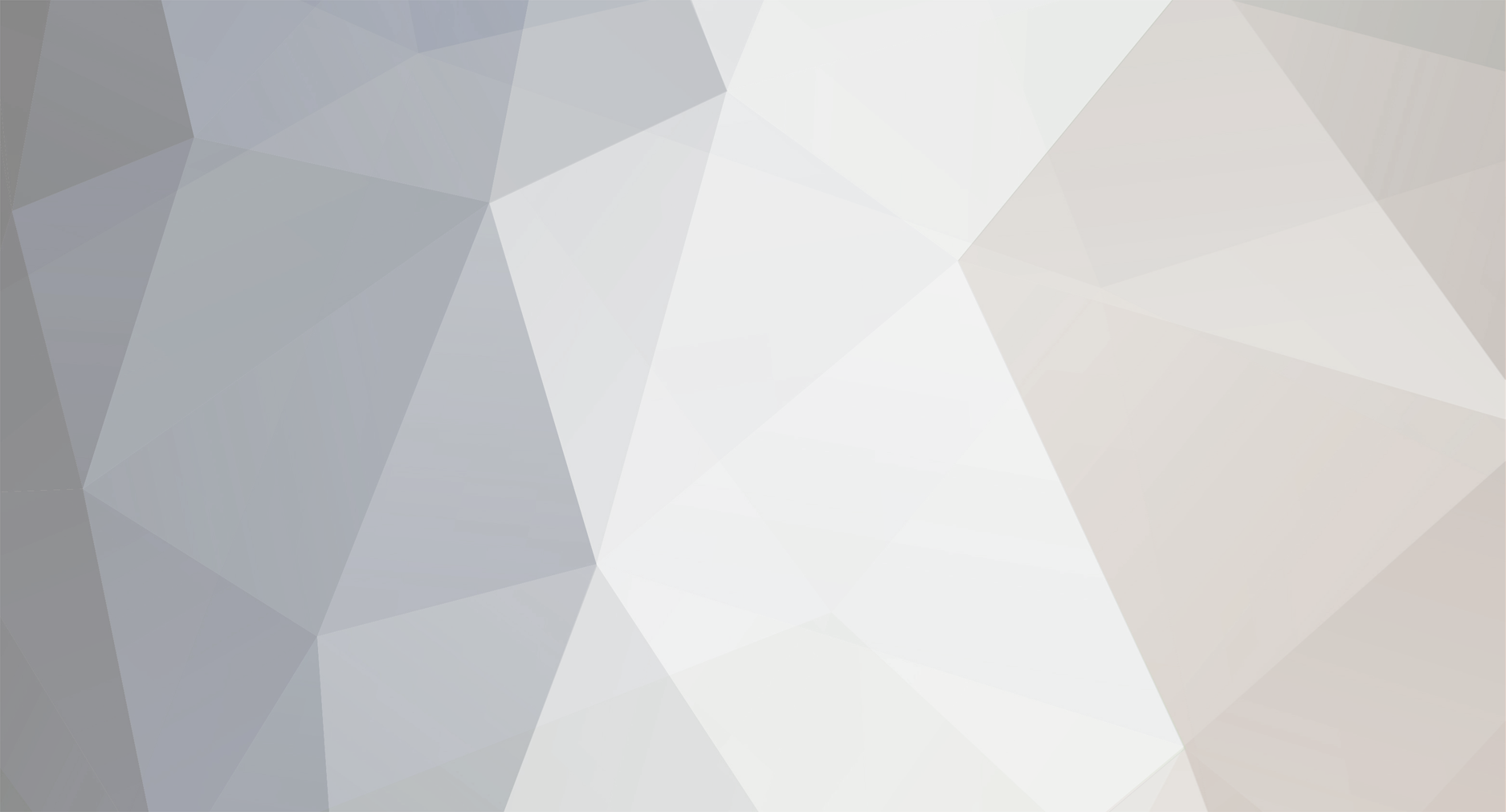
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Trying to pass arguments into function (one arg is missing)
DavidAM replied to n1concepts's topic in PHP Coding Help
Using array_shift() you are essentially passing the array('price' => '12.95','shipping' => '0.00') elements into the function. The key ('Candle Holder') is basically tossed out. Since the product is not in the array you passed in, you're not going to have it inside the function. Since the function appears to be for calculation purposes, you may not actually need it there. I might suggest that instead of this: $sub_total1 = all_products(array_shift($products)); $sub_total2 = all_products(array_shift($products)); $sub_total3 = all_products(array_shift($products)); echo "RESULTS OUTSIDE FUNCTION: ".$sub_total1."<br />"; echo "RESULTS OUTSIDE FUNCTION: ".$sub_total2."<br />"; echo "RESULTS OUTSIDE FUNCTION: ".$sub_total3."<br />"; // Add total sum for each product to define grand total for all products including shipping & taxes $grand_total = $sub_total1 + $sub_total2 + $sub_total3; you use something like this: $grand_total = 0; foreach ($products as $product => $values) { $sub_total = all_products($values); $grand_total += $sub_total; echo '<li>' . $product . ': $' . $sub_total . '<br />'; }?> <B>Total (including tax and shipping): $<?php echo number_format($grand_total, 2); ?></B> (not tested) If you absolutely need the product name inside the function, you will have to change the function definition to something like: function all_products($product, $prod_array) { and then pass it in like: $sub_total = all_products($product, $values); inside the loop. There are other alternatives, such as changing your array structure. $products = array(0 => array('product' => 'Candle