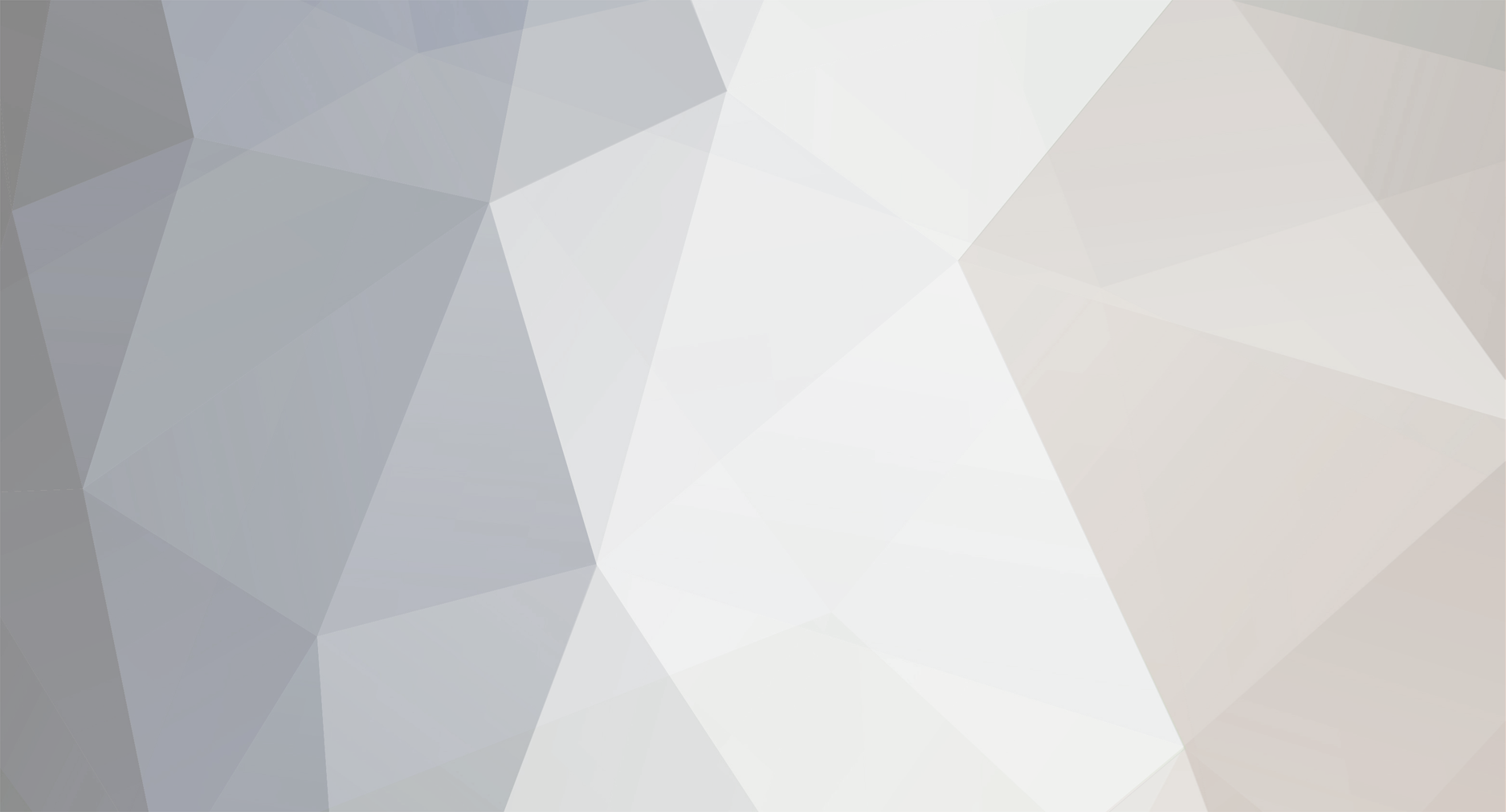
hansford
Members-
Posts
562 -
Joined
-
Last visited
-
Days Won
4
Everything posted by hansford
-
Your JSON object is not correct. Should look like this. data : {action: 'visitaValidacaoByUserAdmin', id_visita: 'id_visita', plano: 'plano', valido: 'valido'} You can only set GET or POST vars and send them to PHP, therefore if you need to call a certain function, just check the POST value in PHP. if (isset($_POST['action'])) { // call your function $_POST['action'](); } I personally wouldn't do it this way, rather get the action and put it in a switch statement which calls the appropriate function. switch($_POST['action']) { }
-
Issue one query to get all the information you need based on that username. If username already exists, issue that error and nothing more. If the username passes but the email matches, issue the email error etc.
