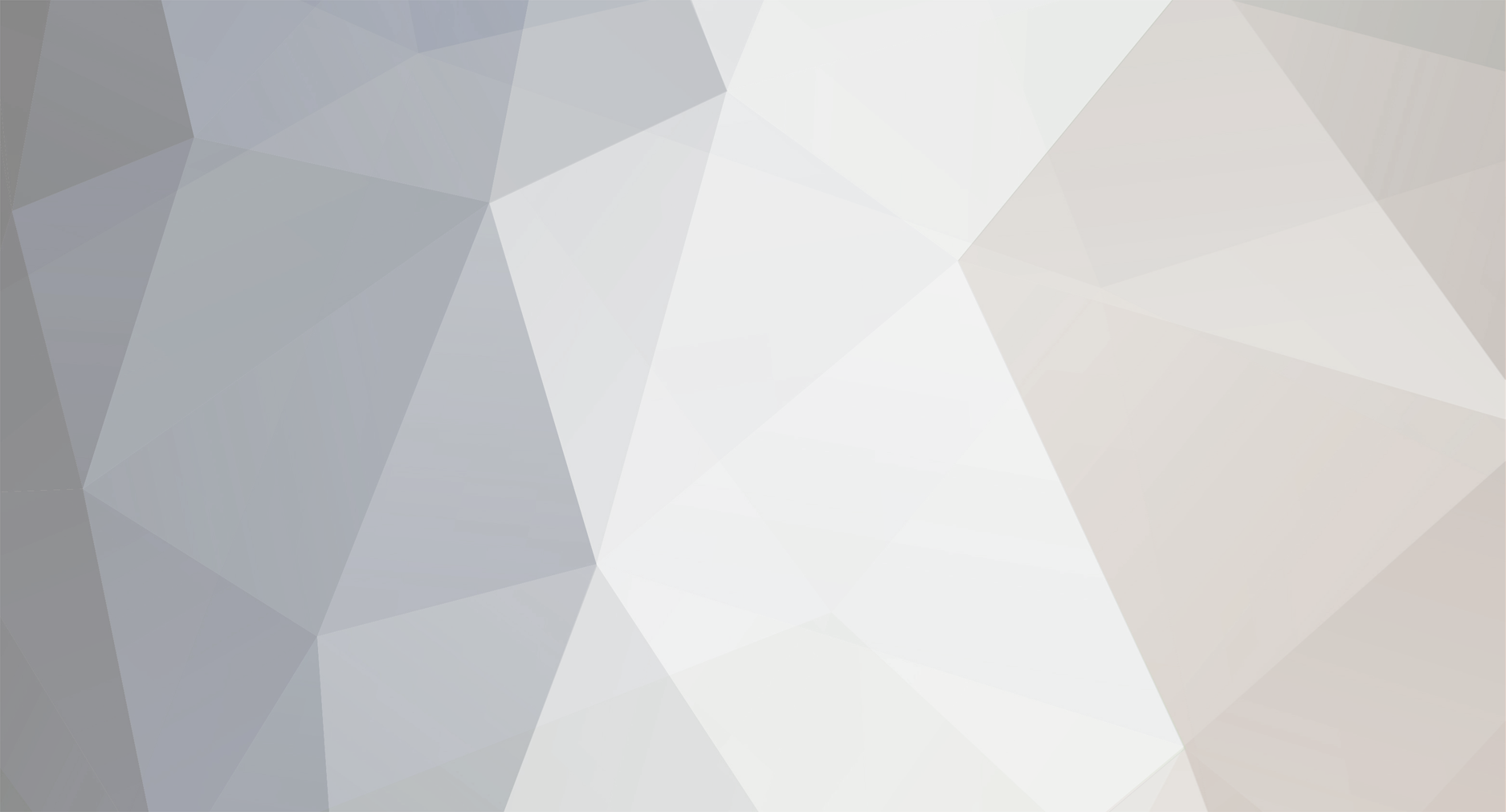
iarp
Members-
Posts
326 -
Joined
-
Last visited
Everything posted by iarp
-
PHP itself has a function called session_id() that returns a string, use that during the INSERT and UPDATE statements. It will always be unique for every session that is running.