Holder', 'price' => '12.95','shipping' => '0.00'), 1=>array('prodcut'=>'Coffee Table','price' => '99.50','shipping' => '0.10'), 2=>array('product'=>'lamp', 'price' => '99.50','shipping' => '0.10')); But I don't know how much effort that would be for you since you might have other code/scripts using this array. -
Hmm, I learn something new every day. At any rate, you definitely can't mix the two.
-
$dbc = @mysqli_connect (DB_HOST, DB_USER, DB_PASSWORD, DB_NAME) You are using mysqli for the connection but then using mysql (no i) functions. You cannot mix the two. Either use mysql_connect or change the others to mysqli functions. $result = mysql_query($dbc, $q); The database connection parameter is the second parameter (and it is optional). Either leave it out, or trade $q and $dbc in this function call. This is what the error message is telling you. mysql_select_db('database'); In your included script, you did not select a database. You need to select the database or qualify all of your table names in all of your queries. Usually, we select the database immediately after connecting to the server.
-
It might be easier to drop out of PHP to output that code (and cleanup the quotes): else { ?> <div id='fcontrol'> <table border='1' class ='tstyle'> <tr height ='700'><td valign='top' class='ssbg' width='120'><div id='menu'> <a href="javascript:ajaxpage('new_section.php', 'contentarea');">Add new section</a><br/><br/> <a href="javascript:ajaxpage('new_sub_section.php', 'contentarea');">Add new section to forum</a><br/><br/> <a href='index.php'>Back to forum</a><br/><br/></div></td><td class='tcontrol' width='700' valign='top'> <center><div id='contentarea'>Control Panel</div></center></td></tr> </table></div> </div> <?php } ?>
-
Statement Comparing dates then display certain records.