-
The loop you wrote is an infinite or endless loop because the loop will continue indefinitely as the conditional value never changes. Add some way for $red to change so the condition will eventually equate to false and the loop will terminate. <?php error_reporting(E_ALL); ini_set('display_errors',1); $red = "exciting"; $feelings = array( 'exciting', 'exciting', 'boring', 'exciting' ); // use do-while loop when you want the code to // run at least 1 time prior to checking the condition do { // run statements echo "<p>It's $red to be red.</p>"; // get value of $red $red = $feelings[rand(0,3)]; // check condition } while ($red == 'exciting');
-
Sure. Put the script which dynamically generates the banner in a div and just hide the div after it's clicked.
-
I thought you needed the sum of the 2 cards as well. How does this accomplish that task. Ok, Ch0cu3r has already solved this for you. Moving on.
-
You have 2 options to accomplish this. Using plain JavaScript or with jQuery (or other library). I'll post the JavaScript way. <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Insert title here</title> </head> <body> <a id="banner" href="http://www.purple.com" target="_blank"><img src="banner.jpg" width="400" height="150"></a> <script type="text/javascript"> window.onload = function() { var el = document.getElementById('banner'); el.addEventListener("click",function() { this.style.display = "none"; }); }; </script> </body> </html>
-
I just set it up on the same page for illustration purposes. <?php error_reporting(E_ALL); ini_set('display_errors',1); if (isset($_POST['direction']) && !empty($_POST['temp'])) { $direction = $_POST['direction']; $temp = $_POST['temp']; if ( ! is_numeric($temp)) { $error[] = "Input is not a valid number."; } if ( ! isset($error)) { if ($direction == "ftc") { $temp_new = convert_temp($temp); echo "$temp degrees Fahrentheit is <b>$temp_new</b> degrees Celsius " ; } else { $temp_new = convert_temp($temp,true); echo "$temp degrees Celcius is <b>$temp_new</b> degrees Fahrentheit " ; } } else { foreach ($error as $err) { echo $err . '<br />'; } } } function convert_temp($temp, $celcius=false) { if ($celcius) { $ret = ($temp * 9/5) + 32; } else { $ret = ($temp - 32) * 5/9; } return round($ret,1); } ?> <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>convert</title> </head> <body> <form action="" method="post"> <select name="direction"> <option value="ftc" selected>Fahrenheit to Celsius </option> <option value="ctf">Celsius to Fahrenheit </option> </select> <input type="text" name="temp" size="6"/> <br/> <input type="Submit" value="Convert"/> </form> </body> </html>
-
Depending what "note" holds and it's size you may need to create another table for that one and just just link to it using a foreign key. CREATE TABLE IF NOT EXISTS Person( id int unsigned NOT NULL AUTO_INCREMENT, firstname varchar(150), lastname varchar(250), address varchar(250), phone varchar(100), email varchar(150), note varchar(250), PRIMARY KEY(id)) INSERT INTO Person(firstname,lastname,address,phone,email,note) VALUES('jon','lastname','address','phone','email','note'), ('bob','lastname','address','phone','email','note'), ('jess','lastname','address','phone','email','note'), ('cat','lastname','address','phone','email','note')
-
I don't know what you want either, but just for fun. <?php error_reporting(E_ALL); ini_set('display_errors',1); $cards = array( "Red" => 1, "Blue" => 2, "Green" => 3, "Yellow" => 4, "Black" => 5, "White" => 6, "Aqua" => 7, "Fuchsia" => 8, "Gray" => 9, "Lime" => 10, "Maroon" => 11, "Navy" => 12, "Olive" => 13, "Purple" => 14, "Silver" => 15, "Teal" => 16, ); $array2 = shuffle_array($cards); $keys = array_keys($array2); $vals = array_values($array2); $i = 0; foreach ($cards as $key => $value) { $sum = $value + $vals[$i]; echo "$key => $value + {$keys[$i]} => {$vals[$i++]} = $sum<br />"; } function shuffle_array( $arr ) { $keys = array_keys($arr); shuffle($keys); $values = array_values($arr); $arg = array(); foreach($keys as $key) { $arg[$key] = $arr[$key]; } return $arg; }
-
help with php htmlspecialchars function on post data
hansford replied to jvanasco's topic in PHP Coding Help
You need to set up a a local testing ground for such things. Use Wamp, Xampp or another full stack application. Get it working locally and then upload to your server. -
I don't know what you are trying to do, but PHP and JavaScript are two completely different languages and one has no knowledge that the other even exists. You can pass data to PHP through form fields, hidden and others or through ajax.