-
Add a new column to the users table, call it whatever you want maybe TotalLogins, and within whatever script you use to login the user add a mysql statement UPDATE USERS SET TotalLogins = TotalLogins + 1 WHERE id = <the user id> That way you would know just how many times they have logged in. For tracking session length you'll most likely need another table. It'll need to track Users ID, Session ID, Login datetime, Last Activity datetime. When the user logs in, INSERT into this new table using the users id from login, session_id(), now(), now(). Then on every page you need an update statement that says something like UPDATE UsersSessionTracker SET last_activity = NOW() WHERE users_id = <the users id> AND session_id = <session_id() from php> Of course you'll need check to ensure the row exists and what to do if it doesn't...etc. This isn't foolproof, it bases the session length on the last page load that they did from your website. If they site on that page for 15 minutes and then leave, you wouldn't know that they sat there for 15 minutes on a single page. For that type of tracking you would need Javascript that sends calls back to the server every x minutes to keep track..etc.
-
Javascript and PHP are two completely different beasts. You'll need to find out what is triggering the javascript to pop-up, or show where you think the php in the parent element is causing it to appear.
-
You most likely need to turn on display_errors within php.ini to see what message you are getting, or check the server logs.
-
Is there any reason for the need to modify the cookies? I would try commenting out the session_set_cookie_params and seeing if anything changes.
-
Any error messages displayed? What do the server logs say? Unless this is a development server, only change this temporarily. Open php.ini and look for display_errors = and make sure it = On and restart the web service. If you're using apache you'll also want to have a look at /var/logs/apache2/error.log it maybe logging to another location all depends on the web server itself.
-
Where is the code for the receiving end? Removing it from the display will not remove its requirement to be filled out. We need to see register.php to tell you what code needs to be removed.
-
To strictly use PHP you could wrap the <select> in a <form>, add a Submit button and then use the value of the <option> selected to run your SELECT statement against. <form method="post"> <select name="username"> <option value="ScarfaceJM">ScarfaceJM</option> <option value="iarp">iarp</option> </select> <input type="submit" name="submit" value="Submit" /> </form> The selected username would then be available only after submission by: $username = $_GET['username']; The other way would be via jQuery or javascript in some form. That would allow you to stay on the page and load data in the background. <script type="text/javascript"> $(document).ready(function(){ $('select[name="username"]').on('click', function() { // do something ajaxy like and load it from another source maybe? console.log('i am in here'); }); }); </script>
-
Why can I not return a GPIO's status from within a PHP 'if' statement?
iarp replied to MeltingDog's topic in PHP Coding Help
Are you sure you're adding ?trigger=1 to the url? http://localhost/index.php?trigger=1 You may also need to use == '1' instead of just a plain 1, PHP is weird sometimes with comparisons.- 1 reply
-
- gpio
- raspberrypi
-
(and 1 more)
Tagged with:
-
How to retrieve php textbox value to be used with mysql
iarp replied to suneetp's topic in PHP Coding Help
You can access that <inputs> value by either $_GET['seltext'] or $_POST['seltext'] . I would not use $_REQUEST. The other option would be to do away with the scripting, give the <select> a name="" and then access that via $_GET or $_POST -
How to retrieve php textbox value to be used with mysql
iarp replied to suneetp's topic in PHP Coding Help
Your <select> is missing a name="". All elements on a form require a name="" if you wish to use the data when they hit submit. As well I do not see the text field that you're talking about in your code. -
From http://codex.wordpress.org/Function_Reference/get_post_meta you'll see that you're passing true to the third parameter which states: Without a wordpress to test on, i would loop over whatever is returned when that third parameter is set to false and see what is returned. I would expect the boxes you've checked to be returned. However if everything was returned, i would expect some kind of flag that lets you know it was checked.