DavidAM replied to vmicchia's topic in MySQL Help
I think this query should give you the data you are looking for: SELECT * FROM orders AS MainWHERE Main.total < 30AND NOT EXISTS(SELECT * FROM orders AS Later WHERE Later.email = Main.email AND Later.timestamp > Main.timestamp) -
First, you should turn on error reporting and display during development. You should be getting a couple of errors with that code, and it might help determine the problem. At the beginning of the script add: error_reporting(E_ALL); ini_set('display_errors', 1); Second, you are referring to $link outside of your database connection function, but it does not exist outside of the function. I don't think that is the problem with the SELECT box, but it will be throwing errors. Add a line to the end of the function to return the link: return $link; and then capture it when you call the function (see below) I don't really see a problem with this code, which means the query is probably failing. Since the query is being executed inside of the SELECT tag, the browser may be hiding any error messages. After you add the error reporting (above), use the option in the browser to view the source of the page. See if there are any error messages inside of the SELECT tag. You might also test the result of the query execution: <select name="nation"> <?php $link = dbconnect(); // CAPTURE THE DATABASE CONNECTION $result_nation = mysql_query("SELECT * FROM nationalities"); // CHECK TO SEE IF THE QUERY SUCCEEDED // THIS IS NOT THE BEST WAY TO REPORT AN ERROR, BUT FOR TESTING IT WILL DO if (! $result_nation) { echo 'Query Failed: ' . mysql_error(); } while($row = mysql_fetch_array($result_nation)) { ?> <option value="<?php echo $row[0]; ?>"><?php echo $row[1]; ?></option> <?php } mysql_close($link); ?> </select>
-
DISTINCT just means you will not get any duplicate rows; it does not mean you will only get a single row. If you are getting more than one row in the subquery with a DISTINCT, then there are DIFFERENT latitude values for that City/State combination. You might try doing a JOIN instead of a subquery; of course, the latitude/longitude that you get will be randomly selected from the multiple entries in the second table.
-
I see a couple of issues here. 1) If those are your actual database login and password, you should change them NOW since you have just posted them on the internet. 2) There is no real need to connect to the database twice; although it appears you are using different credintials. If you are using the same login and password, I personally would connect before the first IF statement and just use the same connection for all database access. If you are using different credintials, then the code is fine. 3) This statement needs to be revised: $sql="INSERT INTO actors (Actor_ID, Actor_Name, Actor_Nation) VALUES ('$_POST[actorID]','$_POST[actorName]','$_POST[nation]')"; when you use an array element inside of double-quotes, you need to use curley braces: $sql="INSERT INTO actors (Actor_ID, Actor_Name, Actor_Nation) VALUES ('{$_POST['actorID']}','{$_POST['actorName']}','{$_POST['nation']}')"; also, you need to put the element name ( actorID ) in quotes just as you do when referencing the element anywhere else in the code. NOTE: You absolutely need to sanitize any input coming from the user that is going to the database. Use mysql_real_escape_string() to protect against SQL injections. I would, personally, rewrite the INSERT statement as: $sql= sprintf("INSERT INTO actors (Actor_ID, Actor_Name, Actor_Nation) VALUES ('%s', '%s', '%s')", mysql_real_escape_string($_POST['actorID']), mysql_real_escape_string($_POST['actorName']), mysql_real_escape_string($_POST['nation'])); 4) This code is problematic (see comments): $result_nation = mysql_query("SELECT * FROM nationalities"); $row = mysql_fetch_array($result_nation); // RETRIEVING THE FIRST ROW mysql_close($link); // CLOSING THE CONNECTION ?> a) You are retrieving the first row from the result, but you are never using it. You have a WHILE loop later that starts with retrieving the next row -- REMOVE this line entirely b) You are closing the connection to the database, but you have a while loop later trying to retrieve the data, I don't think that will work. -- MOVE this line below the free_result() later in the code 5) This code is not outputting anything for the VALUE of the OPTION tag. I suspect the WHILE is failing because you closed the database connection earlier. But if it is not, you are not echoing the value, so nothing is being put in the tag. The way I see it, with the code you have, there should be NO OPTIONS in the SELECT to choose from. <select name="nation"> <?php while($row = mysql_fetch_array($result_nation)) { ?> <option value="<?php $row[0]; ?>"><?php echo $row[1]; ?></option> <?php } ?> </select> change it to <select name="nation"> <?php while($row = mysql_fetch_array($result_nation)) { ?> <option value="<?php echo $row[0]; ?>"><?php echo $row[1]; ?></option> <?php } ?> </select> The code you have (with these corrections) should POST the value from the selected OPTION in $_POST['nation']. This is the value from the first column in your SELECT statement, it is NOT the value displayed as the text of the OPTION.