-
I wish all questions were like this - oh yeah and I got to them first
-
I would think that big ugly reserved word "function" would be enough to make them stand out. function sore_thumb( $bandaid ) { }
-
I'm pretty much with the other folks on this one. I use Eclipse as my IDE and I use 4 spaces for tabs indent. Try to avoid the hidden \t and other unwanted chars.
-
HTML select and option work with PHP database
hansford replied to sigmahokies's topic in PHP Coding Help
Let's try it out an example to see how it works. <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>whatever title</title> </head> <body> <form action="" method="get"> <select name="month"> <option value="1" selected>January</option> <option value="2">February</option> <option value="3">March</option> <option value="4">April</option> <option value="5">May</option> <option value="6">June</option> <option value="7">July</option> <option value="8">August</option> <option value="9">September</option> <option value="10">October</option> <option value="11">November</option> <option value="12">December</option> </select><br /> <input type="submit" value="submit"> </form> <?php error_reporting(E_ALL); ini_set('display_errors',1); define('br','<br />'); $sql = "CREATE TABLE IF NOT EXISTS Person( id int UNSIGNED NOT NULL PRIMARY KEY AUTO_INCREMENT, firstname varchar(100), lastname varchar(100), dateofbirth DATETIME )"; if (isset($_GET['month'])) { // grab the month from query string $month = (int)$_GET['month']; // database credentials $host = 'localhost'; $user = 'db_user_name'; $password = 'db_password'; $database = 'db_name'; // create our database connection $db = new mysqli($host, $user, $password, $database); $db->set_charset('utf8'); if($db->connect_errno) { echo $db->connect_error . br; exit; } if(($db->query('DROP TABLE IF EXISTS Person')) === false) { echo $db->error . br; exit; } if(($db->query($sql)) === false) { echo $db->error . br; exit; } date_default_timezone_set('UTC'); $date1 = date('Y-m-d', strtotime('1969-09-04')); $date2 = date('Y-m-d',strtotime('1946-02-13')); $date3 = date('Y-m-d',strtotime('1984-07-10')); $sql = "INSERT INTO Person(firstname, lastname, dateofbirth) VALUES('Paul','Ryan','$date1'),('Jessica','Sims','$date2'),('Gary','Kasper','$date3')"; if(($db->query($sql)) === false) { echo $db->error . br; exit; } $sql = "SELECT firstname,lastname,dateofbirth FROM Person WHERE MONTH(dateofbirth) = ?"; $stmt = $db->prepare($sql); $stmt->bind_param('i',$month); $stmt->execute(); ?> <div style="display:block;padding:15px;"> <?php $result = $stmt->get_result(); if($row = $result->fetch_array(MYSQLI_ASSOC)) { $birthday = date('F d, Y', strtotime($row['dateofbirth'])); echo "{$row['firstname']} {$row['lastname']} was born on {$birthday}" . br; } else { echo "No one in database was born in that month."; } } ?> </div> </body> </html> -
Other thoughts: I know it probably doesn't apply to this assignment, but this wouldn't fly in the real world. If there is any chance that two users would be accessing the file at the same time, you can experience data loss. Only way around this is by using a database or applying exclusive file locks which would prevent 2 people from being able to write to the file at the same time. Problem there is...one person will be shut out whereas with a database they would simply be standing in line (queue).
