySQL Error:' . mysql_error() ); // this is the error I get Replace that code as it will provide you with the error MySQL is returning.
-
Question 1: I believe that is the general idea, google just uses regex to find that and use it if it is apparent in the string. I do think that they only apply those if the site: is at index 0 of the string. Question 2: Searching multiple tables is actually not too bad, the issue you will have more or less is having to setup the search terms for each field that can be searched. Depending on your table structure and the references for the table searches you may be able to do it in 1 query (if they all link to each other). If not I think you would be better off doing separate queries for each table. Then when displaying you can display it in "categories" as a sense, IE: Players Found; Teams Found; Venues Found; etc. I would just set it up to search 3 different queries, but I would also make check boxes on the search form so they can actually choose what they want to search. Let me know if you want anymore information
-
You can use md5 to generate a unique hash using the file itself or file location. Then just store that hash as to accessing the file.
-
That code won't work because of the single quotes the { } will only be interepted inside of double quotes: echo "<td><center><input type='hidden' name='hidden_id' value='{$row['id']}' >"; Would be the proper way.
-
But to answer your first question about 1,1,9 doing a var_dump on $A and $B you will see it is now a boolean, so basically they are being assigned the value of true, since $A = 1 was set is what I imagine and since it was a AND. I am not 100% sure why, but I am sure it is how assignments are done with the AND keyword, because all 3 statements must be true. The second part, is the same deal but this one is using OR and since the assignment is generally always done and is true, it sets the first variable to true and the rest do not get executed cause it is an OR statement and exits at the first true condition. I used the following code for testing, to come to these results: $A = 12; $B = 53; $C = 66; if($A = 3 || $B = 6 || $C = 9){ var_dump($A); var_dump($B); var_dump($C); echo "$A,$B,$C<br>"; } $A = 12; $B = 53; $C = 66; if($A = 3 && $B = 6 && $C = 9){ var_dump($A); var_dump($B); var_dump($C); echo "$A,$B,$C<br>"; } $A = "one"; $B = "two"; $C = "three"; if($A = 3 || $B = 6 || $C = 9){ var_dump($A); var_dump($B); var_dump($C); echo "$A,$B,$C<br>"; } //echo "$A,$B,$C<br />"; $A = "one"; $B = "two"; $C = "three"; if($A = 3 && $B = 6 && $C = 9){ var_dump($A); var_dump($B); var_dump($C); echo "$A,$B,$C<br>"; } echo "<br /><b>Lowercase Var Names</b><br/>"; $a = 12; $b = 53; $c = 66; if($a = 3 || $b = 6 || $c = 9){ var_dump($a); var_dump($b); var_dump($c); echo "$a,$b,$c<br>"; } //echo "$a,$b,$c<br />"; $a = 12; $b = 53; $c = 66; if($a = 3 && $b = 6 && $c = 9){ var_dump($a); var_dump($b); var_dump($c); echo "$a,$b,$c<br>"; } $a = "one"; $b = "two"; $c = "three"; if($a = 3 || $b = 6 || $c = 9){ var_dump($a); var_dump($b); var_dump($c); echo "$a,$b,$c<br>"; } //echo "$a,$b,$c<br />"; $a = "one"; $b = "two"; $c = "three"; if($a = 3 && $b = 6 && $c = 9){ var_dump($a); var_dump($b); var_dump($c); echo "$a,$b,$c<br>"; } But yea, I am not sure what is really up, as $A should be 3 by all logic...but yea, that was my findings, perhaps someone knows why, as I do not fully know why just a glimpse in my mind =\
-
$del = 'DELETE FROM recipes WHERE id="$id"'; That is invalid SQL, in MySQL you must use the single quotes around data. $del = "DELETE FROM recipes WHERE id='$id'"; Try that and see if it works.
-
You will want to look into CRON jobs for Linux or Schedule Tasks for Windows, and basically use those to call a php script that fetches the XML feed and save it to your server every 1 minute. Look into that for your answer.
-
As far as I was taught, a margin is the space around an object. Think of it link in Word when creating a document, you have a margin border around the whole document, same deal. So when you say margin-top, it spaces the item that far from the top margin, etc etc. That is the best way I know how to explain it. A way to see this, is the following code: <div style="position: absolute; left: 50px; margin-top: 200px; width: 200px; height: 100px; border: 1px dotted #FF6600; color: #FF0000;"> TEST </div> <div style="position: absolute; left: 50px; margin-top: 205px; width: 200px; height: 100px; border: 1px dotted #FF6600; color: #FF0000;"> TEST </div> You will see that the second div will now be on top of the first just 5 pixels below it. Hopefully that clears it up a bit.
-
Well I would suggest reading this: http://css-tricks.com/absolute-relative-fixed-positioining-how-do-they-differ/ He wrote a pretty good article on that and the difference, chances are what you think it means is completely different then what it actually means.
-
What browser version are you using? That is very important, try that code in Firefox and see if you get the same results. If you are using IE6, well that is why. IE6 is just fubar when it comes to CSS and you have to code it a certain way and that often break Firefox, so yea. It is an endless loop of sucks and you have to hack the style sheets or create multiples. But depending on what browser you are using to test, their could be several answers.
-
I am pretty sure the only way this could be done is using the site that Dee posted above. It is very complicated and cannot be done without SSH access to the server, but yea. If there was a simpler way, I am sure that the tutorial above would not have been written. For more alternatives there is another thread in another forum already about this which you can find here: http://www.zubrag.com/forum/index.php?topic=55.0
-
auto_increment is a basic part of rational databases. What you want is how a database should be setup, see the example linked for more information.
-
Well you also do not have an HD card as Cags does, the HDMI cable and HD card increases the quality tremendously vs the old cards and what they can push out to a monitor.
-
You want to look into mysql_real_escape_string onto data going into the database will do what you want and you do not have to worry about changing anything or modifying data when pulling it out.
-
It is possible that your IE is set to only keep cookies for the browser session. Check your IE settings and make sure that it does not clear out cookies on browser close etc. As it works in FF and Chrome the problem probably lies within the IE browser settings.
-
Do you have PHP on the server? If so you can link all the CSS to a PHP script and use header's / ( file_get_contents or cURL) to grab the css from the remote site and output that CSS. If you do not have PHP on the server, I hardly doubt that without some form of a server side scripting language this would be possible (unless you use server side includes SSI).
-
Without more code it is hard to say. Chances are your query is not pulling all the records you think it should be? Have you tried doing a mysql_num_rows check to make sure that there are 7 rows being returned?
-
Why not try it out? A comment on the imagegif php manual: http://www.php.net/manual/en/function.imagegif.php#67933 And: http://us3.php.net/manual/en/function.imagegif.php#54419 You may have to install ImageMagick, not sure.
-
It will help for anyone who looks at this that knows or has OSCommerce. I moved the topic to the proper section (Third Party). I would suggest going onto OSCommerce forums and seeing if they will help you. I have not used OSCommerce in a long time, and taken that the code was modified I do not know what or why it is not working. I would take it up with the people who modified it.
-
Where did this script come from? I take it you did not write it. Is it a 3rd party script or did you have it custom built? If custom built ask the coder, if third party, what is the script you are using and I will move this post to the proper section so someone familiar with that script may be able to help you.