-
You're not actually incrementing $i, so the loop will never end // THIS for($i = 2; $i <= $nbrweek; $i+2) { # 52 week in one year of course // SHOULD BE THIS for($i = 2; $i <= $nbrweek; $i+=2) { # 52 week in one year of course
-
A VIEW is an object in the database, just like a TABLE is. You only have to create it once, so you don't want it in a script that will be executed repeatedly. In the script you posted, you never executed the SQL you wrote to create the VIEW. So the VIEW does not exist, so the second query, which you did execute, will fail.
-
Add it to the VALUES clause and the INSERT clause $values[] = "({$_SESSION[userid]}, '$name', '$surname', '$age')"; } $query = "INSERT INTO plus_signup (userid, name,surname,age) VALUES " . implode(', ' $values); Of course, if there is no userid column in the table, you will have to ALTER the table before the code will work.
-
Keeping track of changing multiple owners of an object.
DavidAM replied to KitCarl's topic in MySQL Help
Strictly speaking, the PersonAnimalID is not required (although, sometimes it is useful to have). You can use a primary key composed of the two fields: PersonID,AnimalID (or AnimalID,PersonID). This would only be a problem if a single person can own more than one share of the animal. Other than that, your table suggestion is correct for the "many-to-many" relationship you described - many people own many animals -
Something like this(not tested): $fs = fopen('TESTS.TXT', 'r'); while ($line = fgets($fs)) { $name = substr($line, 0, 4); $efdate = substr($line, 4, ; $exdate = substr($line, 12, ; // ... MORE LINES LIKE THAT // NOTE: for substr() the the first character of the string is at position 0 (zero) // DO SOMETHING WITH THE DATA } fclose($fs); There are other ways to do this; like file() which will load the entire file into an array of lines.
-
My first thought was something like this: SELECT user_id, marked_online FROM user_details JOIN (SELECT IF (user_id_[link1]=$loggedInUserId, user_id_[link2], user_id_[link1]) AS FriendID FROM user_friends WHERE $loggedInUserId IN (user_id_[link1], [user_id_[link2])) AS UserFriends ON user_details.user_id = UserFriends.FriendID WHERE marked_online = TRUE ORDER BY ... LIMIT ... but I don't think the inner SELECT will use any indexes, so it will not scale. Then I thought about using a UNION: (SELECT SQL_CALC_FOUND_ROWS user_id, marked_online FROM user_details JOIN user_friends ON user_id = user_id_[link1] WHERE user_id_[link2] = $loggedInUserID AND marked_online = TRUE) UNION ALL (SELECT user_id, marked_online FROM user_details JOIN user_friends ON user_id = user_id_[link2] WHERE user_id_[link1] = $loggedInUserID AND marked_online = TRUE) ORDER BY ... LIMIT ... or even combining the two: SELECT SQL_CALC_FOUND_ROWS user_id, marked_online FROM user_details JOIN (SELECT user_id_[link1] AS FriendID FROM user_friends WHERE user_id_[link2] = $loggedInUserID UNION SELECT user_id_[link2] FROM user_friends WHERE user_id_[link1] = $loggedInUserID) AS UserFriends ON user_id = FriendID WHERE marked_online = TRUE ORDER BY ... LIMIT ... I think either of the last two will use indexes on the user_friends table so performance will not degrade so badly as the table grows. Note: there should -probably- be a separate index on each of the two columns. Actually, as I think about it, I think the middle one will scale best.