-
Here is a csv option if you want to look at that. We aren't writing the year multiple times in either script as there is no need. We write the year one time and then just update the number of times it was accessed. We don't need to use a temp file here, but it's good practice to get in the habit as some files can be massive in size and you could exhaust your memory attempting to read the file all at once into a buffer. if ($BirthYear == '2019' || $BirthYear == '2007' || $BirthYear == '1995' || $BirthYear == '1983' || $BirthYear == '1971') { $CountFile = 'statistics/count.csv'; $count = 1; if (file_exists($CountFile)) { if(($handle = fopen($CountFile, 'r+')) === false) { exit; } //chmod($CountFile, 0777); $count = updateFile($handle, $BirthYear); fclose($handle); } else { if(mkdir("statistics") === false) { exit; } if(($handle = fopen($CountFile, 'w')) === false) { exit; } //chmod($CountFile, 0777); fputcsv($handle, array($BirthYear, 1)); fclose($handle); } echo "<p>You were born under the sign of the <img src='Images/pig-zodiac.jpg' alt=''/></p>\n"; echo "You are person $count to enter $BirthYear"; } else { echo "Your birth year is apparently not that important"; } /** * read cvs file and attempts to find matching year * if match found, number is updated * file is rewritten from temp file * @param file pointer $fp * @param string $year * @return number */ function updateFile($fp, $year) { // counter, default is 1 $num = 1; // flag if match was found $found = false; // open temp file for reading/writing and truncate to zero length $temp_path = 'statistics/temp.csv'; if(($tp = fopen($temp_path, 'w+')) === false) { exit; } // read each line of csv file while (($data = fgetcsv($fp, 1000, ',')) !== false) { // attempt to match year if (trim($data[0]) == $year) { // extract number and increment by 1 $num = (int) trim($data[1]); $num++; // add to temp file fputcsv($tp, array($data[0],$num)); $found = true; } else { // add to temp file fputcsv($tp, $data); } } // no match found, add new year to the temp file if ( ! $found) { fputcsv($tp, array($year,1)); } // place the file pointers at the beginning of each file rewind($fp); rewind($tp); // read line from temp file and then write to main file while(($data = fgetcsv($tp,1000,',')) !== false) { fputcsv($fp, $data); } // close temp file fclose($tp); // return the number of times year was accessed return $num; }
-
The more sensible approach to this would be use a csv file. But it can still be accomplished using a plain text file. if ($BirthYear == '2019' || $BirthYear == '2007' || $BirthYear == '1995' || $BirthYear == '1983' || $BirthYear == '1971') { $CountFile = 'statistics/count.txt'; $count = 1; if (file_exists($CountFile)) { $handle = fopen($CountFile, 'r+'); //chmod($CountFile, 0777); $count = updateFile($handle, $BirthYear); fclose($handle); } else { mkdir("statistics"); $handle = fopen($CountFile, 'w'); //chmod($CountFile, 0777); fwrite($handle, $BirthYear . ':1' . PHP_EOL); fclose($handle); } echo "<p>You were born under the sign of the <img src='Images/pig-zodiac.jpg' alt=''/></p>\n"; echo "You are person $count to enter $BirthYear"; } else { echo "Your birth year is apparently not that important"; } /** * reads each file line and attempts to find matching year * if match found, number is updated * file is rewritten from buffer * line format - year:number example; 1995:2 * @param file pointer $fp * @param string $year * @return number */ function updateFile($fp, $year) { // string buffer to rewrite file $buffer = ""; // counter, default is 1 $num = 1; // flag if match was found $found = false; // read each line of file while (($line = fgets($fp)) !== false) { // place line parts in array $args = explode(':', $line); // attempt to match year if ($args[0] == $year) { // extract number and increment by 1 $num = (int) trim($args[1]); $num++; // add update to buffer $buffer.= $args[0] . ':' . $num . PHP_EOL; $found = true; } else { $buffer .= $line; } } // no match found, so add new year to the file if ( ! $found) { $buffer .= $year . ':1' . PHP_EOL; } // place the file pointer at the beginning of the file rewind($fp); // rewrite the file fwrite($fp, $buffer); // return the number of times year was accessed return $num; }
-
Here is the deal. Our goal here is to help you become a better programmer because we've all been in the same position you are now. You made an attempt and posted your code, so I attempted to help you in hopes that you would understand the logic and be able to apply it in similar coding problems. So, make an attempt at how you think you could solve the problem of keeping track of the number of times a year was entered. It may help to reread the assignment and even post it on here. Does the instructor want it stored in the same file as the year or another file ?