- 3 replies
-
- checkbox
- checkboxex
-
(and 2 more)
Tagged with:
-
Do you have display_errors On ? If not, have you looked at the web server error logs? How long is max_execution_time? What about sufficient filesize allowed via upload_max_size, post_max_size in php.ini ? If it takes too long to upload, it'll time out. If it's bigger than the server allows it'll only stop once that limit has been reached. Your SQL query, what happens if $row is empty because the user_id wasn't found? That'll throw an error. What happens if the filename with no extension isn't located in the path you're specifying and then you try to copy it? Nothing about what you've posted is actual thumbnail generation, it's just a copy script without any checks to ensure the file it's trying to copy actually exists, or that the user exists. What happens if the output file already exists, you'll be overwriting someone elses thumbnail with another users. if (!empty($row) { $input_file = .... $output_file = .... if (!file_exists($output_file)) { if (file_exists($input_file)) { copy($input_file, $output_file); } else { echo 'input file already exists'; } } else { echo 'output file already exists'; } } else { echo 'no user found'; }
-
That's because $status is an array containing the rows that were returned by the query. Do a print_r($status) and you'll see what it contains. Looking at your code i would imagine it'll contain an array of arrays, but it's possible it could just contain the single rows data. echo $status[0]['status']; # or echo $status['status']; BTW you cannot trust num_rows to always return the number of rows it returned, it returns the number of affected rows by the query you issued, if you wanted to be safe you'd need to issue a COUNT(*) statement to get a proper count. Or you could do a COUNT($status) or COUNT($res). The only reason I cannot nail down what to expect from your query is you have some strange things going on, I'm not sure what the purpose of doing this is $res = $stmt->get_result(); $status = $res->fetch_assoc(); $res->num_rows; You should be able to just do (below) and be done with it, then you could issue COUNT($rows) and know how many were returned. $rows = $stmt->fetchAll(); if (count($rows) > 0) { foreach ($rows as $row) { .... Do whatever you want in here foreach row returned } # or $status = $row[0]; if($status['status'] == 0) { echo 'banned'; } else { echo 'not banned'; } }
-
You seem to know the functions you already require. setcookie('mycookie', 'random_image.jpg', time() + 3600); if (!empty($_COOKE['mycookie']) { echo "$_COOKIE['mycookie'] has the value of " . $_COOKIE['mycookie']; }
-
On your download page check for the $_SERVER['HTTP_REFERER'] and see if it matches your site and the deleted documents page name, otherwise redirect them. The referer is spoofable though, so anyone that is smart enough to try it could potentially download the file from elsewhere. Another idea that came to mind, generate random ids in the users session data that maps to the real id, regenerate those ID's on every page refresh. That way they would need the latest ID's, the modified referer and could only grab it once since the other ID's would be regenerated again. This could cause headaches for legitimate people.
-
I had originally just been require_once on all of the required files within my config file which is the first point of entry into the application. I had not been using an autoloader until you mentioned it. I remember that phpmailer uses one so I copied and modified what they used and the result was: <?php function DTBAutoload($class) { $filename = dirname(__FILE__) . DIRECTORY_SEPARATOR . str_replace('\\', DIRECTORY_SEPARATOR, $class) . '.php'; #echo $filename . '<br />'; if (is_readable($filename)) require $filename; } if (version_compare(PHP_VERSION, '5.1.2', '>=')) { if (version_compare(PHP_VERSION, '5.3.0', '>=')) { spl_autoload_register('DTBAutoload', true, true); } else { spl_autoload_register('DTBAutoload'); } } else { /** * Fall back to traditional autoload for old PHP versions * @param string $classname The name of the class to load */ function __autoload($classname) { DTBAutoload($classname); } } It required a bit of file and folder renaming to get it correct, plus separating each of the classes into their own separate files. Afterwards the \DTB\ namespace started working everywhere.
-
I'm not entirely sure what is wrong with me, but I'm having a super difficult time understanding namespaces. I have 2 primary classes # Resides within class.DataTypeBase.php namespace DTB\Base; class DataTypeBase { protected $name = ''; protected function getName() { return $this->name; } protected function setName($name) { $this->name = $name; } } # Resides within class.DataType.php namespace DTB; class DataType extends DataTypeBase { public function process_data(){ .... } } I then have a third file that contains approximately 9 different classes that further extend DataType # Resides within class.DataTypes.php namespace DataTypes; class Silver extends DataType { public function differ($type) { .... } } The namespace system seems to work for class DataTypes, but with the class Silver I get an error message saying that the class DataType does not exist. From what I read online, and using the example above I figured the "extends DataType" may have to be "extends \DTB\DataType" but it results in the same error message. I'm not entirely sure what I need to do for this to work properly
-
Changing Content-Type header causes two database updates instead of one.