-
You don't use stripslashes on the stuff coming from the database. At least I don't, and I have never had to. I have never seen the backslashes on the quotes actually IN the database. Do you have magic_quotes_gpc and/or magic_quotes_runtime turned ON? [*]If magic_quotes is on, you should stripslashes($Text) when you get the data from the form [ You should turn off magic_quotes as it has been depricated and will go away soon ] [*]Use mysql_real_escape_string($Text) when putting the data into the database [*]Use htmlspecialchars($Text) when sending the data back to the form to be edited [*]Use nl2br(htmlspecialchars($Text)) when sending the data to the page to be displayed
-
You should be able to CREATE a VIEW in the database. Then you can select from the VIEW as if it was a table. Something like this CREATE VIEW StaffList AS SELECT * FROM Staff Then select from it SELECT * FROM StaffList WHERE sex='Male'
-
PHP >> Mysql querying database with string array values
DavidAM replied to djinnov8's topic in PHP Coding Help
OR use a loop to build the WHERE clause for a single query: $query_keywords = Array ( 7 => 'business', 10 => 'home', 11 => 'depo' ); $where = ''; foreach ($query_keywords as $keyword) { if (empty($where)) $where = 'WHERE '; else $where .= ' OR '; $where .= "product_name LIKE '%$keyword%' "; $where .= "OR product_comment LIKE '%$keyword%'"; } $query = "SELECT * FROM product $where"; $result=mysql_query($query); -
Working local PHP project doesn't function on web server
DavidAM replied to craigj1303's topic in PHP Coding Help
<<Grasping at straws>> ... Were the include files saved as unicode or utf-8? - although this normally presents as a different error (I think) Is the project server a Linux server? Is it possible the files built on the WAMP (Windows) server have CR-LF line endings and the Linux server is choking on the CR? - Although FTP in ASCII mode should have converted these Did you try crossing your fingers AND your toes the very first time you tried to load the page? -
It seems to me that this happens when there are TAB characters (char(9)) in the code tags. I gather it is the difference between the OP using copy & paste to post the code vs. just typing the code or copying from an editor that has replaced tabs with spaces. It is a pain in the ... rear. There was a time when this did not happen and then there was an update to the forum software and it starting happening. Maybe it has something to do with the javascript on this container and the paste function. All I know is, it is a pain in the ... rear. The first code block below has TAB characters in it. The second code block uses SPACEs // This is just a line all by itself One tab before this line ... None here ... two here // The End // This is just a line all by itself One tab before this line ... None here ... two here // The End I just did a little test, and when I copy the code out of the first box and paste in my favorite editor, it puts one \n (newline) before the first \t (TAB) (that's in addition to the newline that is on the previous line) and TWO newlines ("\n") after EVERY TAB. Did I mention that this is a pain in the ... rear?
-
Working local PHP project doesn't function on web server
DavidAM replied to craigj1303's topic in PHP Coding Help
It says the error was in the output_fns.php file. Did you leave the openning PHP tag ("<?php") out of the file; or use a short tag ("<?")? Short tags are not a good idea and are disabled on some servers. -
Open the window first. No sense in ruining a perfectly good window.
-
These functions convert certain characters into html entities so that the browser can DISPLAY them instead of executing them. If you are sending the results to a browser, you will "SEE" the same thing that you started with. If you look at the page source (View Source in your browser) you should see the "special characters". If you sent those strings without the htmlentities(), the browser would execute that script instead of displaying it.
-
Your query failed for whatever reason. You should always check the return value from mysql_query() and act appropriately. It will return false if the query failed, then look at mysql_error() $query = mysql_query("SELECT * FROM chat_likes WHERE chat_name='{$chat}' AND user_id='{$user_id}'"); if (! $query) { // THE QUERY FAILED /* You should do something here to tell the user there is a problem and maybe provide a link back to another page or something */ trigger_error(mysql_error(), E_USER_ERROR); } while($row = mysql_fetch_assoc($query)) // line 10 I use trigger_error() rather than die() so I can leave the code in when I go to production. That way the error gets logged and the user doesn't get a blank screen.
-
Odd behaviour for a simple SELECT or is my logic wrong?
DavidAM replied to rwwd's topic in MySQL Help
AND takes precedence over OR - wrap the conditions in parenthesis: SELECT * FROM tester WHERE ( `name` = 'POSTED USER NAME' OR user_email = 'POSTED EMAIL') AND password = 'MD5 OF POSTED PASSWORD; The way you had it should have worked as long as the name was correct, since it would have been interpreted as [ name = POSTED OR (email = POSTED AND password = POSTED) ]