-
You'd be surprised how many people applying for programming jobs cannot write the Fizz Buzz code. People get their feet wet in PHP or some other "seemingly friendly" language and suddenly they are confident enough to go out and start applying for professional developer positions. If it were really that easy, we would all be earning minimum wage and having to bag burgers and fries in between designing, coding, testing and debugging.
-
Not sure why you are changing the permissions on the file. If your script creates the file, it has the permissions to write to it. I did a little rewriting of your script to try and make sense of it. if ($BirthYear == '2019' || $BirthYear == '2007' || $BirthYear == '1995' || $BirthYear == '1983' || $BirthYear == '1971') { $CountFile = 'statistics/count.txt'; if (file_exists($CountFile)) { echo"file accepted"; $handle = fopen($CountFile, 'a'); //chmod($CountFile, 0777); fwrite($handle, $BirthYear. PHP_EOL); fclose($handle); } else { mkdir("statistics"); $handle = fopen($CountFile, 'w'); //chmod($CountFile, 0777); fwrite($handle, $BirthYear. PHP_EOL); fclose($handle); } echo "<p>You were born under the sign of the <img src='Images/pig-zodiac.jpg' alt=''/></p>\n"; $readin = file($CountFile); $count = 0; foreach ($readin as $year) { // need to trim the newline/carriage return // or year will never match if (trim($year) == $BirthYear) { //echo $year. $BirthYear; $count++; } } echo "You are person $count to enter $BirthYear"; } else { echo "Your birth year is apparently not that important"; }
-
In addition to what mac_gyver stated, I would do a simple query on the same table and see if that returns any results as it may be simply not returning any rows based on the query.
-
If you already created the file with the headers then all you need to do is append a row each time the form is submitted. You can force users to fill in all values before writing data to the file or simply enter blank data for that column. <?php error_reporting(E_ALL); ini_set('display_errors',1); // set path to file $path = __DIR__ . '\file.csv'; // open the file for appending if(($fp = fopen($path, 'ab')) === false) { exit('failed to open file'); } $posts = array(); if( ! empty($_POST['firstname'])) { $posts['firstname'] = $_POST['firstname']; } else { $posts['firstname'] = ''; } if( ! empty($_POST['lastname'])) { $posts['lastname'] = $_POST['lastname']; } else { $posts['lastname'] = ''; } if( ! empty($_POST['sex'])) { $posts['sex'] = $_POST['sex']; } else { $posts['sex'] = ''; } if( ! empty($_POST['box1'])) { $posts['box1'] = $_POST['box1']; } else { $posts['box1'] = ''; } if( ! empty($_POST['box2'])) { $posts['box2'] = $_POST['box2']; } else { $posts['box2'] = ''; } // write a data row fputcsv($fp, array_values($posts)); // close the file fclose($fp);
-
$array = array('2' => 1, 3); // remove the last element array_pop($array); // add the remaining elements $array['3'] = 3; $array['b'] = 4; $array['4'] = 2; print_r($array); Output: Array ( [2] => 1 [3] => 3 [b] => 4 [4] => 2 )
-
Same as fetching any other db record. Many fetch options. http://php.net/manual/en/function.oci-fetch-row.php while (($startd = oci_fetch_assoc($start)) != false) { // echo $startd['whatever']; }