iarp replied to iarp's topic in PHP Coding Help
Here is a comparison of the only differences between the two $_SERVER arrays. Array ( [HTTP_ACCEPT] => text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8 [HTTP_ACCEPT_ENCODING] => gzip,deflate,sdch ) Array ( [HTTP_ACCEPT] => */* [HTTP_ACCEPT_ENCODING] => identity;q=1, *;q=0 [HTTP_REFERER] => https://mydomain/linker/l/123 [HTTP_RANGE] => bytes=0- ) All I care about is counting whenever the media itself has been delivered. So watching for the HTTP_RANGE I think should work just fine. -
Changing Content-Type header causes two database updates instead of one.
iarp replied to iarp's topic in PHP Coding Help
Oh wow. OK so to clear up some confusion, the query I use has 2 other tables joined in. For simplicity sakes i removed the joins and just wrote out the query to use the media table, the FROM is in my query as it should be. Google Chrome, the first time you ever visit a website that has not been cached chrome sends out two requests at the same time. I have seen two to three other explanations as to why this occurs namely: It sends one request for the favicon to save into cache, and the other request to get the actual page data It sends two simultaneous connections, if one dies and the other works it just seamlessly flips to the working connection. What I am serving is not typically a page, but rather media. Chrome sends one connection requesting a page and once it figures out its media it sends another requesting the media itself. To fix this I have had to wrap the UPDATE statements in an if statement checking for an existing and non-empty $_SERVER['HTTP_RANGE']. I saved the $_SERVER array information for both requests and compared the data. One request was sent for the media, the other for page data. I found that whenever HTTP_REFERER existed that it always asked for page data and when HTTP_RANGE existed that it was looking for the media itself. -
Changing Content-Type header causes two database updates instead of one.
iarp replied to iarp's topic in PHP Coding Help
Doing a tad more research. Turns out it's Chrome sending two separate requests. I've checked $_GET, $_POST, $_SERVER to determine if there is anyway I can stop the update from occurring on this secondary request and everything is identical -
<?php require_once('init.php'); $results = $db->prepare('SELECT file_location, file_type, file_size, id as media_id WHERE id = ? LIMIT 1;'); $results->execute(array($_GET['id'])); while ($row = $results->fetch()) { header('Content-Type: ' . $row['file_type']); header('Content-Length: ' . $row['file_size']); $media_ins = $db->prepare('UPDATE media SET total_clicks = total_clicks + 1 WHERE id = ?'); $media_ins->execute(array($row['id'])); readfile($row['file_location']); } For some reason, putting the header('Content-Type....') causes the UPDATE statement to trigger twice increasing the total_clicks by 2. Commenting out the content-type line causes a single update to occur. init.php contains nothing more than session_start and the database connection. When i comment-out the content-type line the page is blank with no errors or messages. I'm not sure why this is occurring.
-
Parse error: syntax error, unexpected 'echo' (T_ECHO)1
iarp replied to FmanTheCoder's topic in PHP Coding Help
You're missing a closing bracket at the end of the INSERT statement line. I see one opening at the if and another at the mysql_query but only 1 closing. Plus you've spent INSERT wrong. -
Storing and deleting data used to authenticate
iarp replied to alphamoment's topic in PHP Coding Help
You need to create another php script that a cronjob of some type that runs the command "delete from DocumentorTable where date_created >= DATE_SUB(NOW(), INTERVAL 6 HOUR)" -
Storing and deleting data used to authenticate
iarp replied to alphamoment's topic in PHP Coding Help
You already have the logic and code written to do what you want in the second code block. The following isn't actual code, but what you have already written can very easily be turned into this SELECT my,fields,here FROM DocumentorTable WHERE email = ... count = mysql_num_rows.. if($count >= 1) { email already exists, echo error } else { INSERT INTO DocumentorTable (..) values (...) echo success }